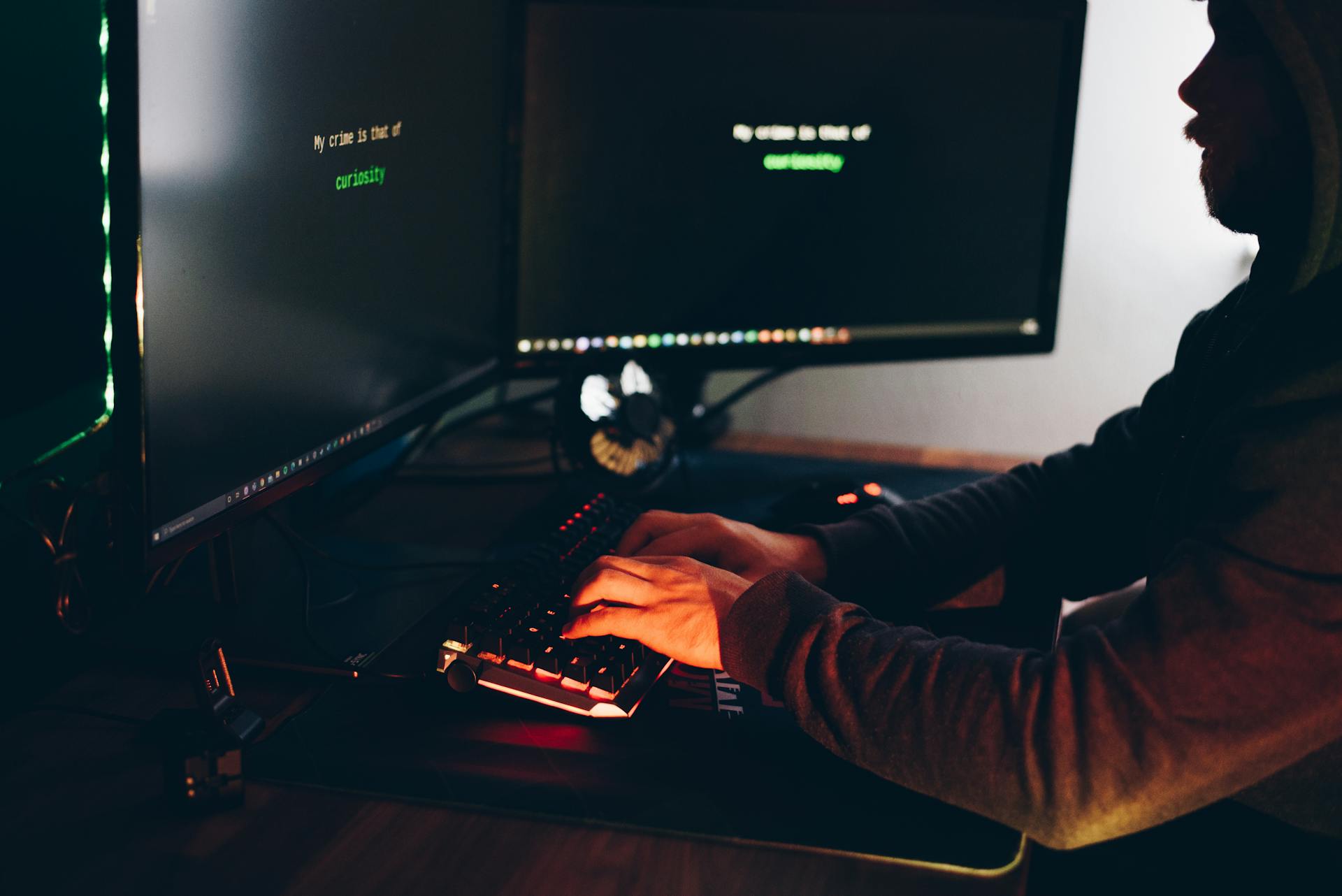
Next.js heap out of memory issues can be frustrating and time-consuming to resolve. According to the article, the main cause of these issues is the large memory usage of Next.js pages.
As mentioned in the article, Next.js pages can consume a significant amount of memory due to the use of server-side rendering and static site generation. This can lead to out-of-memory errors, especially when dealing with complex pages or large datasets.
To resolve these issues, it's essential to identify the root cause of the problem. The article suggests checking for infinite loops, large data structures, and unnecessary computations that can cause memory usage to spike.
By addressing these issues, developers can significantly reduce memory usage and prevent Next.js heap out of memory errors.
For another approach, see: Nextcloud Memory
What Are Memory Leaks?
A memory leak is when your application's memory usage keeps growing and growing, eventually overwhelming the system.
It's challenging to know if you have a memory leak, as it can be hidden until it's too late. At Waitrose, they only noticed a memory leak after their application had been in production for several days.
A different take: Nextjs Memory Cache
Memory leaks are frustrating and tedious to resolve, especially in large monolithic applications. They can be incredibly challenging to diagnose.
