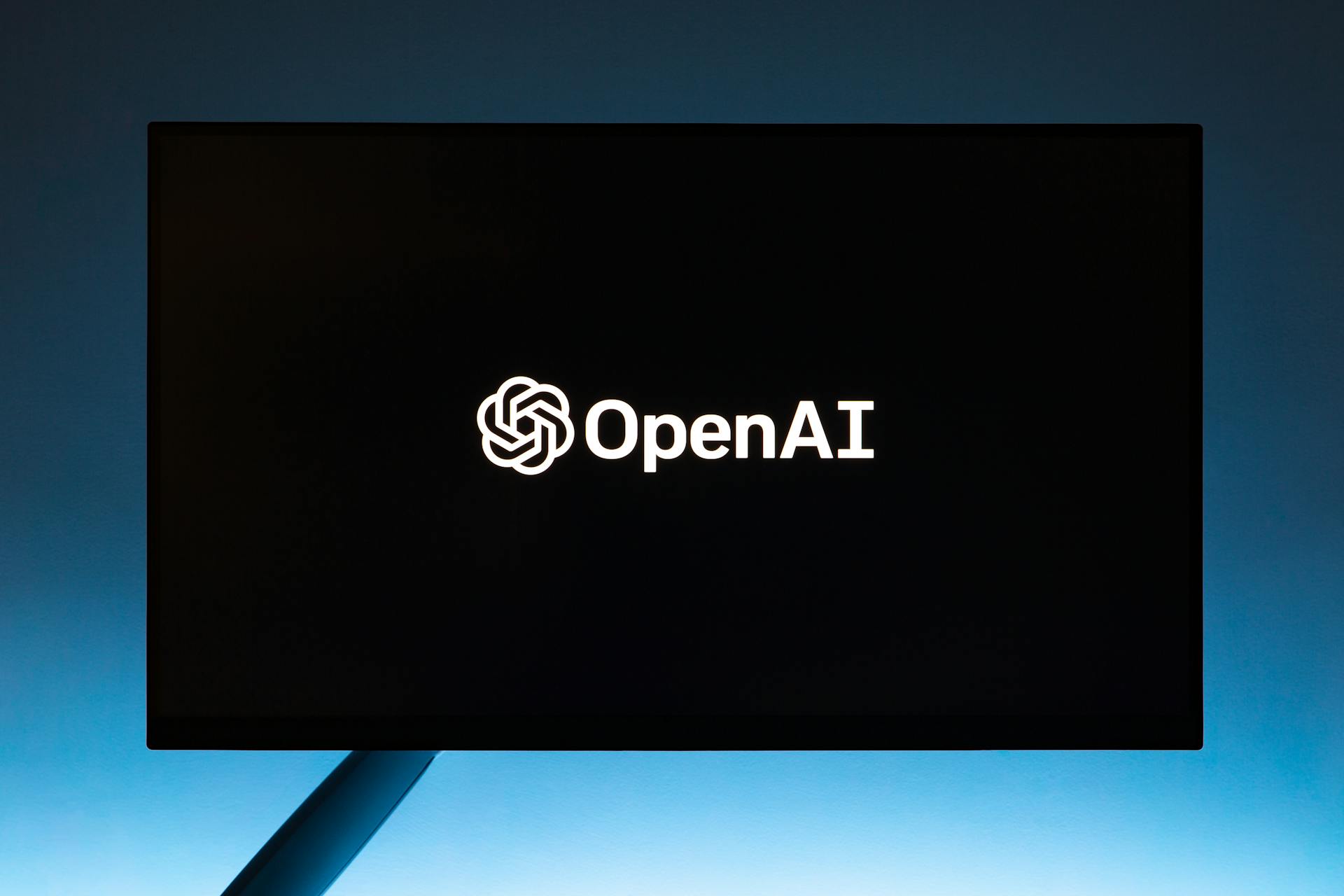
The Azure Openai API is a powerful tool that allows developers to tap into the capabilities of Openai's AI technology.
With the Azure Openai API, you can generate human-like text, translate languages, and even create images.
This API is particularly useful for applications that require natural language processing, such as chatbots and virtual assistants.
