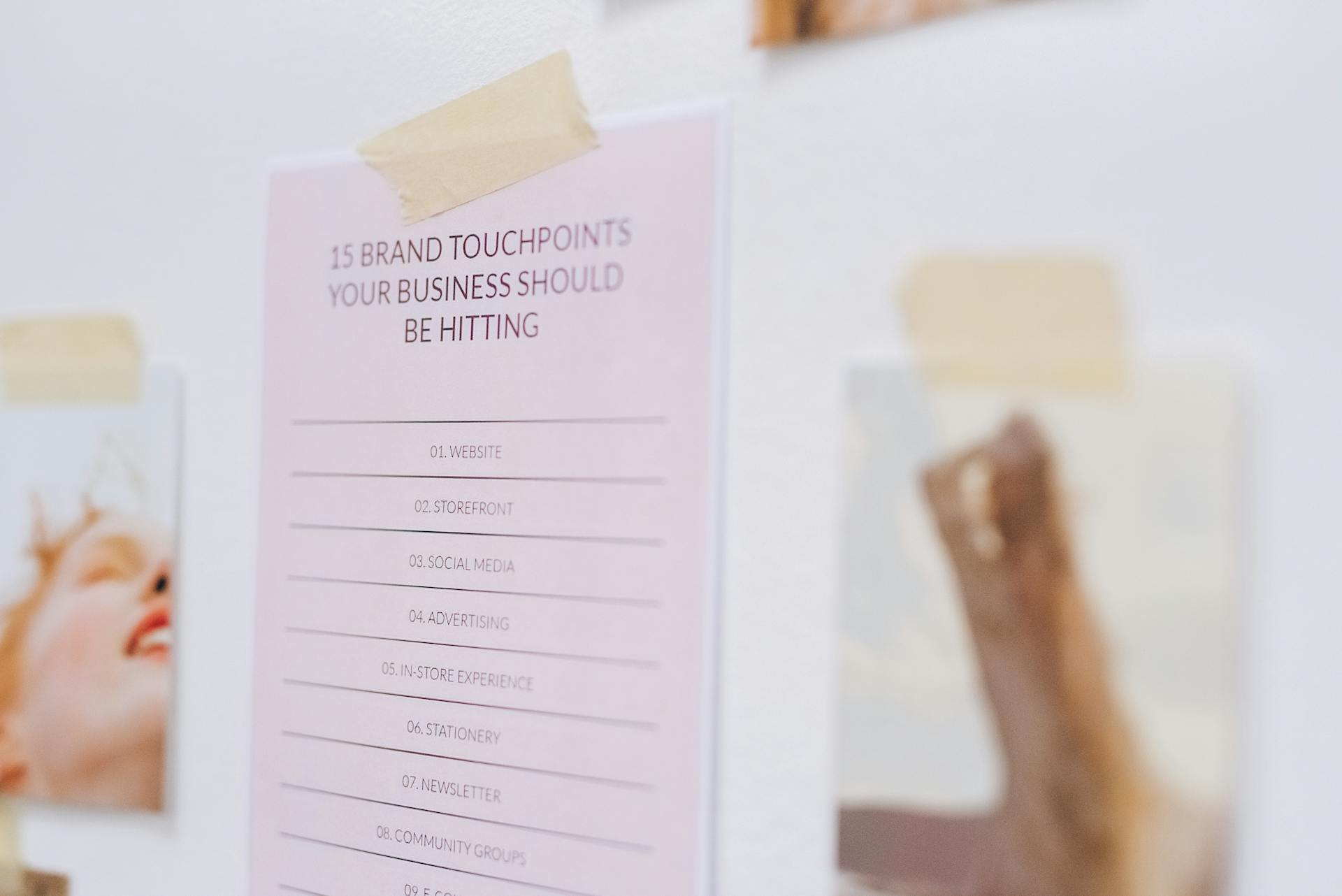
Linked list is a fundamental data structure that's often overlooked in modern programming, but it's still a must-know for interviews. It's a great way to test a candidate's problem-solving skills and understanding of data structures.
In a linked list, each node stores a value and a reference to the next node, allowing for efficient insertion and deletion of elements. This is especially useful when working with large datasets.
Many interviewers use linked list problems to assess a candidate's ability to think recursively and handle edge cases. A good example of this is the problem of finding the middle element of a linked list.
Understanding linked list operations such as insertion, deletion, and traversal is crucial for any programmer. It's not just about knowing the data structure, but also being able to apply it to real-world problems.
See what others are reading: Why Is Candidate Experience Important
What is a Linked List?
A linked list is a data structure where each item is a separate object, linked together via pointers. The head of the list is the first node, which contains data and a reference to the next node.
The nodes in a linked list have two main parts: data and a reference to the next node. This structure allows for efficient insertion and deletion of nodes.
The tail of a linked list is the last node, whose reference points to NULL, indicating the end of the list. This is what distinguishes a linked list from other data structures.
Here's a breakdown of the main components of a linked list:
- Head: The entry point or the first node in the list.
- Nodes: Each node contains two parts — the data it holds and a reference (pointer) to the next node.
