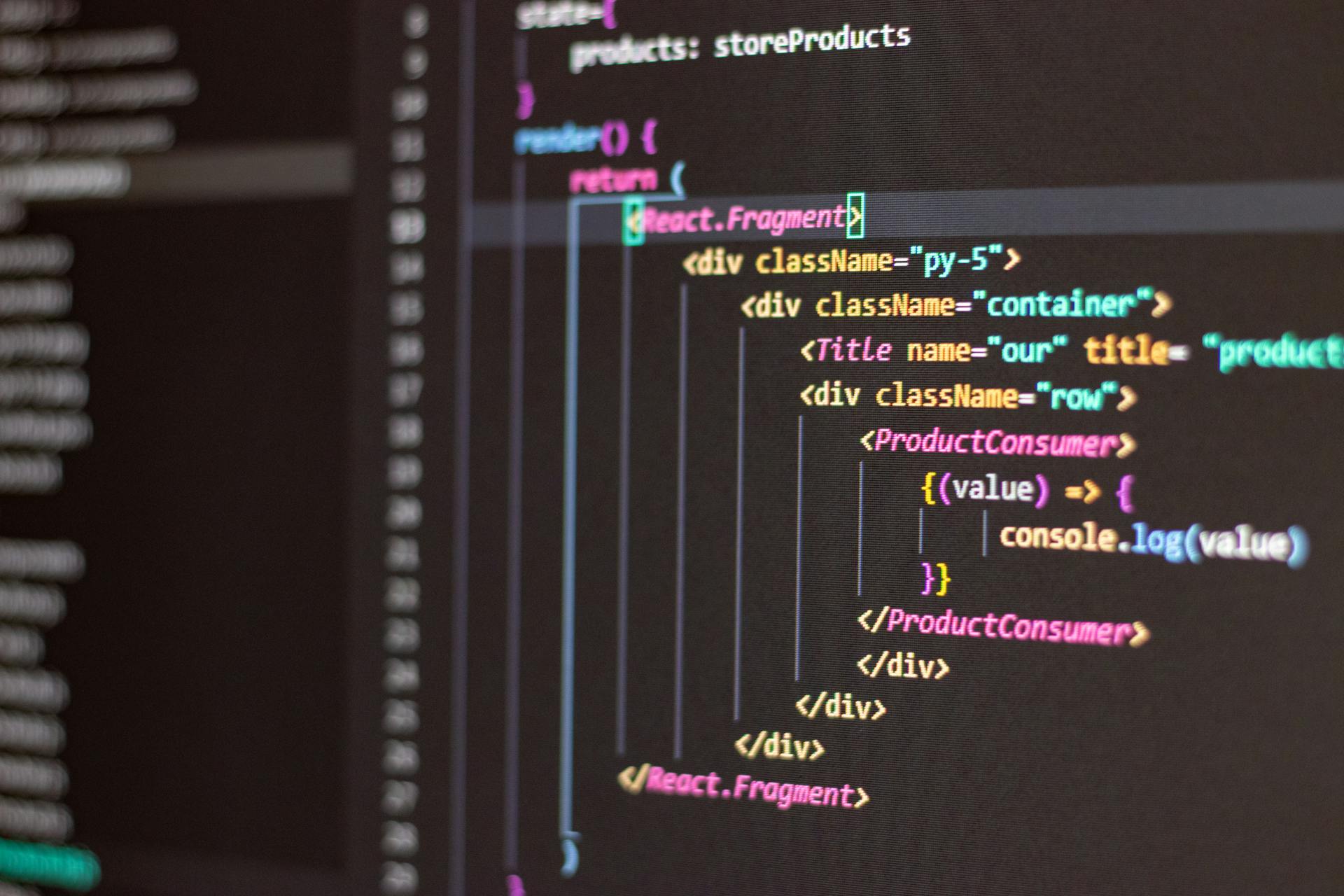
Next.js is a popular React-based framework for building server-rendered and statically generated websites and applications. It provides a lot of built-in features for handling routing, internationalization, and API routes.