By leveraging the Azure Openai API, developers can build more sophisticated and user-friendly interfaces.
Prerequisites
To use an Azure OpenAI resource, you must have an Azure subscription and Azure OpenAI access. This will allow you to create an Azure OpenAI resource.
Having an Azure subscription and Azure OpenAI access will give you the necessary credentials to get started. For more information, see the Quickstart guide to get started generating text using Azure OpenAI Service.
You'll need both a connection URL and API keys to use an Azure OpenAI resource. These can be obtained by following the steps outlined in the Quickstart guide.
A fresh viewpoint: How to Get Access to Azure Openai Service
Authentication and Setup
To get started with Azure OpenAI, you'll need to authenticate your client. This involves creating an instance of the OpenAIClient class and providing a valid endpoint URI to an Azure OpenAI resource, along with a corresponding key credential, token credential, or Azure identity credential.
You can authenticate your client using a subscription key, which is used in most examples in this guide. To do this, you'll need to install the Azure.Identity package and use the DefaultAzureCredential provider.
Alternatively, you can authenticate with Azure Active Directory using the Azure Identity library. This involves installing the Azure.Identity package and using the DefaultAzureCredential provider.
If you choose to use Azure Active Directory, you can create an OpenAIClient instance with an Azure Active Directory credential. This will allow you to use the DefaultAzureCredential provider.
To obtain your API key, navigate to your Azure OpenAI resource in the Azure portal and copy one of the keys from the 'Keys and Endpoint' section.
Discover more: Azure Openai Api Key
Here's a summary of the authentication options:
Once you've obtained your API key, you'll be able to use it to authenticate your client and start interacting with Azure OpenAI.
Key Concepts and Best Practices
