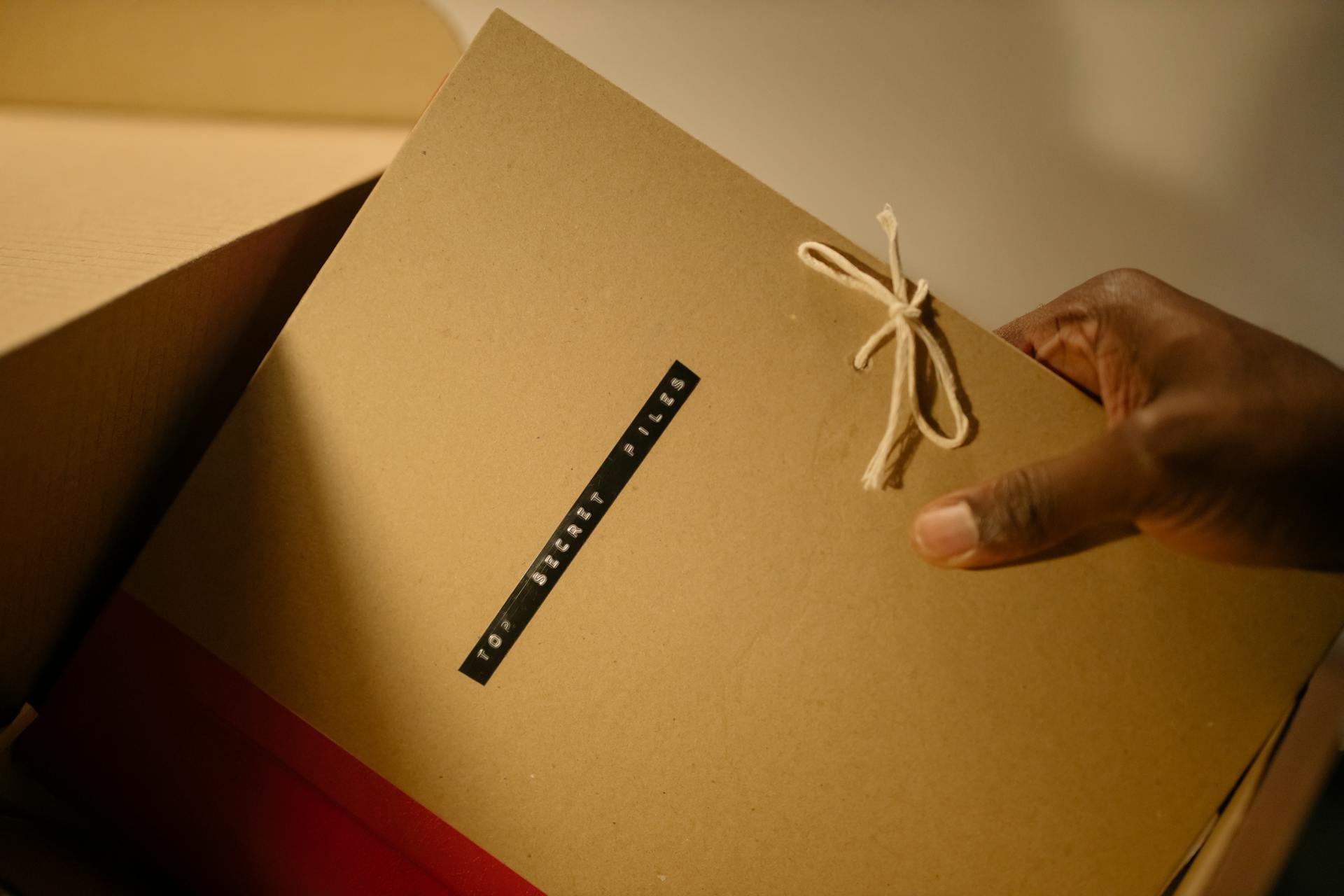
To start working with the Dropbox API in Java, you'll need to create a Dropbox developer account. This will give you access to the Dropbox API console, where you can create an app and obtain the necessary API keys.