To start building an ecommerce storefront with Next.js, you'll need to set up a new project using the Next.js CLI or a tool like Create Next App. This will give you a basic project structure and a few essential dependencies.
Next.js makes it easy to handle routing with its built-in Link component, which automatically generates server-side rendered URLs for you. This saves you from having to manually handle routing and URL generation.
By leveraging Next.js's built-in features and plugins, you can create a fast and scalable ecommerce storefront that meets the needs of your customers.
Setting Up Shopify Integration
To set up Shopify integration, you'll need to use the Shopify Storefront API, which allows your custom frontend to talk to Shopify's backend. This API enables you to make API calls to Shopify and access product data and other services like the checkout page.
You'll need to install graphql-request, a minimal client for sending GraphQL requests, to make GraphQL requests to Shopify. To do this, run the command to install it in your terminal.
To fetch products from Shopify, you'll need to create a GraphQL client instance and define a query for fetching products. You can do this by importing GraphQLClient and gql from graphql-request and assigning the Storefront access token and shop URL to variables.
Here are the steps to create a GraphQL client instance and define a query for fetching products:
- Import GraphQLClient and gql from graphql-request.
- Assign the Storefront access token and shop URL to variables.
- Create a new function called getProducts and define the query for fetching products from Shopify.
You can now call the getProducts function to fetch products from Shopify. To fetch a single product, you'll need to create another function called getProduct that accepts a product ID as an argument.
Creating the Ecommerce Platform
To create a Next.js ecommerce platform, start by bootstrapping a new application with the Next.js create-next-app CLI.
This process is straightforward and can be completed in a few steps. You can then add and style a grid of products with images in your Next.js React app.
Adding products to your online store involves configuring products in the Stripe dashboard. This is a crucial step in setting up your ecommerce platform.
You'll also need to dynamically manage a grid of products in your online store using a JSON document. This allows you to easily update your product offerings.
Here are the key steps to create a Next.js ecommerce platform:
- Create a new React application with the Next.js create-next-app CLI
- Add and style a grid of products with images in a Next.js React app
- Add and configure products in the Stripe dashboard for an online store
- Dynamically manage a grid of products in an online store with a JSON document
Once you've completed these steps, you can host and deploy your Next.js React app on Vercel imported from GitHub.
Shopping Cart Functionality
Implementing shopping cart functionality is a crucial aspect of any e-commerce platform, and Next.js makes it relatively easy. You can store cart items in a context or state, allowing users to add, remove, and update items.
A key feature of any shopping cart is the ability to add products to it. You can create a function that adds a product to an existing cart, making it easy for users to add items they're interested in purchasing.
To manage shopping cart state, you can use React state management or the React Context API. This allows you to store and load cart state from local storage, persisting cart data even when the page reloads.
Here are some ways to manage shopping cart state:
- Create a Shopping Cart with the useState React Hook to Manage Product Quantity and Total
- Create a Custom React Hook to Manage Cart State
- Use the React Context API to Globally Manage Cart State in a Next.js App
- Store and Load Cart State from Local Storage to Persist Cart Data When Reloading the Page
Shopping Cart Management
Shopping Cart Management is a crucial aspect of any e-commerce platform, allowing users to add, remove, and update items with ease.
Adding an item to the cart is a fundamental function that creates a new cart and adds a product to it. This is the starting point for any shopping cart functionality.
To manage shopping cart state, you can use the useState React Hook to store cart items and manage product quantity and total. This is a simple yet effective way to keep track of cart data.
A custom React Hook can also be created to manage cart state, providing a more structured approach to cart management. This can be especially useful for complex e-commerce applications.
The React Context API can be used to globally manage cart state in a Next.js app, allowing cart data to be shared across components. This is a powerful feature that can simplify cart management.
To persist cart data when reloading the page, you can store and load cart state from local storage. This ensures that cart data is retained even after the page is refreshed.