Completions are the main concept to understand in the Azure OpenAI API, providing a text prompt functionality that matches context and patterns to generate output text.
To get started, you can refer to the code snippet in the documentation, which gives a rough overview of how completions work.
Always check the response status code before processing the data to ensure smooth interaction with the API. This is a crucial best practice that helps handle responses and errors effectively.
Here are some key best practices to keep in mind:
- Always check the response status code before processing the data.
- Log errors for further analysis and debugging.
- Implement retries with exponential backoff for transient errors.
- Keep your API key secure and do not expose it in client-side code.
Key Concepts
Completions provide their functionality in the form of a text prompt, which by using a specific model, will then attempt to match the context and patterns, providing an output text.
Additional reading: Azure Text to Speech Api
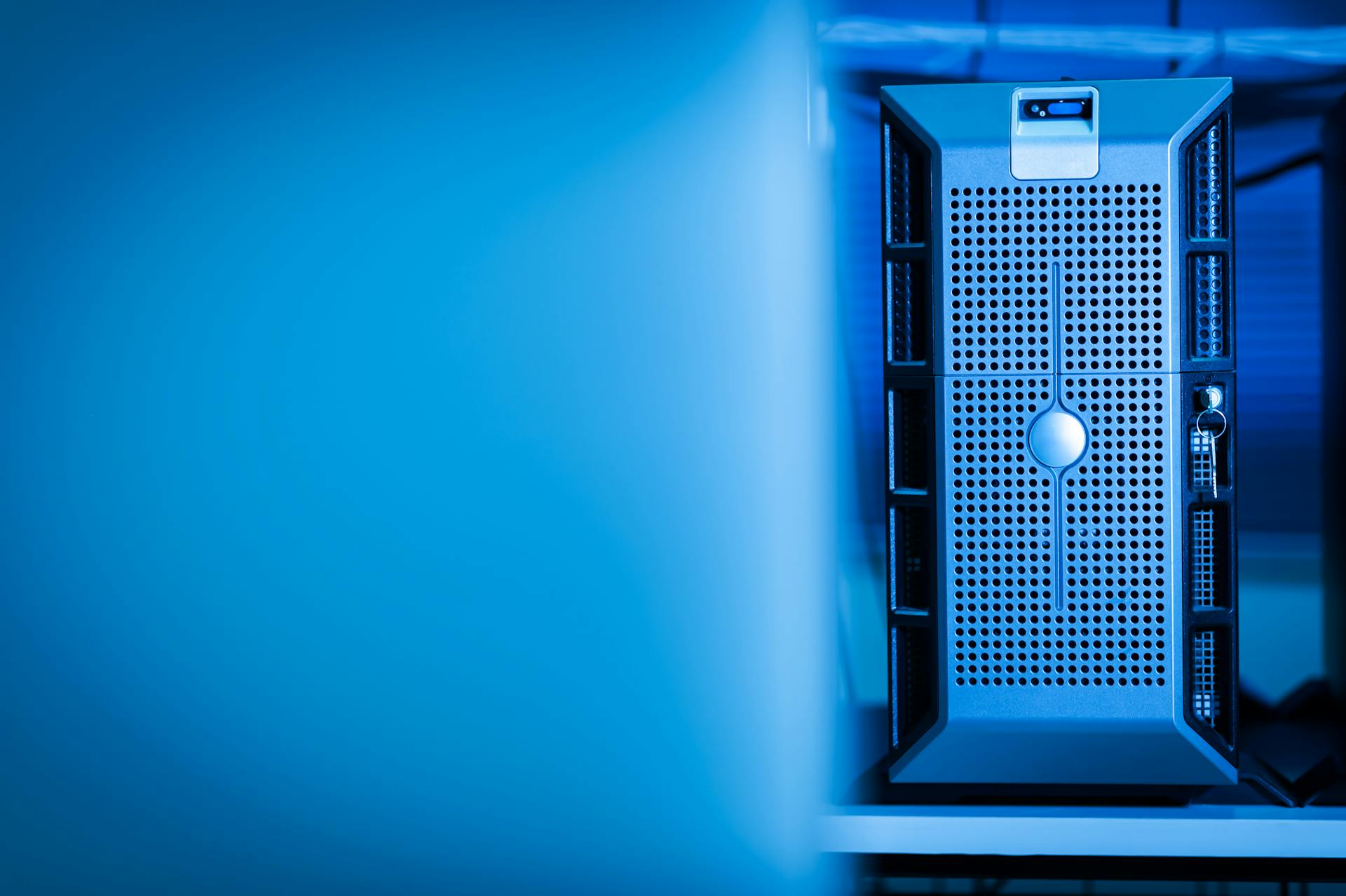
The main concept to understand is Completions, which can be explained by a code snippet that provides a rough overview of how it works.
Completions use a specific model to match the context and patterns of the input text, generating an output text as a result.
This model is used to generate text responses to input prompts, as shown in the GenerateMultipleChatbotResponsesWithSubscriptionKey method.
The GenerateMultipleChatbotResponsesWithSubscriptionKey method gives an example of generating text responses to input prompts using an Azure subscription key.
This method is an example of how Completions can be used to generate multiple chatbot responses, making it a useful tool for developers.
Broaden your view: Azure Openai Completions Playground for Gpt-4o
Best Practices
To interact smoothly with the OpenAI API, it's essential to follow best practices. Always check the response status code before processing the data.
This may seem obvious, but it's crucial to log errors for further analysis and debugging. This will help you identify and fix issues more efficiently.
