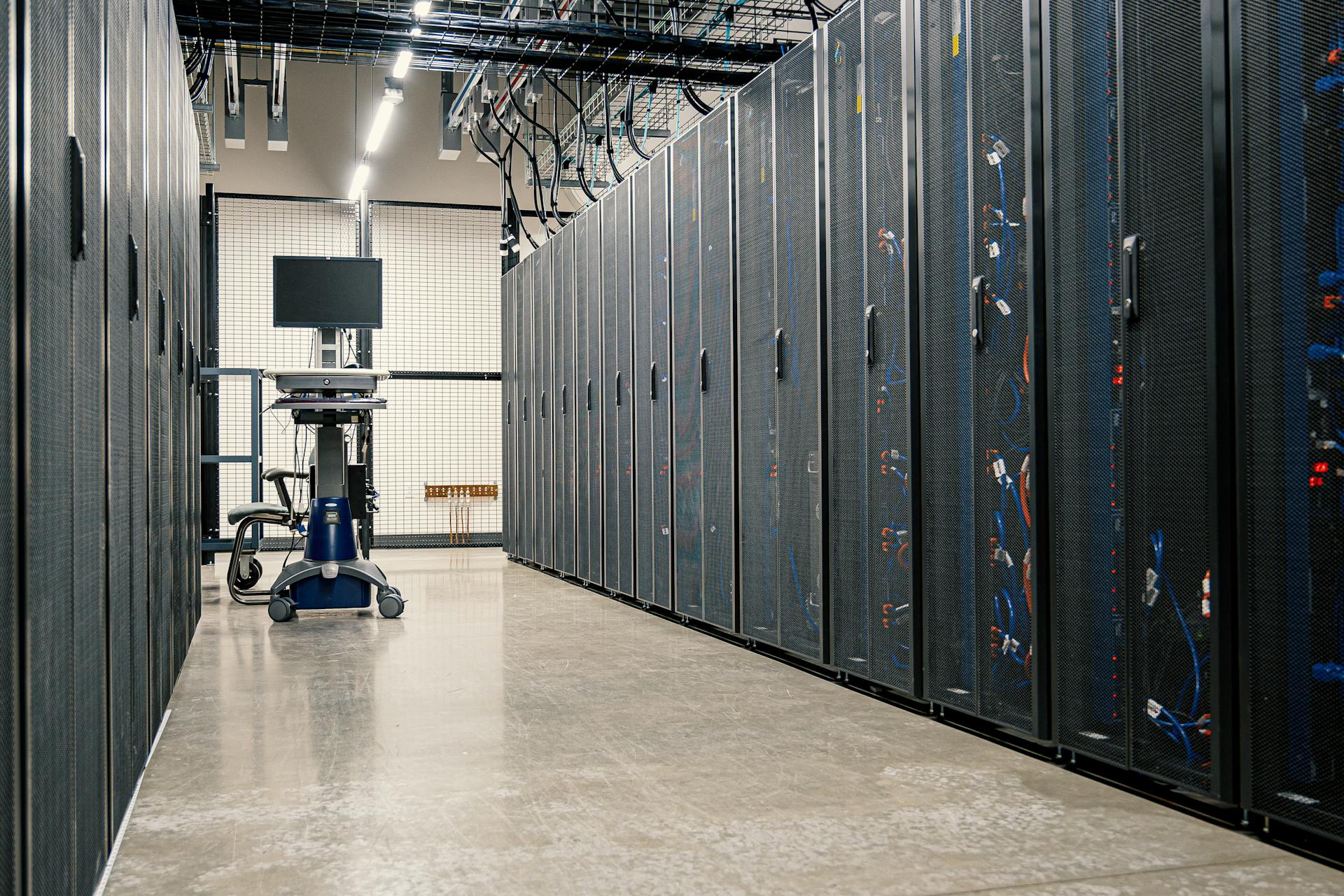
To deploy a React JS application with Nginx in Azure Web App using Docker and Azure App Service, you'll need to create a Dockerfile that specifies the base image for your application. This Dockerfile is where you'll define the build process for your application.
You'll also need to create a Docker Compose file that defines the services and their configuration for your application. This file will help you manage the dependencies and configuration of your application.
Once you have your Dockerfile and Docker Compose file set up, you can use the Azure CLI to create a new Azure App Service and deploy your application to it. This will allow you to take advantage of Azure's scalability and reliability features.
To configure Nginx in your Azure App Service, you'll need to create a configuration file that defines the server block and the location block for your application. This file will specify the server's address, the server's port, and the location of your application's static files.
On a similar theme: How to Make a Website Hosting Server
Project Setup
To set up your project, start by creating a file named web.config at the root of your project. This is required if you have different routes in your application.
Create an Azure account if you don't already have one, and use the link https://azure.microsoft.com/en-in/free/ to do so.
Create a new resource group by navigating to the Azure portal and following these steps: Create a new resource group: codingdeft-react-demo_groupName as codingdeft-react-demoRun time stack: Node 16 LTS (or any latest version)Operating system: WindowsRegion: East US (or leave as default)
Once your project is created, click on Repos on the left to commit your code from the command prompt. Navigate to your project directory and run the following command to add an npm install step.
Expand your knowledge: How to Run Next Js App
Dockerize Your App
To Dockerize Your App, you'll need to install Docker Desktop to manage Docker assets conveniently. This will make it easier to work with Docker.
Create a Dockerfile in the root directory of your project. A Dockerfile is a text file that contains instructions for building a Docker image.
Here's an interesting read: Docker Compose Azure Container Apps
You'll want to utilize a multi-stage build process in your Dockerfile. This involves two stages: building your app and serving it.
