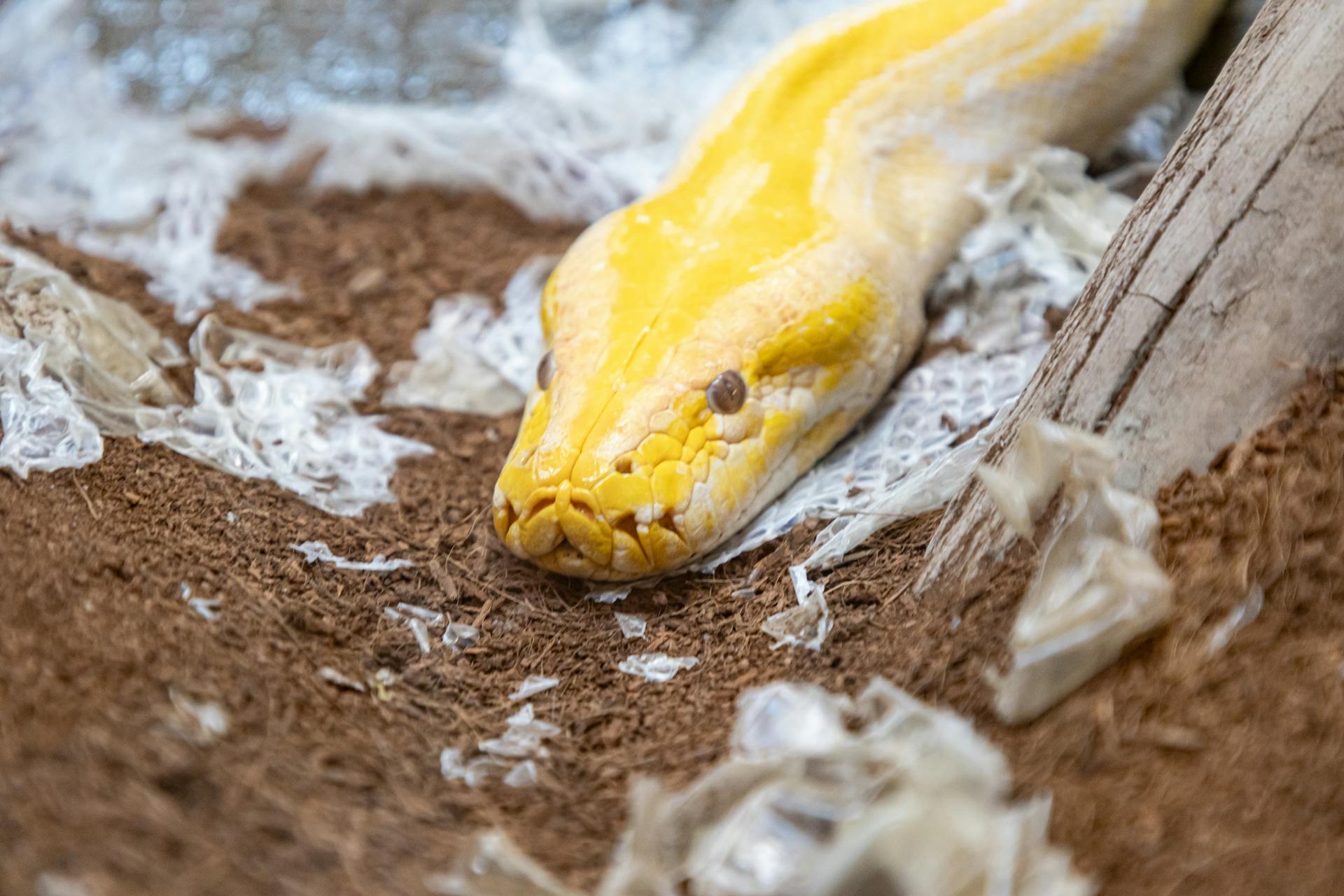
Python Html Text Extraction Techniques involve using libraries like BeautifulSoup and Scrapy to parse HTML content.
