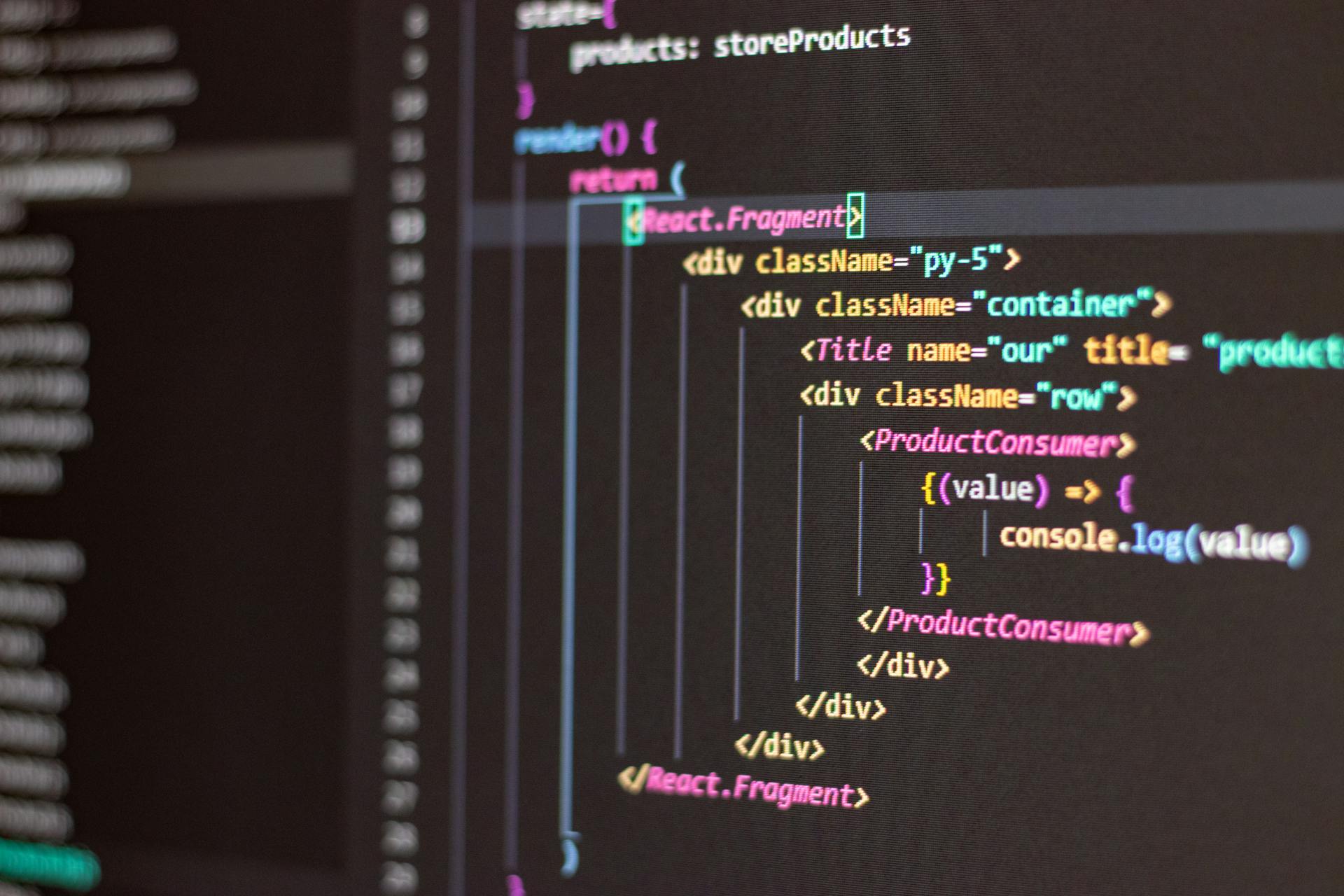
Next Js TypeScript Hooks offer a powerful way to manage state and side effects in your frontend applications.
You can use the `useEffect` hook to handle side effects, such as API calls or DOM updates, in your components.
The `useCallback` hook helps prevent unnecessary re-renders by memoizing functions.
This can be particularly useful when working with complex components or large datasets.
See what others are reading: Next Js Client Side Rendering
React Fundamentals
React hooks are functions that let you use state and other React features without writing a class.
The most commonly used hooks are: useState, useEffect, useContext, and useReducer.
These hooks make your components more functional and easier to understand.
The useState hook allows you to add state to functional components.
The useEffect hook lets you perform side effects in your components.
The useContext hook provides access to the context API, enabling you to share values between components without passing props.
The useReducer hook is an alternative to useState that's more suited for complex state management.
By using these hooks, you can take advantage of type safety and make your code less prone to errors when paired with TypeScript.
A unique perspective: Next Js React Fundamentals
React Hooks
React Hooks are a game-changer for Next.js developers using TypeScript. They allow you to use state and other React features without writing a class, making your components more functional and easier to understand.
The most commonly used hooks are useState, useEffect, useContext, useReducer, useMemo, and useCallback. These hooks make it easy to manage state, perform side effects, and optimize performance in your components.
For example, useState is used to initialize any variable as a state variable, and useEffect is used to get a callback in case of any dependencies being updated with new values. The dependency array in useEffect controls when the effect gets re-ran, and you can use it to synchronise effects to run whenever you like.
Here are some key points to keep in mind when using React Hooks:
- Always declare the state variable as const, do not use let for state variable.
- Updating state variable value is asynchronous.
