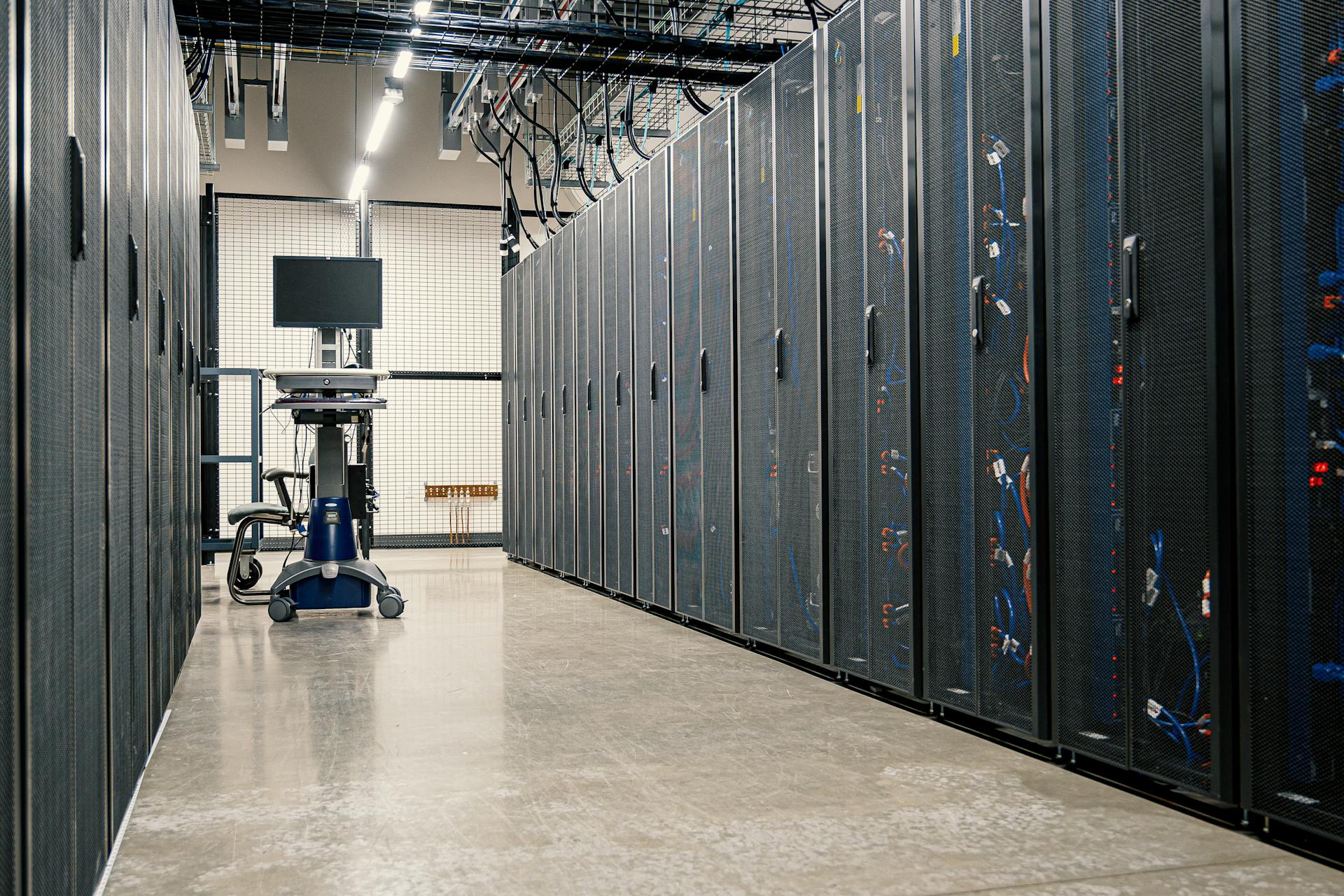
Developing Java applications on Windows Azure has never been easier. The Windows Azure SDK for Java provides a comprehensive set of tools and libraries to simplify the development process.
With the SDK, you can easily deploy your Java applications to the cloud, taking advantage of Windows Azure's scalable and reliable infrastructure.
The SDK includes a set of libraries that provide a simple and intuitive API for interacting with Windows Azure services, such as storage and compute.
By using the Windows Azure SDK for Java, you can focus on writing your application code, without worrying about the underlying infrastructure.
Related reading: Java Azure Openai
Installation and Setup
To get started with the Windows Azure SDK for Java, you'll need to set it up properly. You can create a new Maven project using the Azure SDK for Java Maven archetype, which is published to Maven Central. Simply run the following command to bootstrap a new application.
The Azure SDK for Java Maven archetype will prompt you for details about your project, such as the groupId, artifactId, package name, and Azure libraries to include. You can find a list of required and optional properties in the table below:
Alternatively, you can provide these values when you call the archetype command, which can be useful for automation purposes.
Create Maven Project
To create a new Maven project, you can use the Azure SDK for Java Maven archetype. This archetype is published to Maven Central, making it easily accessible and usable.