- Tail: The last node in the list whose reference points to NULL, indicating the end of the list.
Types of Linked Lists
A linked list is a fundamental data structure that's essential to know for any programming interview. The anatomy of a linked list is crucial to understanding the different types.
The head of a linked list is the entry point or the first node in the list. It's the starting point from which the list is traversed.
There are three main types of linked lists: Singly Linked Lists, Doubly Linked Lists, and Circular Linked Lists. A Singly Linked List has nodes that contain a reference to the next node, while a Doubly Linked List has nodes that contain references to both the previous and next nodes.
A Singly Linked List has a tail node whose reference points to NULL, indicating the end of the list. This is in contrast to a Circular Linked List, where the last node's reference points back to the head, creating a circular structure.
Here's an interesting read: Important Points
Singly
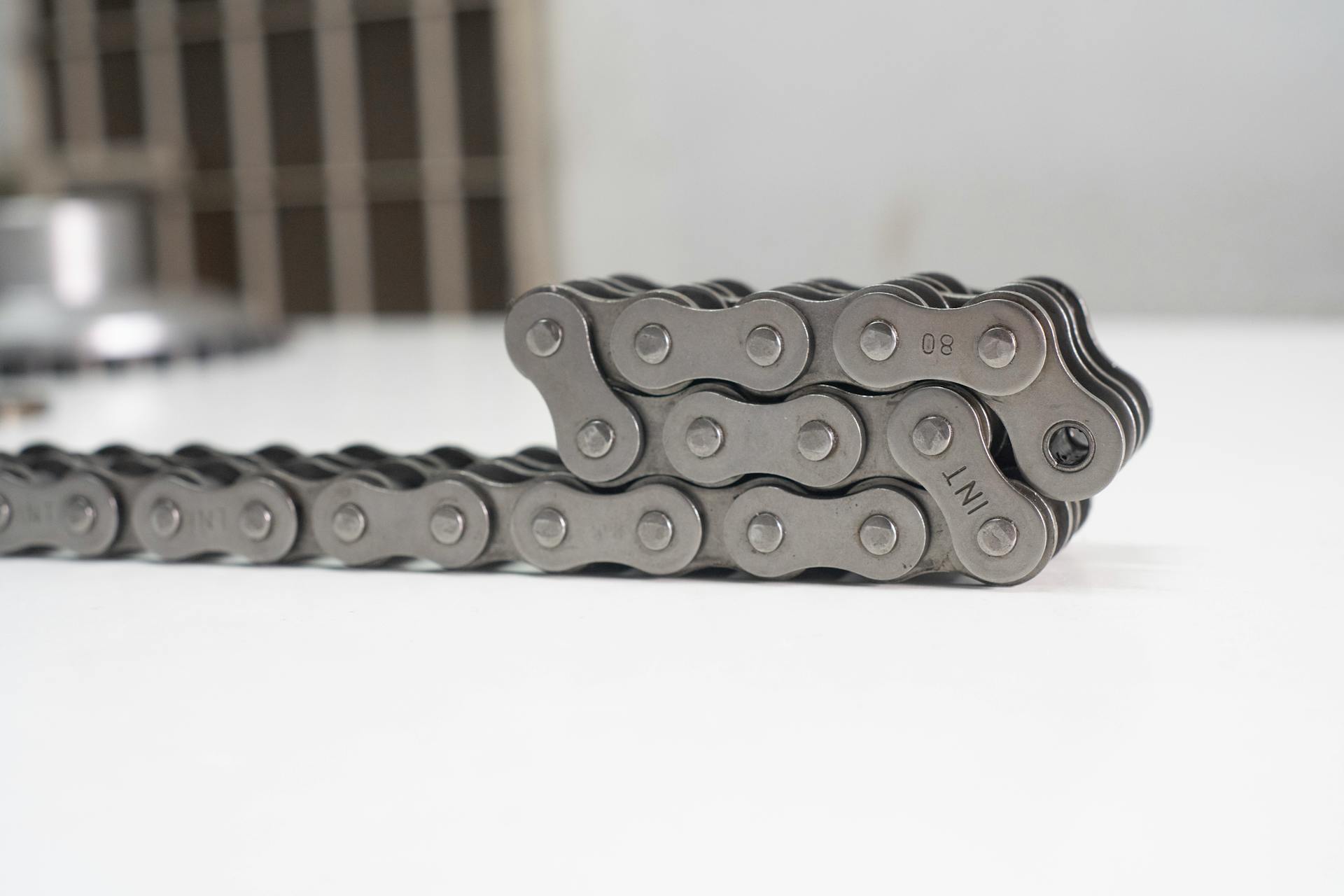
A singly linked list is a type of linked list where each node points only to the next node in the sequence.
This type of list is traversed in one direction only, which means you can move from one node to the next, but you can't move backward.
One of the benefits of using a singly linked list is that operations like insertion and deletion are faster compared to arrays. This is because you don't need to shift elements around, which can be a time-consuming process.
Here are some key characteristics of singly linked lists:
- Each node points only to the next node in the sequence.
