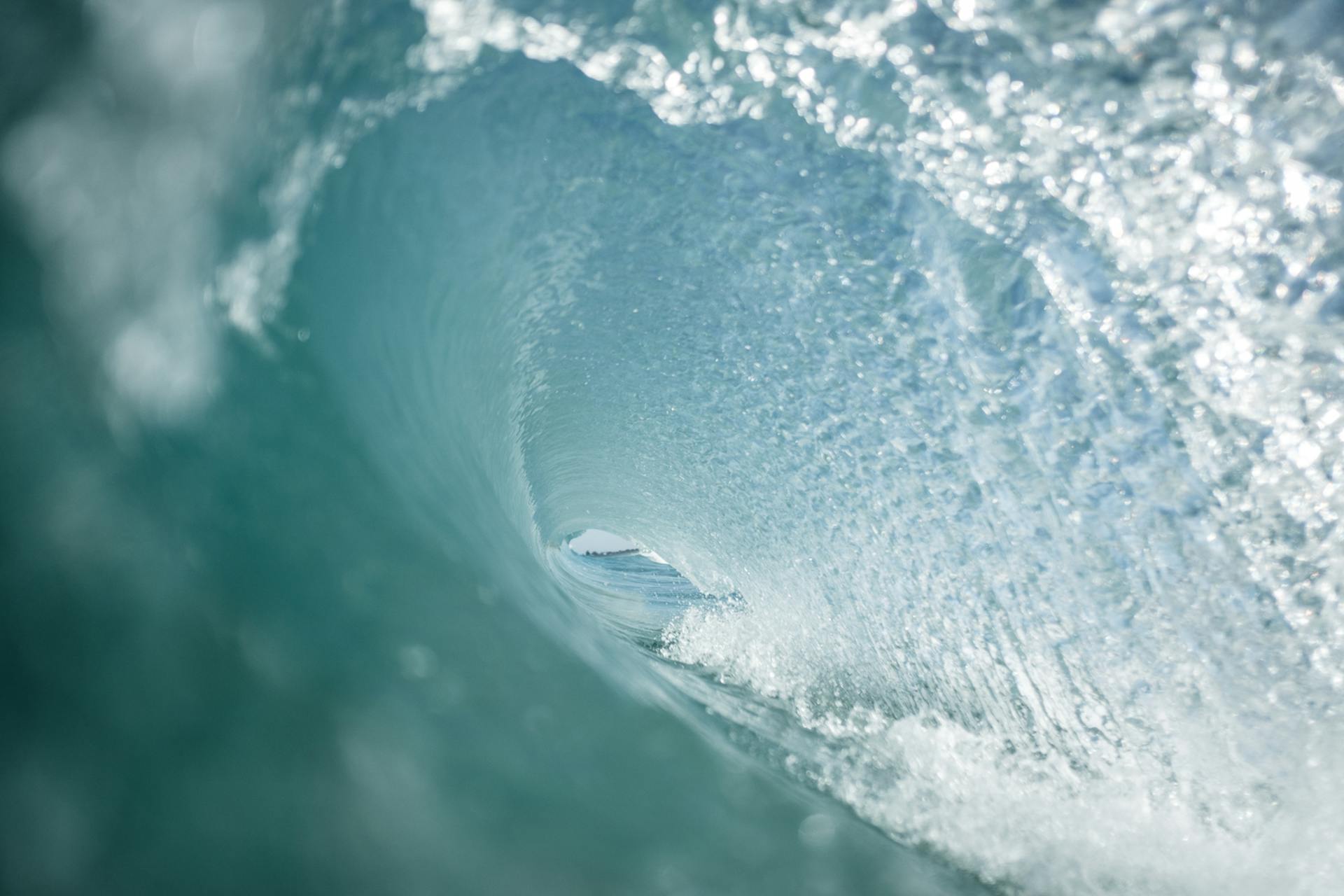
To get started with Azure Speech Service, you'll need an API key, which is a unique identifier that allows you to interact with the service.
First, sign up for an Azure account if you haven't already. This will give you access to the Azure portal, where you can create and manage your API keys.
In the Azure portal, navigate to the Speech Service page and click on the "Get started" button. This will take you to the Azure Speech Service dashboard.
Your API key is displayed on this dashboard, and you can copy it by clicking on the "Copy to clipboard" button. Be sure to store your API key securely, as it's used to authenticate your requests to the Speech Service.
Obtaining a Key
To obtain an Azure Speech Service API key, you'll need to create an Azure Cognitive Services resource. This involves navigating to the Azure portal, clicking on the Create a resource button, and selecting Cognitive Services from the list.
Here's an interesting read: How to Create Service Principal in Azure
You can then follow the prompts to set up your resource, ensuring you select the appropriate region and pricing tier that suits your needs. This will get you started on the path to obtaining your API key.
