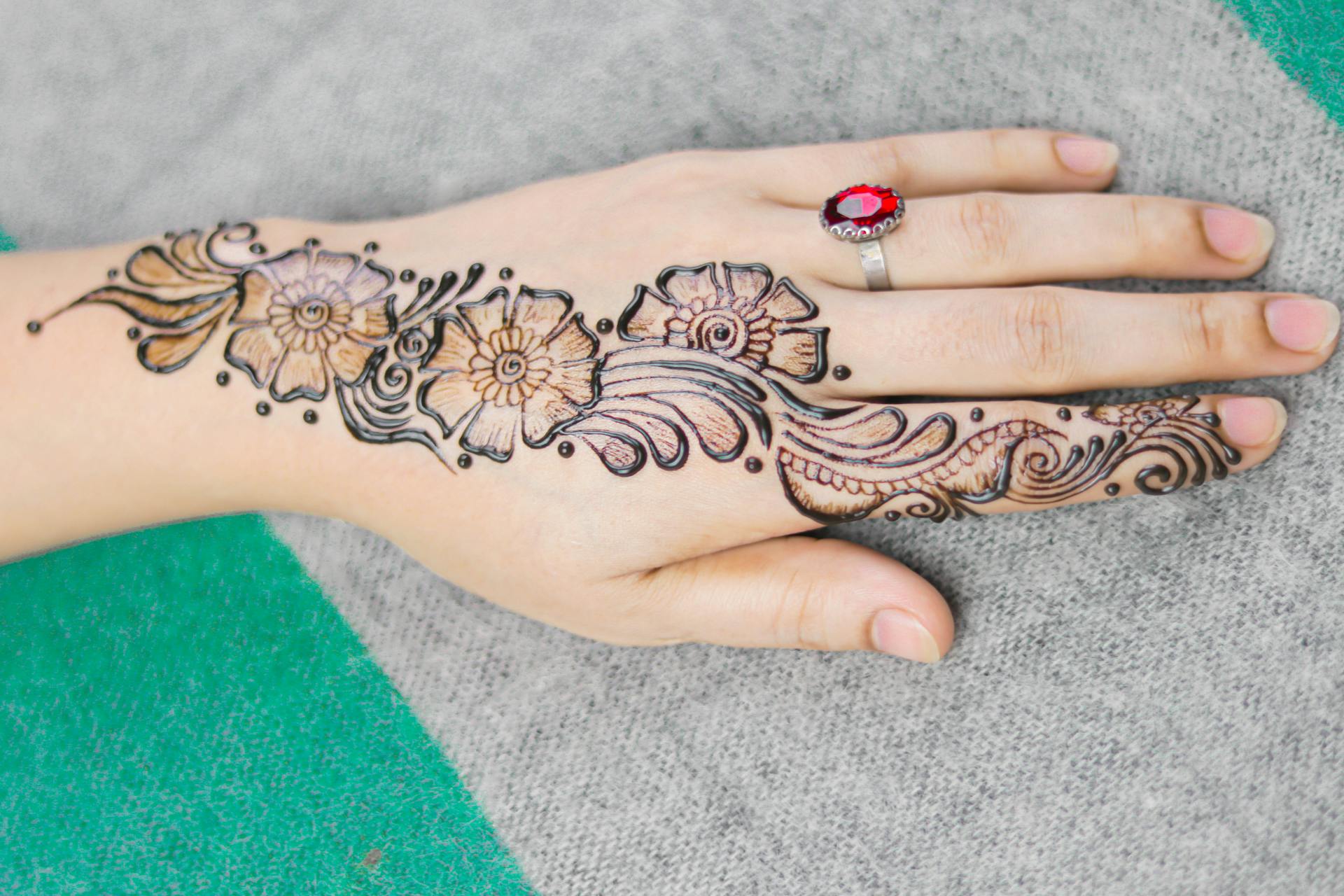
Ruby is a versatile and efficient programming language that's perfect for building web applications.
Ruby is an object-oriented language, which means it organizes code into objects that contain data and functions that operate on that data.
This approach makes it easier to write and maintain large codebases, and it's a key factor in why Ruby is so well-suited for web development.
With Ruby, you can create robust and scalable web applications that meet the needs of your users.
Ruby on Rails, a popular web framework, provides a solid foundation for building web applications quickly and efficiently.
By using Ruby and Ruby on Rails, you can focus on writing code that solves real problems, rather than spending hours setting up a development environment.
Ruby's syntax is designed to be easy to read and write, making it a great choice for developers of all levels.
This means you can get up to speed with Ruby quickly, and start building web applications in no time.
