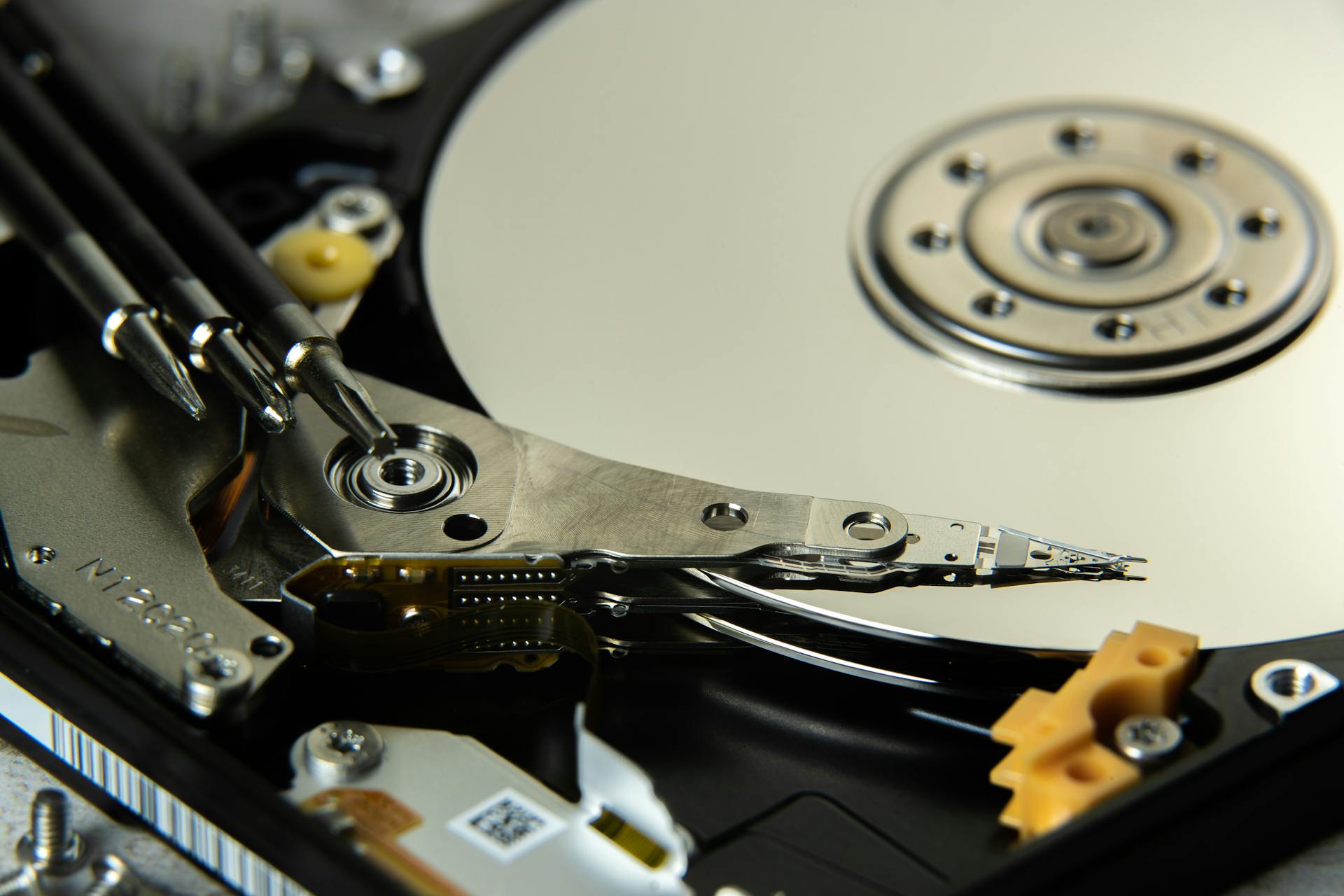
Processing large S3 files with AWS Lambda requires careful planning to ensure scalability and performance.
AWS Lambda functions can handle files up to 50 MB in size, but for larger files, you'll need to use AWS Lambda's streaming feature.
To process large S3 files, you can use AWS Lambda's event-driven architecture, which allows you to trigger a function on S3 object creation or update.
