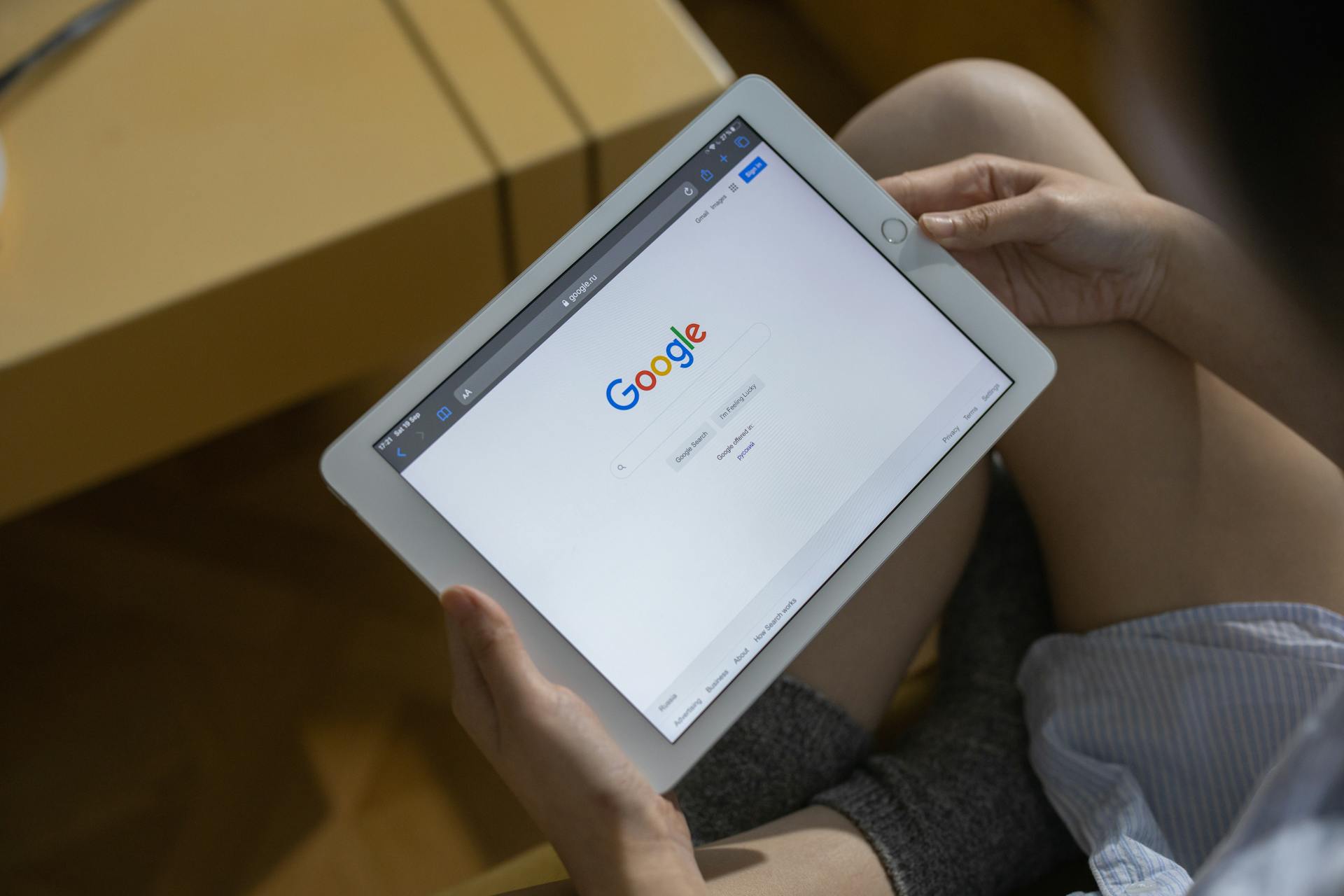
Google Drive scopes are essentially permissions that allow developers to access specific features and data within a user's Google Drive account. There are two main types of scopes: full and limited.
Full scopes, as we've seen, grant access to all files and folders in a user's Google Drive account. This is the most permissive type of scope and should be used sparingly.
Limited scopes, on the other hand, restrict access to specific features, such as reading or writing to a single folder. This type of scope is ideal for applications that only need to access a small portion of a user's Drive data.
