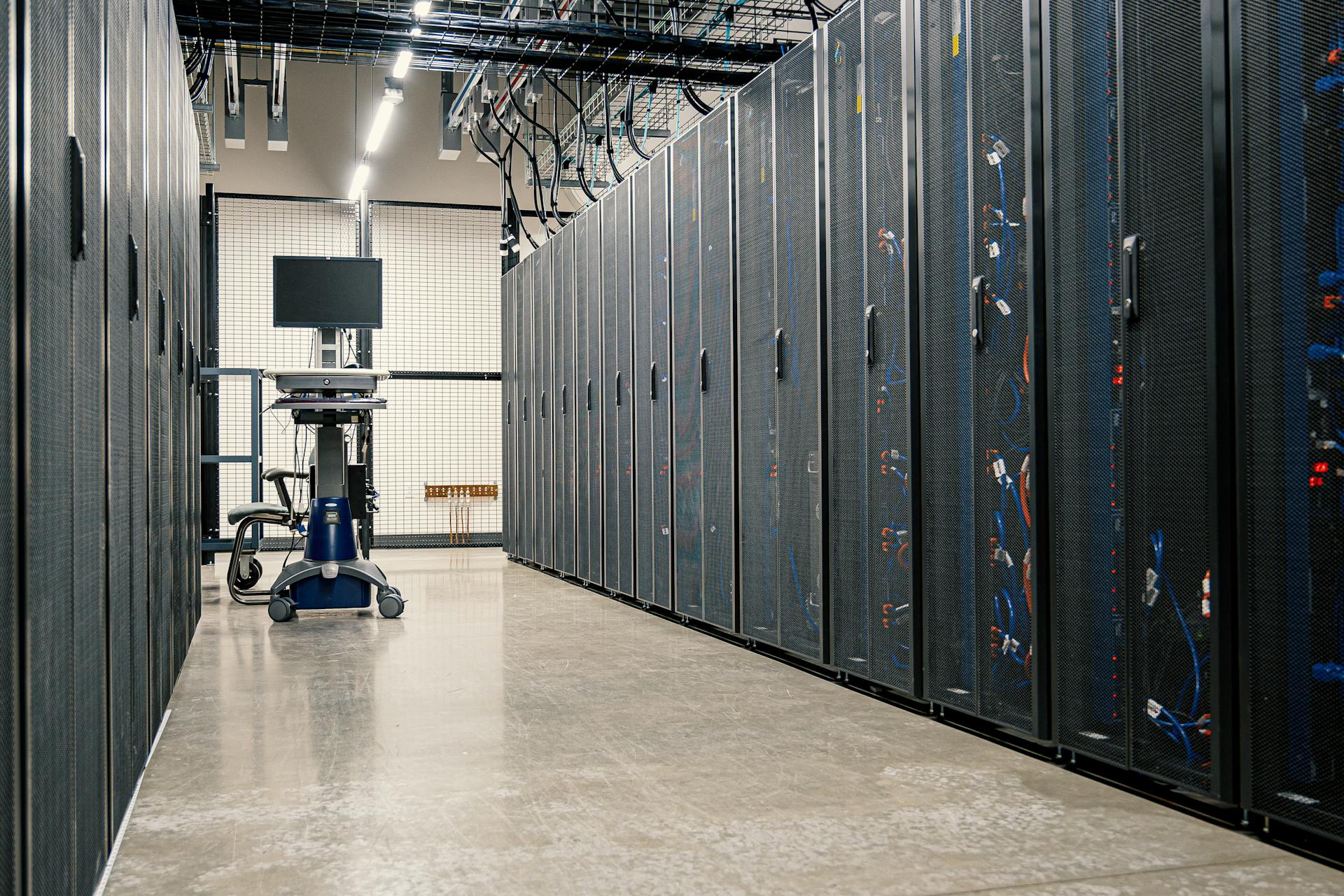
Google Cloud Functions is a serverless compute service that allows you to run small code snippets, called functions, in response to events. You can write functions in various languages, including Python.