First, head over to the Dropbox API console and create a new app. You'll need to provide some basic information about your app, such as its name and description.
Once you've created your app, you'll receive an App Key and App Secret, which you'll need to use in your Java code. These keys will be used to authenticate your app and access the Dropbox API.
With your App Key and App Secret in hand, you're ready to start writing your Java code. In the next section, we'll walk through the process of authenticating your app and making API calls using the Dropbox Java SDK.
assistant
As an assistant, I can tell you that the Dropbox Java API is designed to interact with Dropbox from Java applications.
This API provides a programmatic way to read and write to Dropbox, making it a valuable tool for developers.
The Java Dropbox API is one of the many APIs provided by Dropbox for different programming platforms.
Core Features
The Dropbox Core SDK for Java is a powerful tool that allows you to access Dropbox's HTTP-based Core API v2. This SDK also supports the older Core API v1, but that support will be removed at some point.
The library is well-documented, with Javadocs available to help you get started.
With the Dropbox Core SDK for Java, you can tap into the full potential of Dropbox's API, making it easy to integrate their services into your Java applications.
Core SDK
The Dropbox Core SDK for Java is a Java library that allows you to access Dropbox's HTTP-based Core API v2. This SDK also supports the older Core API v1, but that support will be removed at some point.
You can find the documentation for the Dropbox Core SDK for Java in the Javadocs.
If you're looking for a Java library to access Dropbox's Core API, the Dropbox Core SDK for Java is a great option.
Dropbox's Core SDK for Java is a reliable choice for integrating Dropbox functionality into your Java applications.
Version
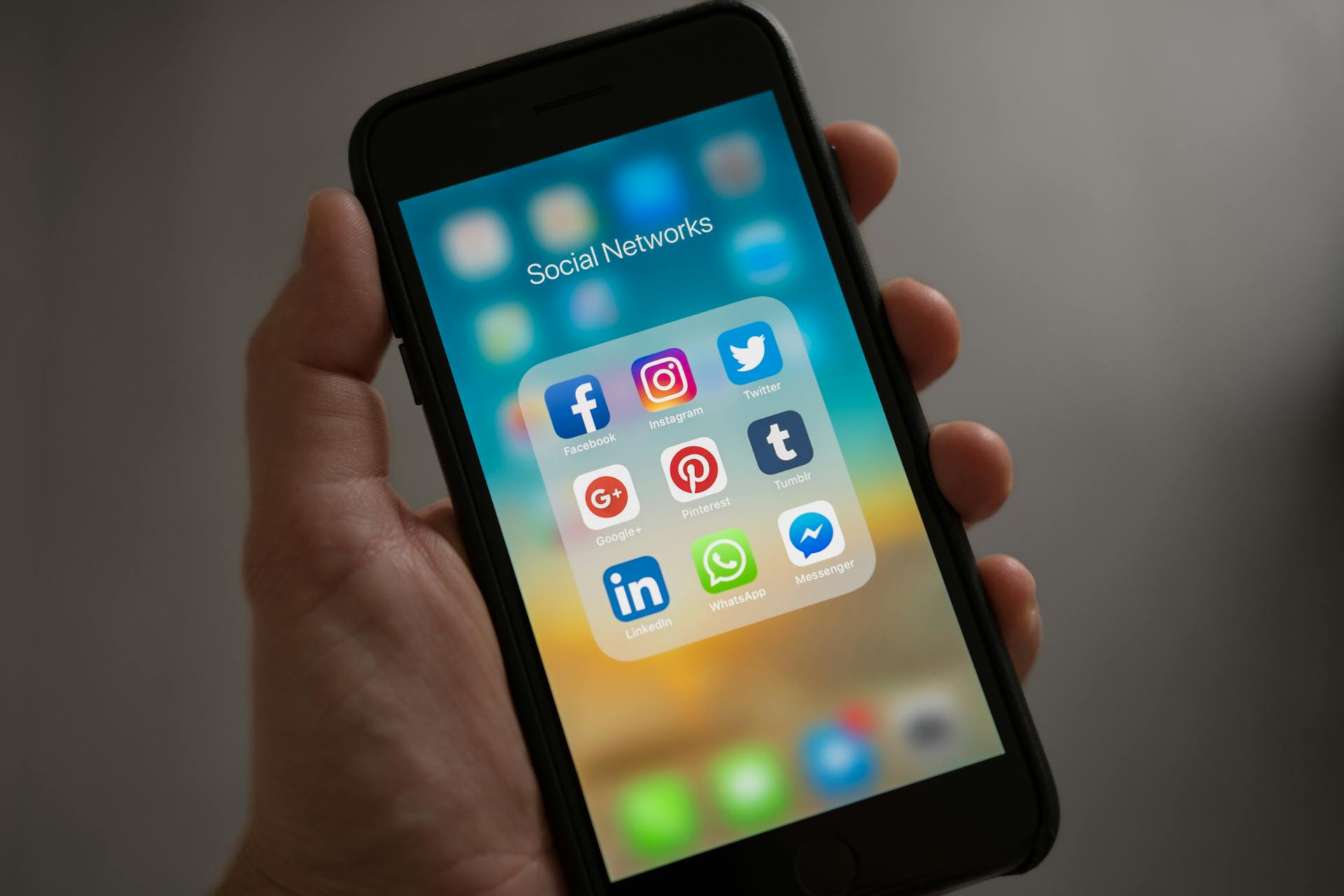
The Java SDK supports Java 8 and above, making it a great option for developers who have experience with this language.
To get started, you'll need to have the Java SDK installed on your computer. Make sure you follow the installation instructions carefully to avoid any issues.
Having the Java SDK installed is a crucial step before diving into the quick tutorial provided by Dropbox. This tutorial is a great resource for learning how to use the Java SDK effectively.
Authentication
To authenticate with the Dropbox API in Java, you need to go through the OAuth 2 authorization flow, which produces a file named "test.auth" with the access token.