To request a scope, developers must specify the exact permission they need, such as "drive.file" for reading and writing files or "drive.folder" for accessing folders. The user then reviews and grants the requested permission.
Curious to learn more? Check out: Google Drive Hidden Folder
OAuth 2.0 Authorization
OAuth 2.0 Authorization is a crucial step in accessing Google Drive resources. The authorization process involves configuring the OAuth consent screen and choosing scopes to define what information is displayed to users and app reviewers.
You can configure OAuth 2.0 for authorization by registering your app and defining the level of access granted to your app. An authorization scope is an OAuth 2.0 URI string that contains the Google Workspace app name, what kind of data it accesses, and the level of access.
To define the level of access granted to your app, you need to identify and declare authorization scopes. You can use the Drive API scopes, which include the following:
Using the drive.file OAuth scope and the Google Picker API optimizes both user experience and safety for your app. This scope lets users choose which files they want to share with your app, giving them more control and confidence that your app's access to their files is limited and more secure.
Google Drive API
The Google Drive API is a powerful tool that allows developers to interact with Google Drive, but it requires careful consideration of scopes to ensure proper authorization. You can use scopes like `https://www.googleapis.com/auth/drive.appdata` and `https://www.googleapis.com/auth/drive.appfolder` to view and manage the app's own configuration data in your Google Drive.
The Drive API supports various scopes, including `https://www.googleapis.com/auth/drive.file` for creating new Drive files or modifying existing files, and `https://www.googleapis.com/auth/drive.readonly` for viewing and downloading all your Drive files. You can also use `https://www.googleapis.com/auth/drive.metadata` to view and manage metadata of files in your Drive.
Here are some recommended scopes for the Google Drive API:
- `https://www.googleapis.com/auth/drive.appdata`
- `https://www.googleapis.com/auth/drive.appfolder`
- `https://www.googleapis.com/auth/drive.file`
- `https://www.googleapis.com/auth/drive.readonly`
Google Drive API
The Google Drive API is a powerful tool that allows developers to interact with Google Drive files and folders. It's a great way to build apps that can access and manipulate Drive data.
To use the Google Drive API, you need to define the level of access granted to your app, which is done by identifying and declaring authorization scopes. These scopes are OAuth 2.0 URI strings that contain the Google Workspace app name, what kind of data it accesses, and the level of access.
The Drive API supports several scopes, including https://www.googleapis.com/auth/drive.appdata, which allows your app to view and manage its own configuration data in your Google Drive. Another scope is https://www.googleapis.com/auth/drive.file, which allows your app to create new Drive files or modify existing files that the user shares with an app.
Here's an interesting read: Google Drive Access Levels
There are three types of scopes: Recommended / Non-sensitive, Recommended / Sensitive, and Restricted. Recommended / Non-sensitive scopes provide the smallest scope of authorization access and are ideal for apps that only need basic access. The drive.file scope is a great example of a non-sensitive scope that allows users to choose which files they want to share with your application.
Here are some benefits of using the drive.file OAuth scope:
• Usability: The drive.file scope works with all Drive API REST Resources.
• Features: The Google Picker API provides a similar interface to the Drive UI.
• Convenience: Apps can apply filters for certain Drive file types.
Using the drive.file scope can also result in a more streamlined verification process, as it's a non-sensitive scope that doesn't require an additional security assessment. This can save you time and effort in the long run.
The Drive Labels API also has its own set of scopes, including https://www.googleapis.com/auth/drive.admin.labels, which allows you to see, edit, create, and delete all Google Drive labels in your organization.
Take a look at this: Google Drive Shared File Easy Transfer to My Drive
Cloud Source Repositories
Cloud Source Repositories is a feature of Google Cloud that allows you to store and manage your source code. It's a great way to keep track of your code changes.
The Cloud Source Repositories API, version 1, provides several scopes that define the level of access you need to manage your repositories. You can choose from four different scopes: https://www.googleapis.com/auth/cloud-platform, https://www.googleapis.com/auth/source.full_control, https://www.googleapis.com/auth/source.read_only, and https://www.googleapis.com/auth/source.read_write.
Here's a breakdown of what each scope allows you to do:
These scopes are important to understand because they determine what actions you can take on your source code repositories.
Cloud Storage, V1
Cloud Storage, V1 is an essential part of the Google Drive API, allowing you to manage and interact with your data in Google Cloud Storage.
The Cloud Storage, V1 API offers four main scopes for accessing and managing data.
