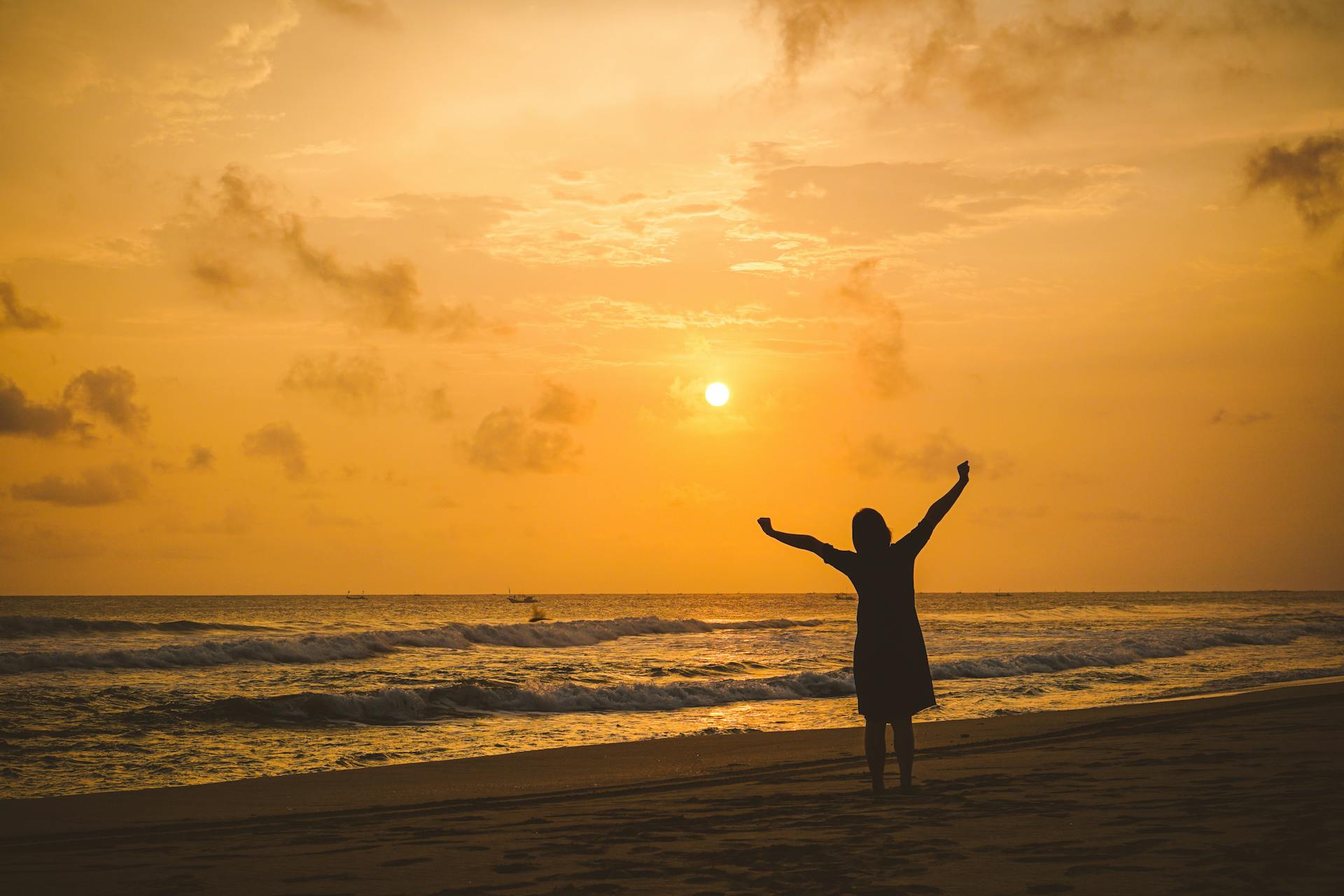
Azure provides a managed service for Java called Azure App Service, which allows you to deploy and run Java applications in the cloud.
This service supports Java 8 and later versions, and you can choose from several deployment options, including containerized and non-containerized deployments.
With Azure App Service, you can take advantage of features like auto-scaling, load balancing, and SSL/TLS encryption to ensure your Java application is scalable, secure, and high-performing.
Azure also offers a Java runtime environment that includes the Java Development Kit (JDK) and the Java Runtime Environment (JRE), which you can use to develop, test, and deploy Java applications in the cloud.
For more insights, see: Java Azure Openai
Getting Started
Azure Java is a great platform to get started with, and I'm excited to guide you through the process.
First, you'll need to install the Azure SDK for Java, which you can download from the official Azure website.
You can create a new Azure account for free and start exploring the platform.
For more insights, see: Windows Azure Sdk for Java
To get started with Azure Java, you'll need to have Java Development Kit (JDK) installed on your system.
