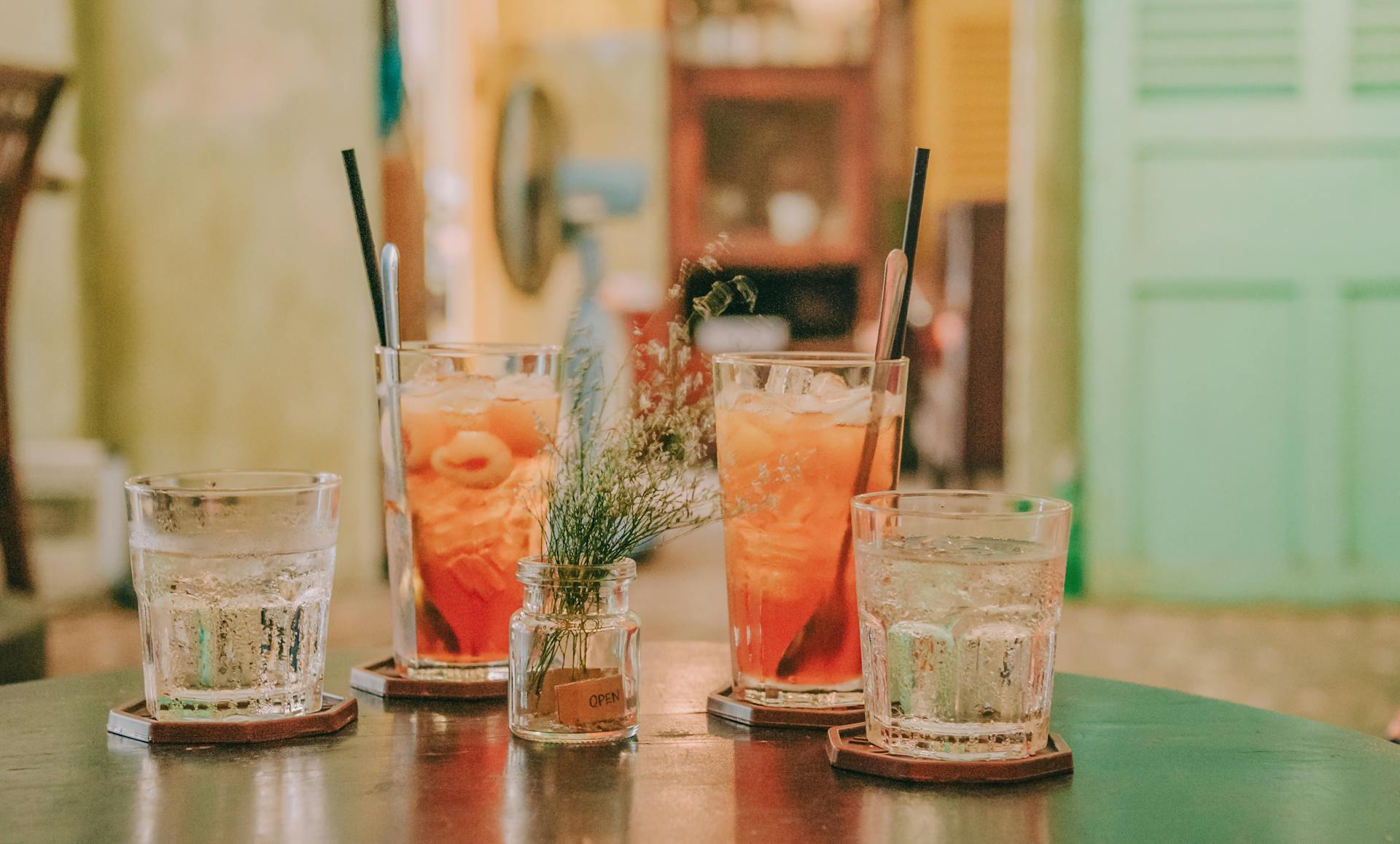
Hydration is a crucial aspect of web development, ensuring that your React application stays up-to-date with the latest changes.
It's essentially a bridge between your React application and the underlying data, keeping your app in sync with the server.
In simple terms, hydration is the process of rendering your React application on the client-side, while also linking it to the server-side data.
This allows for seamless updates and re-renders of your app's components, without requiring a full page reload.