You can view and manage your data across Google Cloud Platform services with the https://www.googleapis.com/auth/cloud-platform scope.
See what others are reading: Google Cloud and Google Drive Difference
This scope also includes the ability to view your data across Google Cloud Platform services.
The https://www.googleapis.com/auth/devstorage.full_control scope allows you to manage your data and permissions in Google Cloud Storage.
You can view your data in Google Cloud Storage with the https://www.googleapis.com/auth/devstorage.read_only scope.
The https://www.googleapis.com/auth/devstorage.read_write scope is used to manage your data in Google Cloud Storage.
Here are the scopes and their corresponding permissions:
Google Docs API
The Google Docs API is a powerful tool that allows you to interact with Google Docs documents.
You can access all your Google Docs documents with the scope https://www.googleapis.com/auth/documents. This scope gives you the ability to see, edit, create, and delete all your Google Docs documents.
The Google Docs API also allows you to see only your Google Docs documents with the scope https://www.googleapis.com/auth/documents.readonly. This scope is useful if you want to view your documents without making any changes.
You can also use the Google Drive API to interact with your Google Drive files. The scope https://www.googleapis.com/auth/drive allows you to see, edit, create, and delete all of your Google Drive files.
A different take: Removing Files from Google Drive
If you want to limit your access to specific files, you can use the scope https://www.googleapis.com/auth/drive.file. This scope gives you the ability to see, edit, create, and delete only the specific Google Drive files you use with this app.
Alternatively, you can use the scope https://www.googleapis.com/auth/drive.readonly to see and download all your Google Drive files.
Here are the scopes and their corresponding permissions:
Google Slides API
Google Slides API is a powerful tool that allows developers to integrate Google Slides functionality into their applications. It's a part of the Google Drive API, which we'll explore further.
You can use the Google Slides API to access and manipulate Google Slides presentations. The API requires specific scopes to function, which determine the level of access your application has to Google Drive files.
There are several scopes available for the Google Slides API, each with its own set of permissions. Here's a breakdown of the available scopes:
As you can see, the scopes range from full access to read-only access, giving developers the flexibility to choose the level of access that suits their application's needs.
When to Use Restricted Scope
If your application needs to access restricted scopes in Google Drive, you're in the right place. For Drive, only specific application types may access restricted scopes.
These application types include platform-specific and web apps that provide local sync or automatic backup of users' Drive files. Productivity and educational applications whose user interface involves interaction with Drive files also qualify. Reporting and security applications that provide user or customer insight into how files are shared or accessed are also allowed.
Restricted scopes are typically used by applications that require more extensive access to Drive files. However, to continue using restricted scopes, you'll need to prepare your app for restricted scope verification.
Here are the specific application types that can access restricted scopes:
- Platform-specific and web apps that provide local sync or automatic backup of users' Drive files.
- Productivity and educational applications whose user interface involves interaction with Drive files.
- Reporting and security applications that provide user or customer insight into how files are shared or accessed.
App Migration and Benefits
Migrating your app to a non-sensitive scope can be a game-changer. It grants per-file access scope and narrows access to specific features needed by an app.
Many apps work with per-file access without any changes. If you're using your own file picker, switching to the Google Picker API is a good idea, as it fully supports different scopes.
