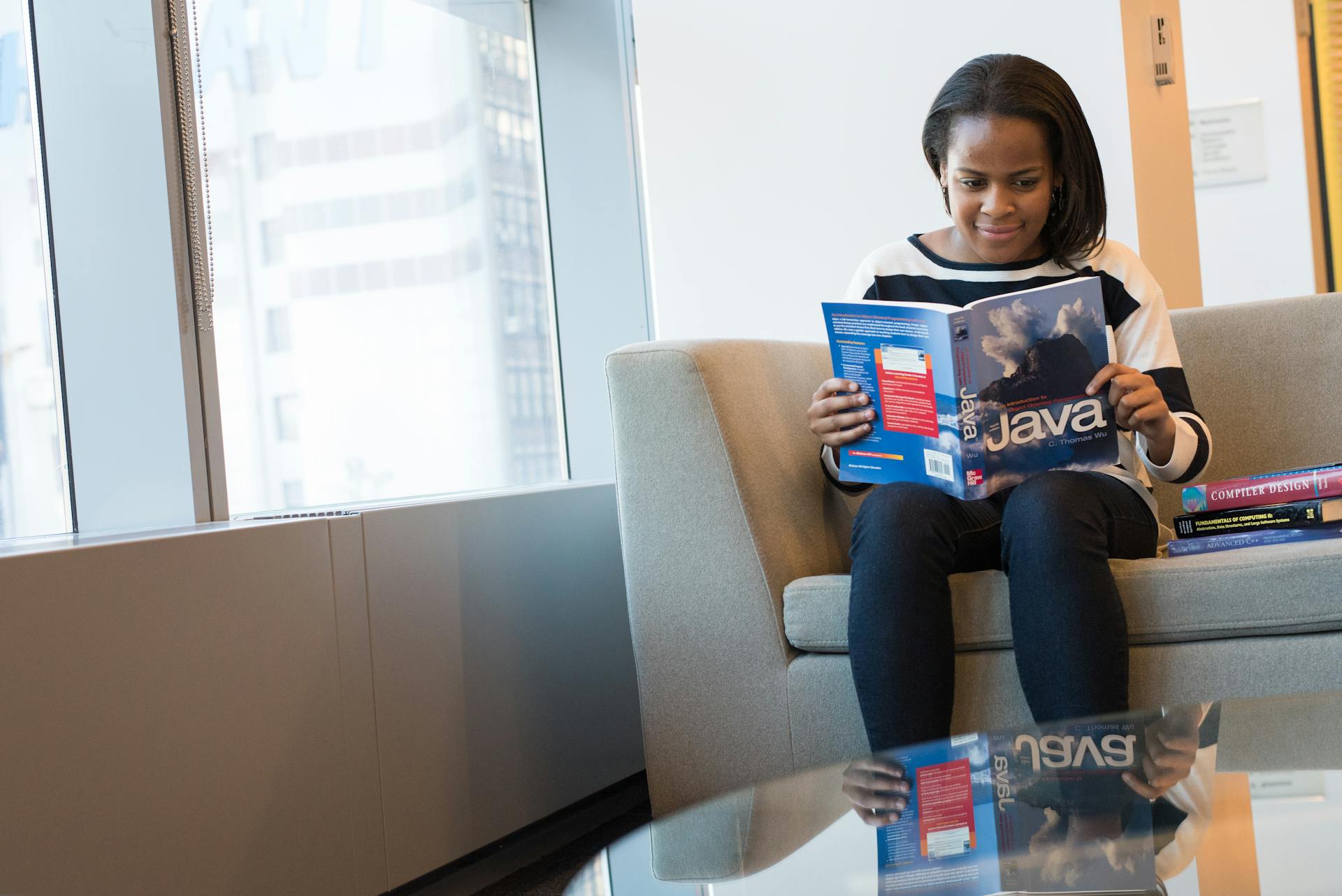
Webservices in Java are a way to communicate with other systems over a network. They allow different applications to exchange data in a standardized way.
A webservice is essentially a remote API that can be accessed over the internet. It's like a messenger that helps different systems talk to each other.