This file can be passed in to the other examples, making it a crucial step in the process.
To use the Dropbox API on Android, you'll need to configure the SDK with the required settings, as specified in the documentation.
The required configuration includes several key pieces of information, but the exact details can be found in the Android-specific documentation.
You'll also need to create a Dropbox App and get the Dropbox App Secret, App Key, which are used to instantiate DbxAppInfo.
Once instantiated, you can authorize using the web request using DbxWebAuthNoRedirect, which will give you the authorize URL.
To use the Dropbox API, you'll need to register a new app in the App Console, selecting Dropbox API app and choosing your app's permission.
This will give you an app key that you'll need to use to access API v2.
To authorize with the Dropbox API, you'll need the Dropbox App secret and Dropbox App key, which can be obtained through the App Console.
These are used to generate an access token for your account, which you can then use to make calls to the API.
Android Integration
To integrate Dropbox API with your Android app, you need to add specific configurations to your AndroidManifest.xml file. This includes adding the AuthActivity entry and its associated intent-filter, using your Dropbox APP Key in place of "dropboxKey".
Your activity starting the authorization flow should be configured with android:launchMode="singleTask" to ensure proper authorization result passing. If that activity is already configured with android:taskAffinity, then the AuthActivity should also have the same task affinity.
There's a known issue with apps targeting SDK 33 and app-to-app authentication when the Dropbox App is installed. A fix is being worked on and will be released in an upcoming version of the Dropbox Mobile App.
Example and Testing
To get started with testing your Dropbox API Java application, you'll want to try out some API requests using the Dropbox object you've instantiated. You can do this by making a call to list the contents of a folder.
One way to test your application is to use the Dropbox Java API Example provided earlier, which shows how to upload a file to Dropbox and create a folder. This example can serve as a starting point for your own testing.
If you're experiencing issues with FileNotFoundException, you may need to adjust your file path or add the image to your project itself instead of the "src" folder. You can also use a JFileChooser to select the file you want to upload, as shown in the solution provided by rpatel.
