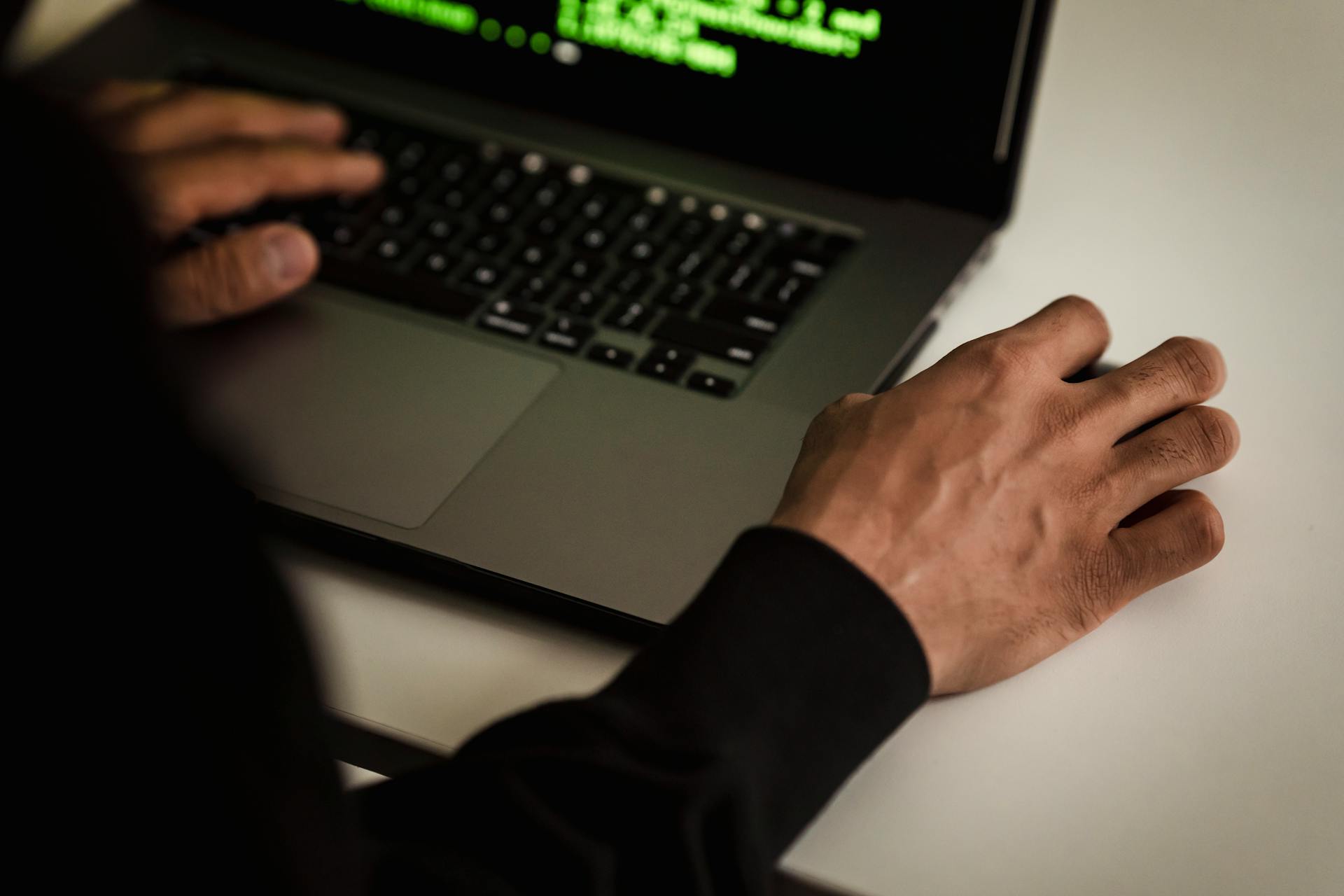
Hydration Issue Nextjs can be frustrating, especially when you're trying to get your app up and running. This issue occurs when Next.js fails to hydrate the server-rendered components.
A common cause of this issue is the use of getStaticProps with getServerSideProps. This can lead to server-side rendering not being triggered, resulting in hydration issues.
To resolve this issue, you can try disabling getStaticProps and using getServerSideProps alone. This can help ensure that server-side rendering is triggered correctly.
See what others are reading: Next Js Hydration
Causes and Solutions
Hydration issues in Next.js can be caused by various factors. Dynamic content and client-side changes can introduce discrepancies between server and client rendering.
Common causes of hydration errors include dynamic content, client-side changes, and third-party libraries that manipulate the DOM directly. These issues can lead to mismatches between server-rendered HTML and client-rendered content.
To solve hydration errors, you can reconfigure logic with useEffect, disable SSR on some components, or suppress warnings by using suppressHydrationWarning={true}.
Consider reading: Azure Issue
Here are some common solutions:
- Reconfigure Logic with useEffect: This involves moving logic that depends on client-side interactions to the client-side using useEffect.
