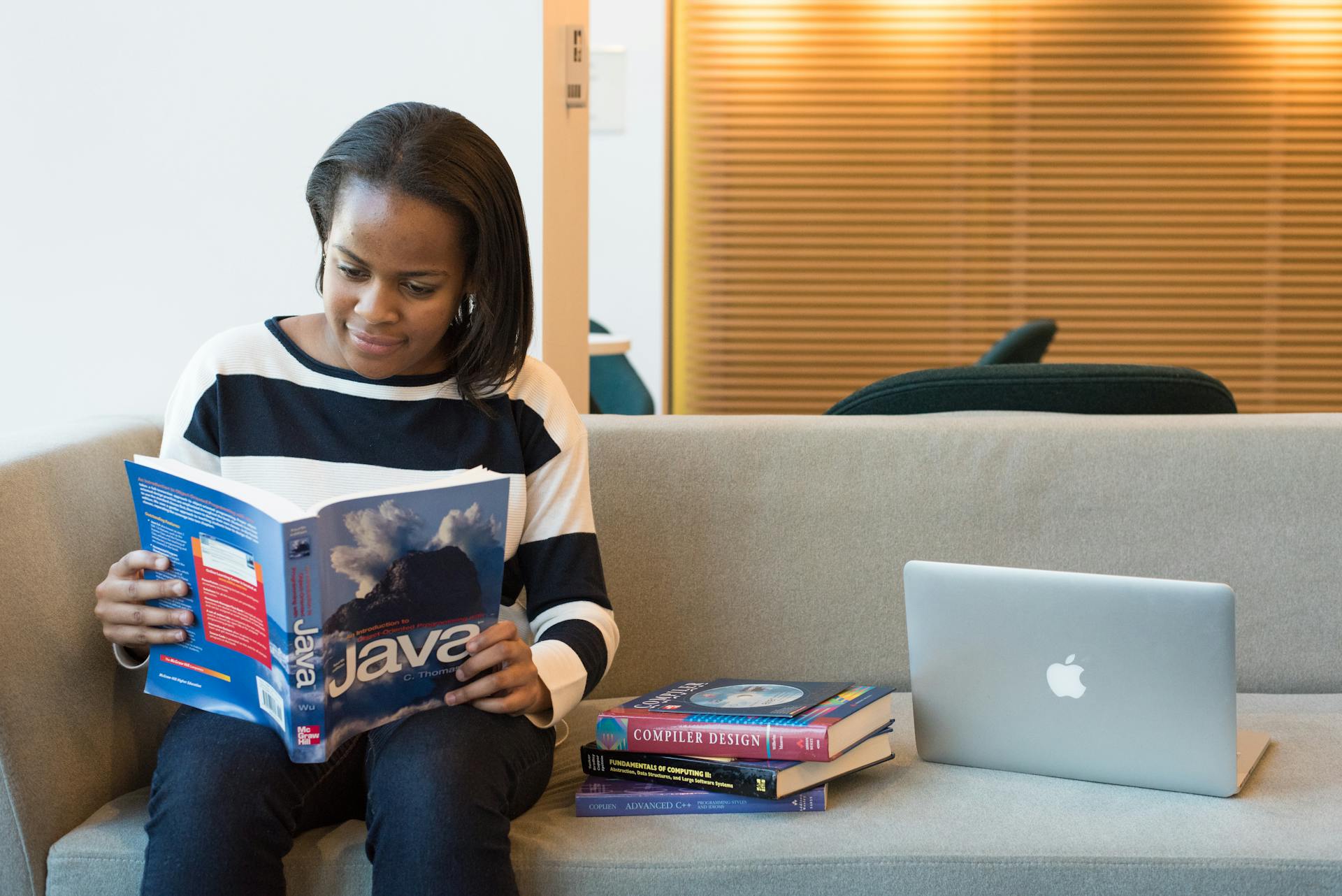
Java is a versatile and widely-used programming language that requires a solid understanding of its core concepts to master.