V6.1.0
V6.1.0 brought some exciting changes to our example and testing workflow.
The DbxSdkVersion value is now generated in a gradle task, making it easier to manage and update our SDK version.
With v6.1.0, we've also added queries to the SDK manifest.
Here are some key features and bug fixes included in this release:
- Generates the DbxSdkVersion value in a gradle task (#508)
- Adds queries to SDK manifest (#509)
- Moves Android dependencies to the drop-sdk-java/android directory (#510)
- Mark StoneTask as cacheable and resolves some dbapp integration issues (#515)
V5.3.0
The latest version, v5.3.0, brings several improvements to the Dropbox API.
Update dropbox-api-spec to point to a more recent version (July 13, 2022) was included in this release.
The generateStone Gradle Task now supports Gradle Configuration Caching, making it easier to manage dependencies.
API Backwards Compatibility Fix - Won't crash when new types are returned from API.
Version Bumps in the Dropbox Android Sample Apps were also updated in this release.
Here are the key changes in v5.3.0:
- Update dropbox-api-spec to point to a more recent version (July 13, 2022)
- The generateStone Gradle Task now supports Gradle Configuration Caching
- API Backwards Compatibility Fix - Won't crash when new types are returned from API
- Version Bumps in the Dropbox Android Sample Apps
Comments on
Testing a Java API can be a bit tricky, but there are some common issues that can arise. One of them is the FileNotFoundException, which can be caused by a missing file.
In the Dropbox Java API tutorial, a user named aritsays encountered this issue and asked how to handle it. The problem was that the file "happy.png" was not found in the specified location. To fix this, you can either add the image to the project itself or use a JFileChooser to select the file.
Using a JFileChooser can make it easier to select the file and avoid the FileNotFoundException. For example, rpatelsuggested using the following code:
```java
public void uploadToDropbox() throws DbxException, IOException {
File inputFile;
JFileChooser filechoose=new JFileChooser();
filechoose.setFileSelectionMode(JFileChooser.FILES_ONLY);
filechoose.showOpenDialog(null);
inputFile=filechoose.getSelectedFile();
s=inputFile.getName();
//File inputFile = new File(fileName);
FileInputStream fis = new FileInputStream(inputFile);
try {
DbxEntry.File uploadedFile = dbxClient.uploadFile("/" + inputFile.getName(), DbxWriteMode.add(), inputFile.length(), fis);
String sharedUrl = dbxClient.createShareableUrl("/" + inputFile.getName());
System.out.println("Uploaded: " + uploadedFile.toString() + " URL " + sharedUrl);
} finally {
fis.close();
}
}
```
This code uses a JFileChooser to select the file and then uploads it to Dropbox. It's a good example of how to handle the FileNotFoundException and make the code more user-friendly.
Another issue that can arise when testing a Java API is the need to get authorization without prompting the user. Marks asked about this in the comments, and it's an important consideration for automated processes. However, there is no straightforward solution provided in the comments, and it may require additional research or experimentation to find a suitable solution.
In terms of testing the Dropbox Java API, it's essential to consider the different scenarios that can occur, such as the FileNotFoundException or the need for authorization. By understanding these potential issues, you can write more robust and reliable code that handles these situations effectively.
Frequently Asked Questions
How to integrate API in Java?
To integrate an API in Java, create a new Java project in Eclipse, then follow steps to develop, export, and test your API, and finally add it to your project's build path. This process involves setting up a Java project, coding and exporting the API, testing it, and incorporating it into your application.
Sources
- https://github.com/dropbox/dropbox-sdk-java/releases
- https://zappysys.com/api/integration-hub/dropbox-connector/java
- https://github.com/dropbox/dropbox-sdk-java
- https://javapapers.com/java/dropbox-java-api-tutorial/
- https://www.omi.me/blogs/api-guides/how-to-use-dropbox-api-to-upload-and-download-files-in-java
Featured Images: pexels.com