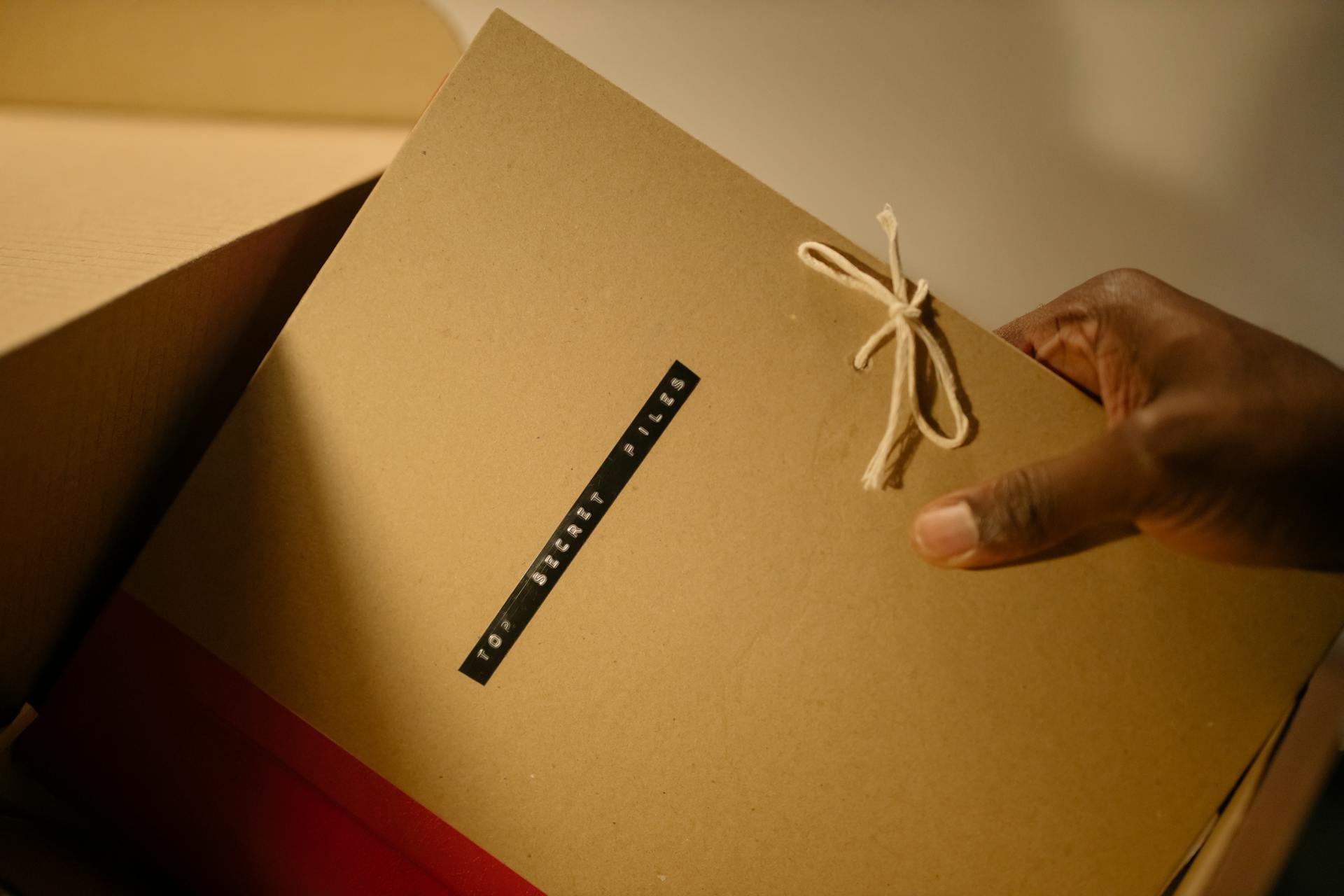
To get started with the Dropbox JS SDK, you'll need to create a Dropbox developer account. This will give you access to the Dropbox API and allow you to create an app.
You'll then need to create an app on the Dropbox Developer Portal, which will give you a Client ID and Client Secret. These are required for the OAuth flow.
The OAuth flow is a two-step process that involves redirecting the user to the Dropbox authorization page and then back to your app with an authorization code. This code can then be exchanged for an access token.
With the access token, you can make API calls to Dropbox to perform actions such as listing files and folders, creating new files, and more.
Consider reading: Do I Need Dropbox
Project Setup
To set up a Dropbox JS project, you'll need to create a new project folder and initialize a new Node.js project using npm. This will create a `package.json` file that outlines the project's dependencies and scripts.
