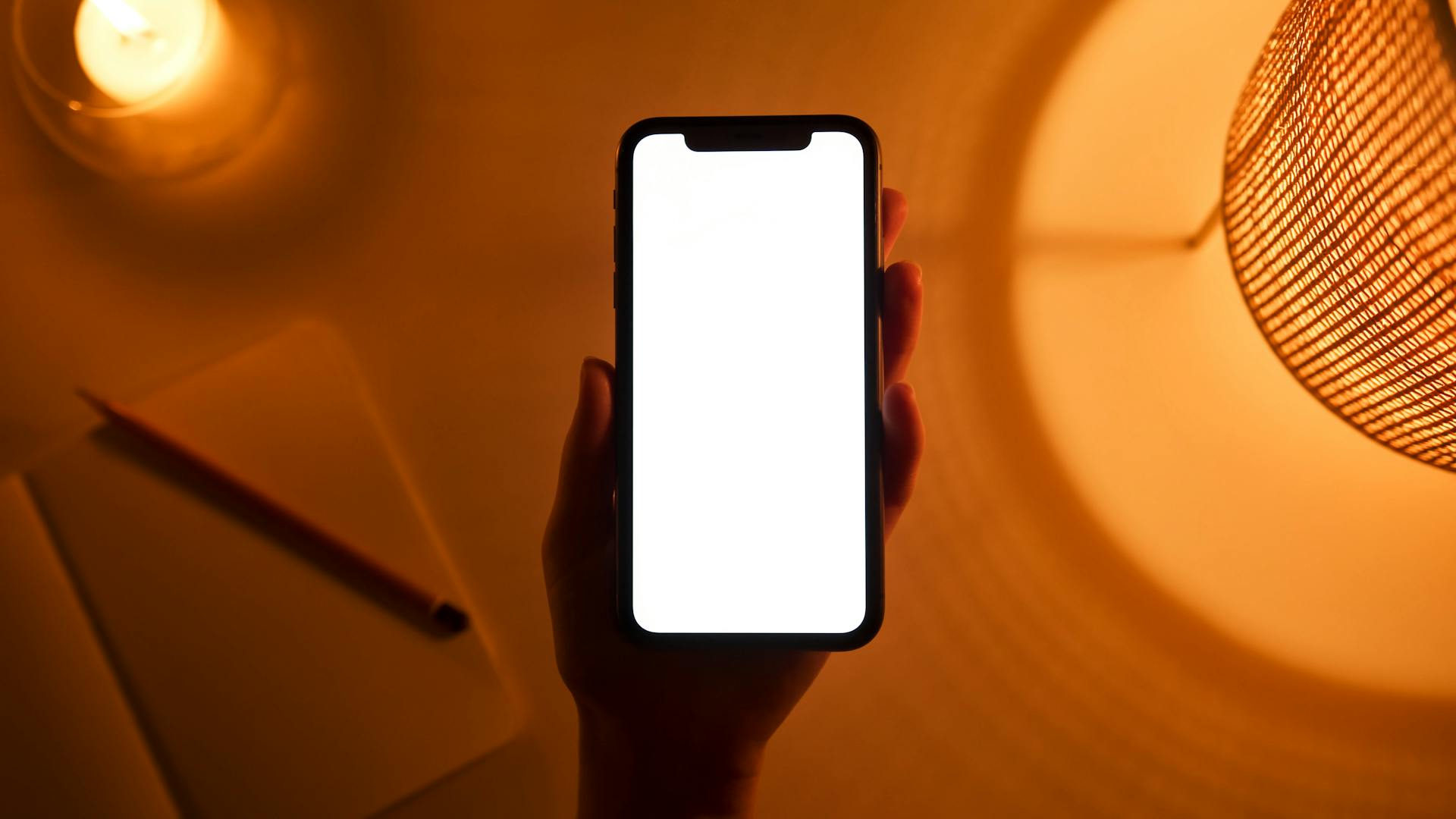
Html2canvas is a JavaScript library that allows you to capture a web page as an image. This can be useful for creating screenshots, sharing web pages, or even generating thumbnails.
With html2canvas, you can specify the elements you want to capture, such as a specific div or an entire page. You can also choose the format of the resulting image, including PNG, JPEG, and SVG.
The library uses a rendering engine to convert the HTML elements into a pixel-perfect image. This process can be customized by adding plugins or modifying the default settings.