- It is traversed in one direction only (forward).
- Operations like insertion and deletion are faster compared to arrays.
Doubly
Doubly Linked Lists offer a unique combination of features that set them apart from other types of linked lists. Each node has pointers to both the next and the previous nodes.
This design allows for traversal in both directions, making it a great choice when you need to move backwards as well as forwards through the list. It's like having a map that lets you navigate in both directions, which can be really helpful in certain situations.
One thing to keep in mind is that doubly linked lists have more memory overhead due to the extra pointer. This can be a consideration when working with large datasets or systems with limited resources.
Here's a quick summary of the key features of doubly linked lists:
- Each node has pointers to both the next and the previous nodes.
- Allows traversal in both directions (forward and backward).
- More memory overhead due to the extra pointer.
Importance in Interviews
Linked list questions are still a staple in technical interviews because they test a crucial skill: understanding pointers. Pointers require a complex form of doubly-indirected thinking that some people just can't do.
Many companies, especially those in the tech industry, have historically found linked list algorithms relevant to their use cases. For example, companies like Microsoft, Apple, and Google have used linked lists in their products and services.
The Guerilla Guide, a popular resource for tech interviews, helped spread linked list questions, and its author, Joel Spolsky, was a minor tech celebrity at the time. This, combined with the release of Cracking the Coding Interview in 2008, made linked list questions a standard part of many interview processes.
Recommended read: Important Technology
Circular
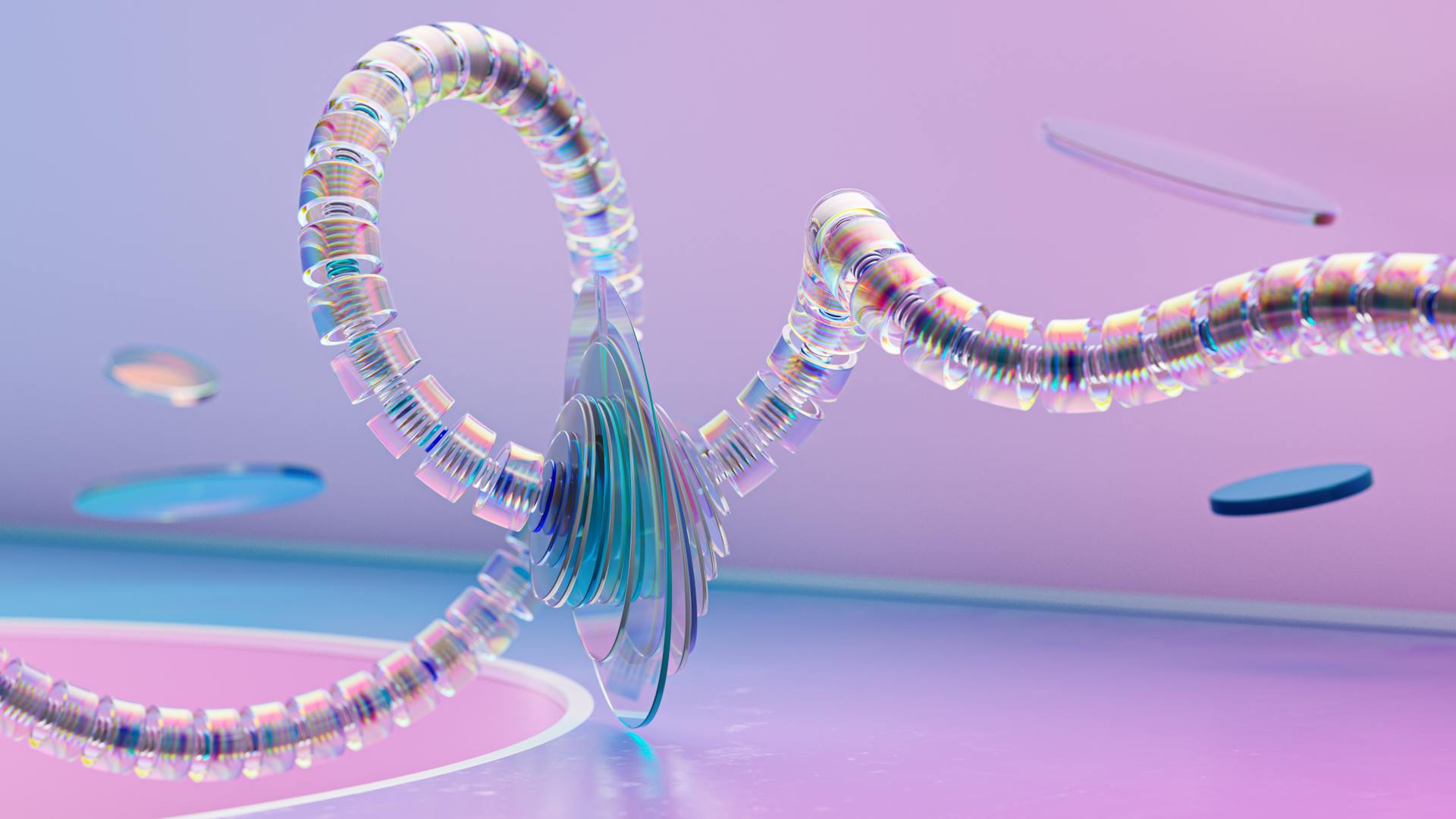
Circular thinking is a key aspect of effective communication in interviews. It involves considering multiple perspectives and being open to new ideas.
