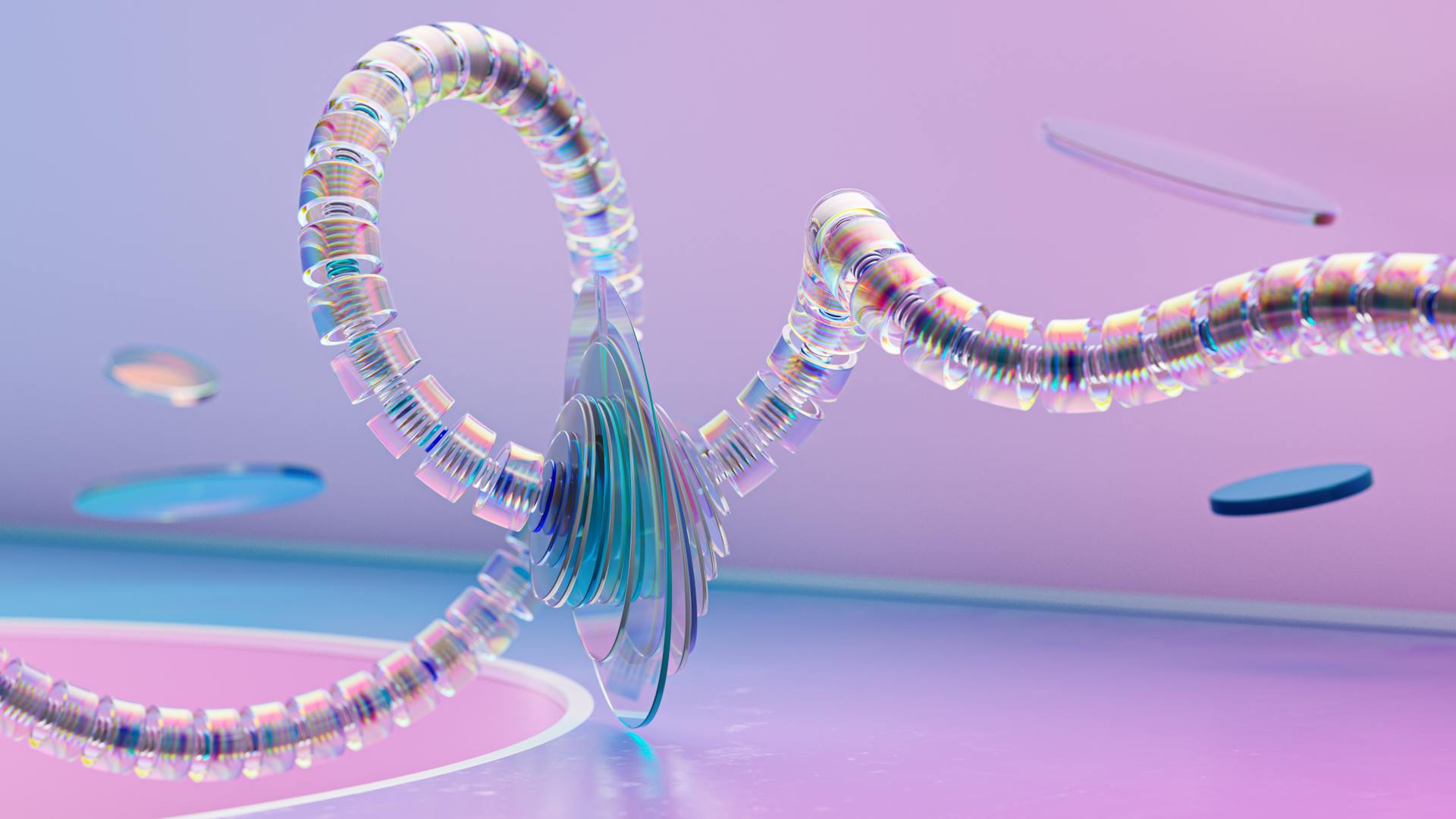
To set up a private S3 bucket with Django, you'll need to use AWS IAM policies to control access to the bucket.
A Django project can be integrated with AWS IAM to restrict access to the S3 bucket.
To start, create an AWS IAM policy that grants the necessary permissions to the bucket. This policy should include actions like "s3:GetObject" and "s3:PutObject" to allow authorized users to read and write files.