To get started with Google Cloud Functions in Python, you'll need to install the Cloud Functions SDK using pip. This will provide you with the necessary tools to create and deploy your functions.
Google Cloud Functions supports Cloud Storage triggers, which allow you to run functions in response to changes in your Cloud Storage buckets. This is particularly useful for tasks like image processing or data ingestion.
Getting Started
To get started with Google Cloud Functions in Python, you'll want to run the Quickstart command.
You can run the following command to get started: `functions-framework-python` or `functions-framework` if you have multiple language frameworks installed.
Curious to learn more? Check out: Google Cloud Functions Framework
Installation
To get started with OpenTelemetry on Google Cloud Functions, you'll first need to install the Functions Framework. This can be done via pip, which is a package manager for Python.
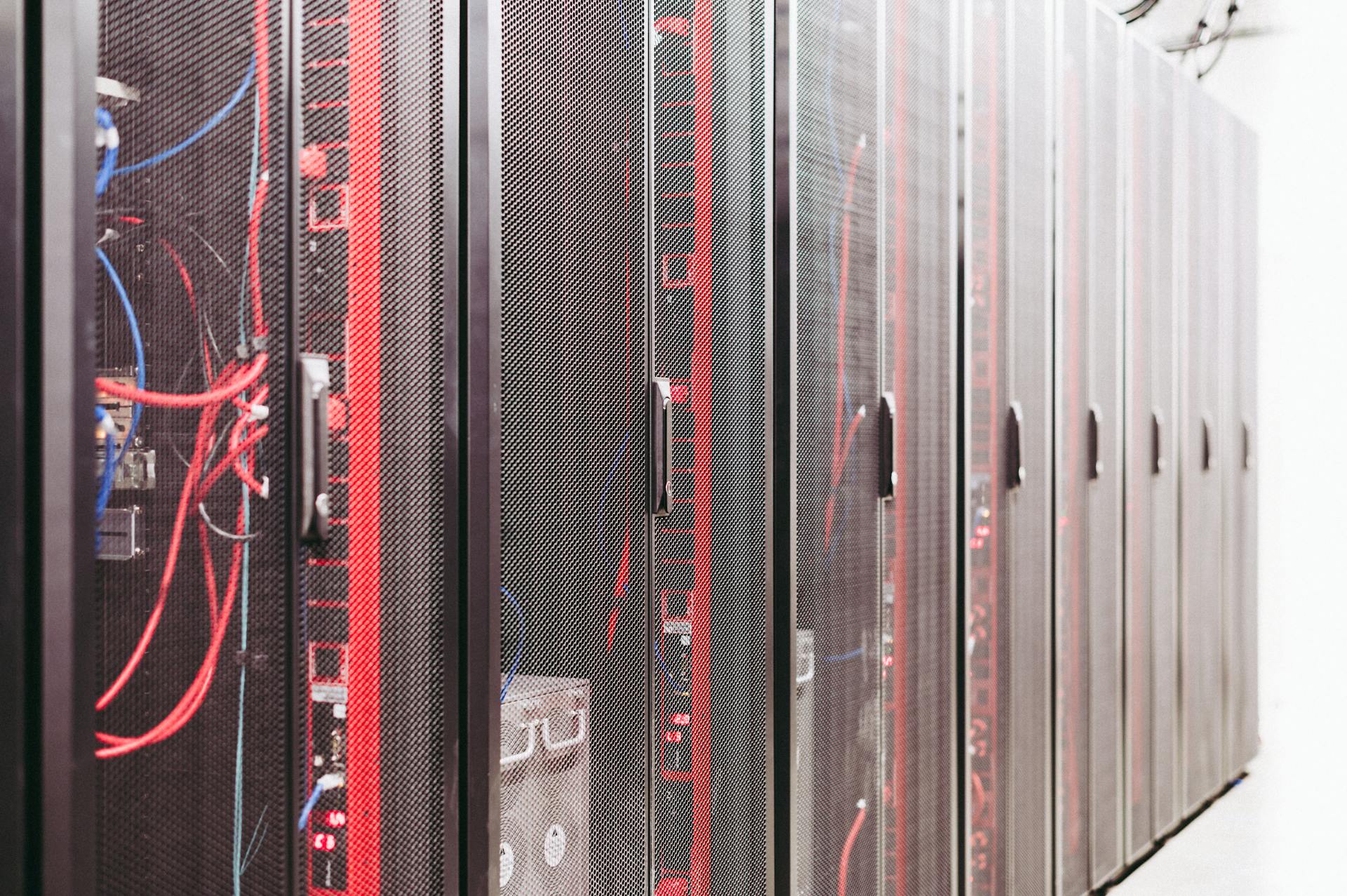
For deployment, add the Functions Framework to your requirements.txt file. This is a text file that lists the dependencies required by your project.