In Java, we can create a webservice using the Java API for RESTful Web Services (JAX-RS) or the Java API for XML-Based Web Services (JAX-WS). These APIs provide a set of annotations and classes that make it easy to create webservices.
To create a simple webservice, we can use the @Path annotation to specify the URL pattern for the webservice, and the @GET, @POST, @PUT, and @DELETE annotations to specify the HTTP method for each endpoint.
Readers also liked: Dropbox Java Api
Setting Up the Environment
To set up a dynamic web project, start by kicking off the dynamic web project creation wizard in Eclipse. Name the project restful-java and choose Apache Tomcat 8.5 as the target runtime, even though you're using TomEE Plus.
Choose a minimal configuration for the project, then click Finish. You'll need to specify 3.1 as the dynamic web module version.
This setup process is a crucial step in creating a JAX-RS web service.
You might like: Project Web Page Design
Create Eclipse Project
To create an Eclipse project, start by creating a dynamic web project in Eclipse. Name it soap-ws-example and use web module version 3.1.
Employ a minimal configuration and associate it with a runtime that supports the Java web profile. For this SOAP web services example in Java using Eclipse, WildFly 10.x is a suitable choice.
To create a JAX-RS web service, follow the same steps but name the project restful-java and choose Apache Tomcat 8.5 as the target runtime.
Here are the specific steps to create the Eclipse project:
By following these steps, you'll be able to create a basic Eclipse project for your SOAP or JAX-RS web service.
Setting Up NetBeans IDE
To set up NetBeans IDE, start by creating a simple web application. This will create a project, and you'll see the file index.jsp appear in the Source pane.
To create a RESTful web service, right-click the project and select New, then select RESTful Web Services from Patterns. This will create a new class, HelloWorld.java, where you can start implementing your web service.
Discover more: New Relic Java Agent
You can test the web service by right-clicking the project node and clicking Test RESTful Web Services. This will deploy the application and bring up a test client in the browser.
To deploy the web service, right-click the project and select Deploy. This will run the wsimport tasks, build and package the application into a WAR file, and deploy it to the server.
Here are the steps to follow in more detail:
- Create a simple web application in NetBeans IDE.
- Right-click the project and select New, then select RESTful Web Services from Patterns.
- Test the web service by right-clicking the project node and clicking Test RESTful Web Services.
- Deploy the web service by right-clicking the project and selecting Deploy.
To build, package, deploy, and run the web client, start by opening the webclient folder in NetBeans IDE. Then, right-click the webclient project and select Deploy. This will run the wsimport tasks, build and package the application into a WAR file, and deploy it to the server.
Take a look at this: Maven Aws Java Sdk S3
Java Web Services Basics
Java web services are built using the Jakarta EE API, specifically JAX-WS, which simplifies the development and deployment of web services clients and endpoints with annotations.
JAX-WS provides two ways to write application code: RPC Style and Document Style.
Related reading: External Cascading Style Sheet
These styles are used to create web services, especially for SOAP service users, which is a protocol employed for exchanging structured information within the implementation of web services.
SOAP web services are characterized by XML-based messaging, WSDL for service description, and protocol independence.
Here are the characteristics of SOAP web services:
- XML-based Messaging: SOAP messages are formatted using XML, providing a standardized structure for data exchange.
- WSDL for Service Description: A WSDL (Web Service Description Language) document describes SOAP web services, specifying their operations, data types, and endpoints.
- Protocol Independence: SOAP supports various transport protocols, including HTTP, SMTP, and more.
What Is a Web Service
A web service is a protocol used for exchanging structured information within the implementation of web services. It's a way for different systems to communicate with each other, and it's especially useful for enterprise-level applications that need a standardized and structured messaging format.
SOAP (Simple Object Access Protocol) is a type of web service that uses XML-based messaging to provide a standardized structure for data exchange. This is a big deal because it allows different systems to communicate with each other, even if they're not using the same programming language.
One of the key features of SOAP is its ability to support various transport protocols, including HTTP, SMTP, and more. This means that SOAP web services can be accessed and used by different systems, regardless of the underlying technology.
