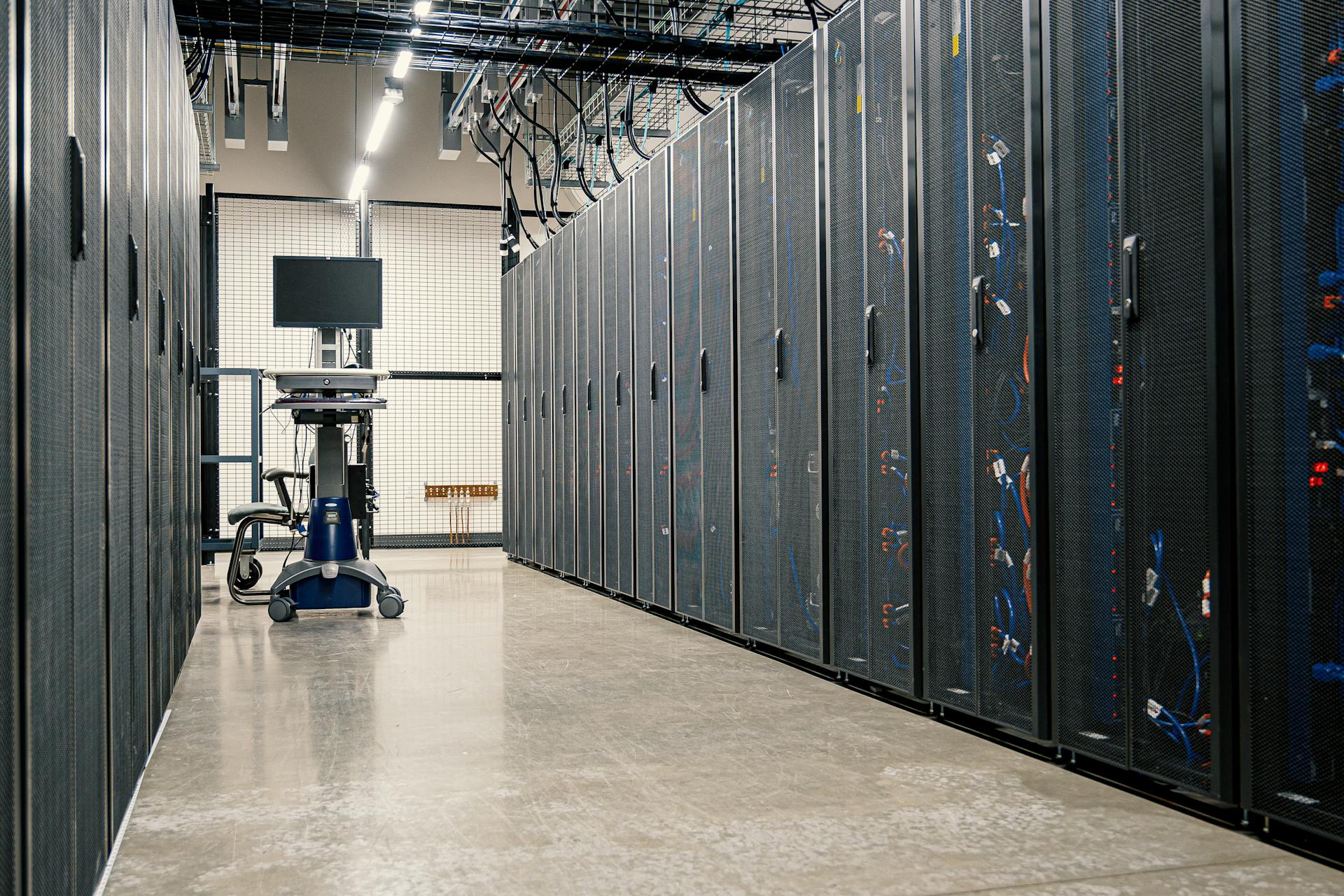
To integrate Java Azure OpenAI, you need to install the Azure OpenAI SDK for Java, which provides a set of APIs to interact with the OpenAI services.
First, you need to create an Azure account and subscribe to the Azure Cognitive Services or Azure Machine Learning plan to access the OpenAI services. This will give you a subscription key to use in your Java application.
Next, you need to install the Azure OpenAI SDK for Java using Maven or Gradle in your project. This will provide you with the necessary libraries to make API calls to the OpenAI services.
Suggestion: Azure Java
Prerequisites
To get started with Java Azure OpenAI, you'll need to meet the following prerequisites.