A memory leak occurs when some of the memory used is never "cleaned up", leading to a sustained upward trend in usage. This can happen when your application's memory usage fluctuates based on usage, but some memory is left behind.
In a healthy application, memory usage should decrease when users leave, but with a memory leak, it just keeps growing.
Causes of Memory Leaks
Memory leaks are a real issue in Next.js development, and it's essential to be aware of the common causes to avoid them. Global variables can cause memory leaks if they persist for the application's lifetime and continue to grow.
Closures, which are functions enclosed within another function, can also lead to memory leaks if they continue to have access to the outer function's scope and "remember" the outer function's variables used in the closure.
Timers and intervals are a common source of memory leaks, as the function you pass to either setInterval or setTimeout will never be cleared by the garbage collector unless you use clearInterval or clearTimeout.
Event listeners can cause memory leaks if they're not removed once they're no longer needed, leaving behind a reference to the element that's been removed from the DOM.
Third-party libraries can introduce memory leaks, especially if they're not designed for use with server-side rendering, which can be a problem in Next.js.
Diagnosing Memory Leaks
Memory leaks in Next.js can be caused by components not being properly unmounted, leading to a gradual increase in memory usage.
One common cause of memory leaks is using the `useEffect` hook without a cleanup function, as seen in the example where a component is not properly cleaned up.
A memory leak can also occur when using a library like `react-query` without properly handling cache invalidation.
To diagnose memory leaks, you can use tools like the Chrome DevTools Memory Profiler, which can help identify memory-intensive components or libraries.
The `next/image` component can also cause memory leaks if not used correctly, especially when using the `blur` option.
In some cases, memory leaks can be caused by circular references in your code, which can be difficult to identify without using a tool like a memory profiler.
By identifying and addressing these common causes of memory leaks, you can help prevent Next.js from running out of memory.
Problem
Our Next.js app is constantly running out of memory. This is a frustrating issue that can be caused by a variety of factors.
The first step in troubleshooting is to examine the error logs. In this case, we see that running Node commands like `node --max_old_space_size=4096 node_modules/.bin/react-scripts start` allocates extra memory to certain functions, but doesn't seem to work when running a command like `npm run dev`.
A cyclic reference, where a component is inside the same component, is a common cause of memory leaks. This can lead to the app crashing again, as infinite RAM does not exist.
Here are some possible causes of memory leaks in Next.js apps:
- Cyclic references
- Automatic imports of unnecessary modules
In one case, a developer encountered a similar issue due to VS code automatically importing a log method from an existing npm module. This caused the server to crash in dev mode and also affected the storybook CLI.
Solutions
To fix the Next.js heap out of memory issue, you can try the following solutions.
If you're getting the error "Unknown or unexpected option: --max_old_space_size" when running npm run dev, try using "NODE_OPTIONS=\"--max_old_space_size=4096\" next" as the dev script in your package.json file.
You can also try deleting the .next folder and running npm run dev again. This solution worked for someone who had a similar issue.
To avoid circular dependencies, check your imports and use madge to help you identify any issues. This can help you solve the problem and improve your code.
For more insights, see: Hydration Issue Nextjs
If you're experiencing the issue on a VPS, try building your project locally and then moving the built folder to the VPS. This worked as a workaround for someone who had the issue.
Here are some specific steps to try:
- Delete the .next folder
- Run npm run dev
Encapsulating children results into the template type everywhere you're getting children items and removing fragments from your GraphQL query can also help solve the memory heap issue.
Updating TypeScript
Updating TypeScript can be a straightforward process, but it's not always a guaranteed solution to out-of-memory errors.
Updating to the latest version of TypeScript was attempted to resolve out-of-memory errors, but it didn't resolve the issue in this case.
The internet, including GitHub issues and Stackoverflow, is full of posts about out-of-memory errors while using the TypeScript compiler, which were often resolved by updating to a newer version.
In some cases, updating to the latest version can resolve issues, but it's essential to try other solutions as well.
