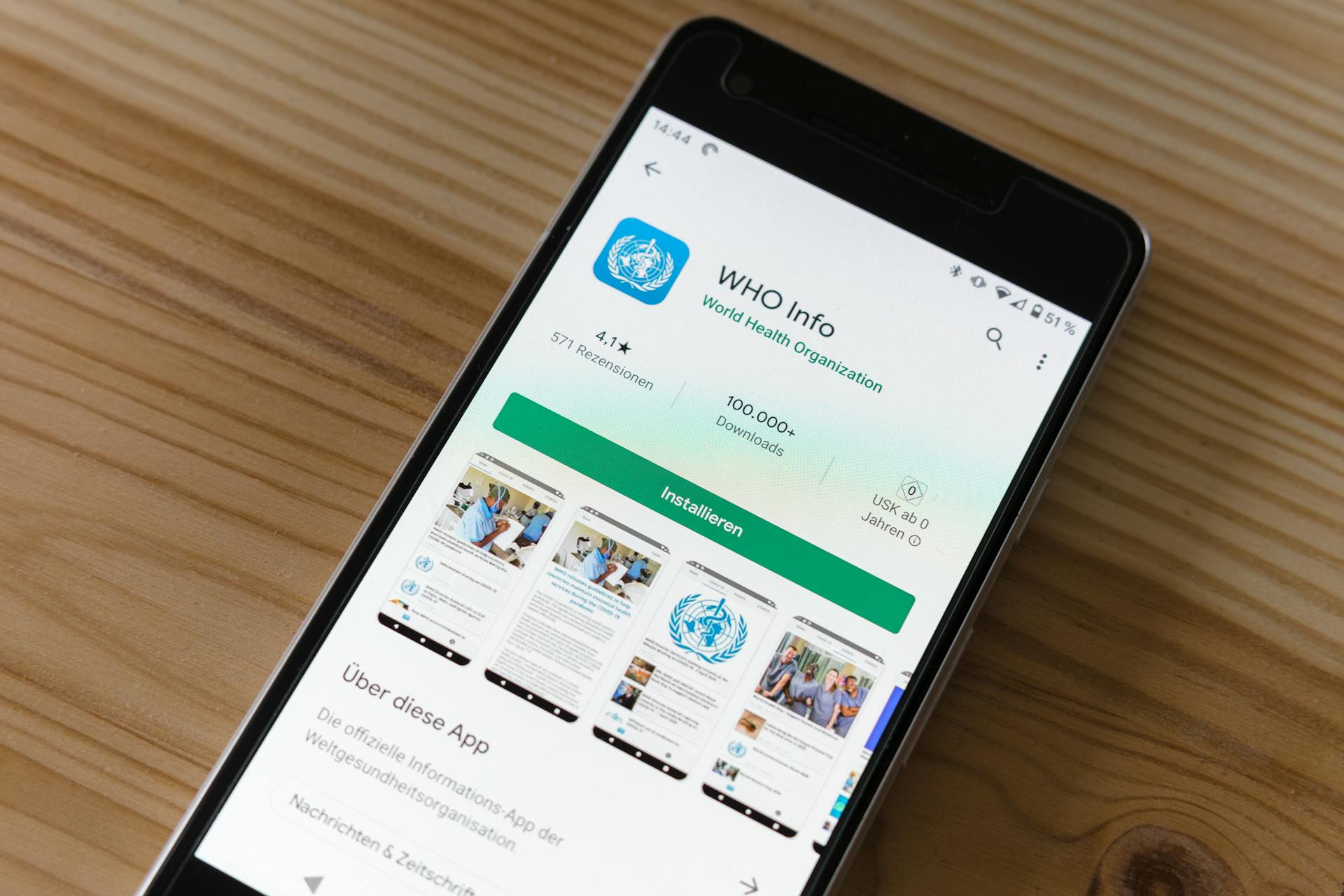
React Native Devtools is a powerful tool that helps you debug and optimize your React Native apps. It's like having a superpower that allows you to see what's going on behind the scenes.
To start debugging with React Native Devtools, you need to open the Chrome DevTools by going to chrome://inspect/#devices. This will allow you to inspect your React Native app on a device or emulator.
React Native Devtools has a Profiler that helps you identify performance bottlenecks in your app. The Profiler shows you a timeline of your app's performance, highlighting areas where your app is slow or consuming too much memory.
You can also use the React Native Devtools to inspect the props and state of your components. This is especially helpful when trying to debug issues with your app's UI.
