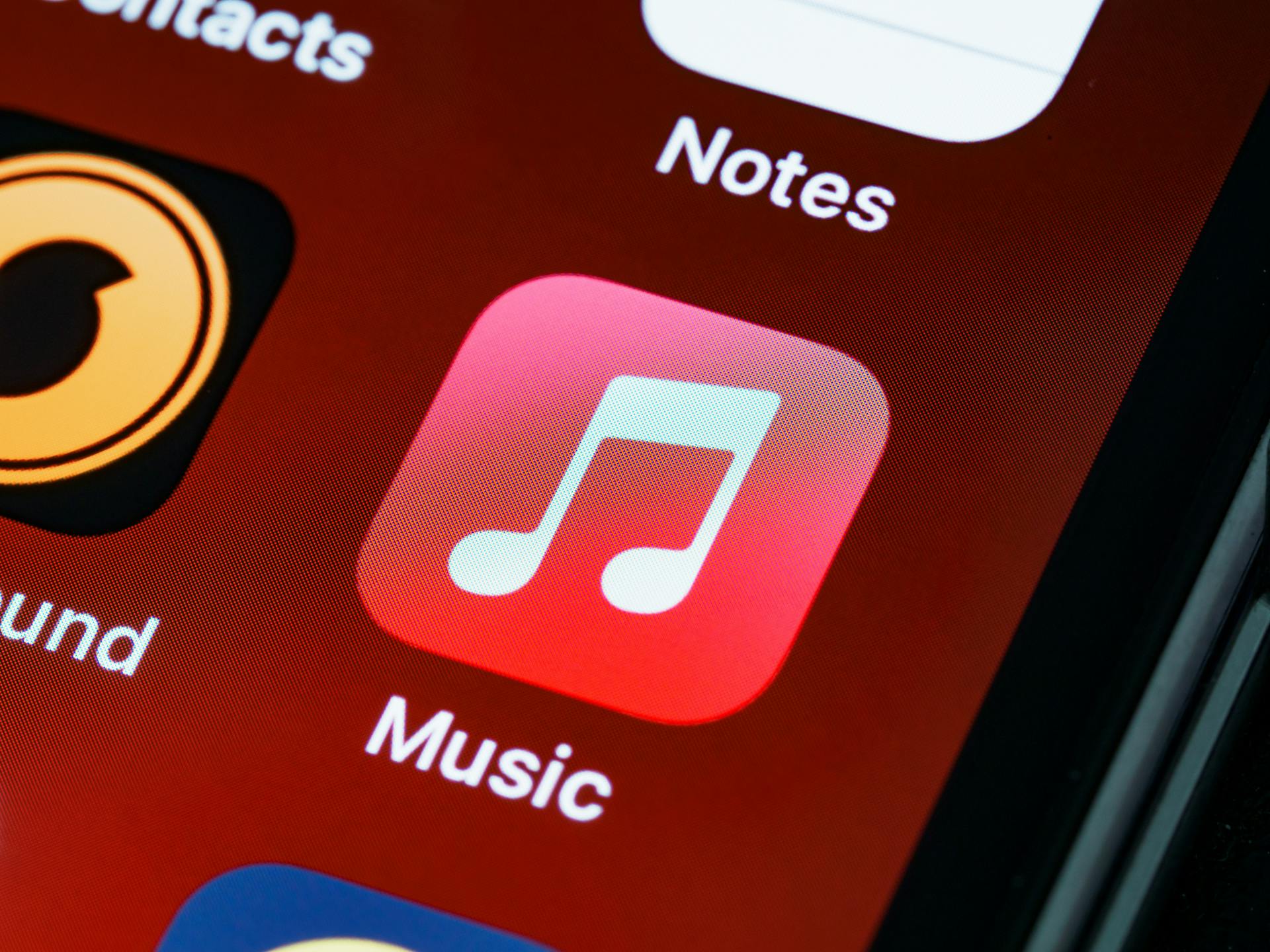
Setting up a Next.js PWA is a breeze, and it all starts with installing the necessary packages. You'll need to run `npm install next-pwa` in your project directory to get started.
Next, you'll need to configure your `next.config.js` file to enable PWA support. This involves adding a few lines of code to set up the necessary plugins and configurations.