According to the article, the ability to think circularly can be developed through practice and experience. This means that even if you're not naturally inclined to think this way, you can still work on it.
Circular thinking can help you ask more insightful questions, which is essential in an interview. By considering different viewpoints, you can create a conversation that's more dynamic and engaging.
The article highlights the importance of active listening in circular thinking. This means paying close attention to what the other person is saying and responding thoughtfully.
In an interview, circular thinking can help you build rapport with the interviewer and create a more positive atmosphere. By being open to their perspective and showing genuine interest, you can establish a connection that goes beyond just the questions and answers.
By adopting a circular approach, you can turn an interview into a conversation that's more like a discussion. This can help you stand out from other candidates and make a more lasting impression.
Discover more: Why Is It Important to Create Measurable Goals
Why Ask?
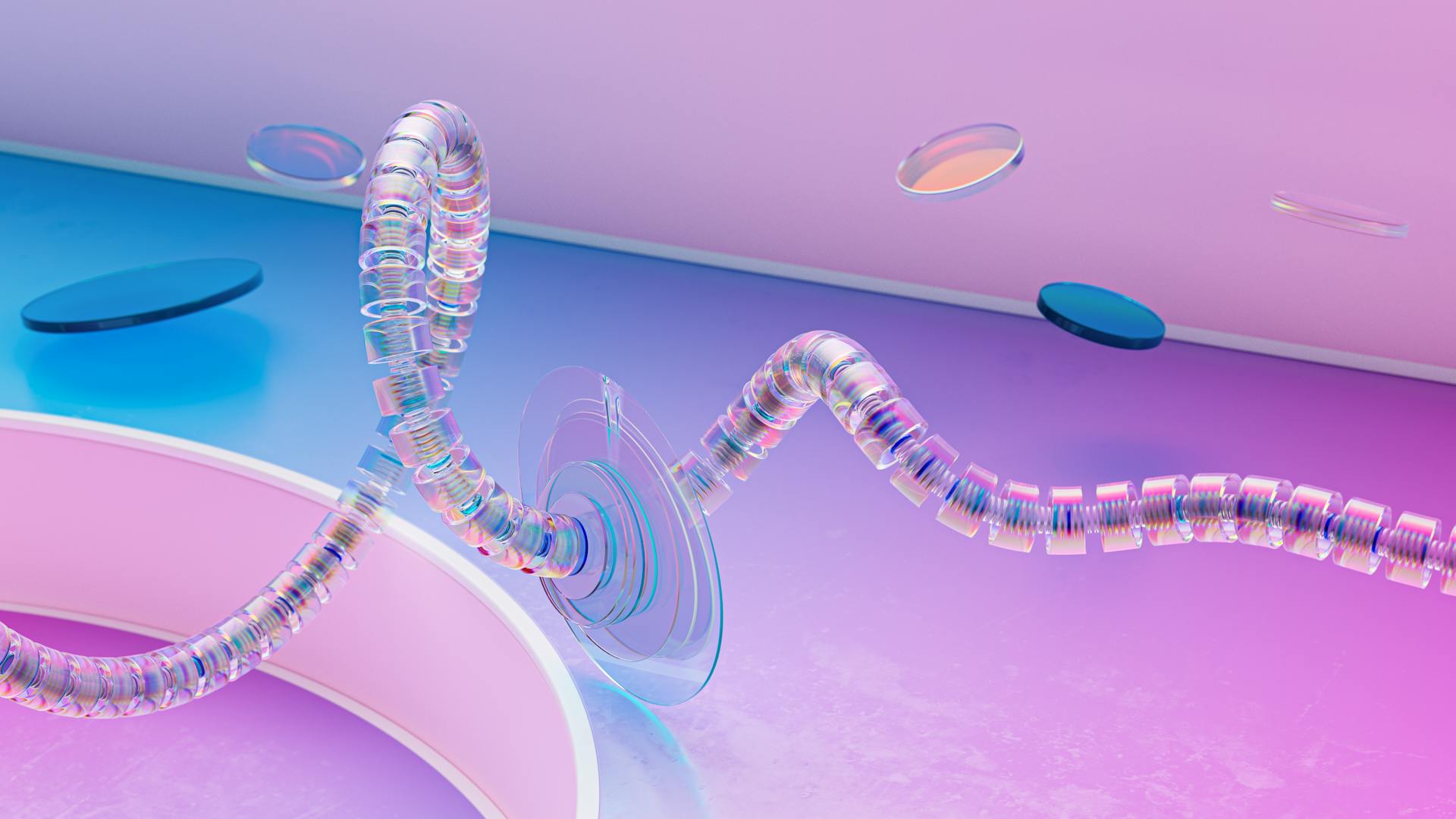
So, you're wondering why linked list questions are still a staple in programming interviews. Many of us don't work in low-level languages anymore, so it's not like we need to manipulate linked lists in our daily work. But, it's crucial for good programming, and some people just can't wrap their heads around pointers.