Here's a summary of the ways to manage shopping cart state:
- Manage local state with React Hooks
- Manage global state with React Context
- Purchasing flow with Stripe Checkout
Retrieving The Cart
You can retrieve the cart by adding a function named retrieveCart in utils/shopify.js. This function will make a query to Shopify to fetch the cart data.
The retrieveCart function will use the cart ID to query for the IDs of the products in the cart and the total estimated cost. This data will be retrieved from Shopify using the Storefront API.
Here's a step-by-step guide to implementing the retrieveCart function:
1. Create a new function named retrieveCart in utils/shopify.js.
2. Add a query to retrieve the cart data from Shopify using the cart ID.
3. Use the GraphQL client instance to make the request to Shopify.
4. Return the cart data, including the product IDs and total estimated cost.
By following these steps, you'll be able to retrieve the cart data from Shopify and display it in your application.
Payment and Checkout
To set up a payment and checkout system for your Next.js e-commerce store, you'll need to integrate Stripe Checkout. This can be done by configuring a Stripe Checkout Domain for Client-Only Integration.
You'll also need to add a Stripe API Key as an Environment Variable in Next.js & Vercel. This will allow you to use Stripe's payment processing capabilities in your store.
To integrate Stripe Checkout into your Next.js store, you'll need to create a checkout endpoint by adding a checkout folder within the api folder. This endpoint will accept POST requests and create a checkout session for the customer, returning the session URL.
Here are the key steps to integrate Stripe Checkout into Next.js:
- Configure a Stripe Checkout Domain for Client-Only Integration
- Add a Stripe API Key as an Environment Variable in Next.js & Vercel
- Integrate Stripe Checkout to Purchase Products in Next.js with Stripe @stripe/stripe-js Cl
By following these steps, you'll be able to set up a working checkout flow for your Next.js e-commerce store.
Accept Payments & Sell with Stripe
To build a fully custom e-commerce store, you'll want to use modern tools like React and Next.js. With React, you can create flexible and customizable components that follow modern best practices.
React Hooks will help you manage local component state, while React Context will give you clear and cohesive shared state.
You can deploy your custom store to Vercul, the platform behind Next.js, and even make it portable to deploy to other platforms.
To integrate Stripe Checkout into Next.js, you'll need to configure a Stripe Checkout Domain for Client-Only Integration, add a Stripe API Key as an Environment Variable, and integrate Stripe Checkout to Purchase Products using the @stripe/stripe-js library.
Here are the key steps to integrate Stripe Checkout:
• Configure a Stripe Checkout Domain
• Add a Stripe API Key
• Integrate Stripe Checkout to Purchase Products
By following these steps, you'll have a working checkout flow in your own dynamic eCommerce store.
To implement a Stripe payment checkout in Next.js, you'll need to create a Stripe account, install the Stripe Node.js SDK, and create a checkout endpoint.
You can use a test mode account for this tutorial and copy your secret key from the API keys menu.
Here's a summary of the key steps to implement Stripe payment checkout:
1. Create a Stripe account
2. Install the Stripe Node.js SDK
3. Create a checkout endpoint
4. Configure the cancel_url and success_url
5. Send the customer's cart to the checkout endpoint
By following these steps, you'll be able to add a Stripe payment checkout to your Next.js application.
JSON Validation with Serverless API
To validate your products and enable a seamless checkout experience, you'll want to implement JSON validation using Next.js serverless API. This technique allows for more complex scenarios, such as returning product information in a JSON file.
You can start by configuring static API routes, which will enable you to return a JSON file containing your product list. To do this, create a new folder called products inside the API folder, and add an index.ts file with the following content.
If you now visit https://localhost:3000/${YOUR_PORT}, you'll get a JSON file containing your product list. This is a great way to validate your products and ensure everything is working as expected.
To validate individual products, you'll need to add a new file called [productId].ts to the products folder. This file should contain the following code, which uses a special syntax to tell Next.js that [productId] is a dynamic parameter.