- Disable SSR on some components: Some components may differ on the client side, so disabling SSR on them can help resolve hydration errors.
- Suppress Hydration Warning: You can suppress the warning in cases where pre-rendered HTML will be different from the client version by using suppressHydrationWarning={true}.
Common Causes
Hydration errors can be frustrating to deal with, especially when you're not sure what's causing them. Dynamic content, like components that fetch data asynchronously, can introduce discrepancies between server and client rendering.
Client-side changes, like modifications made to the DOM structure by JavaScript, can conflict with server-rendered markup, leading to hydration mismatches.
Third-party libraries can also disrupt hydration, especially if they manipulate the DOM directly. This can cause the client-side rendering to fail, resulting in a mismatch between the server-rendered HTML and the client-rendered content.
Using browser APIs to conditionally render components or pages can also lead to hydration errors. This is because browser APIs like localStorage and sessionStorage are not available on the server, so pre-rendered HTML can differ from the client side.
Incorrect HTML syntax can also cause hydration errors. This can happen when using JSX in a way that's not standard, like using multiple opening tags or mismatched closing tags.
Some common scenarios where hydration errors can arise include:
- Using browser APIs to conditionally render components or pages
- Incorrect HTML syntax
- Browser extensions changing the HTML on the client side
State Management Problems
State Management Problems are a common issue in web development. This can lead to inconsistencies between the server and client.
Using browser-specific APIs or conditional rendering can cause state inconsistencies between the server and client. This can result in a range of problems, including hydration errors.
The client-side JavaScript may expect a specific state that differs from the server-rendered HTML, leading to hydration errors. This can be frustrating for users and developers alike.
Here are some specific issues that can arise from state management problems:
- Browser-specific APIs can cause state inconsistencies.
- Conditional rendering can also lead to state inconsistencies.
These problems can be difficult to diagnose and fix, but by understanding the root causes, we can take steps to prevent them.
Error Solutions
If you're experiencing issues with your Next.js application, there are several solutions to try.
Reconfigure Logic with useEffect: This solution involves using the useEffect hook to check for user authentication on the client side. This is because the server ignores useEffect blocks during rendering on the server side, and useEffect only runs on the client side with access to all browser APIs.
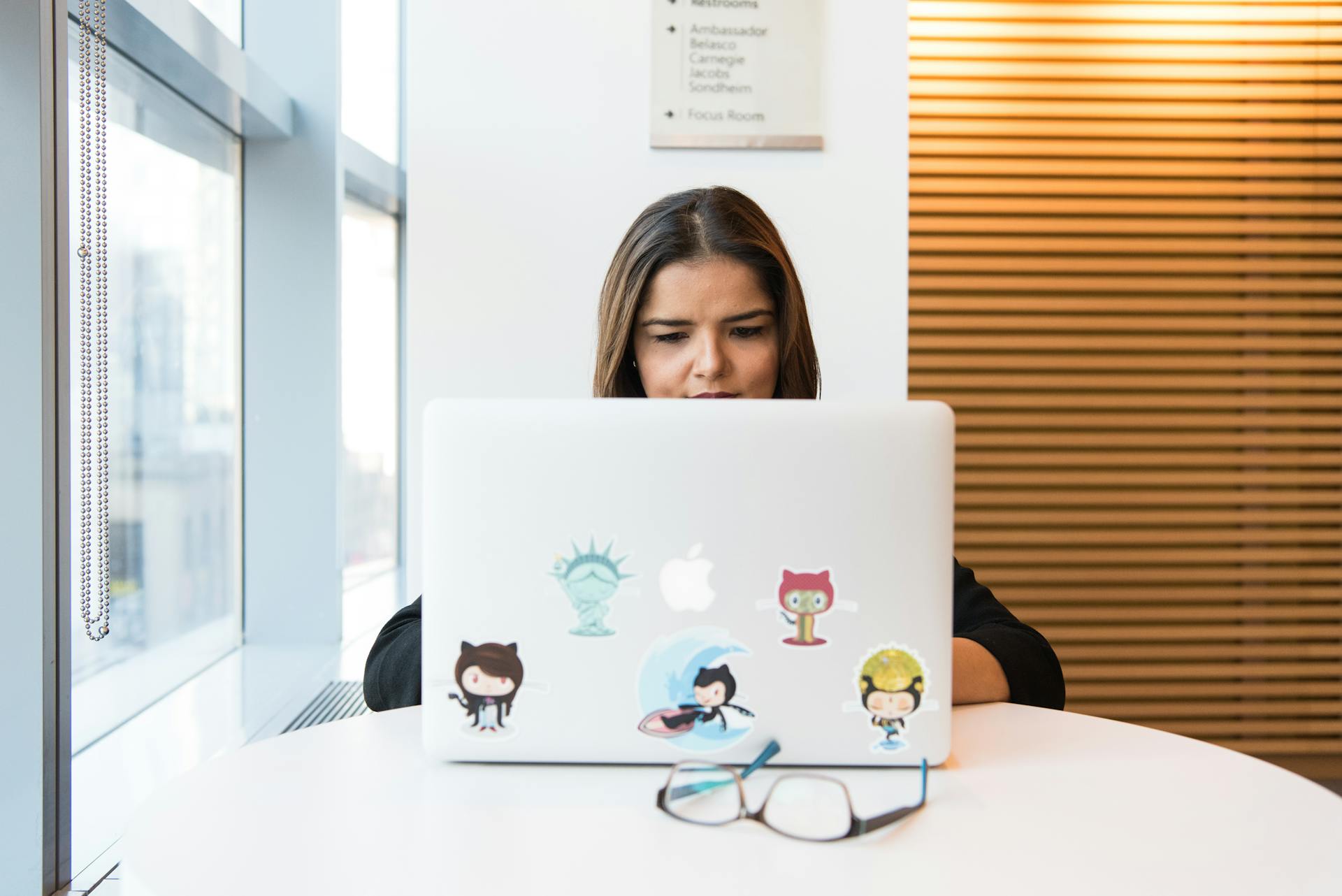
Disable SSR on some components which may differ on the client side: You can use the dynamic function from next/dynamic to disable SSR on specific components. This will prevent the server from rendering those components, allowing the client to take over instead.
