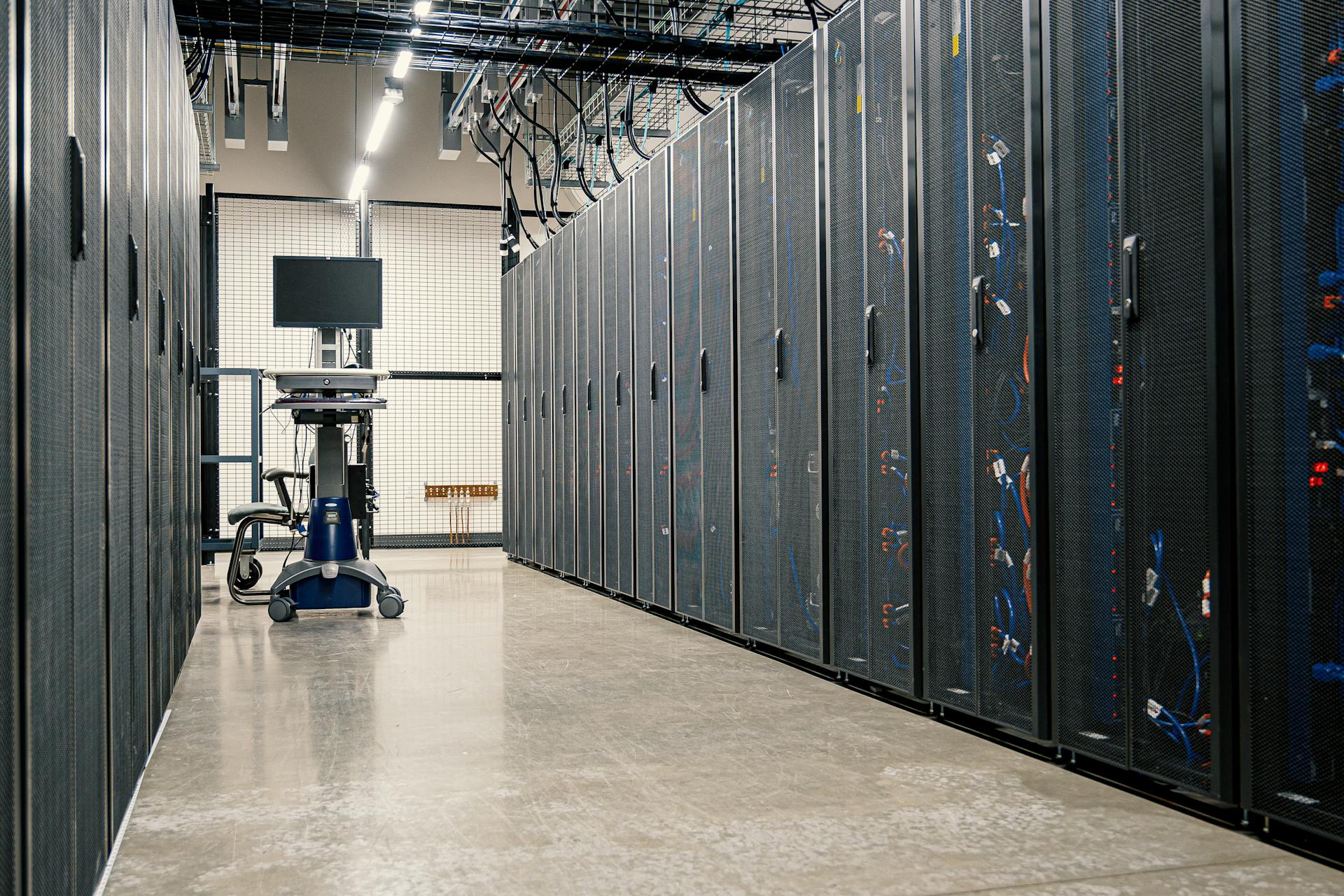
Google Cloud Functions is a serverless compute service that allows you to run small code snippets in response to events. It's a great way to build scalable applications without worrying about the underlying infrastructure.
Cloud Functions supports a variety of programming languages, including Node.js, Python, and Go. It also provides a range of triggers, such as HTTP requests, Cloud Storage changes, and Pub/Sub messages.
