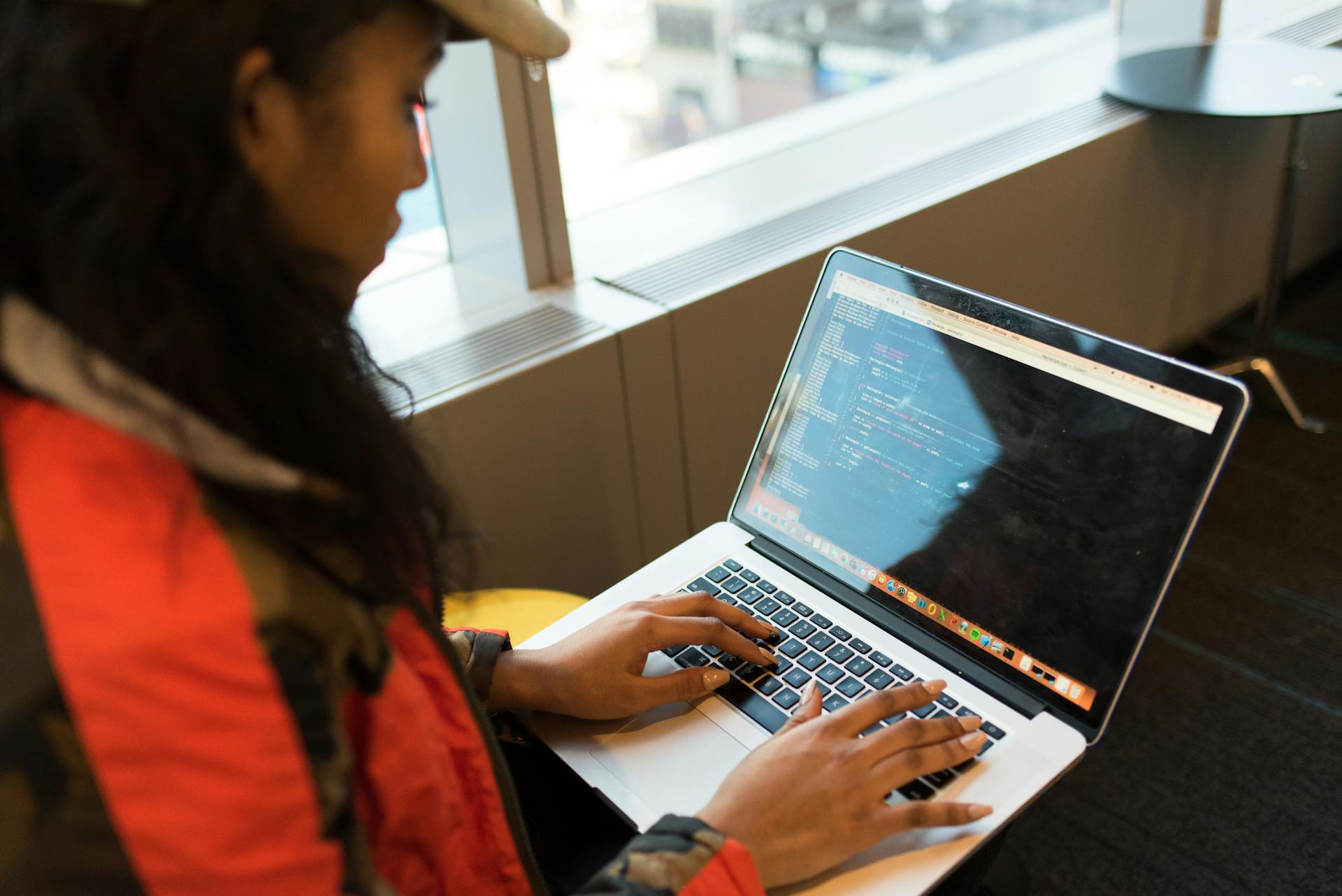
Google's coding interviews are notoriously tough, but with the right preparation, you can crack them. To succeed, you need to have a solid grasp of data structures and algorithms.