You'll need a Java Development Kit (JDK) with version 8 or above installed on your system. This will provide the necessary tools for developing and running Java applications.
An Azure Subscription is also required, which will give you access to the Azure OpenAI Service.
You'll also need to have Azure OpenAI access, which will allow you to use the service to generate text.
Finally, make sure to complete the Quickstart: Get started generating text using Azure OpenAI Service to ensure you have everything set up correctly.
Explore further: Windows Azure Sdk for Java
Setting Up
To set up the Azure Open AI SDK for Java, you'll need to create a token and an endpoint to communicate with the Azure Open AI service. This involves setting up your environment.
The first step is to create an AzureOpenAiChatModel instance. This is done by adding the spring-ai-azure-openai dependency to your project's Maven pom.xml file or Gradle build.gradle build file.
Next, you'll need to create an AzureOpenAiChatModel instance and use it to generate text responses. This is where the magic happens, and you can start using the Azure Open AI service.
To enable the AzureOpenAiChatModel, you'll need to add the following dependency to your project's build file: spring-ai-azure-openai.
The Deployment Name is actually the gpt-4o, which you can find in the Azure AI Portal.
Related reading: Spring Cloud Alibaba
Authentication
To interact with the Azure OpenAI Service, you'll need to create an instance of the OpenAIAsyncClient or OpenAIClient class using the OpenAIClientBuilder.
The first step is to configure a client for use with Azure OpenAI, which requires providing a valid endpoint URI to an Azure OpenAI resource.
You'll also need a corresponding key credential, token credential, or Azure Identity credential that's authorized to use the Azure OpenAI resource.
To get a key credential, navigate to the Azure Portal.
Broaden your view: Azure Openai Key
Azure Integration
To integrate Azure with your Java project, you'll need to obtain an Azure OpenAI endpoint and api-key from the Azure Portal. This can be done by following the steps outlined in the Azure API Key & Endpoint section.
You'll need to set two properties in your project: spring.ai.azure.openai.api-key and spring.ai.azure.openai.endpoint. The api-key is obtained from Azure, and the endpoint URL is obtained when provisioning your model in Azure.
Here are the properties you'll need to set:
- spring.ai.azure.openai.api-key: Set this to the value of the API Key obtained from Azure.
- spring.ai.azure.openai.endpoint: Set this to the endpoint URL obtained when provisioning your model in Azure.
Azure API Key
To obtain your Azure API Key, you'll need to visit the Azure Portal and head to the Azure OpenAI Service section. This is where you'll find the API Key and endpoint URL you'll need to get started.
The API Key is a crucial piece of information that you'll need to set in your application. Specifically, you'll need to set the `spring.ai.azure.openai.api-key` property to the value of the API Key obtained from Azure.
To do this, you'll also need to set the `spring.ai.azure.openai.endpoint` property to the endpoint URL obtained when provisioning your model in Azure. This will ensure that your application is properly configured to interact with the Azure OpenAI service.
Here are the specific properties you'll need to set:
By following these simple steps, you'll be well on your way to integrating your application with the Azure OpenAI service and unlocking its powerful capabilities.
Auto-Configuration
Auto-configuration is a breeze with Spring AI. Spring AI provides Spring Boot auto-configuration for the Azure OpenAI Chat Client. To enable it, add the following dependency to your project’s Maven pom.xml or Gradle build.gradle build files. This allows you to quickly get started with Azure integration without much fuss.
Java SDK
To get started with the Java SDK, you'll need to set up your environment by creating a token and an endpoint, which will allow you to communicate with the Azure Open AI service.
This involves a few steps, but don't worry, it's a straightforward process. Once you've set up your environment, you can choose the model you want to deploy and configure various options.
With the SDK set up, you'll be ready to dive into using it to create powerful AI applications.
Suggestion: Azure Test Environment
Setting Up Java SDK
To set up the Java SDK, you'll need to create a token and an endpoint, which will allow you to communicate with the Azure Open AI service.
This process involves setting up your environment, a crucial step before you can start using the Azure Open AI SDK for Java.
Creating a token will give you the necessary credentials to authenticate with the Azure Open AI service, while setting up an endpoint will determine how you'll communicate with the service.
Once you've set up your environment, you can choose the model you want to deploy and configure various options, such as configuring the model's parameters and settings.
With your environment set up, you'll be ready to start using the Java SDK and exploring its capabilities.
Worth a look: Azure Dev Environment
Function Calling
You can register custom Java functions with the AzureOpenAiChatModel and have the model intelligently choose to output a JSON object containing arguments to call one or many of the registered functions.