Intriguing read: Web Dev Services
There are two main ways to write JAX-WS application code: RPC Style and Document Style. These styles are used to develop and create web services, especially for SOAP service users.
Here are some key characteristics of SOAP web services:
- XML-based Messaging: SOAP messages are formatted using XML, providing a standardized structure for data exchange.
- WSDL for Service Description: A WSDL (Web Service Description Language) document describes SOAP web services, specifying their operations, data types, and endpoints.
- Protocol Independence: SOAP supports various transport protocols, including HTTP, SMTP, and more.
These characteristics make SOAP web services a popular choice for enterprise-level applications that require a standardized and structured messaging format.
Importance and Benefits
Java web services offer numerous benefits that make them an attractive choice for developers. They promote interoperability by utilizing standard communication protocols, enabling seamless communication among applications developed in various languages and operating on different platforms.
This means you can create a web service in Java and have it interact with applications built in other languages without any issues. Interoperability is a crucial aspect of modern software development, and Java web services make it a reality.
Web services also provide scalability, allowing applications to manage augmented workloads through the dynamic addition of resources. This is achieved through the use of Java web services, which empower scalable solutions.
Related reading: Building Web Application
In addition to scalability, web services promote code reusability by encapsulating business logic into reusable components that multiple applications can access remotely. This approach reduces code duplication and makes maintenance easier.
Here are some key benefits of using Java web services:
- Interoperability
- Scalability
- Reusability
- Platform Independence
- Loose Coupling
These benefits make Java web services an essential part of any Java development project. By utilizing web services, you can create applications that are flexible, maintainable, and scalable.
Inter-System Communication
Inter-System Communication is a fundamental aspect of Java Web Services. It enables seamless interaction between different systems, applications, and languages.
SOAP Web Services, for instance, use standardized protocols like XML to format messages, ensuring a structured and platform-independent data exchange.
By utilizing protocols like SOAP, developers can create web services that communicate effectively with non-Java systems, satisfying integration scenarios that demand interoperability.
Here are some key benefits of using web services for inter-system communication:
- Interoperability: Web services promote seamless communication among applications developed in various languages and operating on different platforms.
- Platform Independence: Java’s platform independence ensures developers can deploy web services developed in Java on any platform supporting the Java Virtual Machine (JVM), offering flexibility and portability.
- Loose Coupling: Web services support loose coupling between client and server applications, enabling them to evolve independently without impacting each other’s functionality.
Web services facilitate communication between diverse systems via the Internet or an Intranet by adhering to standardized protocols and formats. This ensures smooth integration and remote data exchange among disparate systems.
By leveraging web services, developers can create scalable solutions that manage augmented workloads through the dynamic addition of resources, empowering applications to grow and adapt to changing demands.
Java Web Services Implementation
Java Web Services Implementation is a crucial aspect of building web services in Java. JAX-WS (Java API for XML-Based Web Services) is a popular choice for developing web services, especially for SOAP service users.
JAX-WS simplifies the development and deployment of web services clients and endpoints using annotations. Two ways to write JAX-WS application code are RPC Style and Document Style.
To implement SOAP Web Services with JAX-WS, you need to define Service endpoint interfaces (SEI) which specify the methods to expose as web service, implement SEI with a Java class, annotate the SEI and its implementation class with JAX-WX annotations, and package web service classes to the WAR file and deploy it to a web server.
Here are the basic steps to implement RESTful Web Services with JAX-RS:
- Define the resources that represent the web services and its methods.
- Annotate the resource class and its methods with JAX-RS annotations.
- Package the web service classes to the WAR file and deploy it to a web server.
SOAP (Simple Object Access Protocol) is a protocol employed for exchanging structured information within the implementation of web services. Its characteristics include XML-based Messaging, WSDL for Service Description, and Protocol Independence.
Here are the steps to create a simple web service and clients with JAX-WS:
- Code the implementation class.
- Compile the implementation class.
- Package the files into a WAR file.