With Cloud Functions, you can write code that runs in response to events, making it ideal for building real-time applications. For example, you can use Cloud Functions to update a database in real-time when a user submits a form.
Here's an interesting read: What Are Some Important Functions of Google Classroom
Getting Started
To get started with the Google Cloud Functions Framework, you'll first need to create an index.js file with the following contents: exports.helloWorld = (req, res) => { res.send('Hello, World'); };.
Create a new project by running npm init to create a package.json file, then install the Functions Framework with npm install @google-cloud/functions-framework.
A unique perspective: How to Upload File to Google Cloud Storage Using Reactjs
To set up a new project, you can follow these steps:
- Create a package.json file using npm init: npm init
- Create an index.js file with the following contents: const functions = require('@google-cloud/functions-framework'); functions.http('helloWorld', (req, res) => { res.send('Hello, World'); });
- Now install the Functions Framework: npm install @google-cloud/functions-framework
- Add a start script to package.json, with configuration passed via command-line arguments: "scripts": {"start": "functions-framework --target=helloWorld"}
- Use npm start to start the built-in local development server: npm start
To run a quickstart, you can use the following command: npx @google-cloud/functions-framework --target=helloWorld, and open http://localhost:8080/ in your browser to see the result.
To serve a function, you can use the following command: npm start, and then send requests to the function using curl from another terminal window: curl localhost:8080.
You might enjoy: How to Use Google Drive Cloud Sync
Configuring the Environment
To configure the Functions Framework, you can specify the type and name of the function you want to run using either command-line interface (CLI) flags or environment variables. If you specify both, the environment variable will be ignored.
You can configure the Functions Framework using command-line flags or environment variables. The following table summarizes the available options:
You can also configure the Functions Framework using environment variables, such as HOST, FUNCTION_TARGET, and FUNCTION_SIGNATURE_TYPE.
Set Up Node.js and Firebase CLI
To set up Node.js and the Firebase CLI, you'll need to install Node.js and npm first. Node Version Manager is recommended for installing Node.js and npm.
You can install the Firebase CLI via npm by running the command: `npm install -g firebase-tools`. If the command fails, you may need to change npm permissions.
To update to the latest version of firebase-tools, simply rerun the same command.
If this caught your attention, see: Firebase and Google Cloud Platform
Configure the
Configuring the environment is a crucial step in setting up your project. You can specify the type and name of the function you want to run using either command-line interface (CLI) flags or environment variables.
You can configure the Functions Framework using environment variables. These variables include PORT, FUNCTION_TARGET, FUNCTION_SIGNATURE_TYPE, FUNCTION_SOURCE, and LOG_EXECUTION_ID. For example, you can set the port using the PORT variable.
The Functions Framework can also be configured using command-line flags. If you specify both flags and environment variables, the environment variable will be ignored. Some common command-line flags include --port, --target, --signature-type, --source, and --log-execution-id.
You can set command-line flags in your package.json via the start script. For example, you can specify the host and port using the --host and --port flags.
Here's a summary of common command-line flags and their corresponding environment variables:
You can also configure the Functions Framework using the Firebase CLI. When initializing your project, you can choose either TypeScript or JavaScript for composing functions. You'll also need to initialize Cloud Firestore, which can be done by running firebase init firestore.
Recommended read: Google Cloud Functions Python
Knative Container Environments
Cloud Run and Cloud Run for Anthos both implement the Knative Serving API, making it easy to build and deploy containers to a Knative environment.
You can also use Cloud Run on GKE, which implements the Knative Serving API as well.
The Functions Framework is designed to be compatible with Knative environments, allowing you to build and deploy containers with ease.
Here are the steps to deploy a container to a Knative environment:
- Build a container from your function using the Functions buildpacks: pack build --builder gcr.io/buildpacks/builder:v1 --env GOOGLE_FUNCTION_SIGNATURE_TYPE=http --env GOOGLE_FUNCTION_TARGET=hello my-first-function
- Start the built container: docker run --rm -p 8080:8080 my-first-function
- Send requests to this function using curl from another terminal window: curl localhost:8080
If you want even more control over the environment, you can deploy your container image to Cloud Run on GKE, which gives you additional control over the environment.