The source of these famous interview questions, like "reversing a linked list" or "detect loops in a tree structure", is largely due to the influence of Joel Spolsky and his book "The Guerilla Guide." This book was a launching point for building interview processes, and Spolsky was a minor tech celebrity at the time, so it's no wonder linked list questions became a thing.
In fact, Gayle Laakmann McDowell's book "Cracking the Coding Interview" took off in 2008 and further popularized linked list questions. She based her book on her experiences at companies like Apple, Microsoft, and Google, where LL algorithms are directly relevant to their use cases. It's no surprise she placed an outsized focus on them in her book.
Take a look at this: Important Questions to Ask during an Interview
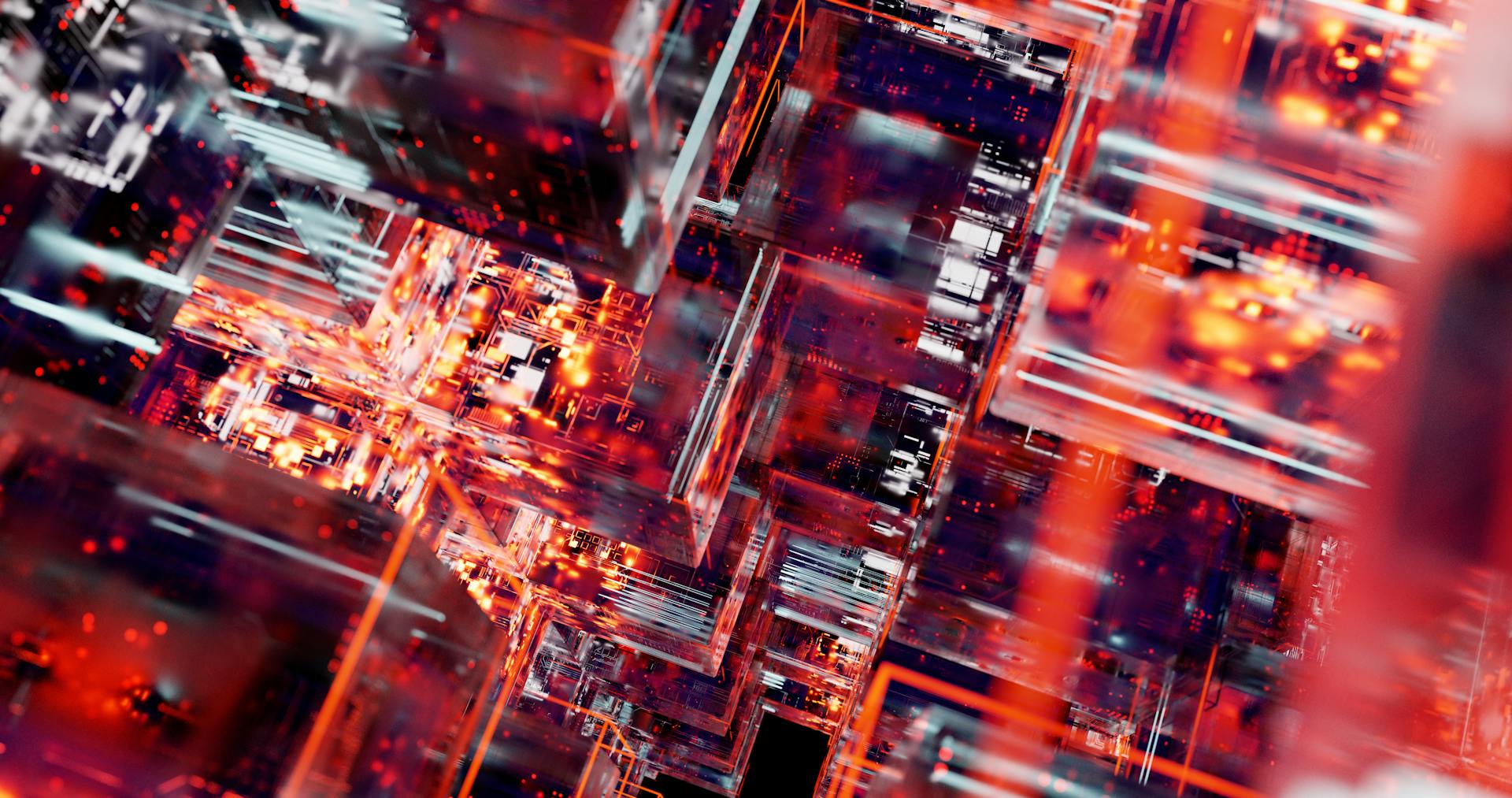
Here are some examples of why linked list questions are still asked in interviews:
- Print the Middle of a given linked list
- Reverse a Linked List
- Reverse a Doubly Linked List
- Rotate a linked list
- Nth node from end of linked list
- Delete last occurrence of an item from linked list
These questions are still relevant today, even if we don't work in low-level languages anymore. They test our ability to think critically and solve problems, which are essential skills for any programmer.