BeautifulSoup is a powerful library that allows you to navigate and search through the parts of a document in a hierarchical and human-friendly way.
You can use the find() method to find a specific tag within the HTML content, as shown in the article section example where it's used to find the title tag.
BeautifulSoup also allows you to find all instances of a tag, which can be useful for extracting multiple pieces of information from a webpage.
Scrapy is another popular library used for web scraping, which involves extracting data from websites.
It's a more advanced library compared to BeautifulSoup, but it's well-suited for large-scale web scraping projects.
For more insights, see: Content Type Text Html
Getting Started
You can extract HTML tags from parsed HTML using the find_all() and find() methods.
To extract specific elements, you can use the find_all() method with a dictionary argument, like finding all h3 headings of the class panel-header.
To find a single element, use the find() method with the id parameter, such as extracting the div with the ID header.
You can also use the select() method and pass a CSS selector as a string, like selecting elements using a CSS selector.
Beautiful Soup has many more methods to offer, but these basics will get you started on parsing HTML in Python.
HTTP Request
To make an HTTP request, you need to use a library like Requests, which returns a status code indicating whether the request was successful or not. A status code of 200 means the request was successful.
You can make an HTTP request to a URL and receive some data as a response, which is the HTML content of the web page. The HTML content is hidden inside the response data, so you pass it to the BeautifulSoup constructor for parsing.
Parsing the HTML content makes it easy to navigate through the tree using built-in methods, allowing you to extract the text you need. You can print the text up to a certain number of characters to avoid large returned text.
Worth a look: Data Text Html
The requests-html library extends the HTTP-making library with HTML parsing abilities, making it easy to make an HTTP request and navigate the HTML. This library has full JavaScript support, allowing you to interact with web pages that use JavaScript to render dynamic content.
You can use requests-html to find an HTML element from a web page using its ID and extract the text, making it a useful tool for web scraping.
Curious to learn more? Check out: Editor Html Javascript
Working with HTML
Working with HTML is a fundamental aspect of Python HTML text manipulation. You can use the html.parser module, which is a built-in HTML parser in Python, for simple tasks.
This module defines a class named HTMLParser that serves as the basis for parsing HTML and XML files. By subclassing HTMLParser, you can implement custom parsing behavior.
The html.parser module automatically invokes handler methods such as handle_starttag, handle_endtag, and handle_data when you pass HTML data to an instance of HTMLParser. These methods can be overridden to tailor the parsing behavior to your specific needs.
Recommended read: Html to Pdf Python 3
Basic
Working with HTML can be a bit overwhelming at first, but don't worry, it's actually quite straightforward once you get the hang of it. Beautiful Soup and PyQuery are two popular libraries that make HTML parsing a breeze.
You can use Beautiful Soup to parse HTML files, and it's as simple as importing the library, opening the file, and creating a soup object. From there, you can use the .text function to retrieve the text of specific elements, like the title of the page.
One of the most useful methods in Beautiful Soup is find_all(), which returns a list of all elements that match a certain string. For example, if you want to find all div elements with the class "post", you can use find_all() like this.
Beautiful Soup also has a find() method, which works similarly to find_all() but returns the first matching element instead of a list.
You can also use PyQuery to parse HTML files, and it has a similar syntax to Beautiful Soup. In fact, if you're familiar with jQuery, you'll find PyQuery to be very easy to use.
One of the coolest things about PyQuery is its ability to extract HTML elements with a simple string. For example, if you want to get the text of all h2 headings, you can pass the string "h2" to the PyQuery object and use the .text() method.
Here are some common methods used in Beautiful Soup and PyQuery:
These are just a few examples of how you can use Beautiful Soup and PyQuery to parse HTML files. With a little practice, you'll be a pro in no time!
Component Properties
When working with HTML components in Dash, you have access to properties like style, class, and id.