Suppress the warning for timestamps: If you're working with timestamps that will be different between the server and client, you can suppress the warning using the suppressHydrationWarning prop. This will prevent the browser from complaining about the mismatch.
To fix these errors, consider using the following methods: Reconfigure Logic with useEffectDisable SSR on some componentsSuppress the warning for timestamps
Broaden your view: Nextjs Loading Page Ssr
Debugging and Testing
Debugging and testing are crucial steps in identifying and fixing hydration errors in Next.js applications. Utilizing debugging tools like React DevTools can help you inspect the component hierarchy and state during server-side rendering and client-side hydration.
React DevTools can pinpoint the causes of hydration errors for effective troubleshooting. You can use these tools to identify differences and fix issues before they reach production.
Broaden your view: Disable Hydration Nextjs
Comprehensive testing is also essential for preventing hydration errors. You can use Next.js's built-in testing tools, such as next test, to run automated tests on your application. Writing custom tests to verify that your application renders correctly on both the server and client is also a good approach.
Here are some testing approaches to consider:
- Use Next.js's built-in testing tools, such as next test.
- Write custom tests to verify server and client rendering.
- Use tools like Cypress or Jest for end-to-end testing.
Debugging Tools
Debugging Tools are essential for identifying and fixing issues in your code. Utilize debugging tools like React DevTools to inspect the component hierarchy and state during server-side rendering and client-side hydration.
These tools help you identify differences and pinpoint the causes of hydration errors for effective troubleshooting. They can make a huge difference in saving time and reducing frustration.
React DevTools, in particular, is a powerful tool that can help you visualize the component hierarchy and state, making it easier to debug complex issues.
Intriguing read: Next Js Component Rendering Analyzer
Testing for Integrity
Testing for Integrity is crucial to catch and fix errors before they reach production. You can use Next.js's built-in testing tools, such as next test, to run automated tests on your application.
Writing custom tests can help verify that your application renders correctly on both the server and client. This is especially important for catching hydration errors.
Tools like Cypress or Jest can be used to write end-to-end tests that simulate user interactions and verify that your application behaves as expected. By using these tools, you can ensure a seamless user experience.
To get started, try the following approaches:
- Use next test to run automated tests.
- Write custom tests to verify server and client rendering.
- Use Cypress or Jest for end-to-end tests.
By following these strategies, you can prevent hydration errors and ensure a smooth user experience.
Using useEffect
Using the useEffect hook is a great way to fix hydration errors in Next.js applications. It allows you to isolate client-side logic and prevent server-client mismatches.
The useEffect hook can be used to fetch data on the client-side, reducing the likelihood of hydration errors. This is especially useful when dealing with server-rendered components.
To use the useEffect hook, you can create a function that fetches data from an API and update the component's state with the response. This function should be called only once, when the component mounts.
For more insights, see: Next Js Build Collecting Page Data
Here are some common use cases for the useEffect hook:
- Fetching data from an API on the client-side
- Accessing browser-only APIs like window or localStorage
- Modifying the component after it's been hydrated
By using the useEffect hook, you can ensure that your component's state is updated correctly on the client-side, reducing the likelihood of hydration errors.
Frequently Asked Questions
How to fix next.js hydration error?
To fix a Next.js hydration error, ensure that server-side and client-side rendering produce the same content. Use the useEffect hook to intentionally render different content on the client if needed.
Sources
- https://www.geeksforgeeks.org/how-to-fix-hydration-errors-in-server-rendered-components-in-next-js/
- https://blog.logrocket.com/resolving-hydration-mismatch-errors-next-js/
- https://plainenglish.io/blog/how-to-solve-hydration-error-in-next-js
- https://nextjsstarter.com/blog/nextjs-hydration-errors-causes-fixes-tips/
- https://chirag-gupta.hashnode.dev/how-to-solve-hydration-error-in-nextjs
Featured Images: pexels.com