Top 50 Problems
Preparing for linked list interview questions can be a daunting task, but having a solid grasp of the top problems can make all the difference.
The top 50 problems on linked lists can be found on Leetcode, Code chef, and GFG IDE, which cover each aspect of linked lists.
These problems are enough to prepare for interview questions based on linked lists.
In fact, having a strong understanding of these problems can help you ace your next coding interview.
The problems on linked lists include deleting nodes, reversing a linked list, and detecting cycles, among others.
Understanding these problems will not only improve your coding skills but also boost your confidence in tackling complex interview questions.
Suggestion: Why Is Initializing so Important in Coding
Data Structure Challenges
Mastery of linked lists is a fundamental requirement in technical interviews, particularly in software engineering roles. Linked lists are a type of data structure commonly encountered in interviews, and understanding them is crucial for solving complex problems efficiently.
Many interview questions still focus on linked lists, despite the shift away from low-level languages. This is because linked lists require a complex form of doubly-indirected thinking, which is essential for good programming.
The Guerilla Guide and Cracking the Coding Interview played a significant role in popularizing linked list questions. These resources provided a good launching point for building interview processes and made linked list questions a staple in technical interviews.
Here are some common linked list design problems:
These design problems require a deep understanding of linked lists and are commonly asked in technical interviews.
Mastering Linked Lists
Mastering Linked Lists is crucial for acing technical interviews. Linked lists are fundamental data structures that demonstrate a solid understanding of data structures and help in solving complex problems efficiently.
In a linked list, the head is the entry point or the first node in the list. Each node contains two parts: the data it holds and a reference (pointer) to the next node. This allows for efficient insertion and deletion of nodes.
Here's a quick rundown of the key components of a linked list:
By understanding these components and mastering linked lists, you'll be well-prepared to tackle technical interview questions and impress your interviewer.
Understanding
A linked list is a linear data structure consisting of nodes, where each node contains data and a reference (or pointer) to the next node in the sequence.
Each node in a linked list is made up of two parts: data and a pointer/reference. The data holds the actual value or payload, while the pointer/reference points to the next node in the sequence.
This structure allows for dynamic memory management, unlike arrays which require contiguous memory allocation. The lack of contiguous memory allocation makes linked lists more flexible and efficient in terms of memory usage.
Additional reading: Why Is Parallel Structure Important
A linked list typically starts with a head node, which is the entry point or the first node in the list. The head node contains data and a reference to the next node in the sequence.
The last node in the list is called the tail, and its reference points to NULL, indicating the end of the list.
Here's a summary of a linked list's components:
- Head: The entry point or the first node in the list.
- Nodes: Each node contains data and a pointer/reference to the next node.
- Pointer/Reference: Points to the next node in the sequence.
- Tail: The last node in the list whose reference points to NULL.
Master with Code Examples & Interview Questions
Mastering linked lists is a must-have skill for any software engineer, as it demonstrates a solid understanding of data structures and helps in solving complex problems efficiently.
Mastery of linked lists is often a key requirement in technical interviews, especially in software engineering roles.
Linked lists are fundamental data structures that you'll encounter frequently in your coding journey.
They're commonly used to implement dynamic memory allocation, efficient insertion and deletion of elements, and more.
To ace your interview preparations, you'll need to understand the basics of linked lists, including their operations and advanced techniques.
Mastery of linked lists can help you solve problems more efficiently, making you a more effective and sought-after programmer.
With practice and dedication, you'll be able to implement linked lists like a pro and tackle even the toughest interview questions with confidence.
You might like: The Most Important Work You'll Ever Do
Design and Reordering
Design and Reordering is a crucial aspect of Linked Lists. These types of problems are frequently asked in interviews to check understanding of data structures.
Some examples of design problems related to Linked Lists include Design Browser History, LRU Cache, and Design Authentication Manager. These problems require using a combination of data structures, such as HashMaps and Linked Lists, to solve the problem efficiently.
The problem of flattening a multilevel doubly linked list is also a common design problem. This can be solved using a depth-first search approach, as hinted in the article.
Here's a list of some of the top design problems on Linked Lists:
- Design Browser History: (Amazon, Apple, Google, Microsoft, Snap)
- LRU Cache (Amazon, Google, Meta, Microsoft)
- Design Authentication Manager (X, LinkedIn)
- Flatten a Multilevel Doubly Linked List (Amazon, Apple, Google, Meta)
Design Problems
Design problems are a crucial aspect of linked list challenges. These problems are frequently asked in interviews to assess your understanding of different data structures.
One common design problem is the Browser History challenge, which has been asked by companies like Amazon, Apple, Google, Microsoft, and Snap. This problem requires you to design a data structure to efficiently manage browser history.