The Azure SDK for Java provides a simple and intuitive way to interact with Azure services.
You can use the Azure CLI to manage your Azure resources and services from the command line.
Make sure you have the Azure subscription and the correct permissions to use the services.
Azure provides a wide range of services, including compute, storage, and database services, all of which can be accessed through the Azure SDK for Java.
You can use the Azure portal to manage your resources and services visually.
Curious to learn more? Check out: How to Use Azure Key Vault C#
Azure Java Basics
To get started with Azure Java, you'll need a few basic things. You'll need an Azure account with an active subscription, which can be created for free.
To deploy and manage Java applications, you'll need an Azure Storage account and Java Development Kit (JDK) version 8 or above, along with Apache Maven.
Here are the prerequisites you'll need to get started:
Prerequisites
To get started with Azure Java, you'll need a few things. First, you'll need an Azure account with an active subscription, which you can create for free.
You'll also need an Azure Storage account, which you can create in your Azure dashboard.
Java Development Kit (JDK) version 8 or above is required for Azure Java development.
Apache Maven is another essential tool you'll need for Azure Java development.
Here's a quick rundown of the prerequisites:
Setting Up
To get started with Azure Java, you'll need to set up a few things. First, you'll need an Azure account with an active subscription, which you can create for free.
You'll also need an Azure Storage account, which you can create separately. Then, you'll need to install the Java Development Kit (JDK) version 8 or above and Apache Maven.
In addition, you'll need to install the Azure Developer CLI.
Here's a quick rundown of the prerequisites:
Once you have these prerequisites set up, you can start preparing your project to work with the Azure Blob Storage client library for Java.
Function Basics
A Java function in Azure is a public method decorated with the @FunctionName annotation. This method defines the entry point for a Java function and must be unique in a particular package.
In Azure, a single package is deployed to a function app, which provides the deployment, execution, and management context for individual Java functions.
The package can have multiple classes with multiple public methods annotated with @FunctionName.
Programming Model
In Azure Functions, triggers start the execution of your code. Triggers are the fundamental building blocks of Azure Functions, and they determine when your code is executed.
You can add triggers to your Azure Functions to start the execution of your code. Triggers are used to connect to data from within your code.
To add triggers, you can use the concepts of bindings, which give you a way to pass data to and return data from a function, without having to write custom data access code.
For another approach, see: Azure Code
Bindings in Azure Functions are used to connect to data from within your code. A function can have multiple input and output bindings.
Here's a breakdown of the types of bindings you can use in Azure Functions:
You can use bindings to connect to various data sources, such as Table storage and Queue storage.
A unique perspective: Azure Blob Storage Move Files between Containers C#
Supported Versions
Azure supports Java versions 8, 11, and 17 for Functions runtime on Windows, and versions 21 (Preview), 17, 11, and 8 on Linux.
For Functions version 4.x, you can use Java 17, 11, or 8 on Windows, and Java 21 (Preview), 17, 11, or 8 on Linux.
If you don't specify a Java version, the Maven archetype defaults to Java 8 during deployment to Azure.
Here is a table showing the supported Java versions for each major version of the Functions runtime:
Dependency Injection in Functions
Dependency Injection in Functions is a technique to achieve Inversion of Control between classes and their dependencies.
Azure Functions Java supports Dependency Injection through the FunctionInstanceInjector interface, allowing frameworks like Spring, Quarkus, and Google Guice to create function instances and register them into their IOC container.
This means you can use Dependency Injection frameworks to manage your functions naturally, giving you more control over your code.
Microsoft Azure Functions Java SPI Types is a package that contains all SPI interfaces for third parties to interact with the Microsoft Azure functions runtime.
The FunctionInstanceInjector interface is the key to integrating Dependency Injection frameworks with Azure Functions Java, and it's part of the azure-function-java-spi package.
For more examples of using FunctionInstanceInjector to integrate with Dependency Injection frameworks, you can refer to the repository mentioned in the documentation.
A different take: Azure Functions Dependency Injection
Azure Java Deployment
Azure Java Deployment is a straightforward process that allows you to deploy your Java applications to Azure. You can control the version of Java targeted by the Maven archetype by using the -DjavaVersion parameter, with values 8, 11, 17, or 21.
To deploy your Java application to Azure, you can use the Maven plugin for the Web Apps feature of Azure App Service. This allows you to create and package your Java web app and deploy it to Azure.
The operating system on which your function app runs in Azure can also be specified using the os element, with options for Windows, Linux, and Docker.
Azure provides a range of tools and services to help you build, debug, and deploy Java applications, including Eclipse, IntelliJ, and Visual Studio Code for Java, as well as GitHub Copilot.
