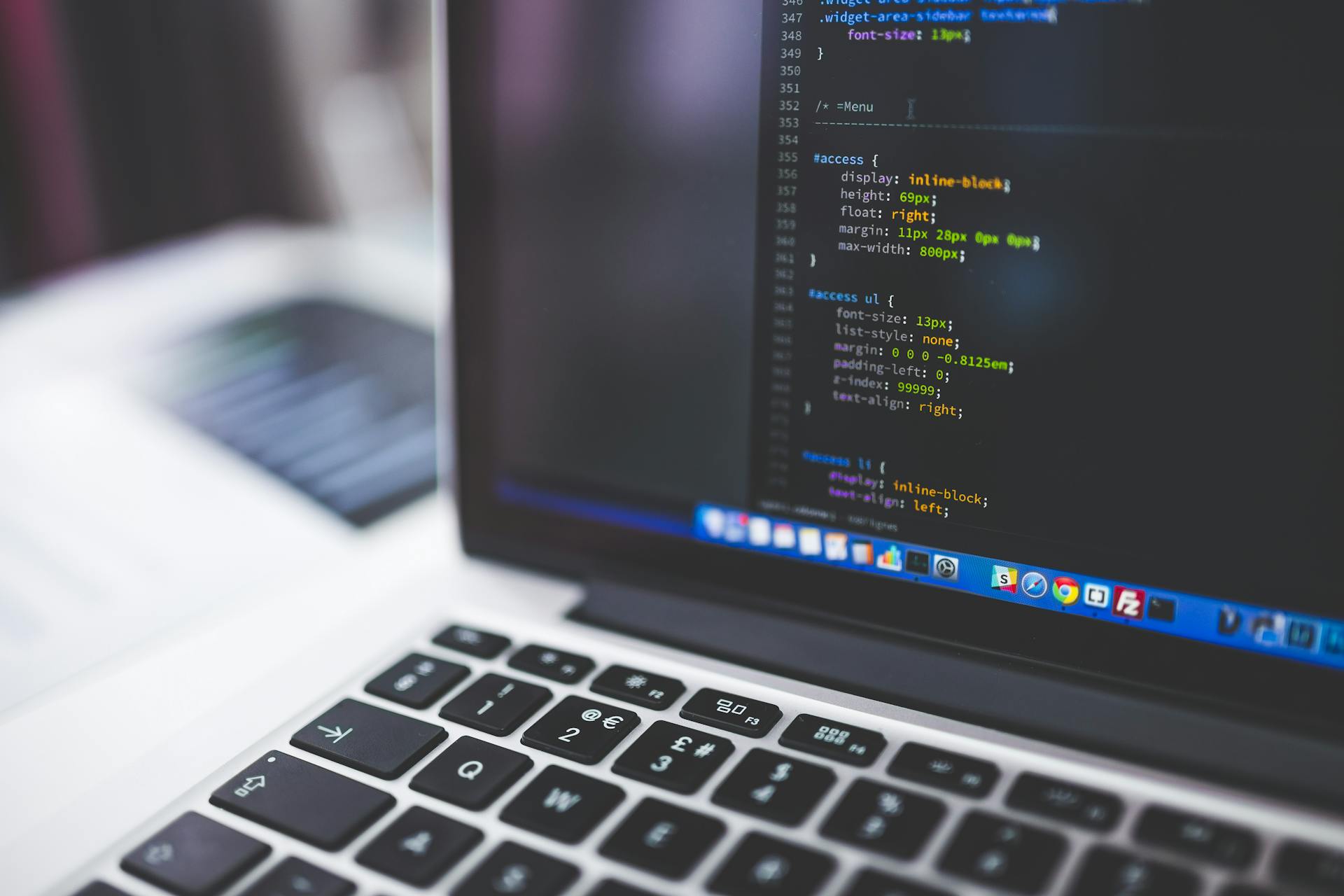
Removing HTML tags from text is a common task in web development, and it's surprisingly easy to do in various programming languages. In Python, you can use the `BeautifulSoup` library to remove HTML tags, as seen in the example where we used `soup.get_text()` to extract the text from an HTML document.
