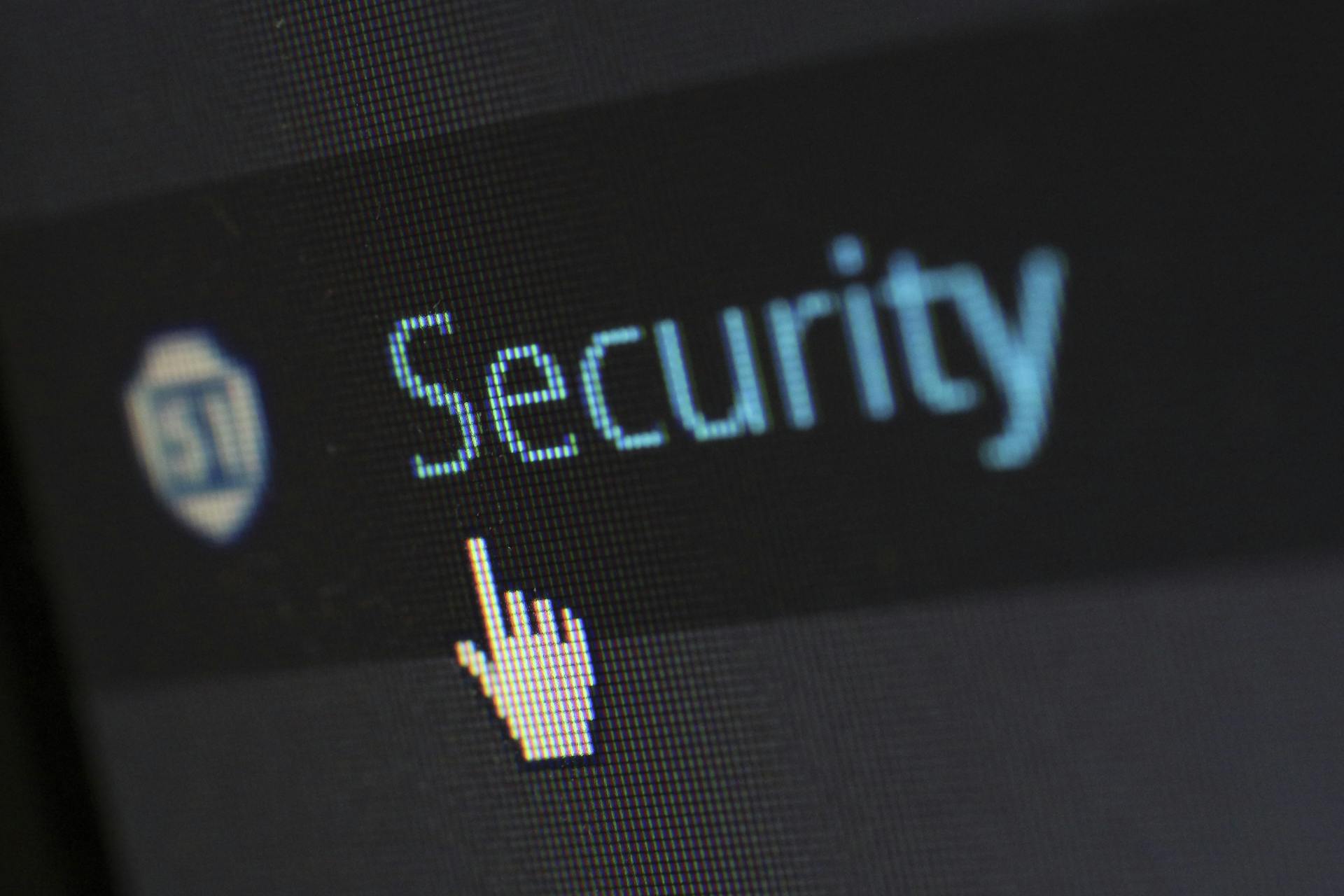
Configuring and securing your applications with JWT Azure AD authentication is a crucial step in protecting your users' sensitive information.
Azure AD provides a built-in token validation mechanism that checks the signature of the JWT token. This ensures that the token has not been tampered with or altered during transmission.
To configure JWT Azure AD authentication, you need to register your application in the Azure portal and obtain a client ID and client secret. These credentials are used to authenticate your application with Azure AD.
The client secret is a sensitive piece of information that should be kept secure to prevent unauthorized access to your application.
Azure AD Authentication
Azure AD Authentication is a crucial step in implementing JWT Azure AD authentication. To start, you'll need to configure your appsettings.json file with the Authority, which can be https://login.microsoftonline.com/your-tenant-id for general Azure AD endpoints.
The Authority might be common, so you can put it in the appsettings.json file, but never put secrets in these files. You'll also need to add authentication services, configure JWT Bearer authentication as the default, and configure authorization in your dependency injection and middleware configuration.
For Azure AD Auth, you'll need to set the authType in arcentry-conf.yml to azureAdJwt and specify the tenant ID, client ID, and client secret. You can also list alternative ad audiences and disable check nonce if you want to reuse previously issued tokens.
