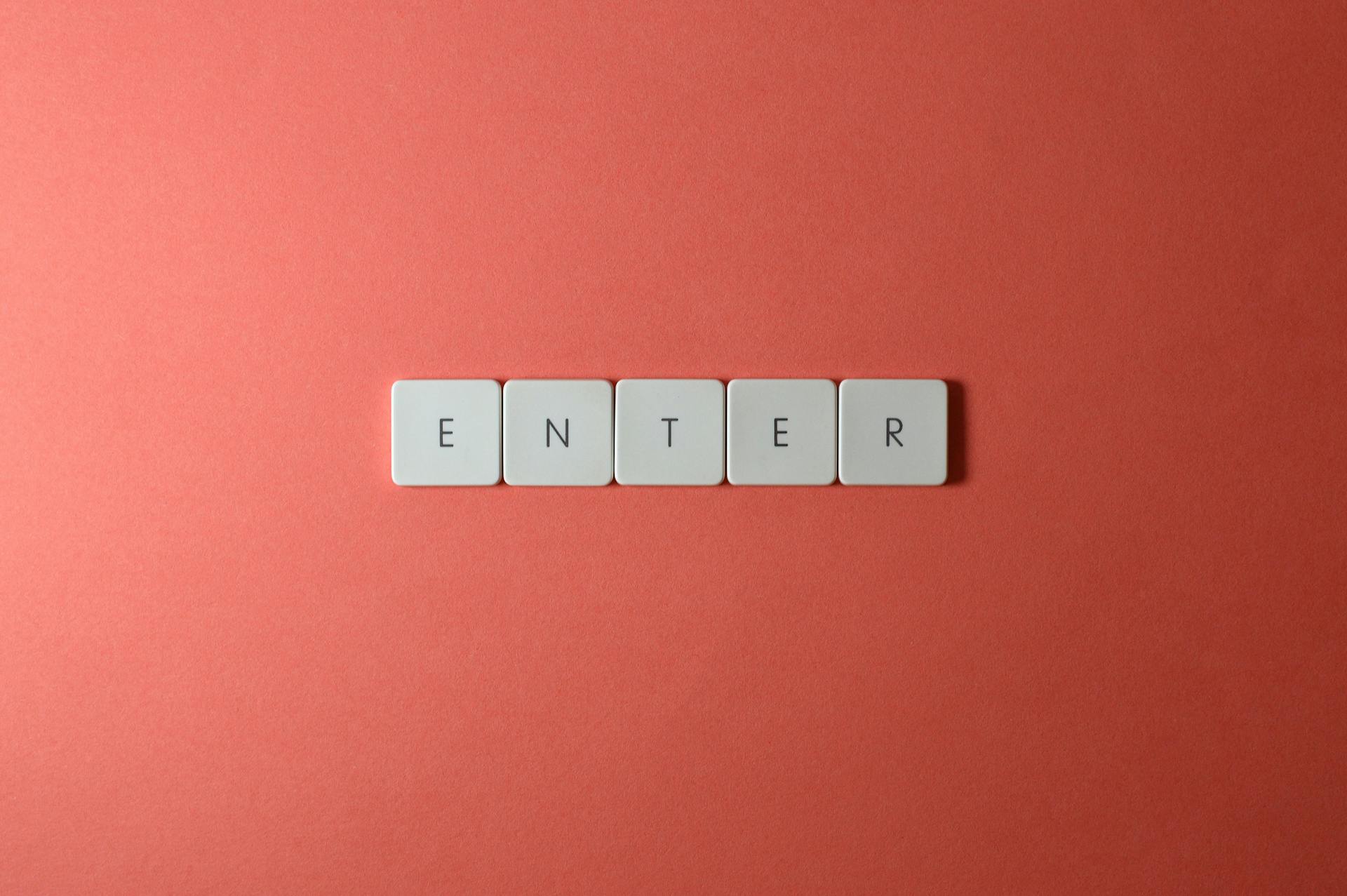
The Nextjs Button Mailto functionality is a powerful tool that allows you to create clickable buttons that can send emails directly to a user's inbox.
This functionality is achieved through the use of the "mailto" protocol, which is a standard way of creating email links in HTML.
To create a Nextjs button that opens a user's default email client, you can use the "href" attribute in your HTML code, setting it to "mailto:[email protected]".
In the example code from the "Creating a Basic Mailto Button" section, we see that the "href" attribute is set to "mailto:[email protected]", which will open the user's default email client and prefill the recipient's email address.
Getting Started with Next.js Button
To create a Next.js button, you'll need to add the `button` component to your project. This can be done using the `import` statement, as shown in the example code.