You can also send requests to your function using curl from another terminal window. This is useful for testing and debugging your function.
To set up OpenTelemetry Python integration on Google Cloud Functions, add the following line to the requirements.txt file of your function:
This adds the latest version of the dynatrace-opentelemetry-gcf package as a dependency to your function.
Before you start, make sure you've followed the initial configuration steps described in the documentation for setting up OpenTelemetry monitoring for Google Cloud Functions.
Here are the prerequisites you'll need to meet:
- dynatrace-opentelemetry-gcf version 1.247+
- Cloud Functions product version: 1st gen, 2nd gen
Quickstart: Hello World
Getting started with a new project can be both exciting and intimidating. Run the following command to get started.
You can use the functions-framework-python command if you have multiple language frameworks installed.
Google Cloud Functions
Google Cloud Functions is a powerful tool for building portable Python functions. It allows you to write lightweight functions that can run in various environments, including Google Cloud Functions, your local development machine, Cloud Run and Cloud Run for Anthos, and Knative-based environments.
You can write functions that can be invoked in response to a request and automatically unmarshal events conforming to the CloudEvents spec. This makes it easy to build scalable and flexible applications.
The Functions Framework for Python is an open-source FaaS (Function as a Service) framework that lets you write portable Python functions. It's brought to you by the Google Cloud Functions team and supports multiple platforms.
Here are some of the key features of Google Cloud Functions:
- Spin up a local development server for quick testing
- Invoke a function in response to a request
- Automatically unmarshal events conforming to the CloudEvents spec
- Portable between serverless platforms
When building a Cloud function artifact, you need to specify the target runtime. This can be done in three ways: by providing an explicit value for the complete_platforms field, an explicit value for the runtime field, or by inferring it from the relevant interpreter constraints.
Here's a breakdown of the three options:
- An explicit value for the complete_platforms field
- An explicit value for the runtime field
- Inferred from the relevant interpreter constraints
For example, you can specify the runtime explicitly using the python_google_cloud_function target, like this:
```python
python_google_cloud_function(
name="cloud_function",
handler="google_cloud_function_example.py:example_handler",
type="event",
# Explicit runtime, `complete_platforms` taken from Pants' built-in defaults:
runtime="python312",
)
```
This tells Pants to use the python312 runtime and pick an appropriate "complete platforms" value from its built-in defaults.
Building and Deploying
Building and Deploying Google Cloud Functions with Python is a straightforward process. You can use the Functions Framework for Python to write lightweight functions that run in many different environments, including Google Cloud Functions, your local development machine, Cloud Run and Cloud Run for Anthos, and Knative-based environments.
To build a deployable container, you'll need to install Docker and the pack tool, then build a container from your function using the Functions buildpacks. This can be done with a single command: `pack build --builder gcr.io/buildpacks/builder:v1 --env GOOGLE_FUNCTION_SIGNATURE_TYPE=http --env GOOGLE_FUNCTION_TARGET=hello my-first-function`.
You can then start the built container with `docker run --rm -p 8080:8080 my-first-function`, and send requests to the function using `curl localhost:8080`. This will output "Hello World!".