- Deploy the WAR file. The web service artifacts, which are used to communicate with clients, are generated by the GlassFish Server during deployment.
- Code the client class.
- Use a wsimport Ant task to generate and compile the web service artifacts needed to connect to the service.
- Compile the client class.
- Run the client.
Java Web Services Annotations
Java Web Services Annotations are a crucial part of developing web services in Java. They help simplify the development and deployment of web services clients and endpoints.
To turn a Java class into a SOAP web service, you'll need to decorate it with annotations, such as @XMLType and @XmlAccessorType, to specify how the XML engine should access the class's properties.
The JAX-WS API is used to develop and create web services, especially for SOAP service users. It provides a simpler way to write web services code by using annotations.
Here are the key request method designator annotations defined by JAX-RS:
- @GET
- @POST
- @PUT
- @DELETE
- @HEAD
The @Consumes annotation is used to specify which MIME media types a resource can accept from the client. If applied at the class level, all response methods accept the specified MIME types by default.
Add XML Annotations
Adding XML annotations to your Java classes is an essential step in creating web services. This is because the data encapsulated in your classes will be sent to SOAP web services clients in XML format.
The Score class is a great example of this. To decorate it with the necessary annotations, you'll need to add an @XMLType annotation, which indicates that the class will be used to represent XML data.
You'll also need to add an @XmlAccessorType annotation, which specifies how the XML engine should access the properties of the class. In this case, you'll want to use field-based access, so you'll set the value of the @XmlAccessorType annotation to XmlAccessType.FIELD.
This will allow the XML engine to look directly at the properties of the Score class, rather than relying on getter methods.
By adding these annotations, you'll be able to create a web service that can be easily consumed by SOAP clients.
Suggestion: Web Page Design Classes Online
Use the Annotation
Annotations are a key part of developing web services in Java, and they can make a big difference in how your services are exposed and consumed.
To turn a Java class into a SOAP web service, you need to decorate it with two specific annotations: one to indicate compliance with the EJB architecture and another to indicate that the public methods can be accessed through a SOAP-based service.
You can use the @WebMethod annotation to override the default method name used by the SOAP web services client. This is useful when you want to provide a different name for the method in the WSDL.
JAX-WS is the API used to develop and create web services, especially for SOAP service users. It simplifies the development and deployment of web services clients and endpoints by using annotations.
Request method designator annotations are used to map Java programming language methods to HTTP methods. JAX-RS defines a set of request method designators for common HTTP methods like GET, POST, PUT, DELETE, and HEAD.
You can use the @Produces annotation to specify the MIME media types or representations a resource can produce and send back to the client. This annotation can be applied at the class or method level.
The @Consumes annotation is used to specify which MIME media types of representations a resource can accept from the client. This annotation can also be applied at the class or method level.
Here are some examples of how to use the @Produces and @Consumes annotations:
For example, if you apply the @Produces annotation at the class level with the value "text/plain", all methods in the class will produce plain text by default. If you apply it at the method level, it will override the class-level setting.
Similarly, if you apply the @Consumes annotation at the class level with the value "text/plain", all methods will accept plain text by default. If you apply it at the method level, it will override the class-level setting.
You can also use the @Produces annotation to specify multiple MIME media types, and the JAX-RS runtime will choose the most acceptable media type based on the Accept header of the HTTP request.
By using these annotations effectively, you can make your web services more flexible and easier to consume.
Frequently Asked Questions
What are the two main types of web services?
There are two main types of web services: SOAP and REST, which enable communication between applications and devices over the web. Understanding the differences between these two types can help you choose the best approach for your web service needs.
Sources
- https://www.theserverside.com/video/Step-by-step-SOAP-web-services-example-in-Java-using-Eclipse
- https://www.theserverside.com/video/Step-by-step-RESTful-web-service-example-in-Java-using-Eclipse
- https://www.geeksforgeeks.org/java-web-services/
- https://docs.oracle.com/cd/E19798-01/821-1841/bnayk/index.html
- https://www.educba.com/how-to-create-webservice-in-java/
Featured Images: pexels.com