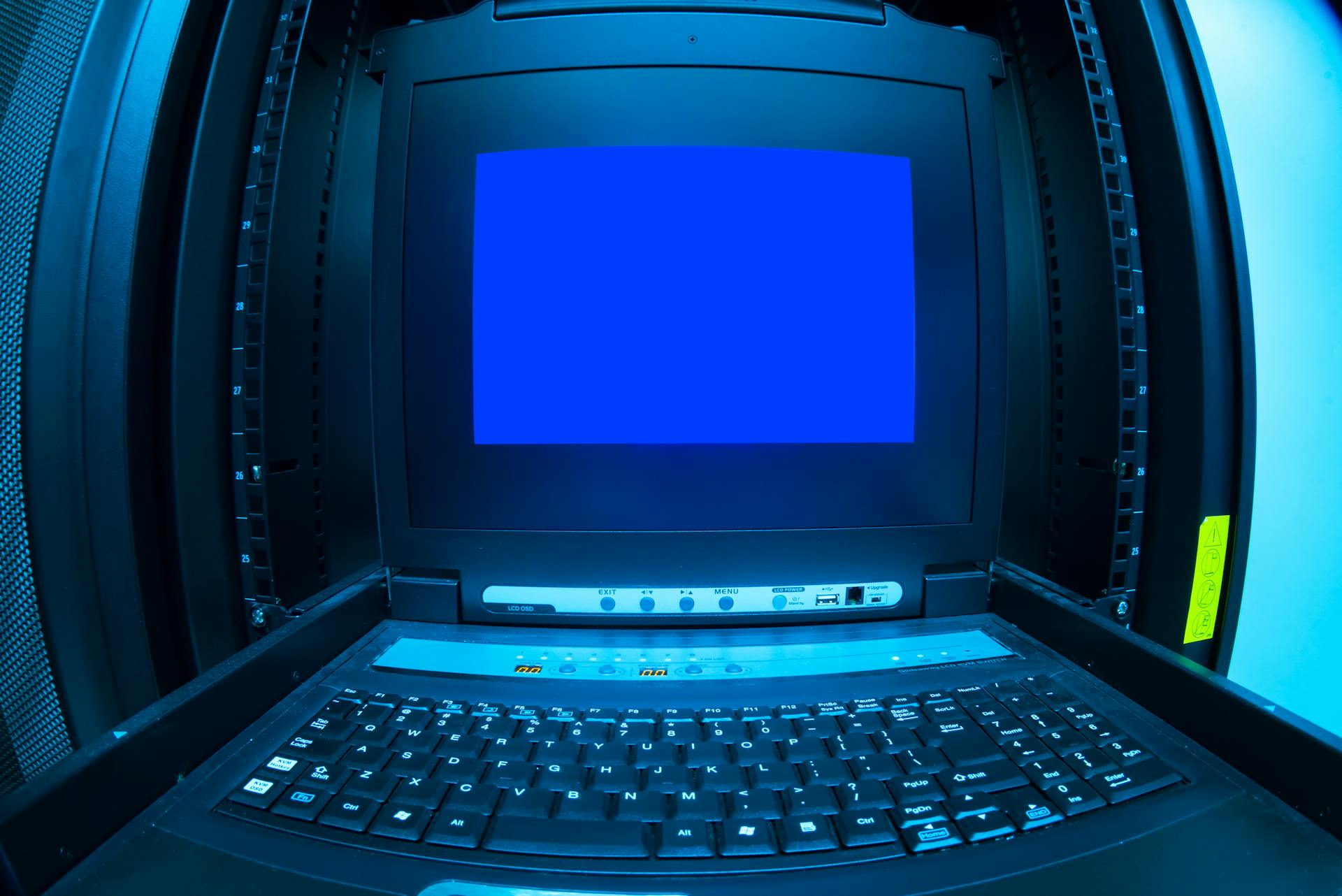
Implementing retries with exponential backoff for transient errors is also a good idea. This will prevent your application from crashing due to temporary issues.
One common mistake to avoid is exposing your API key in client-side code. Keep your API key secure and don't share it with anyone.
Here's a summary of the best practices to keep in mind:
- Always check the response status code before processing the data.
- Log errors for further analysis and debugging.
- Implement retries with exponential backoff for transient errors.
- Keep your API key secure and do not expose it in client-side code.
Own Your Data
In Azure OpenAI, you have the unique ability to use your own data, which isn't possible with the non-Azure service.
The "use your own data" feature is only available with Azure OpenAI, and you'll need to follow the setup instructions in the Azure OpenAI using your own data quickstart for detailed guidance.
To take advantage of this feature, you'll need to have a client configured specifically for Azure OpenAI, as it won't work with the non-Azure service.
This feature is designed to give you more control over your data and how it's used, which is especially important for businesses and organizations with sensitive information.
Explore further: Azure Speech Service Api Key
Use Chat Tools
Using chat tools is a powerful way to extend chat completions by allowing an assistant to invoke defined functions and other capabilities in the process of fulfilling a chat completions request.
To use chat tools, start by defining a function tool. This involves creating a new definition that the assistant can use to invoke specific functions.
Including the new definition in the options for a chat completions request is the next step. This will allow the assistant to access the tool and use it to fulfill the chat completions request.