For another approach, see: Create React App vs Next Js
Installation
Installing React Native Debugger is a straightforward process. To get started, you can use Homebrew on MacOS to install it with a single command.
On MacOS, you can install Homebrew and then use it to install the React Native Debugger app in your /applications folder by running a specific command.
If you don't have Homebrew installed on your Mac, you can also download the app directly from the releases page.
For Windows users, there's a simpler option: you can download the .exe installer from the releases page and get started right away.
Consider reading: React Js Deploy Nginx in Azure Web App
Usage and Configuration
If you're using React Native, you'll need to forward ports used by React DevTools if you're not using a local simulator. This will allow you to connect to your simulator within a few seconds.
If you're using React Native 0.43 or higher, you should be able to connect automatically, but if not, try reloading the React Native app.
Usage
If you're using React Native, you'll need to forward ports used by React DevTools if you're not using a local simulator. This will allow your app to connect to your simulator within a few seconds, which is the case for React Native 0.43 or higher.
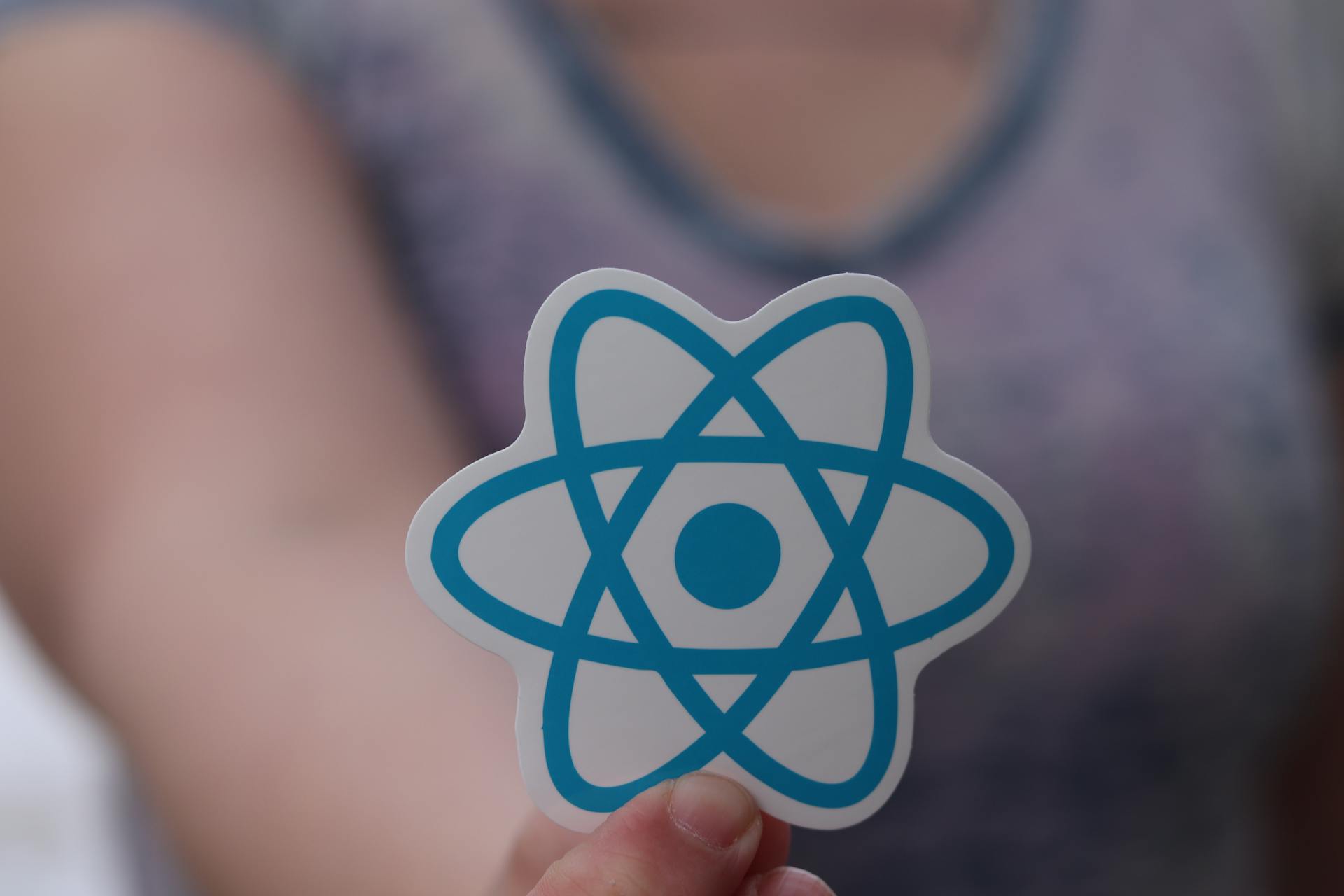
To use a custom JavaScript debugger in place of Chrome Developer Tools, you'll need to set the REACT_DEBUGGER environment variable to a command that will start your custom debugger. This command should be short-lived and produce no more than 200 kilobytes of output.