This approach enables you to process files in chunks, reducing memory usage and improving performance.
AWS Lambda Configuration
When creating an AWS Lambda function, you can choose to author a new function from scratch and use Python 3.9 as your runtime. Let's create a new function and add a trigger to it, choosing our S3 bucket.
The handler is the method that's invoked when the Lambda function is triggered, and it's the value of the handler that's the file name and the name of the handler module, separated by a dot.
To configure your Lambda function, you'll want to pay attention to the Runtime settings at the bottom of the page, where you can see the assigned Handler.
Our architecture employs three tailored Lambda functions for processing files of varying sizes, ensuring both efficiency and cost-effectiveness in our cloud operations.
Here's a closer look at how these functions are structured:
Each Lambda function is precisely triggered by multipart upload events matching their file size categories, and they're configured with the maximum allowable timeout to ensure successful execution of tasks within operational bounds.
By thoughtfully mapping Lambda functions to file sizes, we achieve an optimal balance that supports scalability, cost control, and effective processing across all types of file sizes.
File Processing Strategies
You can process large S3 files with AWS Lambda by downloading the entire file into RAM using Python's BytesIO object, which is a convenient and easy-to-use solution. This approach simplifies coding and avoids potential issues with temporary disk space.
Working with the response from an S3 GET request as a stream of bytes is another option, but be aware that the SDK docs caution against this approach in multi-threaded environments.
Using byte-range retrieves from S3 with a relatively small buffer size is not a recommended solution, as it requires handling cases where data records span retrieved byte ranges.
You can process large files line by line with AWS Lambda by using the StreamingBody returned by get_object, which provides options like reading data in chunks or reading data line by line. This approach avoids reading the complete data at once, which is a major advantage.
The iter_lines and iter_chunks methods of StreamingBody are not recommended for reading data, as they read the complete object by default. Instead, use the iter_lines method to yield lines from the raw stream, reading chunk of bytes at a time.
Handling Large Files
Lambda provides 512 MB of temporary disk space, but it's not recommended to store files on attached disk as it may run out of space due to repeated invocations of the same Lambda environment.
You can download the entire file into RAM and work with it there using Python's BytesIO object, which behaves identically to on-disk files. This simplifies coding but may still have an issue with very large files, requiring you to configure your Lambda with enough memory to hold the entire file.
Alternatively, you can work with the response from an S3 GET request as a stream of bytes, but be aware that the SDK for your language might not expose this stream in a way that's consistent with standard file-based IO. Boto3, the Python SDK, is one of the offenders, returning a StreamingBody that has its own methods for retrieving data and does not follow Python's io library conventions.
Here are some alternatives to handling large files:
- Download the entire file into RAM using BytesIO.
- Work with the response from an S3 GET request as a stream of bytes.
- Use byte-range retrieves from S3, using a relatively small buffer size.
Multi-Part Uploads
Handling large files can be a challenge, especially when dealing with multi-part uploads. These types of uploads are designed to break down large files into smaller chunks, making them easier to transmit.
Uploading a 5 GB file in one go can be a daunting task, especially if your internet connection is slow. In such cases, breaking down the file into smaller chunks of 2 GB each can significantly improve the upload speed.
Some file transfer protocols, like FTP, support multi-part uploads. This allows you to upload a large file by sending multiple smaller chunks, rather than the entire file at once.
Uploading a 10 GB file in 5 chunks can take significantly less time than uploading the entire file at once. This is because the upload speed is not affected by the size of the file, but rather by the speed at which the chunks are transmitted.
Using a tool that supports multi-part uploads, like curl, can make the process much easier. These tools can automatically break down the file into smaller chunks and handle the upload process for you.
Uploading a large file in chunks can also help prevent errors and timeouts. If one chunk fails to upload, the entire process doesn't come to a halt, allowing you to simply re-upload the failed chunk.
Large File Issues
Large files can be a challenge when working with AWS Lambda. Lambda provides 512 MB of temporary disk space, but it's not enough for large files and you need to be careful to clean up temporary files to avoid running out of space.
One potential solution is to download the entire file into RAM and work with it there. Python's BytesIO object makes this easy, but you'll need to configure your Lambda with enough memory to hold the entire file, which may increase your per-invocation cost.