To deploy your function to Google Cloud, you'll need to create a zip file or directory, and specify the `--entry-point` as `handler`. You can use any of the various Google Cloud methods to upload your zip file or directory, such as the Google Cloud console or the Google Cloud CLI.
Here's a step-by-step guide to building and deploying a Google Cloud Function with Python:
By following these steps, you can deploy your Python function to Google Cloud Functions and start using it to process requests.
Build a Deployable Container
Building a deployable container is a crucial step in deploying your function to Google Cloud. You'll need to install Docker and the pack tool to get started.
To build a container from your function, you can use the Functions buildpacks. This involves running the command `pack build --builder gcr.io/buildpacks/builder:v1 --env GOOGLE_FUNCTION_SIGNATURE_TYPE=http --env GOOGLE_FUNCTION_TARGET=hello my-first-function`. This will create a container that can be deployed to Google Cloud.
Here are the steps to follow:
- Install Docker and the pack tool.
- Build a container from your function using the Functions buildpacks.
- Start the built container with the command `docker run --rm -p 8080:8080 my-first-function`.
- Send requests to this function using curl from another terminal window with the command `curl localhost:8080`.
The output will be the result of your function, in this case, "Hello World!".
Migrating from Pants 2.16 and Earlier
Migrating from Pants 2.16 and earlier is a bit of a process, but don't worry, I've got you covered.
If you're still using Pants 2.16 or earlier, you're likely using the Lambdex project to package your Google Cloud Functions. This involves converting your code into a Pex file and then using Lambdex to adapt it for GCF.
Pants 2.17 deprecated the use of Lambdex in favor of choosing dependencies ahead of time, which resulted in a zip file laid out in the format recommended by GCF.
A fresh viewpoint: How to Upload File to Google Cloud Storage Using Reactjs
In Pants 2.18, the new behavior became the default, and in Pants 2.19, the old Lambdex behavior was entirely removed.
If your code can be packaged without warnings using Pants 2.18, you can skip ahead to Pants 2.19, but only after removing the [lambdex] section in pants.toml if it still remains.
However, if your code still generates warnings, you'll need to upgrade to Pants 2.18 first, and then to Pants 2.19.
Here's a quick rundown of the changes:
- Pants 2.16 and earlier: Lambdex project used
- Pants 2.17: Lambdex deprecated, new behavior introduced
- Pants 2.18: New behavior becomes default
- Pants 2.19 and later: Old Lambdex behavior removed
If you encounter a bug with the new behavior, please let the developers know. And if you need advanced PEX features, you can switch to using pex_binary directly.
Using PEX Directly
Using PEX Directly can be a convenient way to access advanced features like dynamic selection of dependencies, but it comes with a trade-off of larger packages and slower cold starts.
A PEX file created by pex_binary is a carefully constructed zip file that can be understood natively by Google Cloud Functions.