Understanding the fundamentals of data structures such as arrays, linked lists, stacks, queues, trees, and graphs is crucial. These data structures are the building blocks of many algorithms.
The key to mastering Google's coding interviews is to practice, practice, practice. You can start by solving problems on platforms like LeetCode, HackerRank, and Google's own interview practice portal.
Data Structures
Data structures are a crucial part of Google's coding interviews, and understanding them is essential to acing the tech giant's challenges. Data structures provide a way to organize and store data efficiently, and they come in various forms, such as arrays, linked lists, stacks, queues, trees, and graphs.
Some fundamental data structures include arrays, linked lists, stacks, queues, trees, and graphs. Arrays are a collection of elements, each identified by an index or a key. Linked lists are a data structure where each element points to the next one, forming a chain. Stacks and queues are linear data structures that follow the Last-In-First-Out (LIFO) and First-In-First-Out (FIFO) principles, respectively.
Here are some key data structures you should be familiar with:
- Arrays: A collection of elements, each identified by an index or a key.
- Linked Lists: A data structure where each element points to the next one, forming a chain.
- Stacks: A linear data structure that follows the Last-In-First-Out (LIFO) principle.
- Queues: A linear data structure that follows the First-In-First-Out (FIFO) principle.
- Trees: A hierarchical data structure with a root element and child elements.
- Graphs: A collection of nodes connected by edges.
Arrays
Arrays are a fundamental data structure in computer science. They are a collection of elements, each identified by an index or a key.
To master arrays, you should understand indexing and accessing elements. This involves knowing how to retrieve and modify elements in an array.
Arrays are also used in various operations like insertion, deletion, and searching. You should learn common array operations to efficiently manage data.
Arrays are crucial in coding interviews, particularly at Google. You'll need to be well-versed with arrays to solve array-based questions. This includes knowing basic programming constructors like recursion, loops, and fundamental operators.
Here are some common array operations:
- Insertion: Adding a new element to an array.
- Deletion: Removing an element from an array.
- Searching: Finding a specific element in an array.
Arrays can be used to solve various problems, such as finding the duplicate number in an array or reversing an array in place. You can also use arrays to find the largest and smallest number in an unsorted integer array.
Arrays are a fundamental data structure in computer science, and mastering them is essential for coding interviews. With practice and understanding, you can efficiently use arrays to solve complex problems.
Hash Tables
Hash tables are a fundamental data structure in computer science, and mastering them is crucial for any programmer. They provide efficient data retrieval using key-value pairs.
Hash tables rely on hashing, a technique that maps data to a fixed-size array, allowing for efficient data retrieval. This technique also involves collision resolution techniques to handle duplicate keys.
To implement a basic hash table, you need to understand how to perform operations like insertion, deletion, and retrieval. This involves learning how to handle collisions and optimize the hash function for efficient data retrieval.
Here are the key skills to master hash tables:
- Understand the concept of hashing and collision resolution techniques.
- Learn how to implement a basic hash table.
- Practice solving problems involving hash tables.
Trie (Prefix Tree)
A trie, also known as a prefix tree, is a data structure that stores strings.
Each node in a trie represents a single character, and paths from the root to the leaves represent words or prefixes.
Tries are particularly useful for handling prefix-based searches efficiently, such as in autocomplete systems.
In these scenarios, a trie can perform better than other data structures, like hash maps, when multiple prefix searches are required.
I've seen candidates in coding interviews underestimate the power of tries, but they're definitely worth considering for the right problems.
Skip List
A skip list is a data structure that combines elements of a binary search tree and a linked list.
Its main purpose is to enhance the performance of operations such as search, insert, and delete.
It's underutilized during coding interviews because it's less commonly taught, with alternatives like balanced trees and hashing stealing the spotlight.
Google tends to test knowledge on scalable algorithms, where a skip list can be a unique approach in concurrent programming, especially for lock-free algorithms.