One Answer
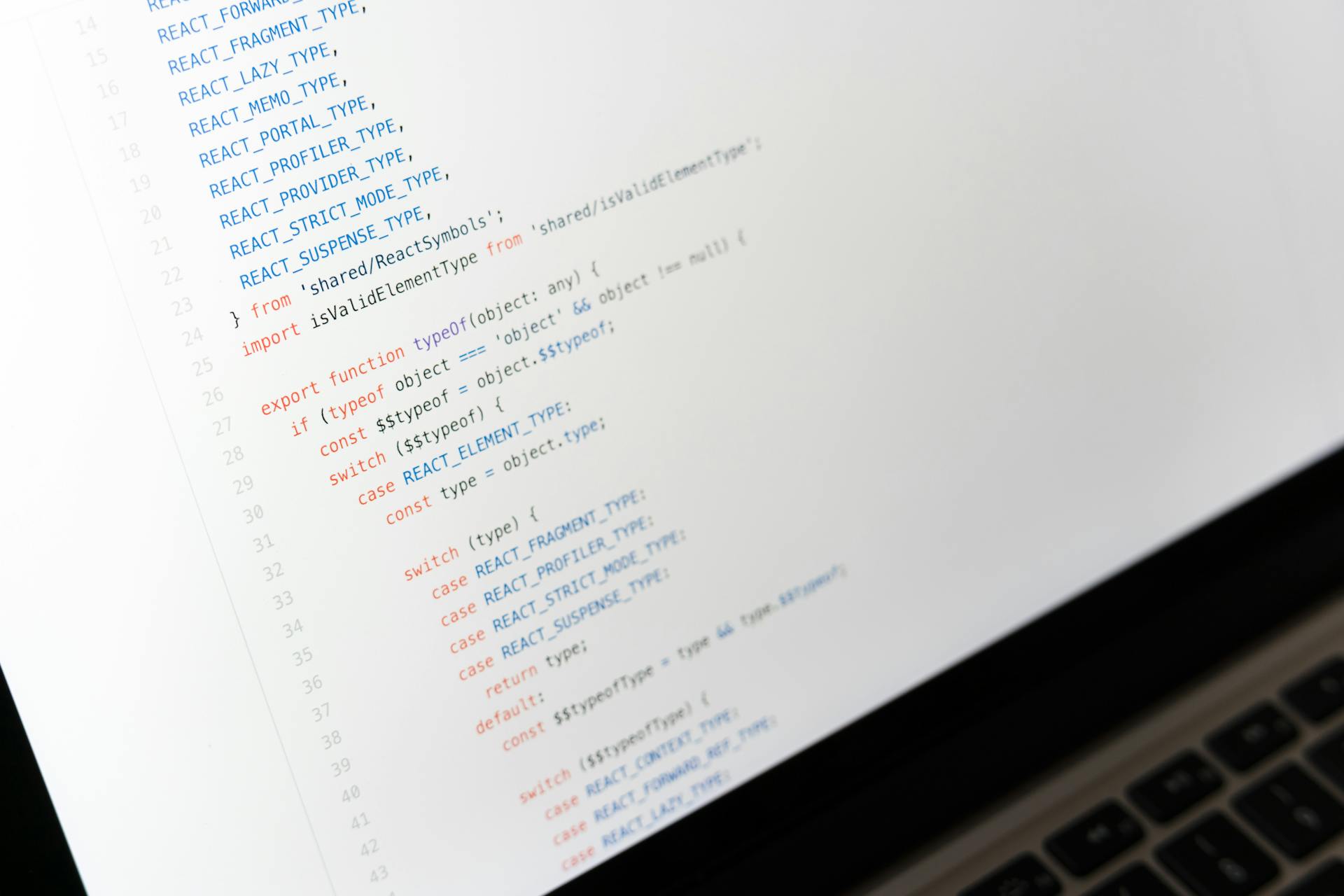
Increasing the Node.js heap size can be done by running `node` with the `-max-old-space-size` flag and specifying the amount of memory in megabytes. To allocate 4GB, use the command `node -max-old-space-size 4096`.
You can also set the V8 heap size by using the `NODE_OPTIONS` environment variable, like this: `NODE_OPTIONS=--max-old-space-size=4096 next`. This is the syntax that worked well for some users.
The effect on performance will depend on how much RAM you have and how big you make the cache. It's worth noting that increasing the heap size may not always solve the problem, as the root cause may be a circular dependency or other issue.
If you're experiencing issues with imports, make sure to check for circular dependencies. You can use tools like Madge to help identify any issues.
To troubleshoot the problem, try building your project on a local machine to see if the error occurs. If it does, you may want to try deleting the `.next` folder and building again.
Worth a look: Why Use Nextjs
Frequently Asked Questions
What does heap out of memory mean?
A "heap out of memory" error occurs when a program runs out of allocated memory, often due to inefficient code or handling large datasets. This can cause a JavaScript application to crash or freeze.
How to detect memory leak in NextJS?
To detect memory leaks in NextJS, use tools like Chrome DevTools, Node, or third-party monitoring tools to identify and track memory usage, and then review best practices such as avoiding unintentional globals and cleaning up event listeners. By following these steps, you can pinpoint and fix memory leaks in your NextJS application.
Sources
- https://posthog.com/docs/libraries/next-js
- https://medium.com/john-lewis-software-engineering/we-had-a-leak-identifying-and-fixing-memory-leaks-in-next-js-622977876697
- https://stackoverflow.com/questions/63854361/how-to-allocate-more-memory-to-javascript-heap-using-next-js
- https://sitecore.stackexchange.com/questions/36120/graphql-let-codegen-giving-javascript-heap-out-of-memory-exception
- https://carlrannaberg.medium.com/overcoming-javascript-heap-out-of-memory-error-during-typescript-compilation-in-a-mui5-react-21396cc8a4e1
Featured Images: pexels.com