You might like: Google Drive File Restrictions
App Migration
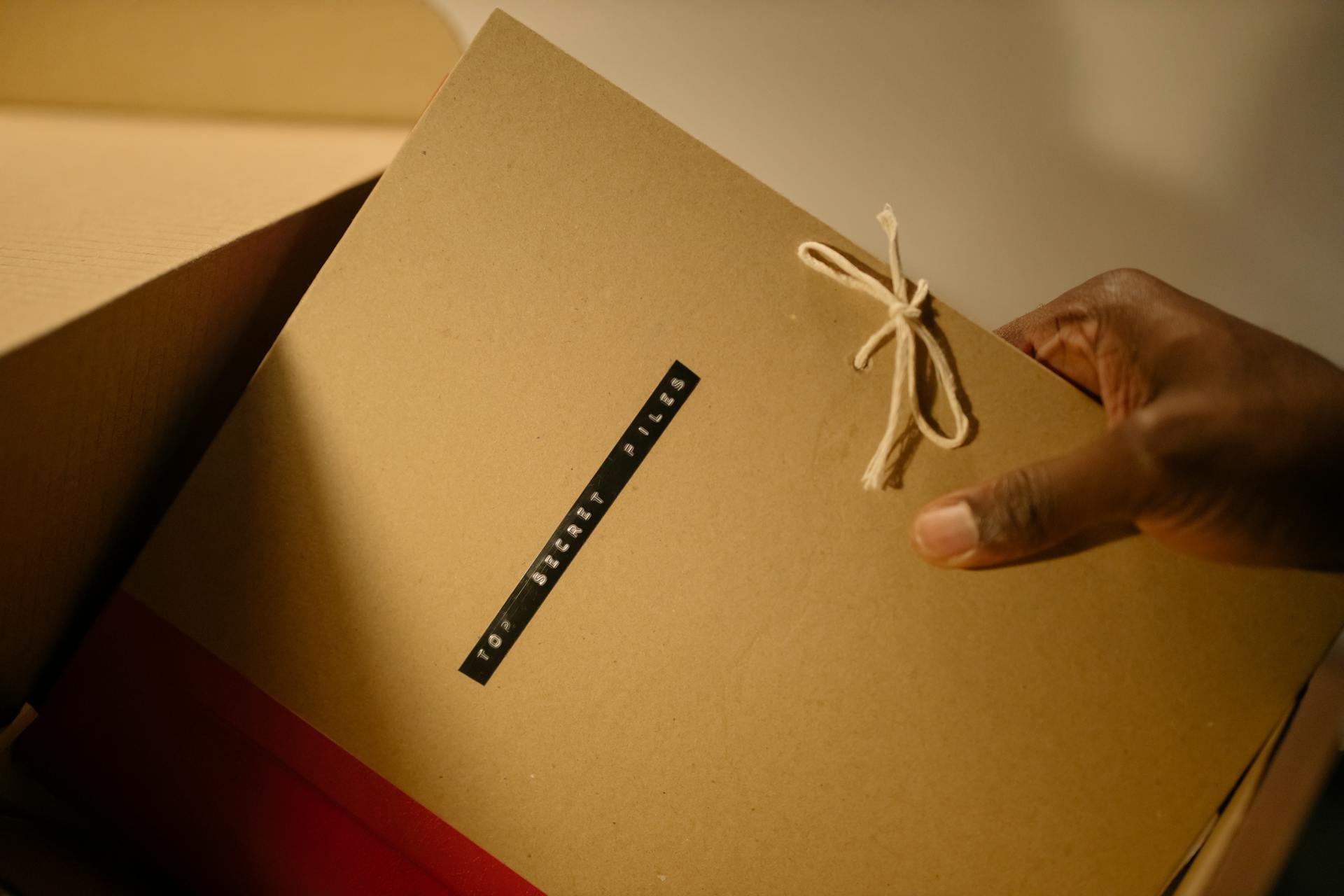
Migrating your app can be a smooth process, especially if you're aware of the benefits. Many apps can work with per-file access without any changes.
If you've developed a Drive app using restricted scopes, it's recommended to migrate to a non-sensitive scope. This grants per-file access scope and narrows access to specific features needed by an app.
Switching to the Google Picker API is a good idea if you're using your own file picker. It fully supports different scopes and can make the transition easier.
Migrating your app can also mean updating your file access permissions. This can help prevent unauthorized access and ensure your app's security.
You can narrow access to specific features needed by an app by using a non-sensitive scope. This can be a big advantage, especially if you're working with sensitive data.
By switching to a non-sensitive scope, you can grant per-file access and reduce the risk of unauthorized access. This can be a big win for your app's security and user trust.