The archetype can be used directly to bootstrap a new application with a single command. After entering this command, a series of prompts will ask for details about your project, which will be used to generate the right output for you.
Here are the properties you need to provide values for:
You can provide these values when you call the archetype command, which is useful for automation purposes. Alternatively, you can specify the values as parameters using the standard Maven syntax of appending -D to the parameter name.
Conventions
As you set up Azure SDK tracing, it's essential to understand the conventions that govern how it emits spans and attributes.
The Azure SDK semantic conventions are not stable and may change in the future, so be sure to check the Azure SDK semantic conventions specification for the latest information.
Resource Management
You can use the management libraries to create, provision, and manage Azure resources from Java application code. These libraries are found in the com.azure.resourcemanager Maven group ID.
All Azure services have corresponding management libraries that provide a Manager class as service API. For example, the ComputeManager is for the Azure compute service.
With these libraries, you can write configuration and deployment scripts to perform the same tasks that you can through the Azure portal or the Azure CLI.
A unique perspective: Windows Azure Service Management Api
Supported Platforms
The Azure SDK for Java has specific requirements for supported platforms.
Developers should always use the latest Java long-term support (LTS) release in development and when releasing to production.
Using the latest LTS release ensures the availability of the latest improvements within Java, including bug fixes, performance improvements, and security fixes.
The Azure SDK for Java is tested and supported on Windows, Linux, and macOS.
It is not tested on other platforms that the JDK supports, and does not support Android deployments.
For developers wanting to develop software for deployment on Android devices and which make use of Azure services, there are Android-specific libraries available in the Azure SDK for Android project.
Consider reading: Azure Java
Resource Management
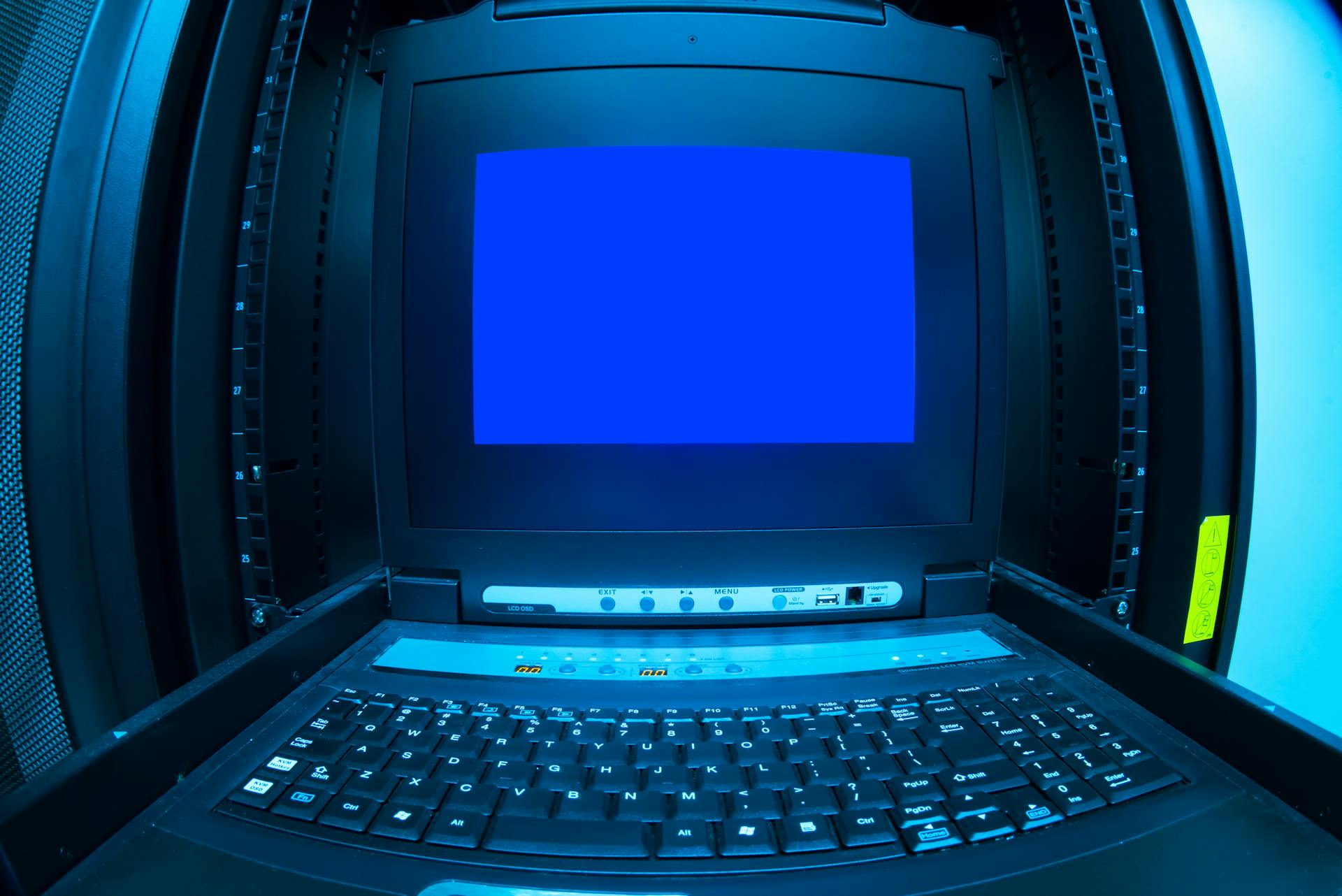
Resource Management is a crucial aspect of working with Azure services. You can manage Azure resources from Java application code using the management libraries, which are found in the com.azure.resourcemanager Maven group ID.