Alternatively, you can work with the response from an S3 GET request as a stream of bytes. This is a good option for large files, but be aware that some SDKs, like Boto3, may not expose this stream in a way that's consistent with standard file-based IO.
If the file's too big to be transformed by a Lambda, you may need to look at other AWS services. AWS Batch is a good option for running long-running tasks, as it allows you to create a Docker image that packages your application with any necessary third-party libraries.
Here are some key considerations when working with large files in Lambda:
- Be aware of the 512 MB temporary disk space limit
- Consider downloading files into RAM using BytesIO
- Use S3 GET requests as a stream of bytes
- Consider using AWS Batch for long-running tasks
In the worst-case scenario, you may need to use byte-range retrieves from S3, but this can be cumbersome and may require handling edge cases where data records span retrieved byte ranges.
API Gateway and Security
Using AWS API Gateway to process large S3 files with AWS Lambda requires careful consideration of security. This method can bypass limitations of API Gateway for large file uploads, offering a scalable solution directly from client applications.
By utilizing S3 presigned URLs, you can simplify the process and keep your data secure during transit. This is a significant advantage, especially when dealing with sensitive information.
S3 presigned URLs provide a secure way to upload files, and API Gateway can help manage the process efficiently.
API Gateway Presigned URLs
API Gateway has a file upload limit of 10 MB, but you can bypass this limit by using S3 presigned URLs. This approach allows direct uploads to S3, which can handle larger file sizes up to 5 TB with multipart uploads.
To work around this limit, you can return a S3 presigned URL in the API Gateway response, allowing direct uploads to S3. This involves two main steps: fetching the presigned URL from S3 via an API Gateway to Lambda setup and using the obtained URL to upload the file directly from the client.
Using presigned URLs is a scalable and secure solution directly from client applications. This method simplifies the process and keeps your data secure during transit.
To generate a presigned URL, you can use the S3 SDK, which makes it easy to create a pre-signed URL for a PUT request. You'll need to provide the expected content type, such as text/plain or image/jpeg.
Here's a step-by-step process to generate a presigned URL:
- Fetch the presigned URL from S3 via an API Gateway to Lambda setup
- Use the obtained URL to upload the file directly from the client
- Provide the expected content type when generating the presigned URL
IAM Permissions
The principle of least privilege is key when it comes to IAM permissions for a Lambda function. This principle says that the function should only be allowed to perform the tasks it needs to, and nothing more.
To manage these permissions, I personally prefer using inline policies in the Lambda's execution role. This allows me to tailor the permissions to the specific application using the role.
However, as the example shows, a real-world Lambda will often require additional privileges to do its work, which can lead to hitting IAM's 10kb limit for inline policies. If that's the case, managed policies might be a better solution.
I would still target managed policies at a single application to keep things organized and easy to manage.
CORS Configuration
CORS Configuration is a crucial aspect of API Gateway and Security.
One common issue during implementation is CORS errors, which can be resolved by configuring CORS in your S3 bucket settings.
To configure CORS, you can follow the steps outlined in the S3 documentation, which involves adding a CORS configuration to your bucket's permissions.
This configuration typically includes elements such as allowed origins, methods, and headers, which need to be specified in the CORS configuration.
For example, you might allow GET and POST requests from a specific origin, while blocking PUT and DELETE requests from others.
In some cases, you may need to configure CORS at the API Gateway level, which can be done using the API Gateway console or via the AWS CLI.
Remember to test your CORS configuration thoroughly to ensure that it's working as expected and not causing any issues with your API.
Sources
- https://chariotsolutions.com/blog/post/two-buckets-and-a-lambda-a-pattern-for-file-processing/
- https://alexanderhose.com/how-to-efficiently-transform-s3-objects-with-a-simple-lambda-function/
- https://builtin.com/articles/presigned-url-s3-api-gateway-upload-file
- https://articles.wesionary.team/mastering-large-file-processing-with-aws-s3-lambda-and-go-3dde0a4c29c6
- https://medium.com/analytics-vidhya/demystifying-aws-lambda-deal-with-large-files-stored-on-s3-using-python-and-boto3-6078d0e2b9df
Featured Images: pexels.com