You can then attach this policy to an IAM user or group, and use Django's built-in authentication system to authenticate users before granting them access to the bucket.
Discover more: Use an S3 Bucket for a Website
Installation and Setup
To install a private S3 bucket with Django, you'll need to install the boto3 library, which is the backend's foundation. The minimum required version is 1.4.4, but we always recommend using the most recent version.
Add boto3 to your project's requirements or use the optional s3 extra, for example, to ensure a smooth installation process.
A unique perspective: Aws Python S3
Create S3 Bucket
To create an S3 bucket on AWS, you'll need to log in to the AWS Management Console and change your region to where you want your bucket to reside.
First, log in to the AWS Console. This will give you access to your account and allow you to start creating your S3 bucket.
Next, change your AWS region to where you want your bucket to reside. This is an important step, as it will determine the location of your bucket.
To proceed, you'll need to create a bucket policy. This will define the rules and permissions for your S3 bucket.
You might enjoy: S3 Console Aws
Amazon File Storage
Amazon File Storage is a great way to store files for your project. You can use Amazon S3, which is a cloud-based storage service.
To use Amazon S3 with Django, you'll need to replace the prefix AWS_ with AWS_PRIVATE_ in your django-storages settings. This includes settings like AWS_ACCESS_KEY_ID, AWS_SECRET_ACCESS_KEY, and AWS_S3_URL_PROTOCOL.
A different take: Google Storage Bucket
Some settings can be reused without an AWS_PRIVATE_... counterpart, including AWS_ACCESS_KEY_ID, AWS_SECRET_ACCESS_KEY, AWS_S3_URL_PROTOCOL, AWS_S3_REGION_NAME, and AWS_IS_GZIPPED. However, all other settings should be explicitly defined with AWS_PRIVATE_... settings.
By default, all URLs in the admin return the direct S3 bucket URLs, with query parameter authentication enabled. If you want to proxy file downloads through a PrivateFileView URL, you can set AWS_PRIVATE_QUERYSTRING_AUTH to False.
Here are some settings you'll need to define explicitly with AWS_PRIVATE_...:
- AWS_PRIVATE_S3_BUCKET_NAME
- AWS_PRIVATE_S3_BUCKET_ACL
- AWS_PRIVATE_S3_ENCRYPTION
- AWS_PRIVATE_S3_SIGNATURE_VERSION
To enable encryption, you can configure AWS_PRIVATE_S3_ENCRYPTION and AWS_PRIVATE_S3_SIGNATURE_VERSION, or generate an encryption key on Amazon.
Intriguing read: Aws S3 Encryption
MinIO Storage
To set up MinIO storage, you'll need to define the necessary settings. This includes replacing the prefix MINIO_ with MINIO_PRIVATE_ in the django-minio-storage settings.
MINIO_STORAGE_ENDPOINT and MINIO_STORAGE_ACCESS_KEY are required settings for MinIO storage. MINIO_STORAGE_SECRET_KEY, MINIO_STORAGE_USE_HTTPS, and MINIO_STORAGE_MEDIA_BUCKET_NAME are also important.
You can reuse the all settings when they don't have a corresponding MINIO_PRIVATE_... setting. This can save you some time when setting up MinIO storage.
Here are the required settings you'll need to define:
- MINIO_PRIVATE_STORAGE_ENDPOINT
- MINIO_PRIVATE_STORAGE_ACCESS_KEY
- MINIO_PRIVATE_STORAGE_SECRET_KEY
- MINIO_PRIVATE_STORAGE_USE_HTTPS
- MINIO_PRIVATE_STORAGE_MEDIA_BUCKET_NAME
- MINIO_PRIVATE_STORAGE_MEDIA_URL
- MINIO_PRIVATE_STORAGE_AUTO_CREATE_MEDIA_BUCKET
- MINIO_PRIVATE_STORAGE_AUTO_CREATE_MEDIA_POLICY
- MINIO_PRIVATE_STORAGE_MEDIA_USE_PRESIGNED
- MINIO_PRIVATE_STORAGE_MEDIA_BACKUP_FORMAT
- MINIO_PRIVATE_STORAGE_ASSUME_MEDIA_BUCKET_EXISTS
- MINIO_PRIVATE_STORAGE_MEDIA_OBJECT_METADATA
If you want to enable proxy through our PrivateFileView URL, simply specify PRIVATE_STORAGE_MINO_REVERSE_PROXY = True.