In the `next-pwa` configuration, you can customize the appearance of your PWA by adding a manifest file. This file contains metadata about your application, such as its name, description, and icons.
To make your PWA work offline, you'll need to set up service worker support. This involves adding a `sw.js` file to your project directory and configuring it to handle cache updates and other offline-related tasks.
Why Convert Your Web App
Converting your web app into a PWA brings several benefits, including cross-platform availability. This means you only need to develop and maintain one codebase for web, mobile, and desktop apps, saving time and ensuring a consistent experience across all platforms.
A PWA can work offline or in areas with poor connectivity by caching essential resources, keeping your app functional even without internet access. This is especially useful for users who may not always have a stable connection.
PWAs are built to load quickly and run smoothly, even on slow networks, thanks to techniques like service workers and caching. This improved performance can lead to a better user experience and increased engagement.
Users can add PWAs directly to their home screen without needing an app store, making it easy for them to access your app. This, combined with features like push notifications, helps keep users engaged and coming back.
Here are some of the benefits of converting your web app into a PWA at a glance:
- Cross-platform availability
- Offline capabilities
- Improved performance
- Increased user engagement
Setting Up the Project
Let's get started with setting up our Next.js PWA project. We'll begin by examining how our app currently behaves, which is similar to any standard web application.
Our app currently behaves like a standard web application, lacking the features of a Progressive Web App (PWA). To set up the necessary files in our project, we can start by looking at the benefits of PWAs.
Next, we'll need to set up the necessary files in our project, which involves creating a new Next.js project.
Setting Up the Project
Our goal is to set up a Next.js project. We'll start by looking at how our app currently behaves, which is similar to any standard web application.
Next.js is a popular framework for building server-side rendered (SSR) and statically generated websites and applications. We'll use it to create a Progressive Web App (PWA).
Our app currently behaves like a standard web application, but we want to improve its performance and user experience by turning it into a PWA. To do this, we need to set up the necessary files in our project.
Zero Config Plugin
Setting up a Progressive Web App (PWA) for your Next.js project just got a whole lot easier with the Zero Config Plugin. This plugin takes care of registering and generating a service worker for you, so you don't have to worry about the technical details.
The Zero Config Plugin offers a range of features to help you build a robust PWA. Here are some of the key benefits:
- Zero config for registering and generating service worker
- Optimized precache and runtime cache
- Maximize lighthouse score
- Completely offline support with fallbacks example
- Use workbox and workbox-window v6
- Work with cookies out of the box
- Default range requests for audios and videos
- No custom server needed for Next.js 9+ example
- Handle PWA lifecycle events opt-in example
- Custom worker to run extra code with code splitting and typescript support example
- Public environment variables available in custom worker as usual
- Debug service worker with confidence in development mode without caching
- Internationalization (a.k.a I18N) with next-i18next example
- Configurable by the same workbox configuration options for GenerateSW and InjectManifest
- Spin up a GitPod and try out examples in rocket speed
- Support blitz.js (simply add to blitz.config.js)
- Precaching .module.js when next.config.js has experimental.modern set to true (Experimental)
Just remember that the Zero Config Plugin is only compatible with Next.js 9.1+ and requires static files to be served through the public directory. This will make things simpler for you.
Web App Criteria
To build a Next.js PWA, you need to meet certain web app criteria. Your app must be served over a secure origin, which is already met if you're developing locally.
A web manifest file is crucial for making your app installable on a user's device. This file provides metadata about your app, such as its name, icons, and start URL.
Your app must register a service worker with at least a fetch event to be recognized as a PWA and be installable. This also enhances your app's performance and reliability, allowing it to cache resources and handle network requests even when offline.
Here are the basic web app criteria you need to meet:
- Served Over HTTPS: Your app must be served over a secure origin (HTTPS) or localhost for development.
- Web Manifest File: A web manifest file provides metadata about your app.
- Service Worker with fetchEvent: Your app must register a service worker with at least a fetch event.
To give your PWA a native feel, you can set the theme color of the browser's UI, such as the address bar, to a specific color.
Offline Operation
Offline operation is crucial for a PWA, and Next.js PWA makes it easy to implement.