Skip lists are a great way to improve the efficiency of operations in certain scenarios, and it's worth brushing up on them if you're preparing for a coding interview.
Sorting and Searching
Sorting and searching algorithms are essential building blocks of Google's data structure and algorithm. Sorting algorithms are used to arrange elements in a specific order, such as quicksort, merge sort, and bubble sort.
These sorting algorithms can be used to organize large datasets in a way that makes it easy to access and manipulate the data. I've seen this in action when working with large datasets, where a well-implemented sorting algorithm can make a huge difference in performance.
Some common sorting algorithms include quicksort, merge sort, and bubble sort. Here are a few examples:
- Quicksort: A divide-and-conquer algorithm that sorts an array by selecting a pivot element and partitioning the other elements into two sub-arrays.
- Merge sort: A divide-and-conquer algorithm that sorts an array by dividing it into smaller sub-arrays and merging them back together in sorted order.
- Bubble sort: A simple algorithm that sorts an array by repeatedly swapping adjacent elements that are in the wrong order.
Searching algorithms, on the other hand, are used to find a specific element in a data structure, such as binary search and linear search. These algorithms can be used to quickly locate a specific piece of data in a large dataset.
Sorting
Sorting is a fundamental concept in computer science that involves arranging elements in a specific order. This can be done using various techniques, such as quicksort, merge sort, and bubble sort.
Quicksort is a popular sorting algorithm that works by selecting a pivot element and partitioning the array around it. Merge sort, on the other hand, uses a divide-and-conquer approach to sort the array.
Bubble sort is a simple sorting algorithm that works by repeatedly iterating through the array and swapping adjacent elements if they are in the wrong order.
The choice of sorting algorithm depends on the specific use case and the characteristics of the data being sorted. For example, quicksort is generally faster than merge sort, but can be slower for nearly sorted data.
Here are some popular sorting algorithms:
Searching
Searching is a crucial step in the sorting and searching process. It's what allows us to find specific items in a collection.
The most basic form of searching is a linear search, which involves checking each item in a collection one by one until we find the one we're looking for. This can be time-consuming, especially with large collections.
In a linear search, the average time complexity is O(n), where n is the number of items in the collection. This means that as the collection grows, the time it takes to find an item increases linearly.
For example, if we have a collection of 10 items and we need to find a specific item, we would have to check each item one by one, which could take some time.
A different take: Google One vs Google Drive
Algorithm Techniques
Becoming proficient in algorithmic techniques is crucial for acing Google data structure and algorithm interviews. Focus on mastering techniques like dynamic programming, greedy algorithms, divide and conquer, backtracking, and more.
To become proficient in algorithmic techniques, practice implementing these techniques to solve a wide variety of problems. Here's a roadmap to help you get started:
- Master dynamic programming techniques for solving problems by breaking them into smaller subproblems.
- Practice solving dynamic programming problems, which often involve optimization tasks.
- Understand the divide and conquer strategy, which involves breaking a problem into smaller subproblems, solving them, and combining the results.
- Practice solving problems using divide and conquer, such as merge sort and the fast exponentiation algorithm.
Some key algorithmic techniques to focus on include dynamic programming, which involves breaking down complex problems into smaller subproblems and storing the results to avoid redundant computations.
Graphs
Graph algorithms deal with problems involving networks and relationships between data points. These algorithms are essential for traversing graphs, and two popular methods are breadth-first search (BFS) and depth-first search (DFS).
BFS and DFS are used for traversing graphs, and they can also be used for solving problems like finding the shortest path in a graph.
To master graph algorithms, you should learn graph algorithms like BFS and DFS, as well as study graph algorithms for solving problems like finding the shortest path (Dijkstra’s, Bellman-Ford) and detecting cycles.
Some common graph algorithms include breadth-first search, depth-first search, Dijkstra’s algorithm, Bellman-Ford algorithm, and cycle detection.
