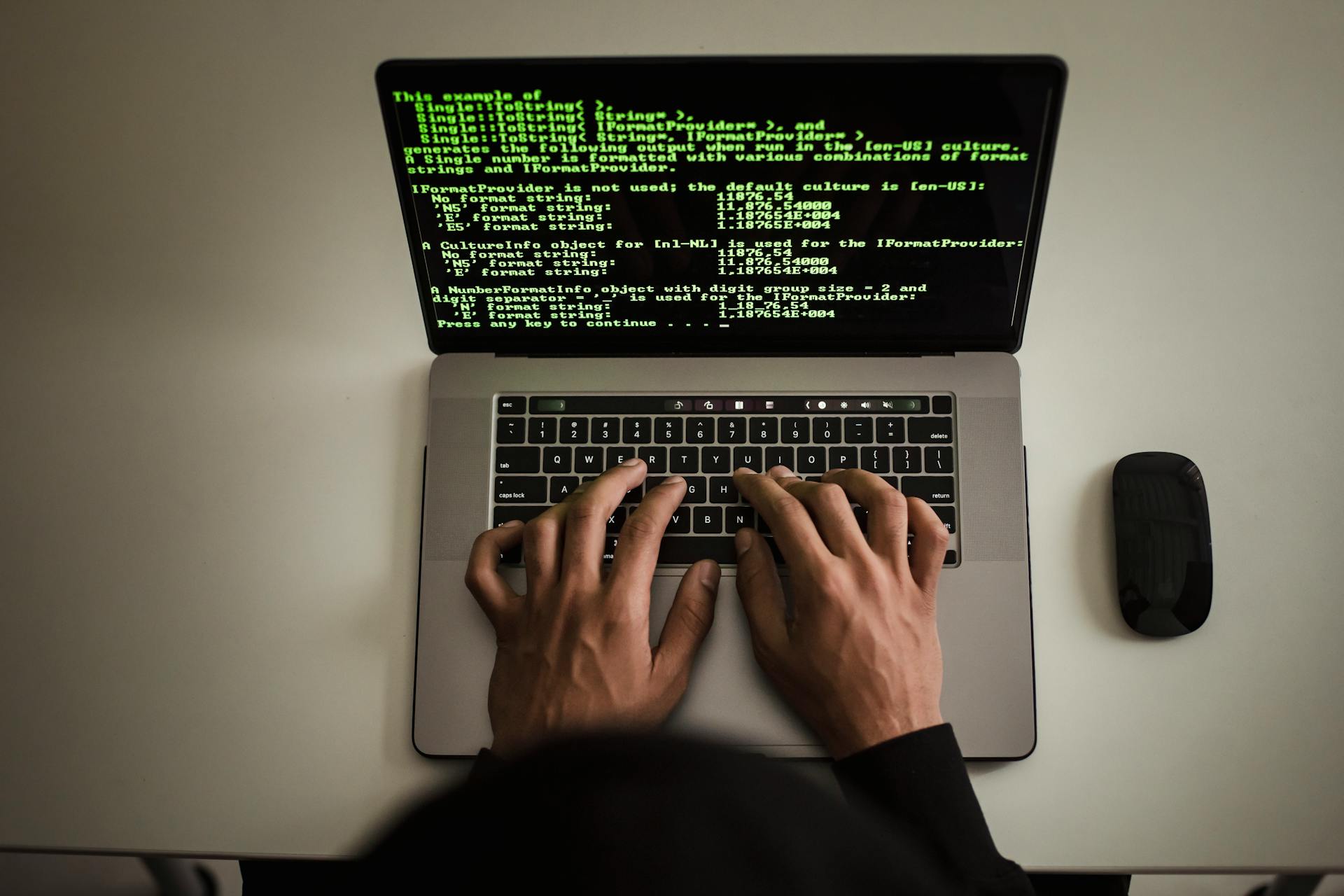
To get started with Next.js development, you'll first need to download the Next.js CLI tool. This can be done by running the command `npm install next` or `yarn add next` in your terminal.
Next.js is built on top of React, so if you're already familiar with React, you'll have a head start. Make sure you have Node.js installed on your machine, as Next.js requires it to run.
To verify that Next.js has been successfully installed, you can run the command `npx create-next-app my-app` in your terminal. This will create a new Next.js project in a directory called `my-app`.
With your Next.js project set up, you can now start exploring the different features and tools that come with it.
Getting Started with Next.js
To start with Next.js, you'll need to install it. Open a WSL command line (like Ubuntu) and create a new project folder: mkdir NextProjects and enter that directory: cd NextProjects.
Next, install Next.js and create a project by running npx create-next-app@latest my-next-app, replacing 'my-next-app' with your desired app name.
Once installed, change directories into your new app folder, cd my-next-app, and use code . to open your Next.js project in VS Code. This will allow you to explore the Next.js framework created for your app.
Notice that VS Code opened your app in a WSL-Remote environment, indicated by a green tab on the bottom-left of your VS Code window.
There are 3 commands you need to know once Next.js is installed: npm run dev, npm run build, and npm run start.
Here are the essential commands to get you started:
After running npm run dev, your local development server will start, and your terminal will display the URL to access your app: http://localhost:3000.
Configuring Next.js
To start configuring Next.js, you'll need to create a new project by running `npx create-next-app my-app` in your terminal. This will set up a basic Next.js project with a directory structure and a few example pages.
The `next.config.js` file is where you'll configure most of your Next.js settings. For example, you can set the `target` option to `'serverless'` to enable serverless mode. This will allow you to deploy your app to a platform like Vercel or AWS Lambda.
You can also use the `export` option to specify which pages should be exported as static HTML files. For example, you might set `exportPathMap` to an object that maps page paths to their corresponding export paths.
Add API Route
To add an API route to your Next.js project, you'll want to create a file called [...nextauth].js in the pages/api/auth directory. This file contains the dynamic route handler for NextAuth.js, which will also hold all your global NextAuth.js configurations.
Requests to /api/auth/*, such as signIn, callback, and signOut, will automatically be handled by NextAuth.js. This means you can focus on building your app's functionality without worrying about authentication details.
If you're using Next.js 13.2 or above with the new App Router, you can initialize the configuration using the new Route Handlers, following the guide provided.
Server Components
Server components are a key part of Next.js. They allow you to render components on the server, which can improve performance and SEO.
This is done through the use of the `getServerSideProps` method, which is used to pre-render pages at request time. The method takes a function as an argument, which returns an object with props for the page.
Server components also have access to the entire request context, including the request and response objects. This allows for more complex server-side logic to be implemented.
By using server components, you can take advantage of the benefits of server-side rendering, including improved SEO and faster page loads.
Straightforward Deployment
Deploying your Next.js project is a breeze, thanks to Prisma's seamless integration with Vercel. This means you can easily host your app on a platform specifically designed for Next.js apps.
Vercel is a platform built for Next.js apps, making it the perfect choice for hosting your project.
Sources
Featured Images: pexels.com