The response message from the assistant will include one or more "tool calls" that must be resolved via "tool messages" on the subsequent request. This resolution of tool calls into new request messages can be thought of as a sort of "callback" for chat completions.
To provide tool call resolutions to the assistant, you'll need to provide all prior historical context, including the original system and user messages, the response from the assistant that included the tool calls, and the tool messages that resolved each of those tools.
A fresh viewpoint: Azure Openai What's New
If you're using tool calls with streaming responses, you'll need to accumulate tool call details much like you'd accumulate the other portions of streamed choices. This involves using the accumulated StreamingToolCallUpdate data to instantiate new tool call messages for assistant message history.
There are three options for controlling the behavior of tool calls: ChatCompletionsToolChoice.Auto, ChatComptionsToolChoice.None, and providing a reference to a named function definition or function tool definition.
Here are the details on each option:
- ChatCompletionsToolChoice.Auto is the default behavior when tools are provided and instructs the model to determine which, if any, tools it should call.
- ChatCompletionsToolChoice.None instructs the model to not use any tools and instead always generate a message.
- Providing a reference to a named function definition or function tool definition will instruct the model to restrict its response to calling the corresponding tool.
Note that the concurrent use of Chat Functions and Azure Chat Extensions on a single request is not yet supported, so you'll need to consider separating the evaluation of these across multiple requests in your solution design.
For another approach, see: Azure Chat Openai Langchain
Generating Chatbot Responses
Generating chatbot responses is a crucial aspect of building conversational AI models. You can generate multiple chatbot responses with a subscription key using the GenerateMultipleChatbotResponsesWithSubscriptionKey method.
This method allows you to create text responses to input prompts using an Azure subscription key. You can use this method to create a chatbot that can respond to a variety of user inputs.
For another approach, see: Azure Api Key
The response structure from the GenerateMultipleChatbotResponsesWithSubscriptionKey method includes several key elements. Here's a breakdown of what you can expect:
- id: A unique identifier for the request.
- object: The type of object returned (e.g., 'text_completion').
- created: A timestamp indicating when the response was generated.
- model: The model used to generate the response.
- choices: An array containing the generated text and additional metadata.
The main concept to understand when working with chatbot responses is Completions. Completions allows you to provide a text prompt, which is then matched to context and patterns using a specific model to generate an output text.
For more insights, see: Azure Openai Completions Playground
Setting Up and Testing
Setting up your Azure OpenAI API environment is a breeze, just follow the detailed steps to ensure a smooth integration with your applications.
To set up your environment, you'll need to follow the instructions provided, which will take you through the process of ensuring everything is configured correctly.
After setting up your environment and writing the code, run your script to test the connection. If everything is configured correctly, you should receive a response from the Azure OpenAI API.
You can authenticate with Azure Active Directory using the Azure Identity library, or use client subscription key authentication, which is used in most of the examples in this getting started guide.
To use the Azure Identity library, you'll need to install the Azure.Identity package, which will allow you to use the DefaultAzureCredential provider or other credential providers provided with the Azure SDK.
Testing your setup is a crucial step, and it's easy to do - just run your script and check if you receive a response from the Azure OpenAI API.
Troubleshooting and Comparison
Troubleshooting with Azure OpenAI API involves understanding the HTTP status codes returned by the service. If you try to create a client using an endpoint that doesn't match your Azure OpenAI Resource endpoint, a 404 error is returned, indicating Resource Not Found.
The .NET SDK used for Azure OpenAI API returns errors corresponding to the same HTTP status codes as REST API requests. This makes it easier to identify and troubleshoot issues.
Azure OpenAI's API has been found to be more deterministic than OpenAI's, especially in tasks that require consistency, such as reverse-lookup from embeddings to text. This is evident in the comparison of Azure's text-embedding-ada-002 outputs, which are identical given the same input, whereas OpenAI's outputs are noisy.
Worth a look: Azure Openai Endpoint
A comparison of GPT-4-0613 between OpenAI and Microsoft Azure OpenAI's APIs reveals that Azure's API has a better performance profile for tasks that require consistency. In translation tasks, both APIs perform equivalently, but for almost any other task, Azure has a more deterministic output.