Here's a step-by-step guide to accessing your API key once your Azure Cognitive Services resource is approved: navigate to the Azure portal, select your newly created resource, and navigate to the Keys and Endpoint section. Your API key will be listed under Key1 and Key2, and you can use either key for your applications.
Create Cognitive Services Resource
To create a Cognitive Services Resource, navigate to the Azure portal and click on the Create a resource button.
You can select Cognitive Services from the list.
Ensure you select the appropriate region.
Follow the prompts to set up your resource.
Select the pricing tier that suits your needs.
Take a look at this: What Is Azure Cognitive Services
Access Your Key
Once you've created your Azure Cognitive Services resource, it's time to access your API key. You can do this by going to the Azure portal, selecting your newly created resource, and navigating to the Keys and Endpoint section. Here, you'll find your API key listed under Key1 and Key2.
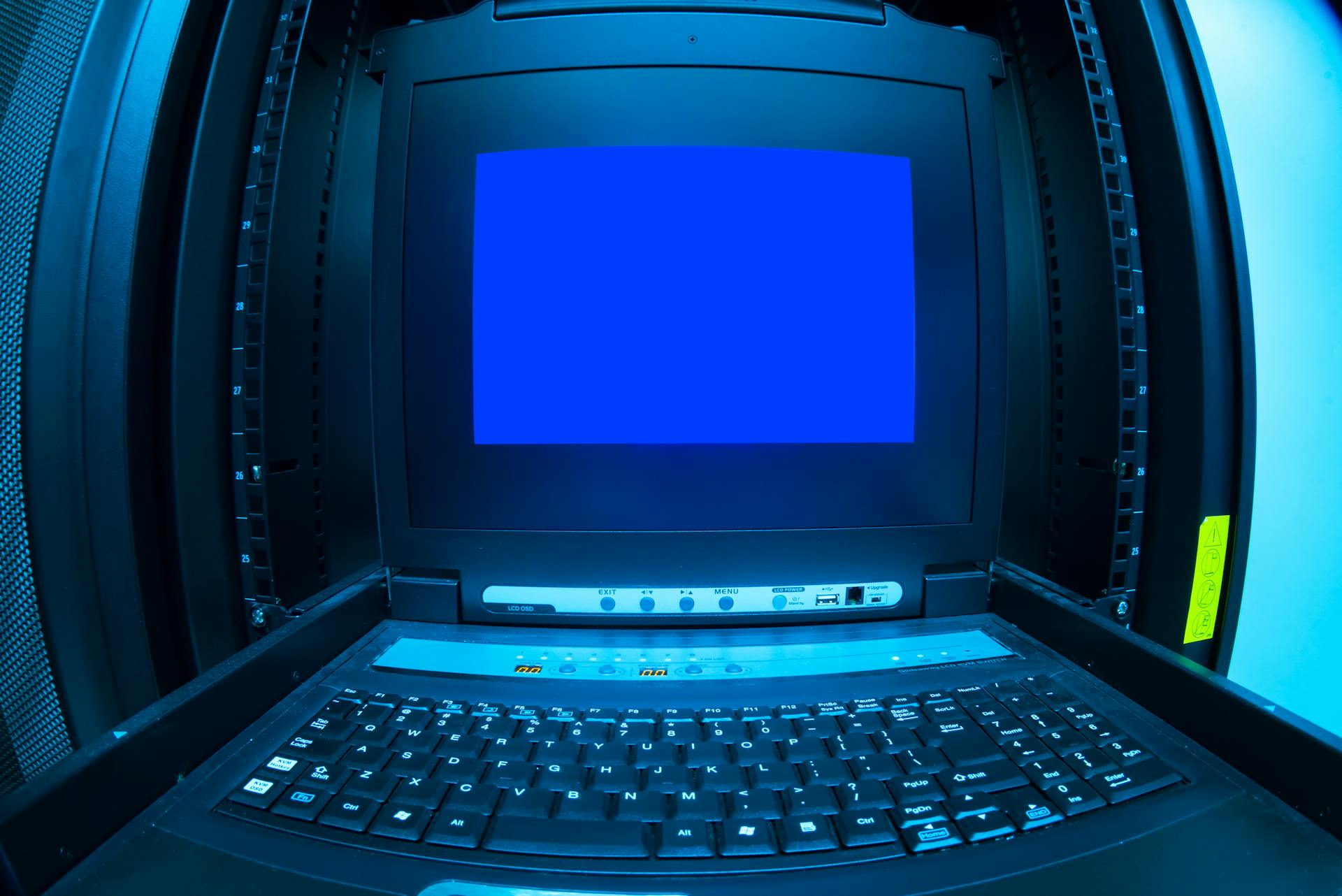
You can use either key for your applications, so don't worry if you're not sure which one to use. Just copy and paste the key into your code or application, and you're good to go.