Here are the Java versions that can be targeted by the Maven archetype:
Azure also supports the deployment of Spring Boot microservices, Java EE applications, and Tomcat-based Java applications, among others.
Azure Java Features
Azure Java offers a range of features that make it an ideal choice for developers.
Azure Java supports Java 8 and later versions, allowing developers to leverage the latest Java features and libraries.
With Azure Java, you can deploy your Java applications to the cloud with ease.
Azure provides a managed Java runtime environment that eliminates the need for manual configuration and maintenance.
Azure Java allows you to leverage the power of Azure services, such as Azure Storage, Azure Cosmos DB, and Azure Active Directory.
This enables developers to build scalable and secure Java applications that integrate seamlessly with other Azure services.
Azure Java provides a range of tools and frameworks for building and deploying Java applications, including the Azure Toolkit for Eclipse and the Azure Toolkit for IntelliJ.
These tools simplify the development and deployment process, making it easier to get your Java applications up and running in the cloud.
Azure Java Development
Azure Java development offers a range of tools and frameworks to build, debug, and deploy Java applications on Azure. You can use your favorite IDEs, including Eclipse, IntelliJ, and Visual Studio Code for Java, with GitHub Copilot.
Developers can leverage serverless development models, which allow them to build and run applications without managing servers. This approach abstracts away servers from application development, making it easier to scale and manage infrastructure.
To get started with Azure Java development, you can initialize the Azure Developer CLI template and deploy resources. This involves cloning the quickstart repository assets from GitHub, initializing the template locally, logging in to Azure, and provisioning and deploying resources to Azure.
To scaffold Java-based function projects, you can use Apache Maven archetypes, specifically the Java Maven archetype for Azure Functions, which is published under the groupId:artifactId: com.microsoft.azure:azure-functions-archetype.
The folder structure of an Azure Functions Java project typically includes a shared host.json file, code files (.java), and binding configuration files (function.json). Each function has its own code file and binding configuration file, and you can have multiple functions in a single project.
Here's a summary of the key Azure Java development tools and frameworks:
- GitHub Copilot for coding and deployment
- Apache Maven archetypes for project scaffolding
- Azure Developer CLI template for deploying resources
- Java Maven archetype for Azure Functions
Folder Structure
When organizing your Azure Java project, it's essential to understand the folder structure.
You can use a shared host.json file to configure the function app.
Each function has its own code file (.java) which is where you'll write your Java code.
A binding configuration file (function.json) is also required for each function.
You can have multiple functions in a single project, but it's not recommended to put them into separate jars.
The FunctionApp in the target directory is what gets deployed to your function app in Azure.
Recommended read: Azure Auth Json Website Azure Ad Authentication
Serverless Development Model
In Azure Java development, serverless is a cloud-native development model that allows developers to build and run applications without having to manage servers.
Servers still exist in the serverless model, but they're abstracted away from application development, making it easier to focus on writing code.
This model is made possible by cloud service providers that automatically provision, scale, and manage the infrastructure required to run the code.
You might like: Test Azure Function Locally
Spring Apps
Spring Apps is a great way to deploy, operate, and scale your Spring Boot apps in a fully managed environment with Azure Spring Apps.
You can easily deploy, operate, and scale your Spring Boot apps in a fully managed environment with Azure Spring Apps. Get even more functionality with Azure Spring Apps Enterprise, which adds fully managed VMware Tanzu components, advanced configurability, and Spring Runtime support.
Azure Spring Apps offers quickstart guides to help you get started. You can also learn more about Azure Spring Apps to understand its features and benefits.
To supercharge your Spring Boot apps, you can watch a demo that shows how to deploy apps to Azure Spring Apps and take advantage of features like autoscaling, monitoring, and end-to-end automation.
You can deploy Spring Boot microservices to Azure using Azure Spring Apps. This involves creating an Azure Spring Apps cluster, building different Spring Boot microservices, configuring a Spring Apps Config server, and building a Spring Apps Gateway.
Some popular Java platforms for building or migrating Java applications are Java SE, Jakarta EE, and MicroProfile.
Take a look at this: Azure Function Environment Variable
Frequently Asked Questions
Does Azure DevOps use Java?
Azure DevOps Services is built with Java teams in mind, but it doesn't exclusively use Java - it supports various programming languages and platforms.
Sources
- https://azure.github.io/azure-sdk/releases/latest/java.html
- https://www.geeksforgeeks.org/microsoft-azure-build-and-deploy-app-with-azure-sdk-for-java/
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-reference-java
- https://azure.microsoft.com/en-us/resources/developers/java
- https://learn.microsoft.com/en-us/azure/architecture/guide/technology-choices/service-for-java-comparison
- https://learn.microsoft.com/en-us/azure/storage/blobs/storage-quickstart-blobs-java
Featured Images: pexels.com