Here is a comparison of the deterministic properties of Azure OpenAI's GPT-4-0613 and OpenAI's GPT-4-0613:
Troubleshooting
Troubleshooting Azure OpenAI errors can be a challenge, but knowing the common issues can help you resolve them quickly. A 404 error is returned when you try to create a client using an endpoint that doesn't match your Azure OpenAI Resource endpoint, indicating Resource Not Found.
To troubleshoot, you can check the HTTP status codes returned by the service. These codes correspond to the same errors returned for REST API requests. For example, a 404 error indicates that the resource was not found.
If you're experiencing issues with the .NET SDK, you can refer to the list of compatible and additional computed target framework versions for different products. This can help you identify potential issues and resolve them.
Here's a list of compatible target framework versions for different products:
Comparing Models vs Microsoft APIs
OpenAI models, like the GPT-4-0163 model, don't always produce the same output, even with the same input. This is because GPT models are non-deterministic by nature.
In one study, researchers found that calling the text-embedding-ada-002 model through OpenAI's API produced a variety of results given the same input string. This highlights the potential for indeterminacy in LLM performance.
Switching from OpenAI's standard API to their Azure endpoint completely eliminated the noise distribution for text-embedding-ada-002. This suggests that using Azure's API might reduce variation in output.
For tasks like creative writing and open-ended factual questions, Azure's API has significantly less variation in output compared to OpenAI's API. However, for tasks like translation or simple factual question answering, Azure's API provides more consistent performance.
Comparison of Text-Embedding-Ada-002
The comparison between OpenAI and Azure on text-embedding-ada-002 is quite telling. Azure's outputs are identical given the same input.
One of the key differences is that OpenAI's outputs are noisy, whereas Azure's are consistent. OpenAI produces about 10 unique embeddings per 100 trials of the same input sentence.
If you need absolute consistency in embedding outputs, such as for a reverse-lookup from embeddings to text, Azure OpenAI's API can support those needs.
You might like: Azure Openai Ada Embedding
Comparison of GPT-4-0613
Azure's gpt-4-0613 is not as deterministic as we thought, even with temperature=0, which should produce mostly deterministic output.
In fact, it's more deterministic than OpenAI's gpt-4-0613, but still produces a variety of potential completions.
We noticed trends in the prompts that helped us understand the differences between the two models. For example, prompts 0-9 are more likely to be "generation" tasks, like writing poems or describing a real place.
Prompts 10-13 are about straightforward facts, and the remainder (14 and to the right) are all translation tasks. This helped us see that the exception to Azure's more deterministic responses is when it comes to simple factual answers and translation.
Both OpenAI and Azure's APIs return similar numbers of distinct completions per input and produce the same variants, largely consistent across both APIs, especially for translation tasks.
However, for almost any other task, if consistency is required, Azure has a better performance profile. This is evident in the overlap in completion between the models, which shows little agreement between outputs depending on the task.
You might like: Langchain Azure Openai Gpt-4
Frequently Asked Questions
What is the difference between OpenAI and Azure OpenAI?
OpenAI offers open access to its models for public experimentation, whereas Azure OpenAI is designed for businesses with a Microsoft Enterprise agreement, providing more control over costs. Azure OpenAI offers flexible pricing models, including Pay-As-You-Go and PTUs, for enterprises.
How to get OpenAI API key from Azure?
To get your OpenAI API key from Azure, log in and navigate to the "Create a resource" section, then follow the prompts to create and access your keys. Once created, find your API key in the "Keys and Endpoints" section of your Azure OpenAI resource.
Does OpenAI have an API?
Yes, OpenAI has an API that allows users to access its capabilities programmatically. Learn more about managing API keys and environments.
Sources
- https://azuresdkdocs.blob.core.windows.net/$web/dotnet/Azure.AI.OpenAI/1.0.0-beta.5/index.html
- https://www.restack.io/p/openai-python-answer-azure-openai-api-tutorial-cat-ai
- https://www.nuget.org/packages/Azure.AI.OpenAI/1.0.0-beta.13
- https://www.willowtreeapps.com/craft/openai-or-azure-openai-can-models-be-more-deterministic-depending-on-api
- https://www.clearpeople.com/blog/overwriting-azure-openai-api-api-version-property-using-semantic-kernel
Featured Images: pexels.com