Notice the brackets around [productId], which are used to indicate a dynamic parameter. With this in place, you can visit /api/products/ONE_OF_YOUR_PRODUCTS_ID to get the JSON information of one of your products.
Database and Storage
To set up a database for your Next.js e-commerce app, start by creating a new database in Appwrite and naming it, for example, "novu store". Then, create a products collection to hold the lists of products within your application.
This collection should have attributes for name, price, and image. You can update the permissions to allow every user to perform CRUD (Create, Read, Update, Delete) operations, but you may want to change this later to ensure only authenticated users can make changes.
To keep your credentials safe, copy your project, database, and collection IDs into an .env.local file. This file will allow you to reference each value from its environment variables.
For Appwrite Storage, create a new bucket to hold all your product images. Set the acceptable file formats to .jpg and .png, as you'll be uploading images. Finally, copy your bucket ID into the .env.local file.
By setting up your database and storage properly, you'll be able to efficiently manage your product data and images. This will make it easier to create, retrieve, and delete products from your app.
Notifications and Workflows
You can send in-app and email notifications with Novu, a unified API for sending notifications through multiple channels, including In-App, Push, Email, SMS, and Chat.
To add Novu to your application, install the required Novu packages and create an account on Novu, setting up a primary email provider like Resend.
When users make a purchase, they will receive a payment confirmation email, and the admin user also receives an in-app notification, all thanks to Novu's unified API.
You can create notification workflows with Novu by building a code-first workflow engine that enables you to create notification workflows within your codebase.
Include the script in your package.json file to give React Email access to the email templates located within the project, and create an emails folder containing an email.tsx file with a customizable email template using React Email.
The Novu notification workflow named novu-store accepts a payload containing the email subject, message, the customer’s name, and the total amount, and has two steps: in-app and email notification.
To sync your workflow with the Cloud, run npx novu-labs@latest echo in your terminal, and copy the generated link along with your Echo API endpoint into the Echo Endpoint field, then deploy the changes.
You can create an endpoint that sends the email and in-app notifications when a user makes a payment, accepting the customer’s email, name, and total amount paid, and triggering the Novu notification workflow to send the required notifications after a payment is successful.
With Novu, you can integrate email, SMS, and chat template and content generators, such as React Email and MJML, into Novu to create advanced and powerful notifications.
The endpoint for sending email and in-app notifications after a payment is successful can be created by copying the code snippet into the api/send route file.
Frequently Asked Questions
Is NextJS Commerce free?
NextJS Commerce is built on top of free, open-source technologies, making it a cost-effective solution for e-commerce platforms. However, its pricing model and any additional costs should be reviewed for a complete understanding of its affordability.
Does Shopify use NextJS?
Shopify integrates with Next.js to leverage the best tools for frontend and backend ecommerce, but it does not use Next.js as its primary framework. Learn how to create a Next.js application using the Shopify GraphQL API.
What is NextJS Commerce?
NextJS Commerce is a high-performance ecommerce application built with Next.js App Router, utilizing server-rendered React components and advanced features for a seamless shopping experience. Explore the code, demo, and release notes for Next.js Commerce v1.
Are NextJS developers in demand?
Yes, NextJS developers are in high demand due to the framework's cutting-edge features and ability to address critical business needs. Companies are actively seeking skilled NextJS developers to stay competitive in the rapidly evolving web development landscape.
Sources
- https://bejamas.io/hub/guides/how-to-build-an-e-commerce-storefront-with-next-js-and-shopify
- https://medium.com/@mail_18109/building-a-custom-e-commerce-platform-with-next-js-a-step-by-step-approach-6268cfb0c89e
- https://snipcart.com/blog/next-js-ecommerce-tutorial-example
- https://egghead.io/projects/create-an-ecommerce-store-with-next-js-and-stripe-checkout
- https://novu.co/blog/building-an-e-commerce-store-with-nextjs/
Featured Images: pexels.com