On a similar theme: Azure Test Environment
Project Setup
To get started with the Google Cloud Functions Framework, you'll need to set up your project. You can do this by initializing your Firebase project using the Firebase CLI. Run `firebase login` to log in via the browser and authenticate the Firebase CLI.
You'll then need to initialize Cloud Firestore and the Functions Framework. To do this, run `firebase init firestore` and accept the default values when prompted for Firestore rules and index files. Next, run `firebase init functions` and choose JavaScript as your language support.
A unique perspective: Google Cloud Functions vs Aws Lambda
The package.json file created during initialization contains an important key: "engines": {"node": "16"}. This specifies your Node.js version for writing and deploying functions. You can select other supported versions.
Here are the steps to initialize your project in a concise list:
- Run `firebase login` to log in via the browser and authenticate the Firebase CLI.
- Run `firebase init firestore` and accept the default values when prompted.
- Run `firebase init functions` and choose JavaScript as your language support.
- Review the "engines" key in your package.json file to ensure your Node.js version is set correctly.
Quickstart: Set Up a Project
To set up a project, create a package.json file using npm init. This file contains important keys, such as "engines": {"node": "16"}, which specifies your Node.js version for writing and deploying functions.
You can choose other supported versions, but for now, let's stick with Node.js 16. Next, create an index.js file with the following contents: const functions = require('@google-cloud/functions-framework'); functions.http('helloWorld', (req, res) => { res.send('Hello, World'); }); This sets up a basic HTTP function that responds with "Hello, World".
To install the Functions Framework, run npm install @google-cloud/functions-framework. This will allow you to use the functions-framework module in your project.
Once installed, add a start script to package.json with configuration passed via command-line arguments. You can do this by adding the following line to your package.json file: "scripts": {"start": "functions-framework --target=helloWorld"}.
To start the built-in local development server, run npm start. This will serve your function at http://localhost:8080/. You can test your function by sending requests to this URL using curl from another terminal window: curl localhost:8080. The output should be "Hello, World".
Check this out: Google Cloud Platform Project Id
Per-Language Instructions
When working on a project, you'll need to set up your environment to accommodate different programming languages. This is where per-language instructions come in.
The Node.js Functions Framework allows you to specify your function's name and signature type as command line arguments or environment variables. You can also specify these values in the package.json buildfile.
The Python Functions Framework requires you to specify your function's name and signature type as command line arguments when you run the framework. This is a straightforward process that ensures your function runs smoothly.
The Java Functions Framework has a more complex setup process, with configuration data accepted from three different sources in the following priority order: command-line arguments, buildfiles, and environment variables.
Here's a table summarizing the CLI arguments and environment variables for each language:
By following these per-language instructions, you'll be able to set up your project and get started with your function.
Use Storage
To set up your project for storage needs, start by using Google Cloud Storage. The google/cloud-storage package with composer registers the gs:// stream wrapper, allowing your function to read and write to Google Cloud Storage like any filesystem.
You can write to Google Cloud Storage as if it were a local filesystem, thanks to the stream wrapper. This makes it easy to store and retrieve data.
To unregister the gs:// stream wrapper, use stream_wrapper_unregister at any time.