You can speed up your development times by enabling automatic reloading, which can be done by selecting "Enable Live Reload" from the Developer Menu. Hot reloading can also be enabled from the Developer Menu, allowing you to persist your app's state through reloads.
Here's a list of tools that support automatic reloading:
- Chrome debugger: Android and iOS
- Flipper (Hermes debugger): Android
- React Developer Tools: Android and iOS
If you're using Create React Native App or Expo CLI, automatic reloading is configured for you already. However, there are some instances where hot reloading cannot be implemented perfectly, such as when you've added new resources to your native app's bundle or modified native code.
Running Debugger
To run the React Native Debugger, you'll need to install it on your MacOS using Homebrew by running the command `brew install react-native-debugger`. You can also download it directly from the releases page if you don't have Homebrew installed.
By default, the app will listen to port 8081, but this might not be the port used by your app's bundler. On MacOS, you can change the port number by running the command `react-native-debugger --port 19001`. Alternatively, you can change the port within the app itself by opening a new tab with cmd + t and setting the port before opening the dev tools.
To enable remote JS debugging, you'll need to access the developer menu on your device or simulator. On Android, this involves enabling developer options by tapping "About Device" or "About Phone" in the Settings app, then tapping the build number 7 times to reveal the "Developer options" menu.
The React Native Debugger is a simple tool that creates a web worker inside your preferred browser to execute your app's JavaScript code. It works with all the runtimes available in React Native, including JSC, Hermes, and V8.
Intriguing read: Change Devtools Language Chrome
Debugging Tools
You can use Chrome DevTools to debug your React Native app, but it's not available for JSC. On Android 5.0+ devices connected via USB, you can use the adb command line tool to setup port forwarding from the device to your computer.
For iOS devices, open the file RCTWebSocketExecutor.m and change "localhost" to the IP address of your computer, then select "Debug JS Remotely" from the Developer Menu.
Chrome DevTools works with all the runtimes available in React Native, except for JSC.
Here are some popular debugging tools for React Native:
- Chrome Debugger: a simple tool that creates a web worker inside your preferred browser which executes your app's JavaScript code.
- Flipper (Hermes debugger): an app created by Facebook that makes it easy to use the Chrome DevTools while providing additional tools for UI inspection and debugging.
- Safari DevTools: Safari's built-in feature that is only available for iOS devices running JSC.
- React Developer Tools: a standalone app that allows debugging UI through the inspector, as well as monitoring performance and profiling your app.
React Native Debugger is available for MacOS, and can be installed using Homebrew or by downloading it directly from the releases page.
Integration with Inspector
You can open the in-app developer menu and choose "Show Inspector" to see information about your UI elements. This will bring up an overlay that lets you tap on any element and view its details.
In this mode, you can tap on something in the simulator to bring up the relevant components in the DevTools. This is a great way to quickly inspect your app's UI and debug any issues.
However, when react-devtools is running, Inspector will enter a special collapsed mode, and instead use the DevTools as primary UI. This means you'll be using the DevTools to view and interact with your app's UI.
You can choose "Hide Inspector" in the same menu to exit this mode, or "Toggle Inspector" to switch between the two modes.
Performance Monitor
The Performance Monitor is a powerful tool in React Native DevTools. It allows you to enable a performance overlay to help you debug performance problems by selecting "Perf Monitor" in the Developer Menu.
To get started, you can access the Performance Monitor by selecting the "Perf Monitor" option from the Developer Menu. This will display a performance overlay that provides valuable insights into your app's performance.
The performance overlay gives you a real-time view of your app's performance, including CPU usage, memory usage, and frame rate. This information can help you identify performance bottlenecks and optimize your app accordingly.
By using the Performance Monitor, you can identify and fix performance issues before they impact your users. This can lead to a better overall user experience and improved app performance.
Frequently Asked Questions
How do I open the dev menu in React Native?
To access the Dev Menu in React Native, shake your device or use keyboard shortcuts: Cmd ⌘ + D (iOS Simulator), Cmd ⌘ + M (macOS), or Ctrl + M (Windows and Linux). This menu offers various debugging options to streamline your development process.
Featured Images: pexels.com