In the first stage, you'll typically use a node-based image to build your app. For example, you might generate output files in the /build folder.
Here's a simplified version of what your Dockerfile might look like:
- From node:14
- WORKDIR /app
- COPY package*.json .
- RUN npm install
- BUILD
- FROM nginx:alpine
- COPY --from=0 /build /usr/share/nginx/html
- EXPOSE 80
ACR and App Service Overview
Your Azure App Service deployment settings are managed through the Deployment Center in the Azure portal.
The Deployment Center is conveniently located in the left panel of the Azure portal, making it easy to access and configure.
Within the Deployment Center, you'll find detailed configuration options, including information about your container registry.
A Webhook URL is a crucial component of the deployment process, serving as a communication mechanism between your container registry and Azure App Service.
Every time a new image is pushed to your container registry, the webhook is triggered, notifying your Azure App Service of any updates to your application's Docker image.
This automated process streamlines the deployment workflow, providing seamless integration between your container registry and Azure App Service.
On a similar theme: Azure App Center
Application Routing
When deploying a React JS app with NGINX in Azure Web App, you'll want to consider the application routing add-on with NGINX features. This add-on delivers easy configuration of managed NGINX Ingress controllers based on Kubernetes NGINX Ingress controller.
You'll need to configure DNS and SSL settings, which can be integrated with Azure DNS for public and private zone management. This allows for secure communication between your app and users.
The application routing add-on also supports SSL termination with certificates stored in Azure Key Vault. This ensures that your app remains secure and trustworthy.
To get started, you'll need to configure the application routing add-on, which involves DNS and SSL configuration. You'll also need to configure the internal NGINX ingress controller for Azure private DNS zone.
Here are the key steps to follow:
- DNS and SSL configuration
- Application routing add-on configuration
- Configure internal NGIX ingress controller for Azure private DNS zone.
Note that using the application routing add-on with Open Service Mesh (OSM) is not recommended due to the retirement of OSM by the Cloud Native Computing Foundation (CNCF).
Deployment
To finalize your deployment, follow these steps to set up your application on Azure App Service.
Access the Azure Portal by going to portal.azure.com and searching for "App Service." Create a new App Service by providing subscription and resource group details, naming your App Service, choosing "Docker Container" under "Publish", and specifying the runtime stack and region.
Configure Docker settings by selecting "Single Container" under the "Docker" tab, setting the image source to "ACR" (Azure Container Registry), choosing your registry from Step 5, and setting the image name as "react-demo" with tag "latest."
Review and create your App Service by skipping other optional steps, clicking "Review + Create" to ensure accuracy, and hitting "Create" to initiate setup.
Pushing Your Image to Azure Container Registry
To deploy your React application, you need to push your Docker image to Azure Container Registry (ACR). There are three options to accomplish this: Build Image in Azure Portal, Using Azure CLI, and Azure DevOps Pipeline.
Intriguing read: Deploy Docker Image on Azure
The Build Image in Azure Portal option is straightforward - navigate to your source code, locate the Dockerfile, and click on it to build the image and push it to ACR directly.
Using Azure CLI offers partial automation, allowing you to build and push the image with some manual effort.
Azure DevOps Pipeline provides full automation, requiring you to create a service connection, configure the pipeline, and let it build and push the image to ACR automatically.
Expand your knowledge: How to Build Next Js App for Production
Deploying Your Application
To deploy your application on Azure App Service, start by accessing the Azure Portal at portal.azure.com and searching for "App Service".
Create a new service by providing subscription and resource group details, naming your App Service, and choosing "Docker Container" under "Publish".
When specifying the runtime stack, choose from options like Node 18 or Node 20, and select your preferred region. Fill in the mandatory fields based on your preferences.
Under the "Docker" tab, select "Single Container" and set the image source to "ACR" (Azure Container Registry). Choose your registry from the previous step and set the image name as "react-demo" with tag "latest".
Review and create your App Service by clicking "Review + Create" and ensuring accuracy, then hit "Create" to initiate setup.
Deployment may take some time, so be patient and wait for it to complete. Once done, click "Go to resource" to access your newly deployed application.
You can find a temporary domain URL in the overview section, which will serve as your application's URL until you configure a custom domain.
Here are the steps to deploy your application on Azure App Service:
- Access Azure Portal at portal.azure.com and search for "App Service".
- Create a new service by providing subscription and resource group details, naming your App Service, and choosing "Docker Container" under "Publish".
- Under the "Docker" tab, select "Single Container" and set the image source to "ACR" (Azure Container Registry).
- Review and create your App Service by clicking "Review + Create" and ensuring accuracy, then hit "Create" to initiate setup.
Frequently Asked Questions
Does Azure Web App use Nginx?
Azure Web App uses Nginx as its default web server when running PHP 8. Note that this may change depending on the PHP version used.
Why use nginx with React?
Using Nginx with React provides benefits like reverse proxy, caching, and security, resulting in high-performance applications. It's a powerful combination that can enhance your React app's speed and reliability.
Sources
- https://www.codingdeft.com/posts/react-deploy-microsoft-azure/
- https://nextjs.org/docs/pages/building-your-application/deploying
- https://learn.microsoft.com/en-us/azure/aks/app-routing
- https://medium.com/@yogender.singh.wd/step-by-step-guide-for-deploying-a-react-application-on-azure-app-service-docker-azure-container-a3ad2f79bdb1
- https://stackoverflow.com/questions/69439095/what-is-the-correct-way-to-deploy-an-nginx-container-on-azure-app-service
Featured Images: pexels.com