Object-Oriented Programming (OOP) is a fundamental concept in Java, which allows developers to create reusable code by organizing it into objects and classes.
In Java, variables are a crucial part of programming, and understanding the different types of variables is essential for effective coding.
Java has a vast range of data types, including primitive types such as int, double, and boolean, as well as reference types like String and Array.
Control structures like if-else statements and switch statements are used to control the flow of a program's execution based on certain conditions.
Methods are reusable blocks of code that perform a specific task, and learning how to create and use them efficiently is vital for writing clean and maintainable code.
Inheritance is a key concept in OOP that allows one class to inherit properties and behavior from another class, promoting code reusability and modularity.
Check this out: Why Is a Code of Conduct Important
Object-Oriented Programming
Object-Oriented Programming is a fundamental concept in Java that revolves around data and objects. It's all about bundling data and methods that manipulate it into objects, ensuring data integrity by encapsulating methods within them.
In Java, classes serve as blueprints for creating objects and defining their attributes and behaviors. This is a key principle of Object-Oriented Programming.
Encapsulation is a crucial aspect of OOP, protecting data by hiding it within objects and allowing controlled access via methods.
Abstraction simplifies complex systems by showing only the necessary features of an object, making it easier to understand and work with.
Inheritance enables one class to inherit attributes and methods from another, promoting code reuse and reducing duplication.
There are three primary types of variables in Core Java Concepts:
- Instance variables
- Local variables
- Static variables
These variables play a vital role in storing and manipulating data in Java programs.
Inheritance is the process of one class inheriting properties and methods from another class in Java. It's used when we have an "is-a" relationship between objects.
Expand your knowledge: Azure Java
There are several types of inheritance in Java, including:
- Single Inheritance: Class B inherits Class A using the extends keyword
- Multilevel Inheritance: Class C inherits Class B, and Class B inherits Class A using the extends keyword
- Hierarchy Inheritance: Class B and Class C inherit Class A in a hierarchical order using the extends keyword
- Multiple Inheritance: Class C inherits Class A and Class B (not supported in Java)
- Hybrid Inheritance: Class D inherits Class B and Class C (not supported in Java)
Polymorphism is the ability to perform many things in many ways. It's a critical feature of Object-Oriented Programming that allows objects to take multiple forms.
There are two types of polymorphism: static (compile-time) and dynamic (run-time) polymorphism. Static polymorphism is achieved through method overloading, while dynamic polymorphism is achieved through method overriding.
Data Types and Variables
Java is a strongly typed language, which means every piece of data or information is associated with a specific data type. This is crucial for defining and organizing information in Java.
Data types can be broadly classified into two categories: primitive and non-primitive. Java includes various primitive data types, each serving a distinct purpose.
Here are some examples of primitive data types in Java:
- boolean: Holds true or false values.
- byte: Stores whole numbers within the range of -128 to 127.
- short: Contains whole numbers within the range of -32768 to 32767.
- char: Represents a single character or ASCII value.
- int: Stores whole numbers within the range of -2147483648 to 2147483647.
- long: Holds larger whole numbers within the range of -9223372036854775808 to 9223372036854775807.
- float: Manages fractional numbers ranging from 3.4e−038 to 3.4e+038 (denoted with “f”).
- double: Manages larger fractional numbers, ranging from 1.7e−308 to 1.7e+308 (denoted with “d”).
Variables in Java are a fundamental concept representing a named memory location used to store data of a specific data type.
Non-Primitive Data Types
Non-Primitive Data Types are a crucial part of Java programming. They store references or addresses to the data's location, rather than the actual data itself.
These data types are also known as reference data types. Examples include classes, arrays, and interfaces. They are used for more complex data structures and custom-defined types.
Non-Primitive Data Types are created by the programmer, except for String. They enable method calls and can be null, unlike primitive types, which always hold values. Primitives begin with lowercase letters with varying sizes, whereas non-primitives start with uppercase letters with consistent sizes.