- Any update on the state variable will execute the useEffect hook.
UseEffect React Explanation
The useEffect React Hook is a game-changer for handling side effects in your components. It's used to get a callback in case of any dependencies being updated with new values.
If no dependency is provided, the useEffect() hook will call every time while rendering or rerendering. This can be a problem if you're trying to optimize performance.
If an empty array is provided, like [], the useEffect() hook will run once after initial rendering. This is a great way to run some code only once when the component mounts.
Having multiple dependencies, like [count, stateVariable, stateArrayVariable], will run whenever any of those dependencies get updated. This is useful for handling complex state changes.
Don't use async on useEffect hook declaration. This can cause unexpected behavior and make your code harder to understand.
Here's a quick rundown of the most commonly used hooks, including useEffect:
The useEffect hook is particularly useful for managing complex state logic, like in the case of the useReducer hook. By using a reducer function and an initial state, you can centralize how state updates occur and make your code easier to understand.
A fresh viewpoint: State Management in Nextjs 14
The useReducer hook is similar to useState, but it's better suited for managing complex state logic. It takes a reducer function and an initial state, and it returns the current state and a dispatch function to update that state.
Here are some benefits of using useReducer:
- Centralized state management
- Complex logic
- Easier testing
Using useReducer with TypeScript ensures that your state management logic remains type-safe and maintainable.
Optimizing Performance with Hooks
Hooks are a powerful tool in React that help you optimize performance by preventing unnecessary re-renders and calculations. By using hooks like `useEffect`, `useCallback`, and `useMemo`, you can ensure that your components only re-render when necessary, resulting in a smoother user experience.
The `useEffect` hook is particularly useful for running side effects in your components, such as fetching data or setting timers. However, if you don't provide a dependency array, the effect will run after every render, which can be inefficient. To prevent this, you can provide an empty array as the second argument, which will cause the effect to only run once after the initial render.
A fresh viewpoint: Nextjs App Router Hooks
Here's a pattern you might see often for data fetching:
```
useEffect(() => {
// fetch data
}, []);
```
This will only run the effect once after the initial render, and then it will only re-run when the dependency array changes. If you have multiple dependencies, you can provide them in the array, like this:
```
useEffect(() => {
// fetch data
}, [count, stateVariable, stateArrayVariable]);
```
This will re-run the effect whenever any of the dependencies change.
Another hook that can help optimize performance is `useCallback`. This hook memoizes callback functions, ensuring that a new function reference is only created when its dependencies change. This can be particularly useful when passing callbacks to optimized child components.
Here are some benefits of using `useCallback`:
- Improved performance: By memoizing callback functions, you can prevent unnecessary re-renders and calculations.
- Clean code: `useCallback` makes your code cleaner and easier to read by avoiding inline functions or unnecessary computations.
You can use `useCallback` like this:
```
const handlePostRemove = useCallback((postId: number) => () => {
removePost(postId);
}, [removePost]);
```
This will create a new function reference only when the `removePost` function changes.
Finally, `useMemo` is another hook that can help optimize performance by memoizing expensive calculations. This can be particularly useful when dealing with large datasets or complex UI components.
Here are some benefits of using `useMemo`:
- Improved performance: By memoizing expensive calculations, you can prevent unnecessary re-renders and calculations.
- Clean code: `useMemo` makes your code cleaner and easier to read by avoiding inline functions or unnecessary computations.
You can use `useMemo` like this:
```
const posts = useMemo(() => {
// calculate posts
}, [dependencyArray]);
```
This will only re-run the calculation when the dependency array changes.
By using these hooks effectively, you can optimize performance in your React applications and create a smoother user experience. Remember to always provide a dependency array to `useEffect` to prevent unnecessary re-renders, and use `useCallback` and `useMemo` to memoize callback functions and expensive calculations.
Curious to learn more? Check out: Dependency Injection Nextjs
React Hooks: Refs
Using the useRef hook, we can access the DOM element in a component. This is especially useful when we need to update a value without triggering a re-render.
If we use useRef() in the same component, we can update the value using .current without causing a re-render. This is a convenient way to manipulate the DOM without unnecessary re-renders.
Here are some key benefits of using useRef() in the same component:
- Update values without re-rendering
- Access the DOM element
However, when we use useRef() as a child component, we need to use forwardRef and useImperativeHandle together to access the parent component's metadata. This allows us to pass actions like register and cancel button actions to the child component.
Take a look at this: Nextjs Server Actions File Upload
Skipping Default Values for createContext
Skipping default values for createContext can be a useful adjustment in our code. Kent C. Dodds suggests this approach in his article.
Providing default values for the createContext function can make our code more similar to our application's behavior, even in testing. However, this approach might not be the best choice for our tests.
To skip default values, we need to adjust our typing slightly. We can do this by using the |undefined type in the createContext function.
See what others are reading: Nextjs Code Block
Here's an example of how to do this:
We can then use the usePostsContext hook instead of calling useContext(PostsContext) every time. This hook checks if the postsContext is defined and throws an error if it's not.
Sources
- https://lazypandatech.com/blog/NextJs/61/React-hooks-explanation-with-an-example-using-NextJs-and-TypeScript/
- https://prateekshawebdesign.com/blog/using-typescript-hooks-in-nextjs-advanced-techniques
- https://news.ycombinator.com/item
- https://upmostly.com/next-js/a-developers-guide-to-using-useeffect-in-next-js
- https://wanago.io/2020/09/28/react-context-api-hooks-typescript/
Featured Images: pexels.com