For another approach, see: Google Drive Features
Benefits of Using Drive
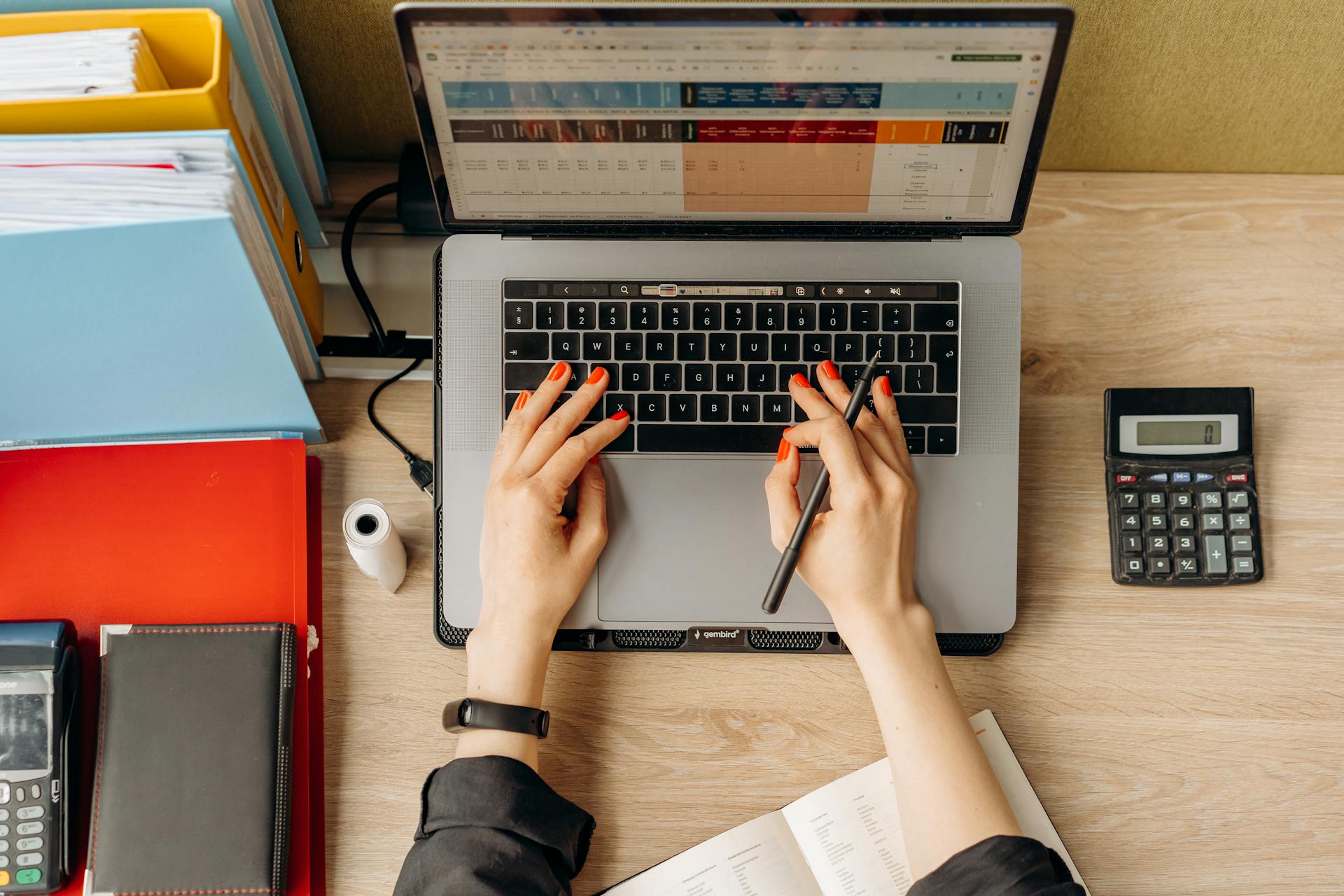
Using the drive.file scope in your application offers several benefits. It allows users to choose which files they want to share with your application, giving them more control and confidence in the security of their data.
This approach is more user-friendly than requiring broad access to all Drive files, which could discourage users from interacting with your application.
The drive.file scope works seamlessly with all Drive API REST Resources, making it easy to use in your application.
The Google Picker API provides a similar look-and-feel to the Google Drive UI, featuring several views that show previews and thumbnails of Drive files, and an inline, modal window that keeps users within your application.
Applications can also narrow down to specific Drive file types, such as docs, sheets, or photos, by applying a filter on the Google Picker files.
Here are some key benefits of using the drive.file scope:
- Usability: The drive.file scope works with all Drive API REST Resources.
- Functionality: The Google Picker API provides a similar look-and-feel to the Google Drive UI.
- Convenience: Applications can narrow to certain Drive file types via a filter on the Google Picker files.
Using the drive.file scope also simplifies the security assessment process, as it's considered non-sensitive, resulting in a more streamlined verification process for your application.
Google Cloud Services
Google Cloud Services provide various levels of access to your Google Drive files and documents.
The Google Docs API, v1, offers several scopes that determine the level of access an app has to your Google Drive files and documents.
The most permissive scope is https://www.googleapis.com/auth/documents, which allows an app to see, edit, create, and delete all your Google Docs documents.
