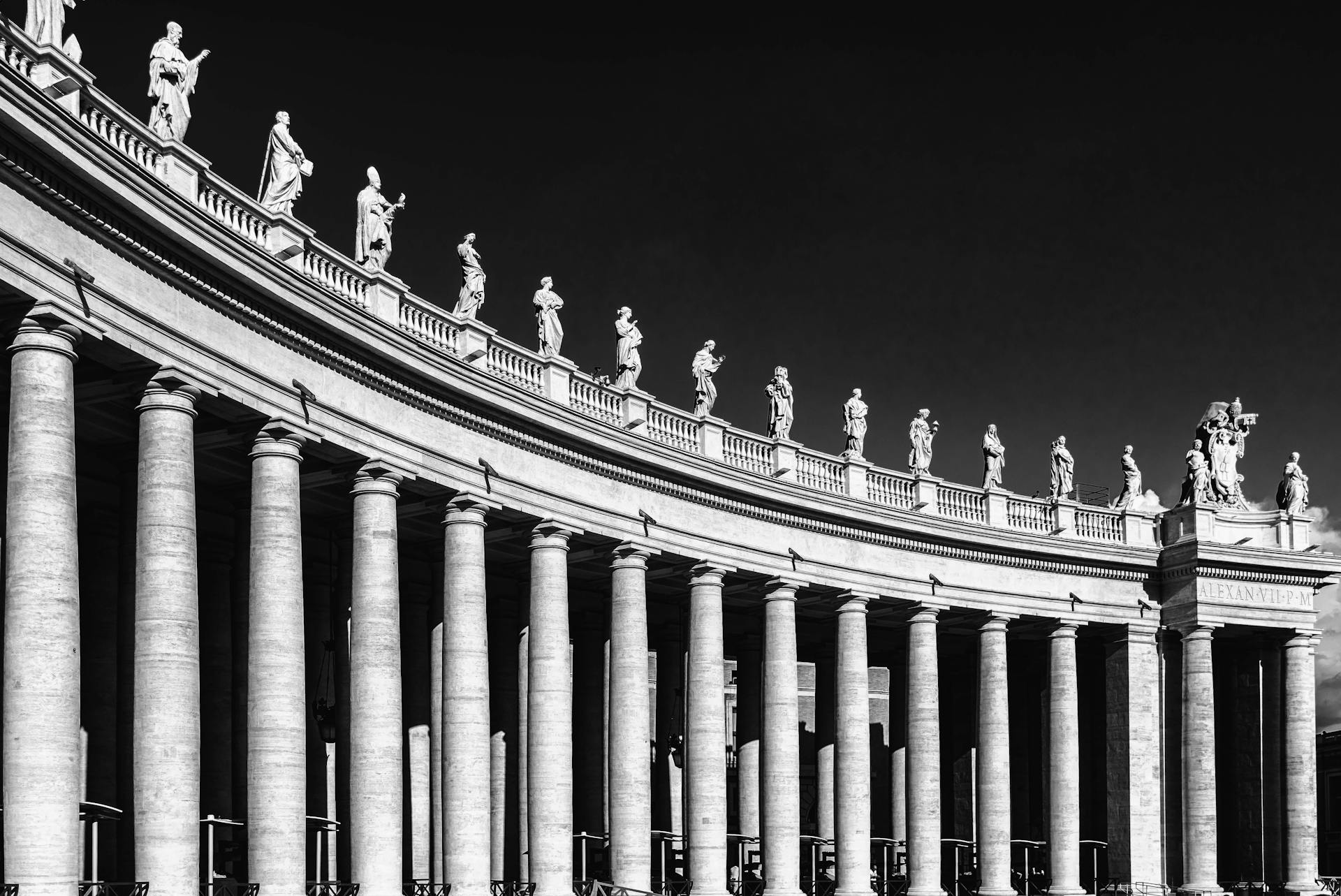
Flexbox is a game-changer for layout design, and creating a 2-column layout is one of its most popular uses. To set up a basic 2-column layout, you'll want to use the display property set to flex on the container element.
The flex-direction property is crucial here, as it determines the main axis of the layout. By default, it's set to row, which means the items will be laid out horizontally. To create a 2-column layout, you'll want to set it to column.
Flex items in a 2-column layout can be evenly spaced using the justify-content property. Setting it to space-between will create a layout where the items are evenly spaced between the two columns. This is achieved by setting the container's width to a specific value, such as 400px, and then using the justify-content property with the space-between value.
The flex-basis property can be used to set the initial width of each flex item in the layout. By setting it to a specific value, you can control the size of each column.
Flexbox Basics
Flexbox is a whole module with a lot of properties that involve setting things on the container (parent element) and the children (flex items). The main axis of a flex container is the primary axis along which flex items are laid out.
