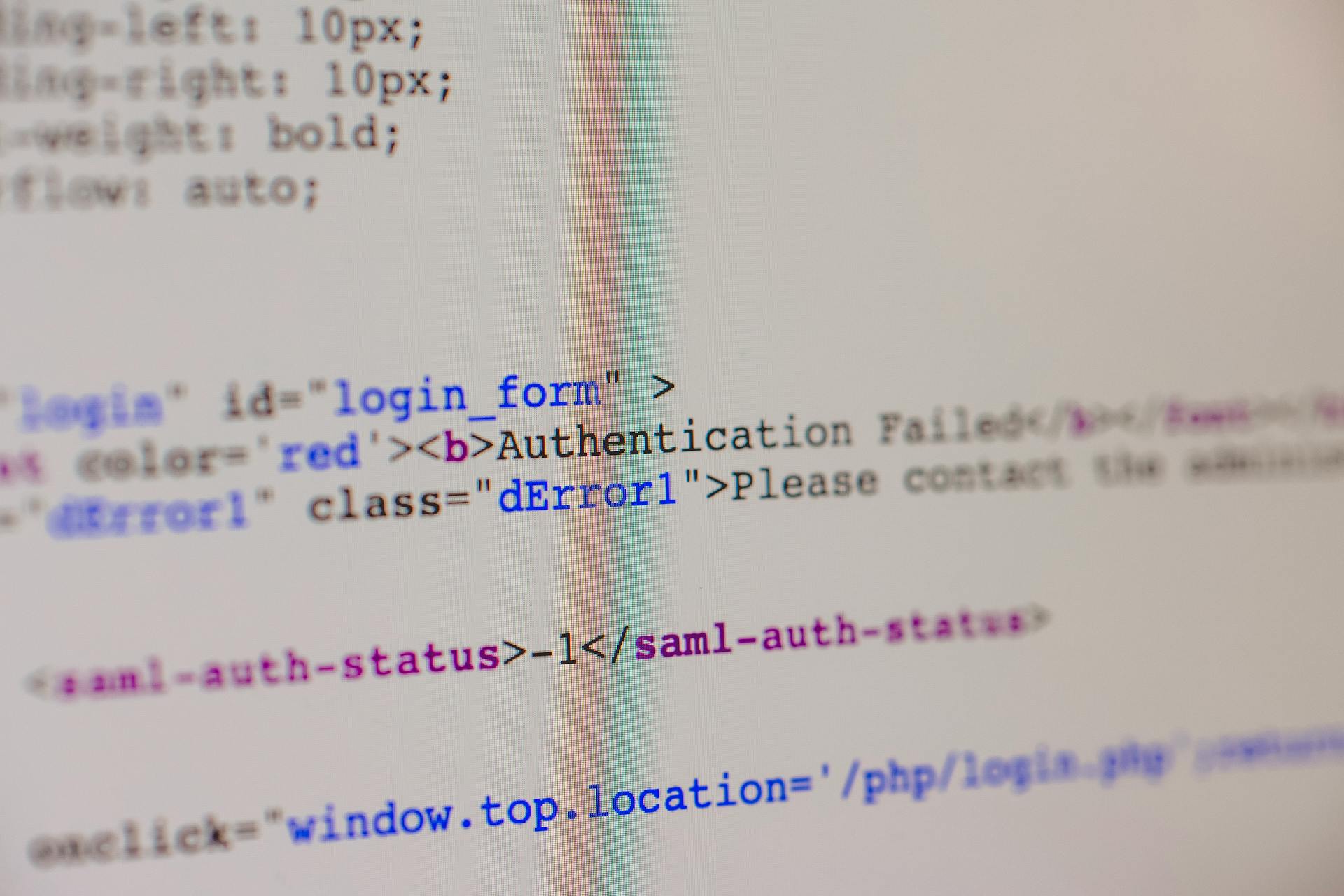
Calling a class in CSS is a fundamental concept that can be a bit tricky to grasp at first, but don't worry, it's easier than you think.
To call a class in CSS, you simply need to use the dot notation, as shown in the example: `.class-name { styles }`. This is the basic syntax for selecting an HTML element that has a specific class.
The order of the class name doesn't matter, so `.class-name` and `.name-class` are treated the same way. This is because CSS selectors are case-insensitive and class names are identifiers.
To apply multiple classes to an HTML element, you can simply separate the class names with a space, like this: `.class-name .another-class { styles }`. This will apply the styles defined in both classes to the element.
For another approach, see: Css Class Inheritance
Adding and Removing Classes
Adding and Removing Classes is a breeze with the classList API. It provides four methods: add, remove, toggle, and contains. These methods simplify the process of adding and removing class values from an element.
The add method allows you to add a class value to an element. For example, if you have a div element, you can add a class value like this: `div.classList.add('bar', 'foo', 'zorb', 'baz')`. The classList API takes care of adding spaces between class values.
Removing a class value is just as easy. You can use the remove method to remove a class value from an element. For instance, if you have a div element with the class value 'foo', you can remove it like this: `div.classList.remove('foo')`.
The toggle method is useful for scenarios where you need to add or remove a class value based on its current state. If the class value exists, it removes it; if it doesn't exist, it adds it.
Here are the four methods provided by the classList API:
- add: adds a class value to an element
- remove: removes a class value from an element
- toggle: adds or removes a class value based on its current state
- contains: checks if a class value exists on an element
These methods make working with class values a piece of cake!
Class Management and Styling
There's a much nicer API for managing class values in HTML elements called classList, which makes working with class values ridiculously easy.