On a similar theme: How Do You Set up a Dropbox Account
Dropbox JS is a JavaScript library that allows you to interact with the Dropbox API, so you'll need to install it by running `npm install @dropbox/js` in your project folder.
You can then import Dropbox JS into your project by requiring it in your JavaScript file, like `const { Dropbox } = require('@dropbox/js');`.
The `Dropbox` object provides several methods for interacting with the Dropbox API, including `getAccountInfo` and `listFolder`.
A unique perspective: Install Dropbox on Ubuntu
OAuth Flow
To start the OAuth process, we form a URL that includes the base URL of the Dropbox OAuth page, client ID, redirect URL, and response type. The client ID is obtained from the app settings, while the redirect URL is one of the configured settings.
We then use the Dropbox JavaScript SDK to obtain the authorization URL and redirect the user to that address. This handles multiple users by generating a random state using the crypto library and saving it in cache for a few minutes.
The user is sent back to the redirect URL along with the state string and a code, which is then used to exchange for a token using the Dropbox JavaScript SDK. The token received is saved within the current session, allowing users to bypass authorization on subsequent visits.
Take a look at this: Dropbox Oauth
Getting Client Keys
To get started with OAuth flow, you'll need to obtain client keys for the API you're working with. Your app key and secret are available in settings.
These keys are essential for authenticating your app with the API.
You can find your app key and secret in the settings area of your app.
Now that you have your client keys, you can move on to creating a service to work with the API.
Using a library like Dropbox SDK can simplify the process of interacting with the API.
A unique perspective: What Is the Dropbox App
OAuth Code Flow
To implement an OAuth authorization code grant flow, we need to check if the user's session already has a token stored in it. If it does, we can use the token directly to access resources from Dropbox.
We generate a random state using the crypto library and save it in cache for a few minutes with the state as the key and the session id as the value. This helps handle multiple users as each user will have a different state and session id.
If the user takes too long to validate credentials and authorize the app with Dropbox, the cache will expire, adding an extra layer of security.
To implement this flow, we need to add two routes to /routes/index.js. We can simply replace the whole file with the following code.
The user will be sent back to the redirect URL along with the state string we sent and a code. We validate the state by checking that there was a session id for it and use the Dropbox JavaScript SDK to exchange the code for a token.
Finally, the token received will be saved within the current session so next time the user reaches the home page /, they will be able to use Dropbox resources as long as their token is still valid, bypassing the need for authorization.
Using Dropbox API
To use the Dropbox API, you'll need to have a Dropbox account and sign up for a free account if you don't already have one.
You'll then need to create an app in the Dropbox App Console, choosing the Dropbox API and App Folder access type. This will allow you to access the API and its features.
To enable additional users to be authorized, you'll need to click on the button to do this in the settings page of your app. This is an important step if you want users different from the owner of the app to be able to access the API.
You can also customize scopes in the OAuth app authorization flow, which involves pre-registering a redirect URI in the Dropbox admin console. For development purposes, you can use localhost as the only permitted http URI.
Using API
Now that we have a token, we can create a helper to get an authorized client for the Dropbox API.
To get started, we need a token, which we can obtain by following the steps outlined in the previous section.
With a token in hand, we can now use the Dropbox API to perform various tasks, such as getting the current user's information.
Getting the current user's information should look like the following example.
Using the Dropbox API, we can also perform other tasks, such as retrieving files and folders, but we'll get to that later.
We can use the API to get an authorized client, which will allow us to make requests to the Dropbox API.
Intriguing read: How to Set up a Dropbox
Downloading a File
Downloading a File is a straightforward process using the Dropbox JavaScript SDK. You'll use the filesDownload method to get a representation of the file, which includes general information about the file itself.
The file representation will have a key named fileBlob of type Blob, which might seem like what you're looking for, but it's actually not directly usable.
The fileBlob key has a method named .text() that returns a promise that resolves to a string, which is exactly what you want, the textual contents of the file. However, this method doesn't work in some browsers, including Safari.
Instead, you should use the FileReader method readAsText(), which has better browser support and can be wrapped in a promise to make it work seamlessly.
Sources
- https://dropbox.tech/developers/oauth-code-flow-implementation-using-node-js-and-dropbox-javascript-sdk
- https://developer.mozilla.org/en-US/docs/Web/API/File_API/Using_files_from_web_applications
- https://stackoverflow.com/questions/56575928/how-to-upload-a-file-to-dropbox-using-js-sdk
- https://www.linkedin.com/pulse/how-set-up-dropbox-api-tanya-stoyko
- https://blog.jim-nielsen.com/2020/transferring-text-files-with-dropbox-js-sdk/
Featured Images: pexels.com