Configuring
Configuring Azure AD authentication can be a bit tricky, but don't worry, I've got you covered. To start, you need to create a basic ASP.NET Core API with authentication, which involves putting the necessary configuration in place.
You should put the Authority in the appsettings.json file, which should be https://login.microsoftonline.com/your-tenant-id if you're using the general Azure AD endpoints. If you're using a different environment, such as Germany, China, or U.S. Government, you'll need to use the corresponding endpoint.
It's also a good idea to have separate appsettings.Development.json and appsettings.Production.json files, and to make two applications in Azure AD. This way, you can use one in dev/test environments and the other in production, and the authority can be common in both cases.
The important bits of dependency injection and middleware configuration include adding authentication services, configuring JWT Bearer authentication as the default, configuring authorization, and applying authentication with app.UseAuthentication();. Don't forget to require the caller to have the user_impersonation scope, which is the default delegated permission that exists in every Web app/API in Azure AD.
You can use a custom claims transformation to split the scope claim into multiple claims, which makes it easier to check if the caller has a specific scope. This transformation allows you to check the caller has a scope with some value without having to do claim.Value.Split(' ').Contains("value") every time.
Here are some specific configuration settings you should be aware of:
- Authority: https://login.microsoftonline.com/your-tenant-id (or corresponding endpoint for other environments)
- user_impersonation scope: required for all callers
- custom claims transformation: splits scope claim into multiple claims
In addition to these configuration settings, you should also consider implementing a separate appsettings.Development.json and appsettings.Production.json file, and making two applications in Azure AD. This will allow you to use one in dev/test environments and the other in production, and the authority can be common in both cases.
Security Considerations
Security considerations are crucial when using Azure AD authentication. Applications that rely on tokens must ensure the integrity of claim values.
Modifying token contents through claims customization can compromise security. This is because applications assume that token contents cannot be tampered with.
To protect against malicious customizations, applications must explicitly acknowledge that tokens have been modified. This can be done by configuring a custom signing key.
Updating the application manifest to accept mapped claims is another way to protect against customizations. This approach allows applications to explicitly acknowledge modifications to token contents.
You can protect your application from inappropriate customizations in one of two ways: by configuring a custom signing key or updating the application manifest to accept mapped claims. Without this, you'll encounter the AADSTS50146 error code.
Claims Transformations
Claims transformations are a powerful feature in Azure AD authentication, allowing you to manipulate claims in a JWT token based on conditions.
You can specify a transformation to a user attribute before emitting it as a claim. For example, if you need to get the NameID from user.email for employees, but from user.extensionattribute1 for guests, you can use a transformation.
Transformations such as IsNotEmpty and Contains act like restrictions, so if a condition is not met, the claim will not be emitted. Microsoft Entra ID evaluates conditions with the same source from top to bottom, so the order in which you add conditions is important.
Here are some examples of transformations:
- IsNotEmpty: Returns true if the value is not empty.
- Contains: Returns true if the value contains a specified string.
These transformations can be used to create complex claim conditions, such as checking if a user is an employee or a guest, and emitting a claim based on that condition.
Security and Configuration
Security and configuration are crucial aspects to consider when implementing JWT Azure AD authentication. You must configure a custom signing key to protect your application from customizations created by malicious actors.
To do this, you can update the application manifest to accept mapped claims, which will allow your application to explicitly acknowledge that tokens have been modified. This will help prevent AADSTS50146 error codes from occurring.
Microsoft Entra ID returns an AADSTS50146 error code if you don't configure a custom signing key or update the application manifest. This error code indicates that your application's assumptions about token contents may no longer be correct due to modifications.
You can also protect your application from inappropriate customizations by updating the application manifest to accept mapped claims. This will allow your application to handle customizations in a secure manner.
To configure Azure AD Auth, you'll need to update the authType in arcentry-conf.yml to azureAdJwt. This will enable your application to use the Microsoft Identity Platform.
Here are the configuration options you'll need to update:
By configuring these options correctly, you'll be able to implement JWT Azure AD authentication securely and effectively.
Frequently Asked Questions
How to validate Azure AD JWT token?
To validate an Azure AD JWT token, use Azure AD's public key to verify the digital signature generated by its private key. This ensures the token's authenticity and data integrity.
What is the difference between JWT token and aad token?
AAD tokens are used for authenticating and authorizing access to Azure resources, whereas JWT tokens transmit claims between parties, consisting of a header, payload, and signature. While both tokens provide identity verification, they serve distinct purposes in different scenarios.
How to use JWT token to authenticate Azure AD using Postman?
To authenticate Azure AD using Postman, send a GET request to your application and include the JWT token in the Authorization tab as a Bearer Token. This will grant access to your application's resources, replacing the 401 response received without the token.
Sources
- https://joonasw.net/view/azure-ad-authentication-aspnet-core-api-part-1
- https://learn.microsoft.com/en-us/entra/identity-platform/jwt-claims-customization
- https://learn.microsoft.com/en-us/azure/event-grid/mqtt-client-microsoft-entra-token-and-rbac
- https://arcentry.com/api-docs/enterprise-auth-azure-ad-jwt/
- https://docs.timbr.ai/doc/docs/api/azure_jwt/
Featured Images: pexels.com