To recap, accessing your API key is a straightforward process that requires just a few clicks in the Azure portal.
Setting Up Your Environment
Setting up your environment for the Azure Speech Service API key is a crucial step in getting started. You'll need to install the required libraries, which can vary depending on your programming language.
For example, if you're using Python, you'll need to install the requests library to handle HTTP requests. This can be done using pip: pip install requests.
To keep your API key secure, store it in a safe place. Avoid hardcoding it directly into your source code, as this can be a security risk. Instead, use environment variables or secure vaults to manage sensitive information.
Readers also liked: Azure App Service Environment Variables Key Vault
Making Calls
To make API calls, you'll need to make a POST request to the Azure TTS endpoint. This can be done using a Python code snippet, which is a good starting point for developers.
You can also use cURL, a command-line tool available in Linux and the Windows Subsystem for Linux, to get an access token. Simply replace YOUR_SUBSCRIPTION_KEY with your actual resource key for the Speech service.
In C#, you can use a class to get an access token by passing your resource key for the Speech service when instantiating the class. Be sure to change the region to match your subscription if it's not in the West US.
Http Sample
You'll need to make a POST request to the Azure TTS endpoint to convert text to speech. This is a simple HTTP request to get a token, where you'll replace YOUR_SUBSCRIPTION_KEY with your resource key for the Speech service.
The body of the response contains the access token in JSON Web Token (JWT) format. If your subscription isn't in the West US region, replace the Host header with your region's host name.
Here are the possible HTTP status codes you might encounter:
If you encounter a 200 OK status code, the body of the response contains an audio file in the requested format.
Handling Responses
When working with the Azure Speech Service API key, it's essential to handle responses correctly to avoid any issues.
The response from the Azure TTS API will contain the audio data in the specified format, such as WAV.
Ensure you handle errors appropriately by checking for status codes.
Logging any issues is crucial for debugging, so make sure to set up a reliable logging system.
You can use the status code to determine the outcome of your request and take necessary actions.
For example, a specific status code might indicate that the audio data is not available, so you can retry the request or provide an alternative solution to the user.
Discover more: Windows Azure Data Services
Text to Speech
The Azure Text-to-Speech (TTS) API is a powerful tool that can convert text into natural-sounding speech. It's perfect for developers who want to create applications like virtual assistants or accessibility tools.
To use the Azure TTS API, you'll need to follow a structured approach to ensure seamless functionality and user experience. This means integrating the API into your application in a way that's both efficient and effective.
A different take: Azure Text to Speech Api
The Azure TTS API uses Speech Synthesis Markup Language (SSML) to convert text to speech. This allows you to control the way the text is spoken, including factors like pitch, rate, and volume.
You can integrate the Azure TTS API into your application to create a wide range of applications, from virtual assistants to accessibility tools. The possibilities are endless, and the API makes it easy to get started.
Check this out: Ms Azure Tts
Prerequisites
To get started with the Azure Speech Service API key, you'll need to meet some basic requirements.
You'll need an Azure account with an active subscription to access the Speech-to-Text API.
An Azure TTS resource needs to be created in the Azure portal to enable text-to-speech functionality.
Your Azure TTS API key is essential for authenticating your requests and should be kept secure.
To interact with the API, you'll need a Python environment set up with the requests library installed.
Access to an audio file stored in Azure Blob Storage is also required.
You can use Postman to test and send requests to the API.
Here are the prerequisites summarized in a list:
- Azure account with an active subscription.
- Azure TTS resource created in the Azure portal.
- Azure TTS API key for authentication.
- Python environment with the requests library installed.
- Access to an audio file in Azure Blob Storage.
- Postman for testing and sending requests.
Regions and Endpoints
The Azure Speech Service API Key requires you to select a region and endpoint. Be sure to choose the endpoint that matches your Speech resource region.
You can use the REST API in various regions, including the ones supported for text to speech.
These regions are necessary to ensure compatibility and functionality with your Speech resource.
Consider reading: Service Endpoint Azure
Sources
- https://www.restack.io/p/custom-tts-application-development-answer-azure-tts-api-key-cat-ai
- https://prashanth-kumar-ms.medium.com/azure-speech-service-automating-speech-to-text-transcription-with-using-python-157827475da0
- https://docs.merkulov.design/how-to-get-microsoft-azure-tts-api-key/
- https://www.pragnakalp.com/speech-recognition-speech-to-text-python-microsoft-azure-aws-houndify/
- https://learn.microsoft.com/en-us/azure/ai-services/speech-service/rest-text-to-speech
Featured Images: pexels.com