Another scope is https://www.googleapis.com/auth/drive, which grants an app the ability to see, edit, create, and delete all of your Google Drive files.
The scope https://www.googleapis.com/auth/drive.file is more restrictive, only allowing an app to see, edit, create, and delete the specific Google Drive files you use with that app.
The scope https://www.googleapis.com/auth/drive.readonly is used when you want to grant an app the ability to see and download all your Google Drive files, but not edit or delete them.
Here's a summary of the scopes mentioned above:
The scope https://www.googleapis.com/auth/documents.readonly is used when you want to grant an app the ability to see all your Google Docs documents, but not edit or delete them.
Google Drive Activity and Data
Google Drive Activity and Data is a powerful feature that allows developers to access and interact with the activity record of files in Google Drive. The Drive Activity API, v2, is the tool that makes this possible.
With the Drive Activity API, v2, you can view and add to the activity record of files in your Google Drive using the scope https://www.googleapis.com/auth/drive.activity. This scope is necessary to access the full range of activity record features.
The activity record includes information about who, when, and what actions were performed on a file. This can be useful for developers building apps that require access to this type of data.
You can also use the scope https://www.googleapis.com/auth/drive.activity.readonly to view the activity record of files in your Google Drive, without the ability to add to it.
Here's a summary of the scopes mentioned:
Google Docs and Slides
Google Docs and Slides are two popular tools within the Google Drive suite.
To access and manage your Google Docs documents, you need to use the Google Docs API, which requires specific scopes. The most basic scope is https://www.googleapis.com/auth/documents, which grants permission to see, edit, create, and delete all your Google Docs documents.
The Google Docs API also offers a read-only scope, https://www.googleapis.com/auth/documents.readonly, which allows you to see all your Google Docs documents.
Google Slides, on the other hand, requires scopes that are similar to Google Docs. You can use the https://www.googleapis.com/auth/drive scope to see, edit, create, and delete all of your Google Drive files, including Google Slides presentations.
Here's a comparison of the scopes required for Google Docs and Google Slides:
The Google Slides API also offers a scope for presentations, https://www.googleapis.com/auth/presentations, which grants permission to see, edit, create, and delete all your Google Slides presentations.
Authentication and Authorization
Authentication and authorization are crucial steps in accessing Google Drive scopes, and Google provides several methods to achieve this.
OAuth2 is a popular choice, allowing you to make API calls on behalf of a given user. The user visits your application, signs in with their Google account, and provides your application with authorization against a set of scopes.
You can access your service from a client or the server using an API key, but this is typically less secure and only available on a small subset of services with limited scopes.
Another option is application default credentials, which provides automatic access to Google APIs using the Google Cloud SDK for local development, or the GCE Metadata Server for applications deployed to Google Cloud Platform.
A service account credential model allows your application to talk directly to Google APIs using a Service Account, useful when you have a backend application that will talk directly to Google APIs from the backend.
Here are the authentication methods in detail:
- Oauth2: This allows you to make API calls on behalf of a given user.
- API Key: With an API key, you can access your service from a client or the server.
- Application default credentials: Provides automatic access to Google APIs.
- Service account credentials: Your application talks directly to Google APIs using a Service Account.
To learn more about the authentication client, see the Google Auth Library.
Google Sheets API
Google Sheets API is a powerful tool that allows developers to interact with Google Sheets from their applications.
The Google Sheets API, v4, offers different scopes that determine what level of access your app has to Google Sheets.
You can choose from four scopes: https://www.googleapis.com/auth/drive, https://www.googleapis.com/auth/drive.file, https://www.googleapis.com/auth/drive.readonly, and https://www.googleapis.com/auth/spreadsheets.
Here are the scopes for Google Sheets API, v4, in a table for easy reference:
Frequently Asked Questions
How do I add scopes to Google Cloud?
To add scopes to Google Cloud, click "Add or Remove Scopes" after completing the app registration form in the OAuth consent screen. This step is required when creating an app for use outside of your Google Workspace organization.
Sources
- https://developers.google.com/drive/api/guides/api-specific-auth
- https://developers.google.com/identity/protocols/oauth2/scopes
- https://github.com/googleapis/google-api-nodejs-client
- https://support.google.com/cloud/answer/13807380
- https://security.stackexchange.com/questions/209317/what-is-the-scope-of-google-drives-oauth-credentials
Featured Images: pexels.com