A service worker is used to make your app work offline, and it can be done by using a persistent storage mechanism for HTTP responses to store assets that can be reused when the app is offline.
This allows your app to serve content from the cache instead of the network, giving users a seamless experience even without an internet connection.
With a service worker, you can also provide access to the documents controlled by the service worker using an interface.
The service worker can also intercept every HTTP request made by your client PWA, allowing you to decide how to serve content – from the network or from a cache.
This control gives you the flexibility to ensure users can still access the app, even without an internet connection.
You can use the Cache Storage API to store resources locally on the user's device, which is essential for providing an offline experience.
The Cache Storage API allows you to cache your app's resources when the user is online, so they can be served from the cache when the user is offline.
Offline fallbacks are also useful when the fetch fails from both cache and network, and a precached resource is served instead of presenting an error from the browser.
This can be achieved by adding a /_offline page, which will be served when the user is offline and the requested page is not cached.
Service Worker Setup
To set up a service worker for your Next.js PWA, start by creating a service-worker.js file in the public/ directory. This script will run in the background, allowing you to control how your app handles network requests, caching, and other tasks.
Registering a service worker with at least a fetch event is essential for your app to be recognized as a PWA and be installable. This means you'll need to add some code to your RootLayoutClient.tsx file.
Create a service-worker.js file in the public/ directory with the following code:
With this setup, your PWA is now fully equipped to handle both static and dynamic content, provide an offline experience, and cache API data intelligently.
To serve cached resources to users when they're offline, you'll need to implement a caching strategy. There are several strategies to achieve this, but one way is to use the service worker to serve cached assets and API data.
Here are the basic steps to create a service worker:
- Create a file called service-worker.js in your project, which will be responsible for caching and managing other background tasks.
- Register the service worker in your main JavaScript file so the browser can find out where it lives and what it does.
These steps will give you a solid foundation for customizing the service worker for your PWA.
Offline Support
Offline support is a crucial feature for any Progressive Web App (PWA). To make your Next.js app work offline, you can use a service worker to intercept every request made by your app and decide how to serve content - from the network or from a cache.
The Cache Storage API allows you to store resources locally on the user's device, which can be used to serve content when the user is offline. This API can be used to cache HTTP responses used to store assets that can be reused when the app is offline.
To provide an offline experience, you need to cache your app's resources when the user is online and then serve these cached resources when the user is offline. This can be achieved using the Cache Storage API.
Offline fallbacks are useful when the fetch fails from both cache and network, a precached resource is served instead of presenting an error from the browser. You can create a fallback page that will be shown whenever a user tries to access content that can't be retrieved from either the cache or the network.
To implement offline support, you can use the Cache Storage API and create a fallback page that will be served when the user is offline or when a resource is unavailable. This approach ensures that users always see a meaningful message instead of an error when they're offline.
The Cache Storage API allows you to store resources locally on the user's device, which can be used to serve content when the user is offline. You can also use dynamic caching, which handles the caching process for you as files are requested.
To serve cached resources, you can use the Cache Storage API and create a fallback page that will be served when the user is offline or when a resource is unavailable. This approach ensures that users always see a meaningful message instead of an error when they're offline.
To add offline support to your Next.js app, you can use a service worker to intercept every request made by your app and decide how to serve content - from the network or from a cache. You can also use the Cache Storage API to store resources locally on the user's device.
Offline support is a crucial feature for any Progressive Web App (PWA). To implement offline support, you need to cache your app's resources when the user is online and then serve these cached resources when the user is offline. This can be achieved using the Cache Storage API.
To get started with offline support, you can add a fallback page that will be shown whenever a user tries to access content that can't be retrieved from either the cache or the network. You can also use offline fallbacks, which serve a precached resource instead of presenting an error from the browser.
To serve cached resources, you can use the Cache Storage API and create a fallback page that will be served when the user is offline or when a resource is unavailable. This approach ensures that users always see a meaningful message instead of an error when they're offline.
Offline support is a crucial feature for any Progressive Web App (PWA). To make your Next.js app work offline, you can use a service worker to intercept every request made by your app and decide how to serve content - from the network or from a cache.
Featured Images: pexels.com