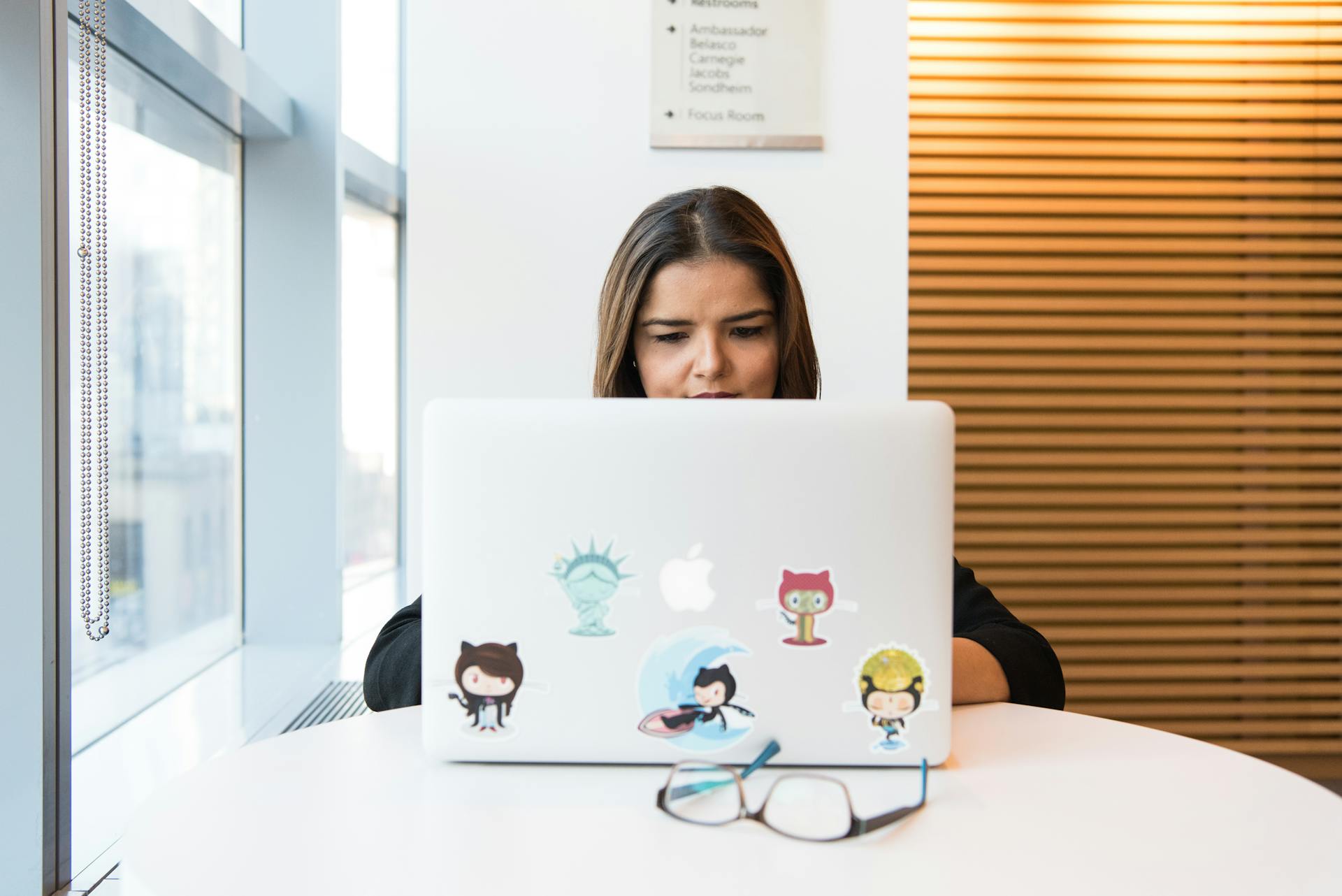
Deploying a MERN app for free is definitely possible, and it's a great way to get started with building and hosting your web applications.
MongoDB Atlas offers a free tier that includes 512 MB of RAM, which is more than enough to get started with a small to medium-sized MERN app.
You can use a free GitHub account to host your code and set up a CI/CD pipeline using GitHub Actions.
This will automatically deploy your app to MongoDB Atlas and make it available to the public.
Setting Up Your Project
To set up your MERN app for deployment, you'll want to start by creating a root folder for your project. Name it anything you like, but for this tutorial, we'll use "mern-deploy".
The first step is to create a root folder for the project. You can name the folder anything you want, but we'll name it "mern-deploy".
There are three possible ways to arrange your files for deployment: keeping them together, splitting them apart, or putting the API behind a proxy. We're choosing to keep them together for this tutorial.
Broaden your view: Free Website Hosting with Own Domain
Here are the three deployment methods mentioned in the article:
- Keep them together – Express and React files sit on the same machine (with all the React files inside a specific client folder), and Express does double duty: serving the React files and also serving API requests.
- Split them apart – Host the Express API on one machine, and the React app on another.
- Put the API behind a proxy – Express and React app files sit on the same machine, but served by different servers
For this tutorial, we'll be creating a package.json file for the root folder by running a specific command. We'll also need to create a .gitignore file to ignore certain files and folders when pushing our code to GitHub.
Configuring Environment
Environment variables are things you can set in the whole environment, not just in your code. In Node, environment variables can be accessed on the process.env object.