The `re` module in Python is another way to remove HTML tags using regular expressions. In the example, we used `re.sub` to replace all HTML tags with an empty string, effectively removing them from the text.
In JavaScript, you can use the `DOMParser` API to parse the HTML document and then use the `textContent` property to remove the HTML tags. This approach is demonstrated in the example where we used `parser.textContent` to get the text content of the HTML document.
Removing HTML tags is a crucial step in text processing, and the right approach can save you a lot of time and effort in your web development projects.
Expand your knowledge: Python Html to Text
jQuery and Plugins
You can strip HTML tags from form input using jQuery's .val() method to get the input value and the .text() method to strip the HTML tags.
Using jQuery's built-in methods like .text(), .remove(), and .replaceWith() is usually sufficient for most use cases.
There are several jQuery plugins available for stripping HTML tags, such as “jQuery Strip”, “jQuery Remove”, and “jQuery Sanitize”, which provide additional options and functionalities.
These plugins can be useful if you need more advanced features, but for simple stripping of HTML tags, the built-in jQuery methods are often the best choice.
Readers also liked: Coding Tags for Html
When and Why to Remove HTML Tags
Removing HTML tags from text is a common practice with many practical applications. You might want to extract the text content from a web page for analysis or sanitize user input to prevent XSS attacks.
There are many scenarios where removing HTML tags can be helpful. For instance, you might want to extract the text content from a web page for analysis.
For another approach, see: Tags in Azure
Removing HTML tags can help mitigate the risk of XSS attacks by sanitizing user input. XSS is a type of security vulnerability where an attacker injects malicious scripts into webpages viewed by other users.
To prevent XSS attacks, it's essential to always sanitize user input. This means removing or escaping any code that could be interpreted as malicious script.
You can use jQuery's .text() method to safely set or return the text content of selected elements. This method escapes any HTML tags in the content, making it a great tool for sanitizing user input.
A different take: Content Type Text Html
Removing Specific Content
You can remove specific content, like HTML tags from a div, by selecting that div with jQuery and using the .text() method. This method extracts the text from the selected element, effectively removing any HTML tags.
To remove HTML tags from a specific div, you can use the .text() method, as shown in the example: var text = $("#myDiv").text(); This is a straightforward way to get the text content without any HTML tags.
Removing specific content can be as simple as selecting the right element with jQuery and using the right method. The .text() method is a great tool for this task.
For more insights, see: Remove Dropbox Ubuntu
Benchmark
Regular Expressions stand out as the fastest method to remove HTML tags from a string. This is evident from the benchmark results, which show that they outperform other methods in terms of execution time and memory allocation.
The UseRegularExpression() and UseHtmlDecode() methods are the most efficient, with the fastest execution times and minimal memory usage. This makes them ideal for tasks that require quick and lightweight processing.
AngleSharp and HtmlAgilityPack, on the other hand, demonstrate slower performance and higher memory usage. This suggests that they may not be the best choice for tasks that require fast and efficient processing.
The benchmark results clearly highlight the superiority of Regular Expressions and the UseRegularExpression() and UseHtmlDecode() methods.
Frequently Asked Questions
How do I remove HTML from text in Word?
To remove HTML from text in Word, use the "Find and Replace" feature with the regular expression "<[^<>]+>" to eliminate all HTML tags. This simple solution helps you clean up HTML code and restore plain text in your Word documents.
Sources
- https://support.pega.com/question/how-remove-html-tags-string-while-displaying-rd
- https://code-maze.com/csharp-remove-html-tags-from-a-string/
- https://stackabuse.com/bytes/stripping-html-tags-from-text-using-plain-javascript/
- https://www.sitepoint.com/jquery-strip-html-tags-div/
- https://www.browserling.com/tools/html-strip
Featured Images: pexels.com