Drawing on the Canvas
Drawing on the Canvas is a fundamental aspect of working with HTML 2 Canvas. You can draw on the canvas after creating a 2D context.
The fillRect() method is one of the drawing methods available, which draws a black rectangle with a top-left corner at position 20,20. The rectangle is 150 pixel wide and 100 pixels high.
Worth a look: Html Canvas Text
You can also create a new canvas element with the document.createElement() method, and add the element to an existing HTML page. This allows you to draw on the canvas and manipulate it in JavaScript.
Here are some of the drawing methods available:
Example
You can access a canvas element with the HTML DOM method getElementById(). You can use this method to retrieve a specific canvas element from your HTML document.
To draw in the canvas, you need to create a 2D context object. This is required to perform any drawing operations on the canvas.
You can load a handy library called html2canvas to help you manipulate the canvas element. This library allows you to create a canvas version of your element with just one line of code.
The html2canvas library returns a Promise along with a canvas version of your element. This is a convenient way to get the canvas version of your element without having to write complex code.
You can use the canvas version of your element as a "screenshot" and as a source for information, such as the color of a pixel at a particular location.
Additional reading: Web Designers Code
Drawing Methods
Drawing Methods are a crucial part of creating engaging visuals on the canvas. You can draw rectangles, clear specified pixels, and even add text to your artwork.
The fillRect() method is a great way to start drawing, as it fills a rectangle with a specified color. You can specify the position, width, and height of the rectangle, and it will be drawn on the canvas.
You can also use the strokeRect() method to draw a rectangle without filling it. This is perfect for creating outlines or borders around your artwork.
To clear specified pixels within a rectangle, you can use the clearRect() method. This is useful for creating animations or deleting parts of your artwork.
Here's a quick rundown of the drawing methods at your disposal:
Remember, practice makes perfect, so don't be afraid to experiment with these drawing methods and see what kind of amazing artwork you can create!