In Node, conventionally, environment variables are created in SCREAMING_SNAKE_CASE. You can access them using the process.env object, like process.env.PORT || 8000. To set environment variables, you can use Heroku's website or the heroku CLI.
To set environment variables in Heroku, open your app, go to "Settings", click "Reveal Config Vars", and add a new variable. You can also use the heroku CLI by typing heroku config:set VAR_NAME_GOES_HERE="value goes here".
Here are some examples of things that should be set in environment variables:
- Port numbers
- JWT Secrets
- Database connection strings that include username and password
- Base URLs (if you're serving your client and server completely separately)
Install CLI
To install the Heroku CLI, visit the Heroku CLI download page and follow the instructions to get set up.
Installing the Heroku CLI with Homebrew is the easiest and quickest way to do it if you have it installed.
It'll ask you to log in to the Heroku account you just created, so make sure you have that account information ready.
The Heroku CLI is a command-line tool that makes it easy to keep your web app updated, allowing you to use git to push your code up and have your website updated instantly.
You'll also need to create a new git repository for your app and link that repo up with your newly-created Heroku app.
Set Environment Variables
Setting environment variables is a crucial step in configuring your environment. Environment variables are accessible to your whole machine, not just any one file, and are useful for storing sensitive information.
In Node, environment variables can be accessed on the process.env object. They conventionally are created in SCREAMING_SNAKE_CASE. For example, if you created a JWT secret and hard-coded it into your JavaScript file, anyone looking at your code on Github would know the secret and could grab information they shouldn't be able to.
To set environment variables on Heroku, you can do it two ways: either online on Heroku's website or through the command line using the heroku CLI. If you choose to do it online, you can follow these steps: Open your app in Heroku, go to "Settings", click "Reveal Config Vars", add a new variable and click "Add". Done!
Alternatively, you can set environment variables from the CLI by typing `heroku config:set VAR_NAME_GOES_HERE="value goes here"`. Heroku will then use these variables when your project is deployed.
Here are some examples of things that should be set in environment variables:
- Port numbers
- JWT Secrets
- Database connection strings that include username and password
- Base URLs (if you're serving your client and server completely separately)
In your code, you can access environment variables using the process.env object. For example, if you have a port hardcoded to a number, you should change it to an environment variable like this: `const port = process.env.PORT || 5000;`
Setting Up MongoDB Atlas
Setting up MongoDB Atlas is a great way to host your databases for free, without needing to input any credit card details at all. You can host your databases for free up to a certain amount.
Atlas is MongoDB's cloud database service, and it's easy to get started. MongoDB Atlas is a cloud-based service.
To set up MongoDB Atlas, simply follow the instructions provided, and you'll be up and running in no time. You don't need to worry about scaling up your app beyond the free tier, as it won't charge you unless you manually do so.
Explore further: Azure Webapp Deploy
Setting Up Frontend
Setting up the frontend is where the magic happens. Once your backend is successfully deployed, copy its URL and add it to the client/App.js file.
You'll need to get your Express app to serve up your React app. This involves setting up Express to handle API calls and serve up your React app when someone visits your main site.
Add the following code to your main server.js file: `express.static` is in charge of sending static files requests to the client, and `app.get("*")` is a "catchall" route handler that sends the main index.html file back to the client if it didn't receive a request it recognized otherwise.
You should create a variable near the top of your file like this: `const path = require('path');`.
A different take: Next Js Free
Setting Up Backend
To set up your backend, you'll need to create a folder named backend inside your project's root folder.
Open any code editor, such as VS Code, and navigate to the root folder. Then, create a new folder named backend.
To get started with coding, open the terminal by pressing CTRL + ` on Windows, or the equivalent on MacOS. This will allow you to run commands and install dependencies.
Readers also liked: How to Code a Website for Free
Setting Up Backend
To set up your backend, open any code editor inside the root folder, such as VS Code, and create a new folder named backend.
Run the command to navigate to the backend folder, and then run another command to install all the required dependencies.
Create a file named .env to store all your secret keys, and make sure it looks something like the example provided, with a MONGODB_URI environment variable.
In this file, you can store your secret keys, such as your MongoLab user and password, which will be used to connect to your database.
Create a new file named app.js, and write some code inside it to get started with your backend development.
Integrate Backend URL with Frontend
Once your backend is successfully deployed, you'll need to add its URL to your frontend. Copy the backend URL and paste it into the client/App.js file.
To request the backend, your frontend needs to be configured correctly. If you haven't done this, you might be experiencing issues with getting or posting data.
To fix this, make sure you've added the backend URL to your frontend, as you would do when deploying the backend successfully but data is not able to get or post.
Open the terminal in your code editor and navigate to the backend folder by running the command "cd backend". Then, install all the required dependencies by running the command "npm install". Create a .env file to store your secret keys, and make sure it looks something like this: "SECRET_KEY=your_secret_key".
If you're still having trouble, double-check that you've added the frontend URL to your backend, just like you would when you haven't configured your frontend to request the backend.
Curious to learn more? Check out: Free Data Website
Sources
- https://coursework.vschool.io/deploying-mern-app-to-heroku/
- https://coursework.vschool.io/deploying-mern-with-heroku-old/
- https://coding-boot-camp.github.io/full-stack/render/render-deployment-guide/
- https://workik.com/ai-powered-assistance-for-mern-development
- https://dev.to/kunalukey/how-to-setup-and-deploy-a-mern-stack-project-for-free-5acl
Featured Images: pexels.com