The style property is a dictionary, which means you need to format it in a specific way. For example, properties in the style dictionary are camelCased.
The class key is actually renamed as className, so make sure to use that in your code. Style properties in pixel units can be supplied as just numbers without the px unit.
If you're using HTML components, you can directly render a string of raw, unescaped HTML using the DangerouslySetInnerHTML component. This component is provided by the dash-dangerously-set-inner-html library.
You might enjoy: Html Text Style
N_Clicks and Disable_N_Clicks
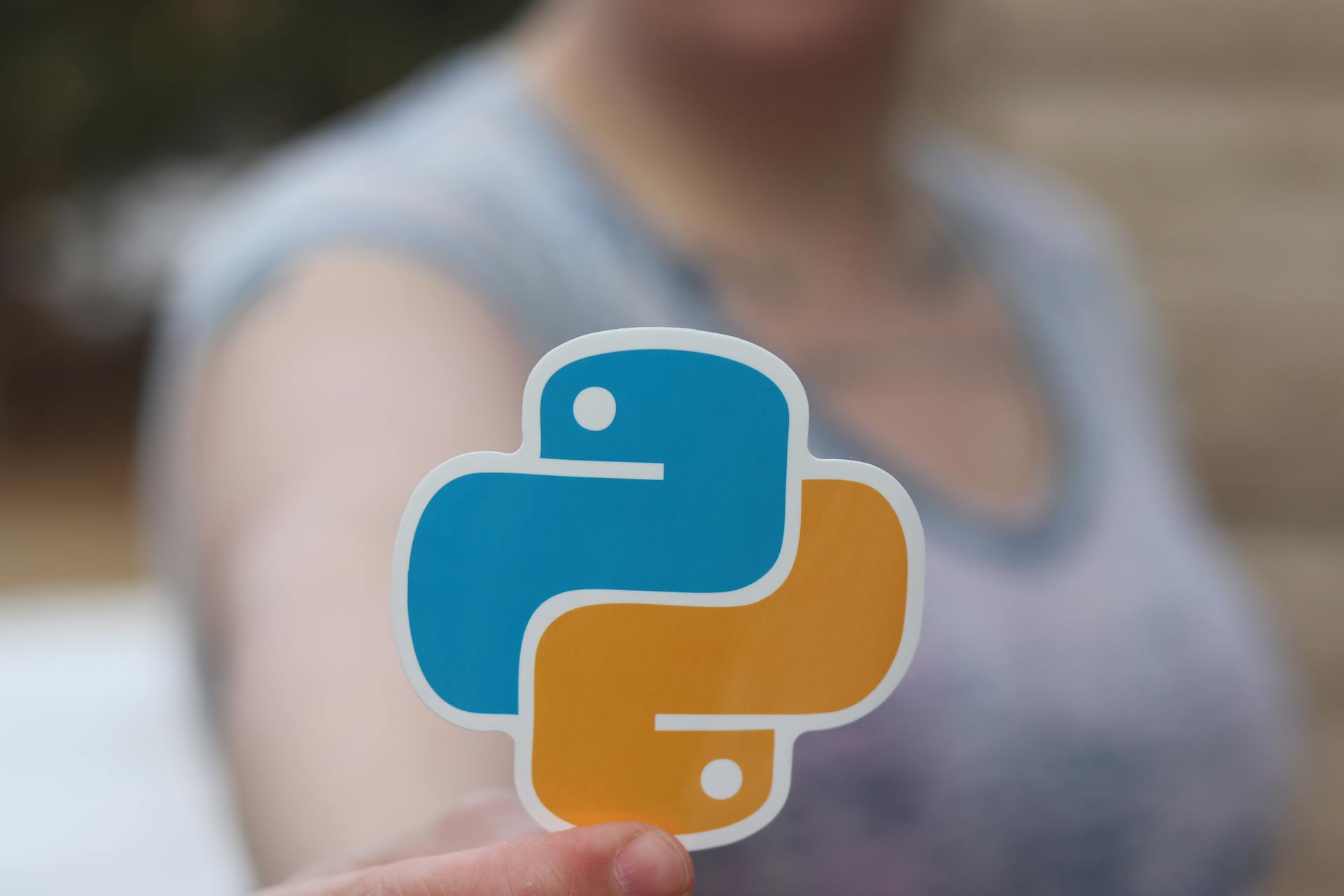
All Dash HTML components have an n_clicks property, which is an integer that represents the number of times the element has been clicked. This property is an integer that increments with each click.