Colors and Styles
Colors and styles are a crucial part of bringing your canvas to life, and HTML 2 canvas has some amazing tools to help you achieve the look you want.
You can use the fillStyle property to set the fill color of your drawing object, making it easy to add some color to your canvas.
The addColorStop() method is a powerful tool that allows you to specify the colors and stop positions in a gradient object, giving you complete control over the color transitions in your design.
Here's a quick rundown of some of the key methods and properties for working with colors and styles:
Whether you're creating a simple design or a complex artwork, having the right colors and styles at your fingertips makes all the difference.
Colors and Styles
Colors and styles are crucial elements in creating visually appealing and engaging graphics. You can use the fillStyle property to set the fill color of a drawing object, making it easy to customize the look of your graphics.
To create a gradient effect, you can use the createLinearGradient() method, which creates a linear gradient that can be used on canvas content. This method is perfect for adding depth and visual interest to your graphics.
For more insights, see: How to Use Inspect Element to Find Answers
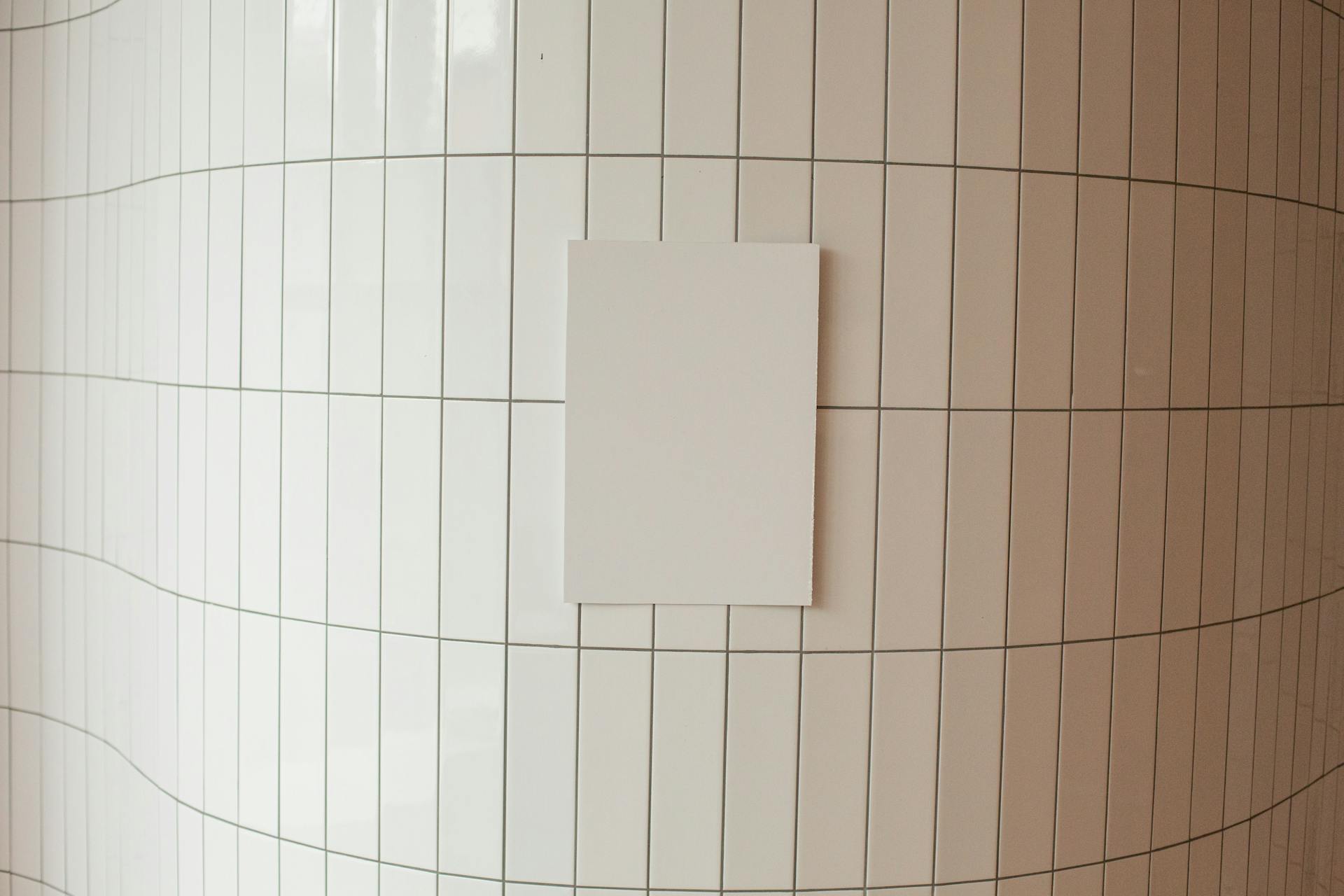
The addColorStop() method is used to specify the colors and stop positions in a gradient object. This method is essential for creating a smooth and seamless gradient effect.
You can use the createPattern() method to repeat a specified element in a specified direction, creating a unique and eye-catching design. This method is perfect for adding texture and interest to your graphics.
The fillStyle property can also be used to set or return the color, gradient, or pattern used to fill the drawing. This property is versatile and can be used in a variety of ways to create different effects.
Here are some common methods and properties used for colors and styles:
The strokeStyle property sets or returns the color, gradient, or pattern used for strokes. This property is similar to the fillStyle property but is used for the stroke of a shape, rather than its fill.
The Secret Sauce
The Secret Sauce is a game-changer when it comes to generating images from HTML. It uses html2canvas to create a PNG image.
