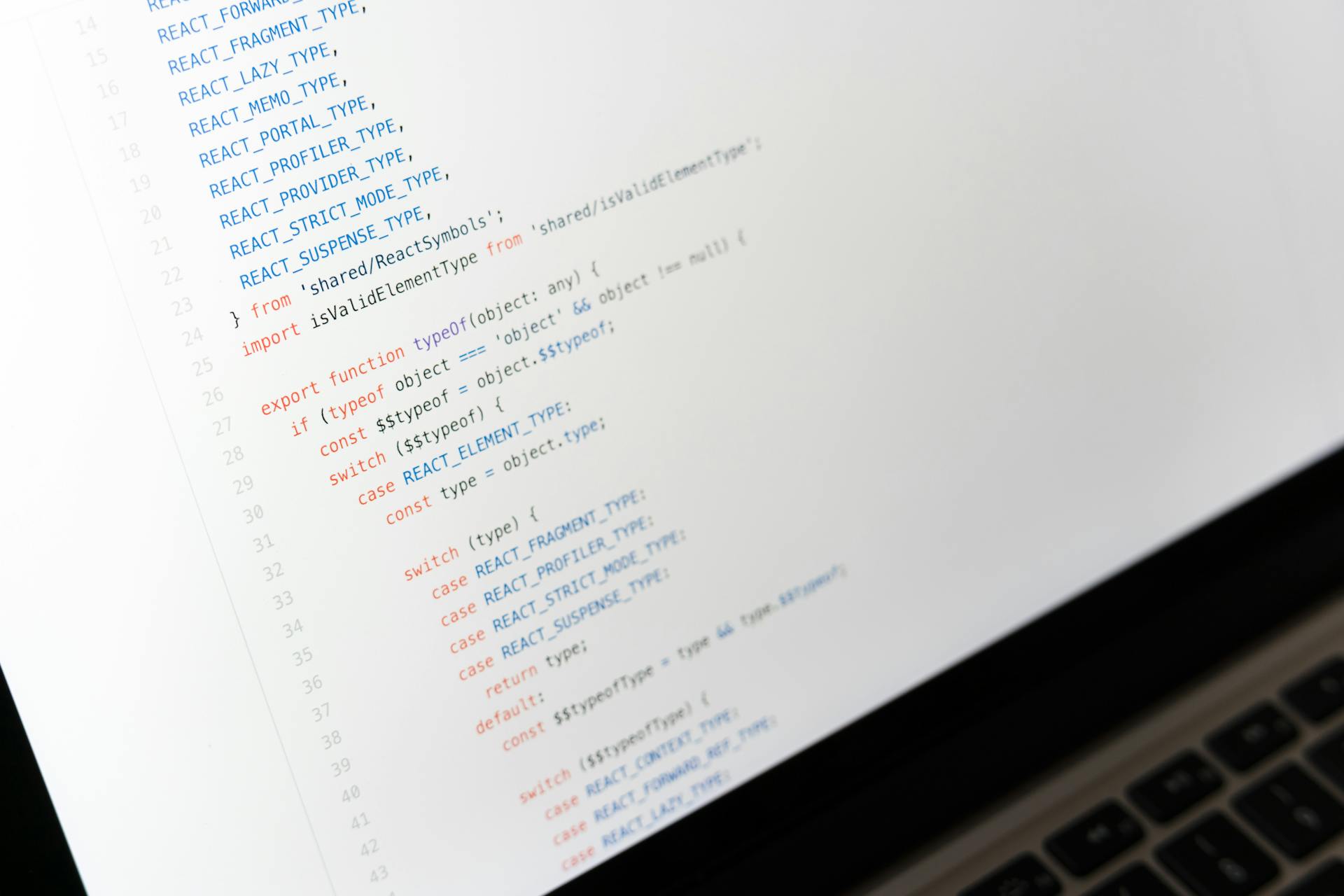
Nextjs App Router Hooks are a game-changer for developers, providing a simplified way to handle client-side routing.
With App Router Hooks, you can create reusable code for handling client-side routing, making it easier to manage complex applications.
One of the key benefits of App Router Hooks is their ability to reduce boilerplate code, allowing developers to focus on more important tasks.
By using App Router Hooks, you can simplify your code and make it more maintainable, reducing the risk of bugs and errors.
A fresh viewpoint: Nextjs Hooks
Use
The Next.js App Router Hooks allow you to tap into the App Router's lifecycle events.
You can use the useAppData hook to access the App Router's internal data, such as the current route and the app's state.
The useAppData hook returns an object with the app's data, which can be used to update the app's state or perform actions based on the current route.
With the useAppData hook, you can easily access the app's data and perform actions without having to manually manage the app's state.
Expand your knowledge: Nextjs App Route Get Ssr Data
Setting Up Next.js App Router
Setting up a Next.js app router is a straightforward process that requires a few key steps. First, you need to create a new file called `app/router.js` in the root of your project.
This file will serve as the entry point for your app router. In it, you'll define the routes for your application. The `app/router.js` file is where you'll use the `app` function from `next/router` to create your router.
To use the `app` function, you need to import it from `next/router`. This is done by adding the following line of code at the top of your `app/router.js` file: `import { app } from 'next/router';`.
The `app` function takes two arguments: the first is a function that returns a React element, and the second is an options object. The options object allows you to customize the behavior of your router.
By default, the `app` function will render the routes in the order they are defined. However, you can change this behavior by setting the `fallback` option to `true`. This will render a loading screen while the routes are being fetched.
Once you've set up your `app/router.js` file, you can use the `app` function to render your routes. This is done by calling the `app` function and passing in the route you want to render.
Take a look at this: Cookies Nextjs
Understanding Hooks
Hooks are a crucial part of React components, allowing you to reuse code and make your components more modular.
They're essentially a way to call a function after rendering a component, which means you can update the component's state without causing a re-render.
Hooks give you the ability to manage state and side effects in functional components, making them a game-changer for building complex UIs.
The `useState` hook is one of the most commonly used, and it lets you add state to a functional component with just a few lines of code.
For example, `const [count, setCount] = useState(0);` creates a state variable `count` and a function `setCount` to update it.
Hooks can be used to handle side effects like fetching data from an API or making network requests, which is essential for building interactive applications.
The `useEffect` hook is particularly useful for this, as it lets you run a function after rendering the component, and it can take an optional dependency array to control when the function runs.
Here's an interesting read: How to Update Next Js
You can also use `useCallback` to memoize a function, which means it won't be recreated on every render, and `useMemo` to memoize a value, which can improve performance by avoiding unnecessary recalculations.
These hooks are designed to be composable, meaning you can use them together to create more complex behaviors, like managing state and side effects in a single component.
Sources
- https://docs.sentry.io/platforms/javascript/guides/nextjs/
- https://auth0.com/blog/auth0-stable-support-for-nextjs-app-router/
- https://www.flightcontrol.dev/blog/nextjs-app-router-migration-the-good-bad-and-ugly
- https://adiati.com/building-portfolio-with-nextjs-migrate-to-app-router
- https://nextjs.org/docs/pages/api-reference/functions/use-router
Featured Images: pexels.com