This technique is a powerful way to connect the LLM capabilities with external tools and APIs.
Read more about Azure OpenAI Function Calling.
A different take: Google Cloud Functions Python
Sample Controller
Creating a Sample Controller in Java SDK is a straightforward process. You can create an AzureOpenAiChatModel implementation that can be injected into your class.
To do this, you'll need to create a simple @Controller class that uses the chat model for text generations. This is similar to the example provided in the Sample Controller section.
The AzureOpenAiChatModel implementation can be used for text generations, which is a key feature of the Java SDK.
Readers also liked: Webservices in Java
Multimodal
The Azure OpenAI gpt-4o model offers multimodal support, allowing it to understand and process information from various sources.
Multimodality refers to a model's ability to simultaneously understand and process information from text, images, audio, and other data formats. The Azure OpenAI can incorporate a list of base64-encoded images or image URLs with the message. You can pass multiple images as well.
Spring AI's Message interface facilitates multimodal AI models by introducing the Media type, which encompasses data and details regarding media attachments in messages. This type utilizes Spring's org.springframework.util.MimeType and a java.lang.Object for the raw media data.
The code example from OpenAiChatModelIT.java illustrates the fusion of user text with an image using the GPT_4_O model. It takes as an input the multimodal.test.png image along with the text message "Explain what do you see on this picture?" and generates a response.
Access the Model
To access the OpenAI Model, you can configure the client to use it directly instead of the Azure OpenAI deployed models. Set the spring.ai.azure.openai.openai-api-key to your OpenAI Key.
If you want to use the Azure OpenAI, you need to set the spring.ai.azure.openai.api-key to your Azure OpenAI Key.
The spring-ai-azure-openai dependency provides access to the AzureOpenAiChatModel, which you can learn more about in the Azure OpenAI Chat section.
For more insights, see: Azure Openai Api
Multimodal
Multimodal refers to a model's ability to understand and process information from various sources, including text, images, audio, and other data formats. The Azure OpenAI gpt-4o model offers multimodal support, allowing it to incorporate a list of base64-encoded images or image URLs with the message.
The Azure OpenAI can handle multiple images, and the Spring AI's Message interface facilitates multimodal AI models by introducing the Media type. This type encompasses data and details regarding media attachments in messages, utilizing Spring's org.springframework.util.MimeType and a java.lang.Object for the raw media data.
You can pass multiple images as well, and the Azure OpenAI can take an input like the multimodal.test.png image. Along with the text message "Explain what do you see on this picture?", it generates a response.
Here are some key features of multimodal:
- Support for text, images, audio, and other data formats
- Ability to incorporate base64-encoded images or image URLs with the message
- Handling of multiple images
- Use of Media type for media attachments in messages
Highlights and Conclusion
The Azure Open AI service offers cutting-edge language processing capabilities, making it a game-changer for developers. You can access these capabilities through the Azure Open AI SDK for Java, which allows for seamless integration and utilization of the service's functionalities.
Chat completions enable interactive conversations between users and the Open AI model, with the ability to prompt accurate or creative answers. Sentiment analysis helps analyze the sentiment of statements or phrases, providing valuable insights. Image generation allows for the creation of custom images based on prompts, opening up creative possibilities.
Here are some of the key features of the Azure Open AI SDK for Java:
- Chat completions
- Sentiment analysis
- Image generation
- Word ladders
With these features, developers can create intelligent and interactive applications that elevate their Java projects to the next level.