For your interest: Google Cloud Bucket How to Store Index File
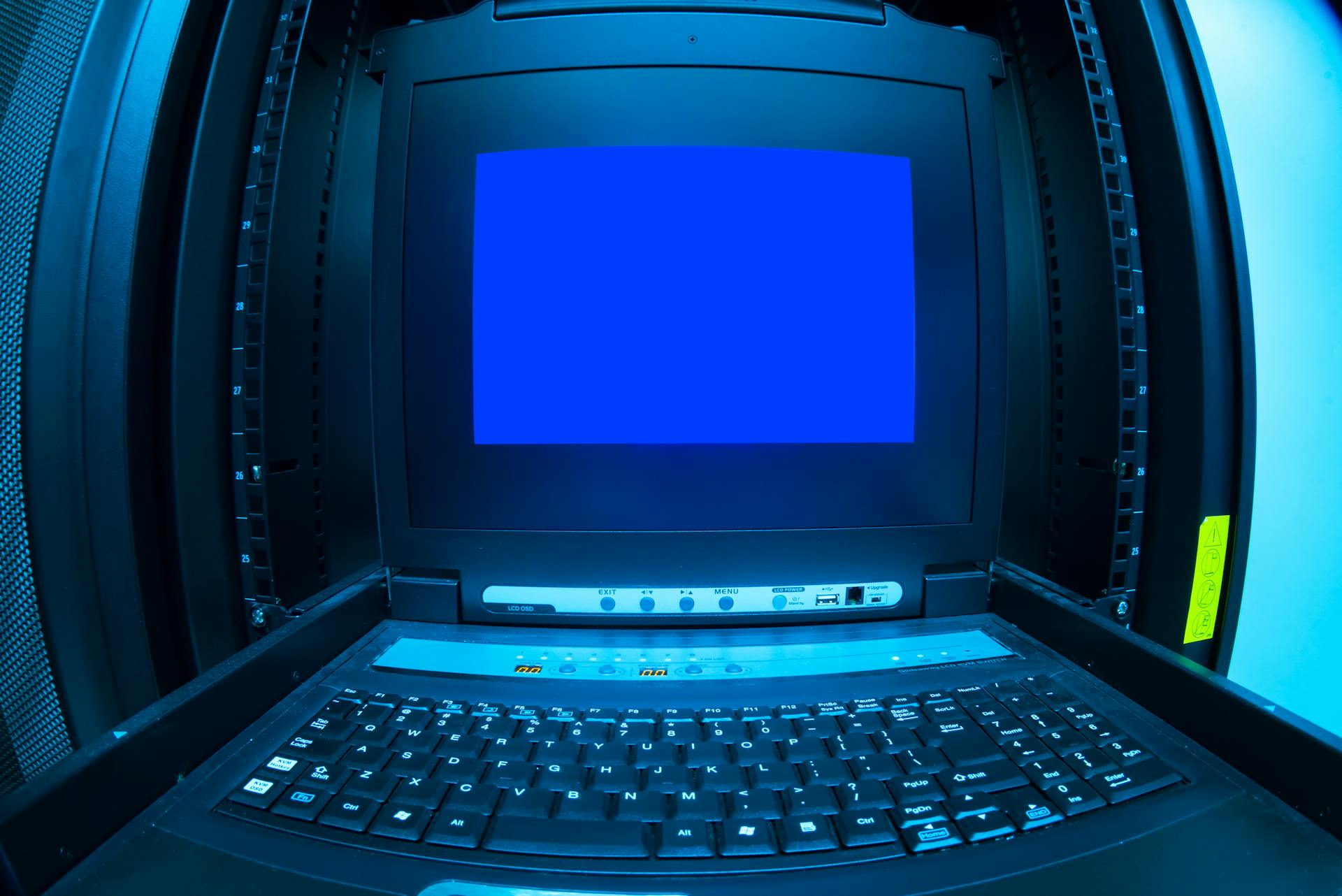
To use a pex_binary, you'll need to configure the Google Cloud Function handler as the __pex__ pseudo-package followed by the handler's normal module path.
For example, if your handler is in some/module/path.py within a source root, you'd use __pex__.some.module.path.
This may require setting the GOOGLE_FUNCTION_SOURCE environment variable to __pex__.gcf_example, assuming your project is a source root.
You can then package your project using pants package project:gcf, which will generate a project/gcf.pex file.
Upload this file to Google Cloud Functions, specifying the handler as example_handler.
Recommended read: How to Use Google Drive Cloud Sync
Frequently Asked Questions
Which language is supported by Google Cloud functions?
Google Cloud Functions supports multiple programming languages, including Python, Java, and Ruby, among others
Sources
- https://github.com/GoogleCloudPlatform/functions-framework-python
- https://codelabs.developers.google.com/codelabs/cloud-functions-python-http
- https://stackoverflow.com/questions/48772583/python-in-google-cloud-functions
- https://docs.dynatrace.com/docs/setup-and-configuration/google-cloud-platform/gcp-integrations/gcp-functions/opentelemetry-on-gcf-python
- https://www.pantsbuild.org/dev/docs/python/integrations/google-cloud-functions
Featured Images: pexels.com