Here's a breakdown of the differences between Non-Primitive and Primitive Data Types:
Non-Primitive Data Types are an essential part of Java programming, allowing for more complex data structures and custom-defined types. They are used to store references or addresses to the data's location.
Operators
Operators are the backbone of any programming language, and Java is no exception. Operators are symbols that facilitate logical and mathematical operations on variables or identifiers.
Arithmetic Operators are used in mathematical expressions, including addition, subtraction, multiplication, and division.
The Ternary Operator acts as an if-then-else statement, evaluating a Boolean expression and returning one of two values based on its result. This operator is a powerful tool for simplifying code.
Relational Operators determine the relationship between two operands, such as equality, inequality, greater than, and less than. These operators are essential for making decisions in your code.
Assignment Operator, denoted by "=", assigns a value to a variable. This operator is used to store the result of an expression in a variable.
Unary Operators work on a single operand and encompass tasks like incrementing, decrementing, negating expressions, and inverting Boolean values. These operators are used to modify the value of a variable.
Intriguing read: Why Is Customer Lifetime Value Important
Control Flow Statements
Control Flow Statements are a crucial part of any Java program, allowing you to control the flow of execution and make decisions based on conditions.
These statements include if, else, for, while, and switch, which are all essential for writing efficient and effective Java code.
The if statement is used to check a condition and execute a block of code if it's true, while the else statement is used to execute a different block of code if the condition is false.
The for loop is used to iterate over a collection of items, and the while loop is used to execute a block of code as long as a certain condition is true.
The switch statement is used to execute a different block of code based on the value of a variable.
Here's a quick rundown of the main control flow statements in Java:
- if: used to check a condition and execute a block of code
- else: used to execute a different block of code if the condition is false
- for: used to iterate over a collection of items
- while: used to execute a block of code as long as a certain condition is true
- switch: used to execute a different block of code based on the value of a variable
Methods and Functions
Methods in Java are blocks of code that perform a specific task, making them essential for encapsulating a set of operations.
A method's signature includes its name, input parameters, and return type, which is crucial for understanding how parameters pass from the calling method to the called method and how the return value is sent back.
Java methods promote code reusability, reduce complexity, and simplify maintenance by eliminating duplicate code.
Check this out: Why Code Switching Is Important
Methods
Methods are blocks of code that perform a specific task. Understanding method declaration, parameters, and return types is essential.
In Java, methods are essential for encapsulating a set of operations that perform a specific function. They promote code reusability, reduce complexity, and simplify maintenance by eliminating duplicate code.
A method's signature includes its name, input parameters, and return type. Understanding how parameters pass from the calling method to the called method and how the return value is sent back is crucial when working with methods.
These core concepts are fundamental to effective Java programming.
Expand your knowledge: Why Is It Important to Perform Data Analysis on Datasets
Lambda Expressions
Lambda expressions provide a concise way to express instances of single-method interfaces (functional interfaces).
In Java, lambda expressions are a powerful tool for simplifying code. They allow you to create small, anonymous functions that can be used in place of traditional methods.
Lambda expressions are often used to implement functional interfaces, which are interfaces that have only one abstract method. This makes them perfect for creating small, focused functions.
Here's an example of how lambda expressions can be used to implement a functional interface:
- Baeldung — Java Lambda Expressions
Sources
- https://medium.com/@ThatTechBro/mastering-java-top-20-fundamental-concepts-every-developer-should-learn-f7d1abad5656
- https://pwskills.com/blog/13-top-core-java-concepts-all-java-programmers-need-to-know/
- https://www.tutorialspoint.com/what-are-the-concepts-every-java-programmer-must-know
- https://www.geeksforgeeks.org/four-main-object-oriented-programming-concepts-of-java/
- https://jenkov.com/tutorials/java/core-concepts.html
Featured Images: pexels.com