For another approach, see: Webflow React
React Hydration
React hydration is a crucial concept in web development, allowing us to bridge the gap between server-side and client-side rendering. It enhances initial rendering speed and SEO compared to traditional single-page apps.
React hydration bridges the gap between server-side and client-side rendering by adding interactivity to static HTML generated on the server. This is done by attaching event listeners to the existing markup.
The hydrateRoot() method is called internally by Gatsby from react-dom/client, which is used to hydrate a container whose HTML contents were rendered by ReactDOMServer. React will attempt to attach event listeners to the existing markup.
Intriguing read: Hydration Issue Nextjs
To hydrate server-rendered HTML, React will attempt to attach event listeners to the existing markup and take over rendering the app on the client. This is done by using the hydrateRoot API.
A popular framework like Next.js uses React hydration under the hood, so we might not be familiar with it because it is usually pre-configured deep inside the Next.js framework. The hydrateRoot API can also be implemented within other frameworks, such as Gatsby and Astro.
The hydrate() function is used to attach a React component into a server-rendered browser DOM node. It takes three parameters: reactNode, domNode, and optional callback.
Here are some parameters that the hydrate() function takes:
- reactNode: The “React node” used to render the existing HTML.
- domNode: A DOM element that was rendered as the root element on the server.
- optional: callback: A function. If passed, React will call it after your component is hydrated.
Using hydrate() to render a client-only app is not supported. Use render() (in React 17 and below) or createRoot() (in React 18+) instead.
Hydration errors can occur when the client-side rendering differs from the server-side rendering, potentially leading to a brief flicker of server-rendered content. To address this, we can utilize useEffect to modify the component after it's been hydrated.
Another approach to mitigate hydration errors is by using suppressHydrationWarning from the react-dom package. This suppresses warnings related to elements that might cause hydration issues, such as dates.
Error Handling
Error handling is crucial in hydration, as React treats differences between server-rendered and client-rendered HTML as errors.
React will actually treat differences as errors, but the errors won’t come out in the production.
These errors can lead to a slower app, or even worse, the event handlers might get attached to the wrong elements.
You need to fix these differences, even if they don't cause immediate issues, to ensure your app runs smoothly and efficiently.
React expects the rendered contents to be identical to the server-rendered contents, so it's essential to maintain consistency between the two.
Server-Side Rendering
Server-Side Rendering is a technique used to render HTML on the server before sending it to the client. This can lead to hydration errors if the client-side rendering differs from the server-side rendering.
Next.js uses server-rendered components, which can cause a brief flicker of server-rendered content. To address this, we can utilize useEffect to modify the component after it's been hydrated, ensuring a consistent user experience.
Gatsby uses the ReactDOMClient.hydrateRoot method to hydrate a container whose HTML contents were rendered by ReactDOMServer. This method is called internally by Gatsby from react-dom/client.
Readers also liked: Text Content Does Not Match Server-rendered Html
Hydrating Server-Rendered Html
Hydrating server-rendered HTML is a crucial process in server-side rendering. It's how React attaches to existing HTML that was already rendered by React in a server environment.
During hydration, React will attempt to attach event listeners to the existing markup. This is done to take over rendering the app on the client.
In apps fully built with React, you will usually only hydrate one "root", once at startup for your entire app. This is because you shouldn't need to call hydrate again or to call it in more places.
React will be managing the DOM of your application after hydration. To update the UI, your components will use state.
Hydrating server-rendered HTML is a one-time process, and after it's done, React will handle the rest.
Static Generation
Static generation is a powerful tool that helps reduce the chances of hydration errors by pre-rendering pages with data.
Utilizing static generation, such as getStaticProps or getStaticPaths in Next.js, allows you to pre-render pages with data, making the HTML content static across server and client renders.
Related reading: Next Js Static
Sources
- https://en.wikipedia.org/wiki/Hydration_(web_development)
- https://www.gatsbyjs.com/docs/conceptual/react-hydration/
- https://blog.logrocket.com/react-hydration-pre-rendered-html/
- https://react.dev/reference/react-dom/hydrate
- https://www.geeksforgeeks.org/how-to-fix-hydration-errors-in-server-rendered-components-in-next-js/
Featured Images: pexels.com