Configuration and Security
To configure and secure your private S3 bucket with Django, you need to define access rules for your files. This is done using the PRIVATE_STORAGE_AUTH_FUNCTION, which by default only includes superusers.
You can create a custom function to allow other users to access your files. The function receives a PrivateFile object, which has several fields, including the request, storage engine, and file name.
To allow authenticated users to access your files, you can add private_storage.permissions.allow_authenticated to your custom function. You can also allow staff users by adding private_storage.permissions.allow_staff.
Here's a list of the default permissions:
- private_storage.permissions.allow_authenticated
- private_storage.permissions.allow_staff
- private_storage.permissions.allow_superuser
To set the bucket policy, go to your S3 console and select your bucket. Click on the "Add bucket policy" link and enter the policy as follows:
- Replace the 12345678901 with your AWS Account Number, which can be found on the AWS Manage Your Account page.
- Set the Resource to your bucket name and path to the folder where your private files are stored.
- The asterisk on the end of the path means the policy applies to all files in that folder.
Making Files Private
Making files private is a crucial aspect of securing your S3 bucket. You can make files private by using a custom field class for private files, which sets the default_acl parameter.
This approach eliminates the need to manually set the acl in the save method. For example, you can use the private_file field on your model instead of the FileField().
To make a file private, you should no longer be able to access it directly. Visiting the URL of the file should return an XML file containing an AccessDenied error.
A new field class can be created to use for private files, and you can then use this for the private_file on your model. This new field class automatically sets the default_acl parameter.
Here are the reusable settings when using django-storages:
- AWS_ACCESS_KEY_ID
- AWS_SECRET_ACCESS_KEY
- AWS_S3_URL_PROTOCOL
- AWS_S3_REGION_NAME
- AWS_IS_GZIPPED
All other settings should be explicitly defined with AWS_PRIVATE_... settings.
Settings
To configure multiple storage objects in Django >= 4.2, you pass them under the key OPTIONS.
You can save media files to S3 by defining the storage backend under the key OPTIONS, like this: "default": {"BACKEND": "django.core.files.storage.S3Boto3Storage", "OPTIONS": {"bucket_name": "your-bucket-name"}}, "default": {"BACKEND": "django.core.files.storage.S3Boto3Storage", "AWS_ACCESS_KEY_ID": "your-access-key-id", "AWS_SECRET_ACCESS_KEY": "your-secret-access-key"}.
Take a look at this: A Private Key Is Important
Collectstatic on Django >= 4.2 requires defining the staticfiles key in the STORAGES dictionary, like this: "default": {"BACKEND": "django.core.files.storage.S3Boto3Storage", "OPTIONS": {"bucket_name": "your-bucket-name"}}, "staticfiles": {"BACKEND": "django.core.files.storage.S3Boto3Storage", "OPTIONS": {"bucket_name": "your-bucket-name"}}.
In Django >= 4.2, you can configure additional storage backends by adding them to the STORAGES dictionary, like this: "default": {"BACKEND": "django.core.files.storage.S3Boto3Storage", "OPTIONS": {"bucket_name": "your-bucket-name"}}, "extra_storage": {"BACKEND": "django.core.files.storage.S3Boto3Storage", "OPTIONS": {"bucket_name": "your-extra-bucket-name"}}.
The key AWS_CLOUDFRONT_KEY is used to configure cloudfront for your S3 bucket.
Additional reading: S3 Bucket Key
Frequently Asked Questions
How to integrate S3 bucket in django?
To integrate an S3 bucket in Django, start by creating an Amazon S3 bucket in the AWS Management Console and then configure your Django settings to use S3 storage. Follow the steps outlined in the official documentation to complete the integration process.
Sources
- https://django-storages.readthedocs.io/en/latest/backends/amazon-S3.html
- https://www.gyford.com/phil/writing/2012/09/26/django-s3-temporary/
- https://medium.com/@anupamkush9/storing-django-static-and-media-files-on-amazon-s3-private-bucket-and-serve-it-through-cloudfront-7e5906735656
- https://github.com/edoburu/django-private-storage
- https://awstip.com/storing-media-and-static-files-in-an-amazon-s3-bucket-with-django-a42db08736f2
Featured Images: pexels.com