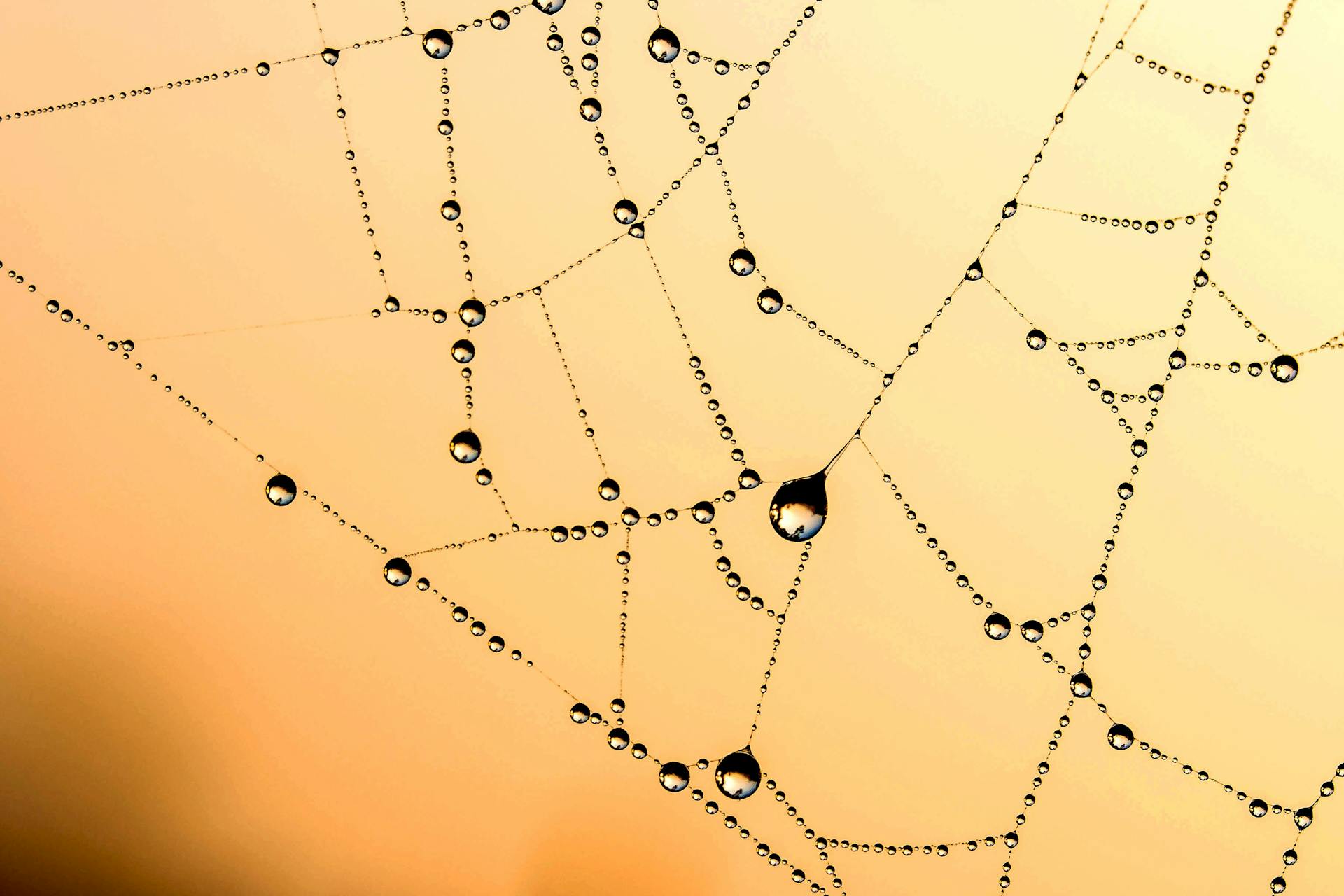
You can querySelector for the container you want to imagify and then call html2canvas with the container and options. It returns a promise that gives you a canvas element.
The image generated is a PNG, but it's not well optimized. You'll likely need to run it through squoosh to get it looking its best.
One thing to note is that the image size is determined by the container's width and height. This is useful for responsive design, but you can fix these values if you want a consistent size.
A unique perspective: Html Size of Text
Paths and Shapes
Creating paths and shapes on a canvas is a fundamental aspect of HTML 2 canvas. You can begin a new path with the `beginPath()` method.
To create a path, you can move to a point on the canvas with `moveTo()`, and then draw lines between points with `lineTo()`. Once you've created your path, you can fill it with `fill()` or draw it with `stroke()`.
Here are the basic steps to create a path:
- Begin a new path with `beginPath()`
- Move to a point with `moveTo()`
- Draw lines between points with `lineTo()`
- Fill or draw the path with `fill()` or `stroke()`
You can also add more complex shapes to your path, such as rectangles with `rect()`, or curves with `bezierCurveTo()`, `arc()`, and `arcTo()`. These methods allow you to create a wide range of shapes and designs.
Some common path methods include `closePath()`, which adds a line to the path from the current point to the start, and `isPointInPath()`, which checks if a specified point is within the current path.
Frequently Asked Questions
What is the difference between html2canvas and jsPDF?
html2canvas captures screenshots of web pages, while jsPDF generates PDF documents, allowing you to create digital versions of your content. The key difference lies in their purpose and output format.
Sources
- https://openmrs.atlassian.net/wiki/display/projects/jsFeedback+implementation+using+html2canvas+plugin+%28Tutorial%29
- https://www.w3schools.com/tags/ref_canvas.asp
- https://andrewwalpole.com/blog/blog-post-image-generator-with-html2canvas/
- https://blog.logrocket.com/export-react-components-as-images-html2canvas/
- https://css-tricks.com/adding-particle-effects-to-dom-elements-with-canvas/
Featured Images: pexels.com