Enable Events
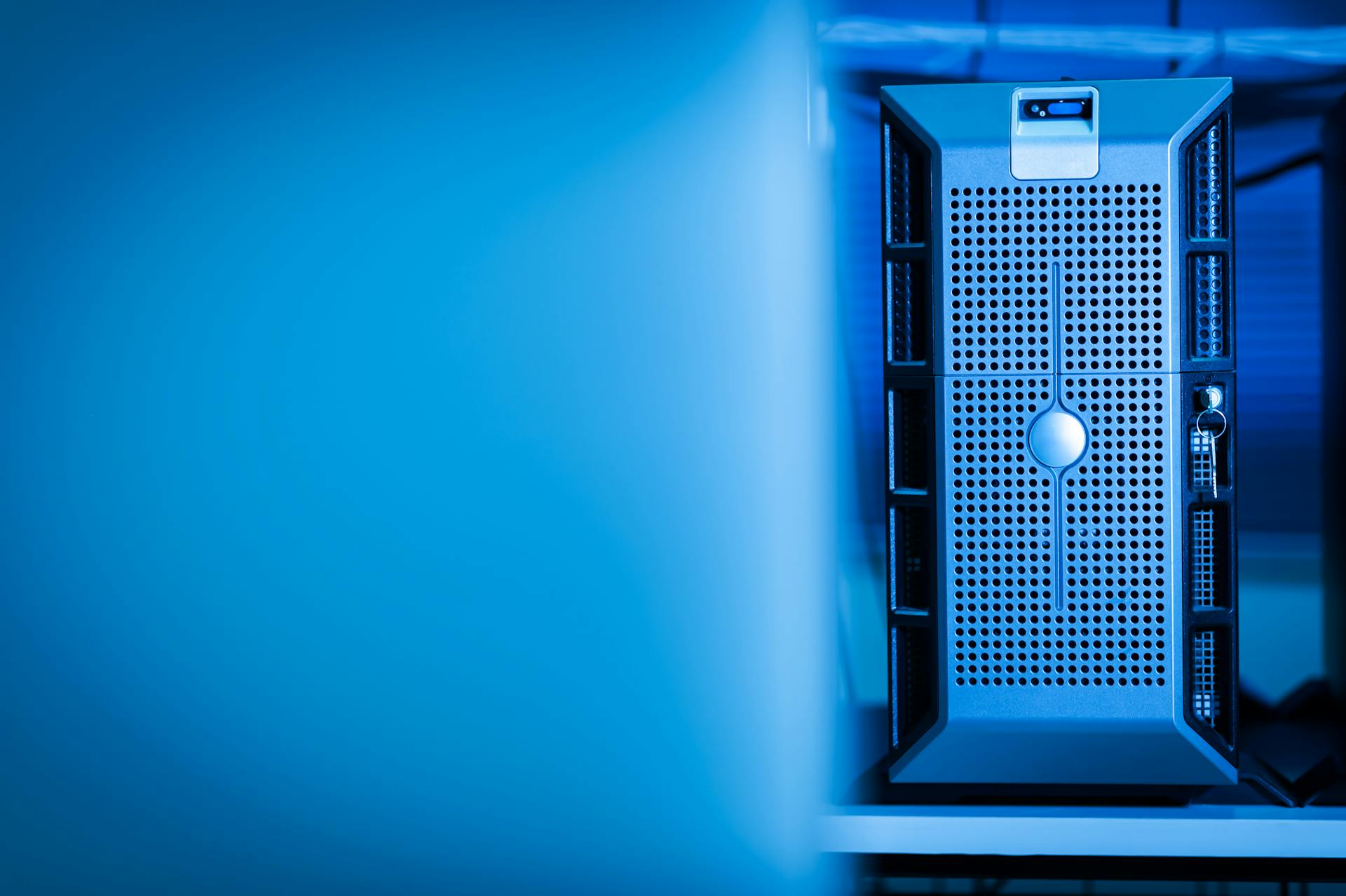
To enable events in your project, you'll need to set the FUNCTION_SIGNATURE_TYPE environment variable to 'event'. This will allow the Functions Framework to unmarshall incoming Google Cloud Functions event payloads to event and context objects.
Automatic unmarshalling is disabled by default, so you'll need to use the --signature-type command-line flag or the FUNCTION_SIGNATURE_TYPE environment variable to enable it.
The Functions Framework can unmarshall incoming CloudEvents payloads to a cloudevent object, which will be passed as an argument to your function when it receives a request.
You can make a cURL request in Cloud Event format to your function to test this feature.
Explore further: Azure Development Environment
Frequently Asked Questions
What is the function framework?
The Functions Framework is a tool for writing lightweight functions that can run in various environments, including the cloud and local machines. It enables developers to create portable and versatile functions with ease.
Sources
- https://cloud.google.com/functions/docs/running/function-frameworks
- https://packagist.org/packages/google/cloud-functions-framework
- https://firebase.google.com/docs/functions/get-started
- https://www.npmjs.com/package/@google-cloud/functions-framework
- https://github.com/GoogleCloudPlatform/functions-framework-python
Featured Images: pexels.com