The classList API provides a handful of methods: add, remove, toggle, and contains. These methods make it easy to add or remove class values from an element without having to deal with error-prone string-related trickery.
To add a class value to an element, you can call the add method on it via classList, like this: `element.classList.add('class-name')`. This will add the specified class value to the element, and the classList API will ensure spaces are added between class values.
Here are the classList methods in detail:
- add: adds a class value to an element
- remove: removes a class value from an element
- toggle: adds or removes a class value from an element
- contains: checks if an element has a specified class value
The toggle method is particularly useful for simplifying the common workflow of checking if a class value exists, and if it does, removing it, and if it doesn't, adding it.
Pseudo-classes: Dynamic Styling
Pseudo-classes allow you to select elements not only by their name but also by their current state, position within the document, or even user interactions. They always begin with a colon (:) and are attached to a regular class or element selector.
Pseudo-classes can be used to style elements based on their state, such as :hover, :focus, or :active. These are commonly used for interactive elements like buttons and links.
To use pseudo-classes, you simply attach them to a regular class or element selector. For example, you could use .button:hover to style a button when it's being hovered over.
Pseudo-classes can also be used to style elements based on their position within the document, such as :first-child or :last-child. These are useful for styling lists and other elements with multiple children.
Some common pseudo-classes include :hover, :focus, :active, :first-child, and :last-child. These can be used to create a wide range of dynamic effects, from simple color changes to complex animations.
Recommended read: Css Selector Two Classes
Creating and Applying CSS
Creating CSS classes is a fundamental step in styling your web page. You define CSS classes within a separate file called a stylesheet, usually with a “.css” extension.
To create a CSS class, start by defining the class name, which must begin with a period (.). This is how the browser knows it's dealing with a class and not a regular HTML element.
For another approach, see: Tailwind Css Class
A good class name should be descriptive and clearly reflect the purpose of the styles you'll be defining within that class. Using lowercase letters is standard, and if you need multiple words, separate them with hyphens (e.g., “error-message”, “product-title”).
The class definition is enclosed in curly brackets {} and lists each CSS property name followed by a colon (:) and then the value you want to assign to that property. Separate each property-value pair with a semicolon (;).
Here are the basic steps to create a CSS class:
- Define the class name with a period (.).
- Choose a descriptive name.
- Use curly brackets {} to enclose the styling properties and values.
- List each CSS property name followed by a colon (:) and then the value you want to assign to that property.
- Separate each property-value pair with a semicolon (;).
Once you've created a CSS class, you can apply it to a specific element on your web page by using the class attribute within your HTML tags.
Targeting Specific Elements
You can pinpoint specific elements within a class by using a combination of class names and nested tag names. For example, to style all links inside elements with the class "callout-box", you simply combine the class name with the name of the nested tag.
Expand your knowledge: Css Name Selector
Descendant selectors are the most basic way to target nested elements. They work by combining the class name with the name of the nested tag you want to style.
Child selectors are more precise than descendant selectors and target only the direct children of an element. They're indicated with a > symbol and can be used to style only the first-level list items within a class.
Combinators allow you to get even more granular with your targeting.
You might enjoy: Css Nested Class
Key Points and Debugging
Debugging CSS class issues can be frustrating, but there are some key points to keep in mind. Browser Developer Tools can be a lifesaver, allowing you to see applied CSS rules, check specificity, and diagnose why styles might not be taking effect.
To avoid specificity wars, ensure that the CSS rule you want is more specific. You can use the browser inspector to see the winning rule.
CSS syntax errors can happen, but online CSS validators can help you identify mistakes.
If your styles seem to be ignored, check for overwrites. Make sure your styles are not overridden by more specific styles or rules later in your stylesheet or possibly by inline styles.
Here are some common causes of CSS class issues:
Key Points
As you start working with HTML classes, it's essential to understand a few key points to avoid common mistakes.
A single HTML element can belong to multiple classes, which is known as a "multiple classes" scenario. This is achieved by simply separating the class names with spaces within the class attribute.
In most cases, the order of classes within the class attribute doesn't affect the styling. However, if there are conflicting styles, the class listed last will take precedence due to a concept called "specificity".
A different take: Float Attribute in Css
Debugging CSS Issues
Debugging CSS issues can be frustrating, but there are some simple tools that can help you out. Browser Developer Tools are a great place to start, and you can access them by right-clicking and choosing "Inspect" (or similar) from your browser.
These tools let you see which CSS rules are being applied, check the specificity of your styles, and diagnose why styles might not be taking effect. I've used this feature countless times to solve CSS puzzles.
Specificity Wars are a common issue, and they occur when multiple styles target the same element. To resolve this, ensure that the style you want is more specific. You can use the browser inspector to see which rule is winning.
Validation is also important, as CSS syntax errors can happen even to seasoned developers. Online CSS validators can help you identify mistakes in your code.
Lastly, always check for overwrites. If your styles seem to be ignored, make sure they're not being overridden by more specific styles or rules later in your stylesheet or possibly by inline styles.
Here's a quick checklist to help you debug CSS issues:
- Use Browser Developer Tools to inspect applied CSS rules and diagnose issues.
- Check specificity to ensure the style you want is more specific.
- Validate your CSS code to catch syntax errors.
- Check for overwrites by more specific styles or rules.
Frequently Asked Questions
How do you call a class attribute in CSS?
To call a class attribute in CSS, use a period (.) followed by the class name. This simple syntax allows you to target specific elements with a particular class attribute value.
Featured Images: pexels.com