Flex items will be laid out following either the main axis or the cross axis. The main axis is not necessarily horizontal; it depends on the flex-direction property.
The main-start and main-end positions refer to where the flex items are placed within the container. The main size of a flex item is either its width or height, whichever is in the main dimension.
The cross axis is perpendicular to the main axis and its direction depends on the main axis direction. Flex lines are filled with items and placed into the container starting on the cross-start side of the flex container and going toward the cross-end side.
Here's a quick summary of the main and cross axes:
- Main axis: primary axis along which flex items are laid out
- Main-start | main-end: where flex items are placed within the container
- Main size: width or height, whichever is in the main dimension
- Cross axis: perpendicular to the main axis
- Cross-start | cross-end: where flex lines are filled with items and placed into the container
- Cross size: width or height, whichever is in the cross dimension
Layout and Alignment
You can align items on the cross-axis using the align-items and align-self properties. The align-self property is applied to individual items, while the align-items property is applied to the flex container.
The initial value of align-self is stretch, which is why flex items in a row stretch to the height of the tallest item by default. You can change this by adding the align-self property to any of your flex items.
To align an item on the cross-axis, use any of the following values: flex-start, flex-end, center, stretch, or baseline. The space available for alignment will depend on the height of the flex container, or flex line in the case of a wrapped set of items.
Here are the possible values for the align-self property:
- flex-start
- flex-end
- center
- stretch
- baseline
The align-items property can be used to set all of the individual align-self properties as a group.
Flex-Grow
Flex-grow is a property that allows a flex item to grow if necessary, taking up a specified proportion of the available space inside the flex container.
It accepts a unitless value that serves as a proportion, dictating how much space the item should occupy.
If all items have flex-grow set to 1, the remaining space in the container will be distributed equally to all children.
This is useful for creating layouts where you want all items to be roughly the same size, but with some flexibility to accommodate varying content.
For example, if one child has a value of 2, that child would take up twice as much of the space as either one of the others.
Gap, Row-Gap, Column-Gap
The gap property is a powerful tool for controlling the space between flex items. It applies this spacing only between items and not on the outer edges.
The gap property can be thought of as a minimum gutter, as if the gutter is bigger somehow, then the gap will only take effect if that space would end up smaller.
Gap works in grid and multi-column layout as well as flexbox, making it a versatile property to use in various design scenarios.
Preventing Shrinking
Setting flex-shrink to 0 allows elements to maintain their shape even when the container gets narrow.
This is especially useful for SVG icons and shapes that become distorted into ovals as the container narrows.
By setting flex-shrink to 0, we essentially opt out of the shrinking process altogether, treating flex-basis or width as a hard minimum limit.
This is demonstrated in a simplified example where two circles remain circular even as the container gets narrower.
The full code for this demo shows how to achieve this effect.
Cross-Axis Alignment
The align-self property is applied to individual items, allowing you to change the alignment of a specific child along the cross axis. This property is used to override the align-items property, which sets the alignment for all children at once.
You can use the align-self property to set the alignment of a single item to one of the following values: flex-start, flex-end, center, stretch, or baseline. The initial value of align-self is stretch, which is why flex items in a row stretch to the height of the tallest item by default.
The space available for cross-axis alignment depends on the height of the flex container. If you want to change the alignment of a single item, simply add the align-self property to that item and set its value to one of the allowed options.
Here are the allowed values for the align-self property:
- flex-start
- flex-end
- center
- stretch
- baseline
The align-items property can be applied to the flex container to set all of the individual align-self properties as a group. This can be a convenient shorthand, but keep in mind that it will override any individual align-self properties that have been set.
Flexbox Shorthand
Flexbox Shorthand is a convenient way to set multiple properties at once. It's a shorthand for flex-direction and flex-wrap properties.
You can use the flex-flow shorthand to set the direction of your flex items. For example, to set flex-direction to column, you can use flex-flow: column.
Flex-flow also allows you to control whether items wrap or not. To set flex-direction to column and allow items to wrap, you can use flex-flow: column wrap.
Main Axes
The main axis is set by your flex-direction property, and it's the direction in which flex items move as a group.
If you set flex-direction to row, the main axis runs along the row, and if you set it to column, the main axis runs along the column.
The cross axis runs in the other direction to the main axis, so if flex-direction is row, the cross axis runs along the column.
You can control the cross axis by moving items individually or as a group to align them against each other and the flex container.
Wrapped flex lines can be treated as a group to control how space is assigned to them.
Frequently Asked Questions
How to divide div into 2 columns?
To divide a div into 2 columns, use the display: flex property and set the width of each column to 50% of the container's width. This creates two equal columns that take up half the container's space.
How to divide flex into two equal columns?
To divide flex into two equal columns, set both sides to flex: 1 and a flex-basis of 0. This will create two columns of equal size, growing and shrinking together.
Featured Images: pexels.com