Highlights
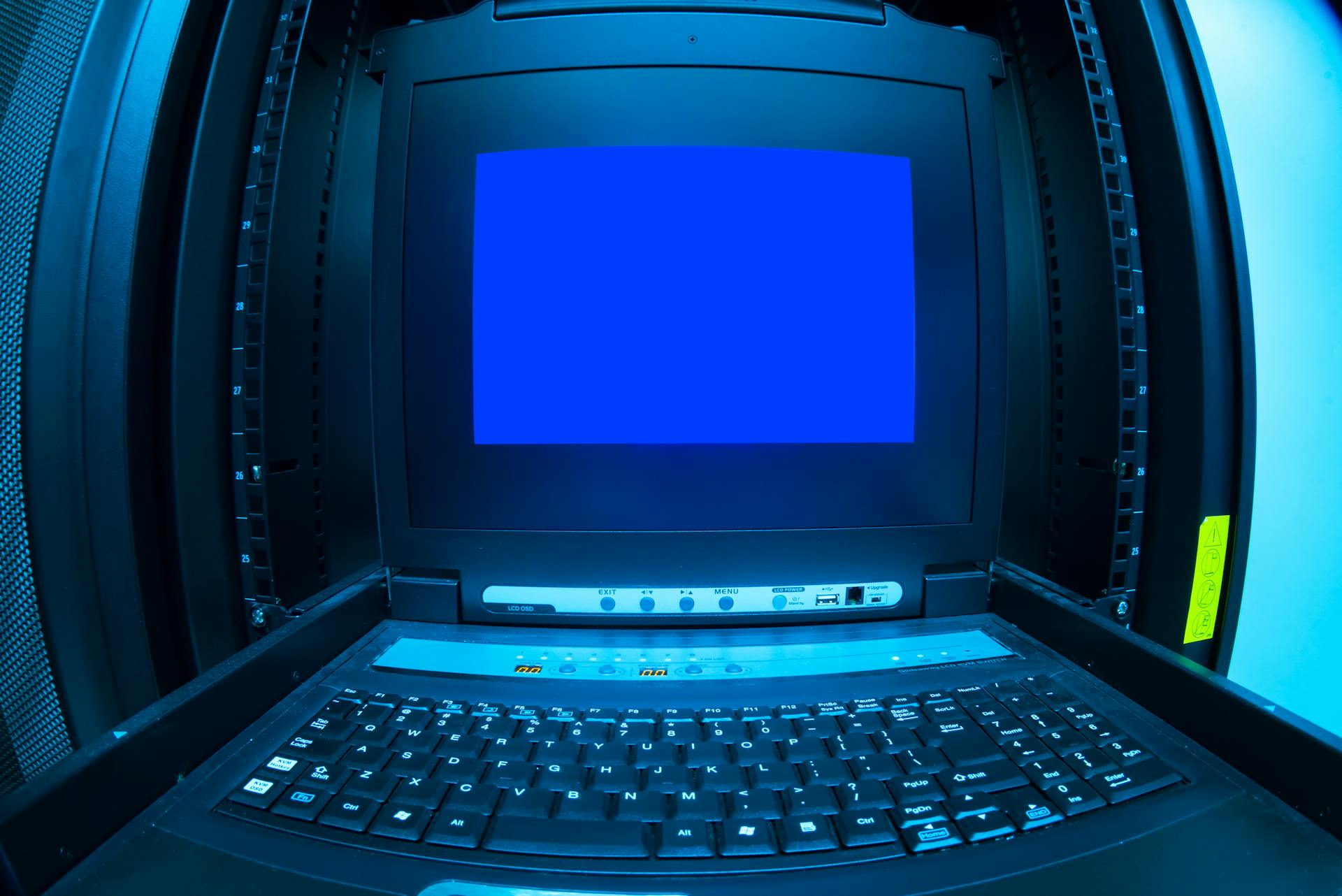
The Azure Open AI service offered by Microsoft provides access to cutting-edge language processing capabilities. This allows for seamless integration and utilization of the service's functionalities.
Chat completions enable interactive conversations between users and the Open AI model, with the ability to prompt accurate or creative answers. You can also use sentiment analysis to analyze the sentiment of statements or phrases, providing valuable insights.
The Azure Open AI SDK for Java offers developers a wide range of possibilities to enhance their applications with AI capabilities. This includes image generation, which allows for the creation of custom images based on prompts, opening up creative possibilities.
Here are some key features of the Azure Open AI service:
Word ladders are another useful feature that can be used to generate word puzzles, adding an interactive element to applications.
Conclusion
We've explored the capabilities of Azure Open AI service and its integration with Java applications through the Azure Open AI SDK.
A different take: Azure Ai Training
This integration empowers developers to create intelligent and interactive applications.
Chat completions, text completions, sentiment analysis, image generation, and word ladders are just a few features that can be easily incorporated into Java projects.
With the Azure Open AI SDK, Java developers can now create state-of-the-art language processing functionalities in their projects.
These features are designed to elevate Java applications to the next level.
So, what are you waiting for?
Recommended read: Azure Features
Sources
- https://docs.spring.io/spring-ai/reference/api/chat/azure-openai-chat.html
- https://learn.microsoft.com/en-us/java/api/overview/azure/ai-openai-readme
- https://learn.microsoft.com/en-us/azure/developer/java/ai/azure-ai-for-java-developers
- https://www.toolify.ai/gpts/learn-ai-with-java-coding-using-azure-openai-sdk-112137
- https://www.toolify.ai/ai-news/begin-your-ai-journey-with-java-using-azure-openai-sdk-984647
Featured Images: pexels.com