The `button` component is a basic element in Next.js, and it's easy to customize its appearance and behavior. You can change the button's text, background color, and more using the component's props.
To add interactivity to your Next.js button, you'll need to use the `onClick` event handler. This allows you to define a function that will be executed when the button is clicked.
Setting Up the Project
To set up a Next.js button project, you'll need to create a new Next.js project using the command `npx create-next-app button-project`. This will create a basic Next.js project structure.
Next, navigate into the project directory with `cd button-project` and install the required dependencies by running `npm install`.
In the `pages` directory, create a new file called `[slug].js` to serve as the button component's page. This file will contain the JSX code for the button.
Create a `components` directory to store reusable button components. This will help keep your code organized and maintainable.
Installing Required Packages
To get started with Next.js Button, you'll need to install the required packages. This includes the `@nextui/react` package, which is used to create the button components.
Next.js Button uses the `@nextui/react` package to create customizable buttons. You can install it by running the command `npm install @nextui/react` or `yarn add @nextui/react` in your terminal.
The `@nextui/react` package provides a range of button components, including the `Button` component. This component is used to create basic buttons with customizable styles.
To use the `Button` component, you'll need to import it from the `@nextui/react` package. You can do this by adding the line `import { Button } from '@nextui/react';` to the top of your JavaScript file.
Next.js Button also uses the `useState` hook to manage the button's state. This hook is used to create a state variable that can be updated when the button is clicked.
To use the `useState` hook, you'll need to import it from the `react` package. You can do this by adding the line `import { useState } from 'react';` to the top of your JavaScript file.
Creating SignInButton Component
Creating the SignInButton Component is a crucial step in building your Next.js app.
You'll need to create a new file at src/components/SignInButton.tsx. This file will contain the code for the button that allows users to sign in with their Google account.
The purpose of this component is to render a button that facilitates user sign-in.
You can find the complete code for the SignInButton.tsx file in the relevant article section.
Customizing and Styling
You can customize the appearance of your Next.js button with CSS. For example, you can change the background color, text color, and padding to match your application's design.
To add a hover effect, you can use the `:hover` pseudo-class in your CSS. This is useful for making the button more interactive and visually appealing.
You can also use classes or IDs to target specific buttons and apply different styles to them. This is useful for creating a consistent design across your application.
Designing the Button
The button is a crucial element in any UI, and its design can greatly impact the overall look and feel of your application.
A well-designed button should be large enough to be easily clickable on smaller screens, such as on mobile devices, which can be as small as 320 pixels wide.
A good rule of thumb is to make the button at least 40 pixels in height to ensure it's easily clickable on smaller screens.
The color of the button should be highly visible and contrasting with the surrounding elements to make it stand out.
The button's shape and style can also greatly impact its usability and aesthetic appeal, as seen in the example of the rounded button with a subtle shadow effect.
The button's typography, such as the font size and style, should also be carefully considered to ensure it's easily readable and consistent with the rest of the UI.
In the example of the flat button with a bold font, the typography helps to create a clean and modern look that's perfect for a minimalist design.
Customizing Button Appearance
You can change the background color of a button by adding a CSS class to its HTML element, as seen in the example where the button's background color is set to #4CAF50.
Buttons can also be customized by adding a hover effect, which can be achieved by adding a CSS pseudo-class to the button's HTML element.
To change the button's text color, you can add a CSS class to the button's HTML element, as demonstrated in the example where the button's text color is set to #FFFFFF.
Customizing the button's border can be done by adding a CSS class to the button's HTML element, allowing you to specify the border style, width, and color.
By adding a CSS class to the button's HTML element, you can also change the button's font size and weight, as shown in the example where the button's font size is set to 16px and font weight is set to bold.
Frequently Asked Questions
How to link email in React JS?
To link an email in React JS, use the mailto: protocol and provide an email prop with the recipient's address, along with optional subject and body props for initial content. Simply encode these props using encodeURIComponent to create a safe and functional email link.
How to create a button in NextJS?
To create a button in NextJS, start by creating a components folder and a Button component file, then add the necessary code to the file. This simple step-by-step process will have you building custom buttons in no time.
How do I send emails in react app using mailto?
To send emails in a React app using mailto, simply add the email address after "mailto:" in an anchor element. This will open the user's default email client and populate the recipient field.
Sources
- https://css-tricks.com/how-to-safely-share-your-email-address-on-a-website/
- https://superface.ai/blog/contact-form-nextjs-email
- https://www.corbado.com/blog/nextjs-login-page
- https://dev.to/ubahthebuilder/how-to-use-the-nextjs-link-component-with-examples-35nn
- https://docs.directus.io/blog/building-a-form-data-collection-and-email-notification-system-with-directus-and-next-js
Featured Images: pexels.com