These libraries help you create, provision, and manage Azure resources, just like you would through the Azure portal or the Azure CLI. You can write scripts to perform tasks that would otherwise require manual intervention.
All Azure services have corresponding management libraries, making it easy to find the one you need. For example, the ComputeManager is used for Azure compute service, while the AzureResourceManager aggregates popular services.
The management libraries provide a *Manager class as service API, which allows you to interact with Azure resources programmatically. This is particularly useful for automating tasks and workflows.
With the management libraries, you can write configuration and deployment scripts to perform tasks that would otherwise require manual intervention through the Azure portal or the Azure CLI. This can save you time and increase efficiency.
A unique perspective: Windows Azure Media Services
Optimization and Performance
Improving reactive code can be a game-changer for Windows Azure SDK for Java applications. Christophe Bornet's tip shows that replacing the .subscribe() part with a .then() preserves backpressure between Spring Webflux and the Azure SDK.
By doing so, errors are also managed by Spring Webflux, resulting in clean 500 errors instead of lost errors. This is especially useful in cases where uploading a file using the Azure SDK fails.
The resulting code is a bit more advanced, but it's worth the trouble for improved performance and error handling.
If this caught your attention, see: Azure Code
Tracing with Monitor
Tracing with Monitor is a powerful tool for optimizing performance. Azure SDK tracing with Azure Monitor Java agent is a great option, as it enables monitoring of your applications without any code changes.
Using an Azure Monitor Java in-process agent can be a game-changer for your development workflow. For more information, see Azure Monitor OpenTelemetry-based auto-instrumentation for Java applications.
Azure SDK support is enabled by default starting with agent version 3.2, which makes it even easier to get started.
Explore further: Azure App Insights vs Azure Monitor
Tracing Calls with OpenTelemetry
You can enable tracing of Azure SDK calls using the OpenTelemetry Java agent, which is enabled out-of-the-box starting from version 1.12.0.
This means you don't need to make any code changes to get started with monitoring your applications. The OpenTelemetry agent artifact is stable, but it may cause span names and attribute names produced by Azure SDK to change over time if you update the agent.
To configure exporters, add manual instrumentation, or enrich telemetry, you can refer to the OpenTelemetry Instrumentation for Java documentation.
Curious to learn more? Check out: Azure Connected Machine Agent
Building a Native Image with GraalVM
You can use GraalVM to create a native image of a Java application, which can yield drastic performance gains in certain situations.
GraalVM compiles the Java code ahead of time into native machine code. The Azure SDK for Java provides the necessary metadata in each of its client libraries to support GraalVM native image compilation.
To get started, you need to install GraalVM and prepare your development system for compiling native images. The installation process for GraalVM is straightforward.
The GraalVM documentation provides step-by-step instructions for installing GraalVM and using GraalVM to install native-image. Follow the prerequisites section carefully to install the necessary native compilers for your operating system.
You can use the Azure SDK for Java Maven archetype to configure your build to support GraalVM native image compilation. Alternatively, you can add it to an existing Maven build.
To run a native image build, use the following command:
This command will output a native executable for the platform it's running on.
The executable appears in the Maven /target directory of your project. You can now run your application with this executable file, and it should perform similarly to a standard Java application.
Consider reading: Trails to Azure Wazy Build
Frequently Asked Questions
What is Windows Azure SDK?
The Azure SDK is a collection of libraries that helps you interact with Azure services from your preferred programming language. It simplifies the process of using Azure services and provides a consistent and dependable way to manage them.
How do I run Java on Azure?
To run Java on Azure, start by using Azure Cloud Shell and follow the steps to create a Java app, configure the Maven plugin, deploy it, and clean up resources. Begin by using Azure Cloud Shell and following the steps outlined in the Azure documentation.
Sources
- https://learn.microsoft.com/en-us/azure/developer/java/sdk/overview
- https://learn.microsoft.com/en-us/azure/developer/java/sdk/get-started-maven
- https://dev.to/azure/using-the-new-azure-sdk-for-java-to-upload-images-asynchronously-using-spring-reactor-20ha
- https://learn.microsoft.com/en-us/azure/developer/java/sdk/tracing
- https://learn.microsoft.com/en-us/azure/developer/java/sdk/get-started-gradle
Featured Images: pexels.com