You can use n_clicks to trigger a callback and use the value of n_clicks in your callback logic. Many Dash HTML components are rarely intended to be clicked.
In Dash 2.8 and later, Dash HTML components are improved for better control over the n_clicks event listener. If you don’t give your HTML component an ID, the n_clicks event listener is not added.
If your HTML component does have an ID but you don’t need to capture clicks, you can disable the n_clicks event listener by setting disable_n_clicks=True. This is useful when you don’t need to capture clicks, but still want to convey to screen reader assisted users that the element is not clickable.
Readers also liked: Html Text Element
Text Extraction
Text extraction is a crucial part of web scraping, and BeautifulSoup makes it a breeze. You can use the `get_text()` method to extract all text from within an element, even if there are inner elements.
To do this, you can simply pass the selected tag to the `get_text()` method, and it will return all the text contained within. This is a great way to extract text without modifying the HTML document.
For example, if you have an HTML document with a `span` element containing inner text, you can use `unwrap()` to remove the `span` tags and leave only the inner text. The `unwrap()` method replaces the outer tags with just the inner contents, making it easy to extract the text you need.
Here are some common HTML tags that you can use for text extraction:
- div
- span
- p
You can also use regular expressions (regex) to parse HTML and extract text, but this method can be more complex and error-prone. However, with the right pattern, you can extract the text you need from HTML content.
Extract Inner Text
You can extract all text from within an element using BeautifulSoup's get_text() method, which will extract all the text contained within a selected tag.
This method is particularly useful when you don't want to change the HTML document, but just need to extract the text.
For example, if you have an HTML document with a span tag containing text, you can use get_text() to extract the text without the surrounding span tags.
The get_text() method is a convenient way to extract text from HTML documents without having to manually parse the HTML.
Here's an example of how you can use get_text() to extract text from an HTML element:
Extracted text: "This is the inner text"
As you can see, get_text() has extracted the text contained within the span tag, without the surrounding span tags.
Here's a list of common use cases for get_text():
- Extracting text from a specific HTML element
- Removing surrounding HTML tags
- Extracting text from a complex HTML document
By using get_text(), you can simplify your text extraction tasks and focus on the data you need to extract.
Advanced Techniques
You can parse HTML using regular expressions, which is a more complex but powerful method.
PyQuery is a great tool for parsing HTML, and it's even more useful when you know how to use its advanced features.
You can use regular expressions to parse HTML, but be aware that this method can be brittle and prone to breaking.
With PyQuery, you can easily fix broken HTML by using its robust parsing capabilities.
Removing unnecessary tags from an HTML document can be a real challenge, but it's a crucial step in cleaning up messy code.
You can use PyQuery's methods to remove unnecessary tags and simplify your HTML.
Understanding the concept of parents, children, and siblings in HTML is essential for navigating complex documents.
With PyQuery, you can easily access and manipulate these relationships using its intuitive API.
Sources
- https://dash.plotly.com/dash-html-components
- https://blog.apify.com/how-to-parse-html-in-python/
- https://scrapeops.io/python-web-scraping-playbook/python-beautifulsoup-eliminate-span-html-tags/
- https://www.roborabbit.com/blog/top-5-python-html-parser/
- https://www.scrapingdog.com/blog/best-python-html-parsing-libraries/
Featured Images: pexels.com