Here are some examples of graph algorithm problems:
- Finding the shortest path in a graph
- Detecting cycles in a graph
- Traversing a graph using BFS or DFS
These algorithms are used in various applications, including social network analysis, traffic routing, and recommendation systems.
Some common graph algorithm interview questions include:
- For a given binary tree, write a function to calculate its height
- For a given binary tree, write a code to find the lowest common ancestors of the nodes
- For a given graph with E edges and V vertices, find out whether the graph contains a cycle or not
Mastering graph algorithms can help you solve complex problems and improve your coding skills.
Master Techniques
Mastering algorithm techniques is a crucial step in becoming proficient in problem-solving. To start, let's break down the key techniques you should focus on: divide and conquer, dynamic programming, backtracking, and greedy algorithms.
Divide and conquer is a strategy that involves breaking down a problem into smaller subproblems, solving them, and combining the results. This technique is particularly useful for problems that have a recursive structure.
Dynamic programming is a technique used to solve complex problems by breaking them into smaller subproblems and solving each subproblem only once, storing the results in a table to avoid redundant computations. This approach is essential for optimization tasks.
A different take: Dynamic Utm Parameters Google Ads
Backtracking is an algorithmic technique used to solve problems that require trying out different possibilities and undoing choices if they don’t lead to a solution. It's often used for problems involving permutations, combinations, and Sudoku.
Greedy algorithms make the locally optimal choice at each step with the hope of finding a globally optimal solution. They are often used for optimization problems, but be aware that they may not always produce the optimal solution.
To become proficient in these techniques, practice is key. Here are some specific techniques to focus on:
- Divide and conquer: practice solving problems like merge sort and fast exponentiation algorithm.
- Dynamic programming: practice solving optimization tasks.
- Backtracking: practice solving problems involving permutations, combinations, and Sudoku.
- Greedy algorithms: practice solving optimization problems.
By mastering these techniques, you'll be well on your way to becoming proficient in algorithmic problem-solving. Remember to analyze the time and space complexity of your algorithms and optimize your code for large input sizes. With practice and dedication, you'll be able to tackle even the most complex problems with confidence.
Disjoint Set (Union-Find)
The disjoint set union (DSU), or union-find, is a powerful data structure used to solve problems related to partitioning a set into disjoint subsets and determining if two elements are in the same subset.
It's an efficient tool for solving graph-related problems, particularly those involving graph connectivity.
Compared to other Google data structures, it's often overlooked because its use is specific to graph connectivity problems.
The DSU is crucial for problems like detecting cycles and finding connected components.
Kruskal’s algorithm (Minimum spanning tree) is one such problem where DSU plays a vital role.
Network connectivity is another area where DSU is essential.
DSU remains an efficient tool for solving these graph-related problems.
Frequently Asked Questions
Is the Google DSA course worth it?
The Google DSA course is a comprehensive resource that can significantly improve your Data Structures and Algorithms skills, making it a valuable investment for those preparing for tech interviews. It's particularly beneficial for cracking both product and service-based company interviews.
Is DSA required for Google?
Yes, a strong foundation in Data Structures and Algorithms (DSA) is crucial for Google's technical interviews. However, other skills like system design, coding proficiency, and behavioral skills also play a significant role in the hiring process.
What is the data structure of Google Maps?
Google Maps uses a graph data structure, which is a collection of nodes connected by edges and vertices, to calculate routes. This graph is the backbone of Google Maps' navigation system.
Sources
- https://www.geeksforgeeks.org/data-structures-algorithms-dsa-guide-for-google-tech-interviews/
- https://www.interviewkickstart.com/blogs/interview-questions/coding-data-structures-and-algorithm-questions-for-google-software-engineer-interview
- https://www.coursera.org/courses
- https://www.interviewkickstart.com/blogs/interview-questions/data-structure-algorithm-interview-questions-at-google
- https://learningdaily.dev/data-structures-you-shouldnt-overlook-in-google-interviews-7bc316b19b2a
Featured Images: pexels.com