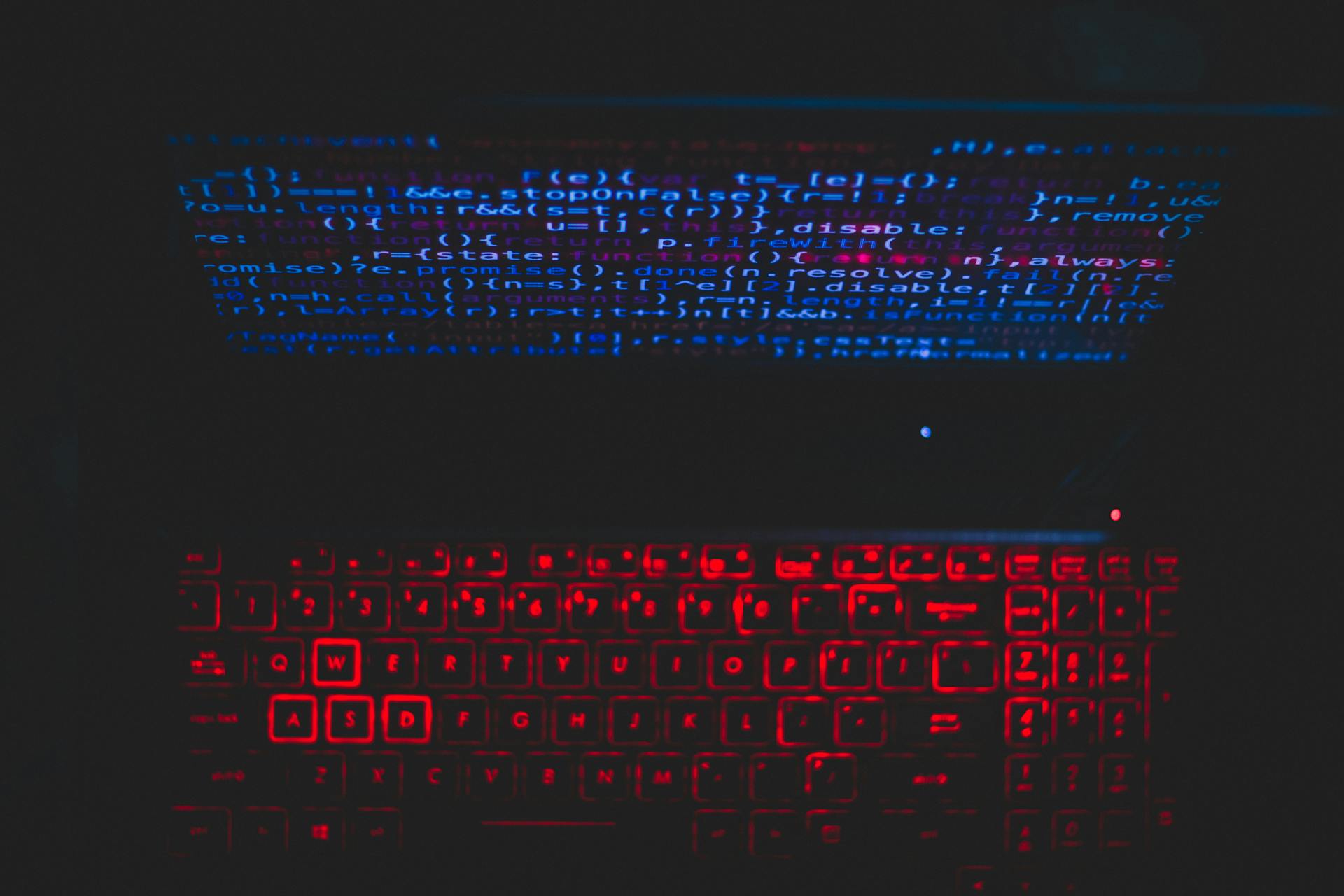
To solve this problem, you can use a combination of a HashMap and a Linked List. The HashMap can store tokens with their expiration times for O(1) access, while the Linked List maintains the order of tokens based on their expiration times.
Another design problem is the LRU Cache challenge, which has been asked by companies like Amazon, Google, Meta, and Microsoft. This problem requires you to design a data structure to efficiently manage cache expiration.
Here are some common design problems related to linked lists:
- Design Browser History: (Amazon, Apple, Google, Microsoft, Snap)
- LRU Cache (Amazon, Google, Meta, Microsoft)
- Design Authentication Manager (X, LinkedIn)
These design problems are essential to master linked list challenges. By understanding and solving these problems, you'll be well-prepared for technical interviews and data structure challenges.
Curious to learn more? Check out: What Are Your Most Important Cybersecurity Challenges
Reordering a List
Reordering a List is a common theme for linked list problems, where you're asked to rearrange an existing linked list. This can be a challenging task, but with the right approach, it's achievable.
One popular problem that involves reordering a linked list is Odd Even Linked List, problem 328. In this problem, you need to reorder the nodes in the linked list such that all odd-numbered nodes come before all even-numbered nodes.
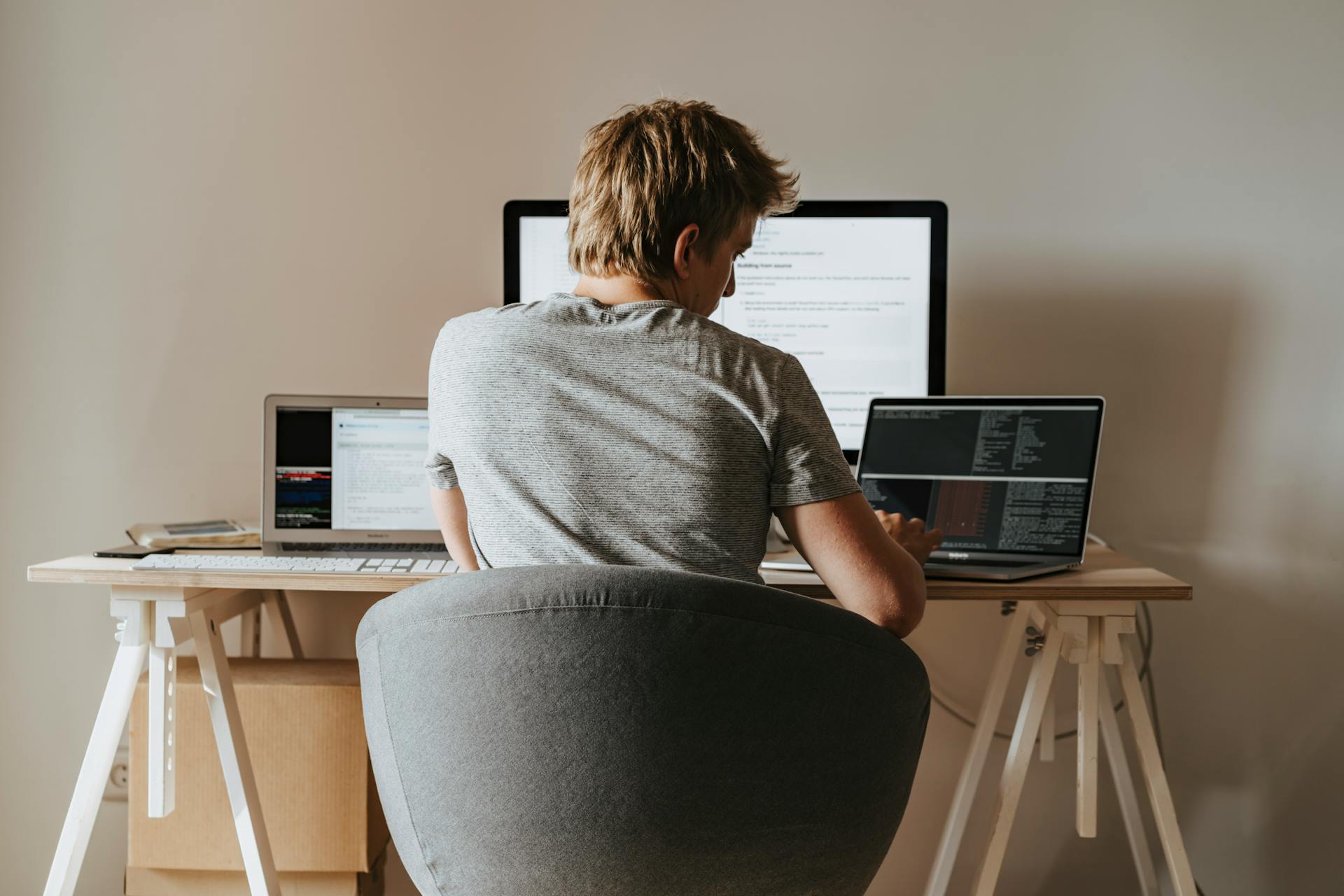
Reordering a linked list requires a clear understanding of the data structure and its properties. For example, you need to be able to traverse the list and identify the nodes that need to be rearranged.
Here's a summary of the key points to consider when reordering a linked list:
- Identify the nodes that need to be rearranged
- Understand the properties of the linked list data structure
- Use a clear and efficient approach to reorder the nodes
By following these steps and understanding the basics of linked list reordering, you'll be well on your way to solving problems like Odd Even Linked List.
Frequently Asked Questions
Are linked lists necessary?
Linked lists offer advantages over arrays, such as dynamic resizing and efficient insertion/deletion operations. While not always necessary, they can be a valuable choice for certain applications.
Why might you want to avoid using a linked list?
You might want to avoid using a linked list because it's expensive to access elements out of order, which can negatively impact performance. This is due to caching effects that affect most computing platforms.
Sources
- https://interviews.school/linkedlist
- https://www.architectalgos.com/linked-list-challenges-identifying-and-solving-key-problem-patterns-e2c674de37c2
- https://medium.com/pythoneers/mastering-linked-lists-for-interview-preparations-885e9d09fc88
- https://softwareengineering.stackexchange.com/questions/129604/is-it-bad-interview-practice-to-have-candidates-write-a-linked-list-implementati
- https://www.hillelwayne.com/post/linked-lists/
Featured Images: pexels.com