Curious to learn more? Check out: Web Designers Code
Development Architecture
Ruby on Rails is built to deliver rapid products, making it a popular choice for startups and rapid MVP capabilities. It closely follows the Convention Over Configuration principle, which means that it has a rigid initial setup, but this is actually an advantage over flexible frameworks like Django and Flask.
The framework uses the MVC (Model-View-Controller) principle to organize applications. In a default configuration, a model in Ruby on Rails maps to a table in a database and to a Ruby file. For example, a model class User will usually be defined in the file 'user.rb' in the app/models directory, and linked to the table 'users' in the database.
Here are the three main components of the MVC pattern:
- Model: Represents the data and business logic of the application.
- View: Handles the presentation layer, defining how data is displayed to the user.
- Controller: Acts as the intermediary between the Model and View, handling user requests and manipulating data as needed.
Ruby on Rails encourages developers to use RESTful routes, which include actions such as create, new, edit, update, destroy, show, and index. These mappings of incoming requests/routes to controller actions can be easily set up in the routes.rb configuration file.
HTTP Servers
Ruby on Rails is not typically connected to the Internet directly, but rather through a front-end web server.
In the early days, Mongrel was often preferred over WEBrick, but it can also run on a variety of other servers, including Lighttpd, Apache, and Nginx.
From 2008 onward, Passenger has become the most-used web server for Ruby on Rails, replacing Mongrel.
Ruby is also supported natively on IBM i.
Recommended read: Web Server Programming
Built-in Libraries (Gems)
Built-in Libraries (Gems) are a treasure trove for Ruby on Rails developers. They provide functionalities for various tasks like authentication, database interaction, image processing, and more.
Rails comes bundled with a vast ecosystem of pre-written code modules called "gems". These gems eliminate the need to reinvent the wheel and empower developers to integrate desired features quickly.
The extensive library collection includes gems for authentication, database interaction, image processing, and more. This means developers can focus on building their application without worrying about writing code from scratch.
Here are some key features of the built-in libraries (gems):
The gems provide a wide range of functionalities, making it easier for developers to build robust and scalable applications.
Model-View-Controller (MVC) Architecture
The Model-View-Controller (MVC) Architecture is a fundamental concept in Ruby on Rails development. This architecture separates an application into three distinct layers: Model, View, and Controller.
The Model represents the data and business logic of the application, and is often linked to a database table. For example, a model class User will usually be defined in the file 'user.rb' in the app/models directory, and linked to the table 'users' in the database.
The View handles the presentation layer, defining how data is displayed to the user. In the default configuration of Rails, a view is an erb file, which is evaluated and converted to HTML at run-time.
The Controller acts as the intermediary between the Model and View, handling user requests and manipulating data as needed. A controller may provide one or more actions, which are basic units that describe how to respond to a specific external web-browser request.
Here's a breakdown of the MVC pattern:
- Model: Represents the data and business logic of the application.
- View: Handles the presentation layer, defining how data is displayed to the user.
- Controller: Acts as the intermediary between the Model and View, handling user requests and manipulating data as needed.
By following the MVC pattern, developers can write clean code, make maintenance easier, and improve testability. This separation of concerns is a key benefit of the MVC architecture.
Automatic Testing
Automatic testing is a crucial aspect of software development, and Rails makes it easy to adopt a test-driven development (TDD) approach.
Rails encourages the use of built-in tools for writing unit and integration tests, which ensure code quality and catch errors early on.
These automated tests are a game-changer, allowing developers to make future code changes with confidence.
With automated testing, you can ensure that your code is stable and reliable, reducing the risk of bugs and errors.
By writing unit and integration tests, you can catch errors early on and avoid costly rework down the line.
For more insights, see: Web & Mobile Development
Choosing the Right Technology
Ruby on Rails was created by David Hansson in 2004 to improve developer productivity.
With its robust ecosystem and community support, Ruby on Rails has powered nearly 3 million websites and applications.
Ruby on Rails is a popular web development framework that's been around since 2004.
Ruby and Rails is a great choice for web projects, considering its extensive pros and cons.
A fresh viewpoint: Ruby Website Hosting
Core Features
Ruby on Rails boasts a collection of features that contribute to its efficiency and developer-friendly nature. One of the key features is its collection of features that contribute to its efficiency and developer-friendly nature.
Ruby on Rails has a modular design, which allows developers to build web applications quickly and efficiently. This is a result of its built-in features that simplify the development process.
By using Ruby on Rails, developers can focus on writing code that solves the problem at hand, rather than worrying about the underlying infrastructure.
Suggestion: Web Programmer Web Developer
Core Features
Ruby on Rails boasts a collection of features that contribute to its efficiency and developer-friendly nature.
One of the key features of Ruby on Rails is its collection of features that contribute to its efficiency and developer-friendly nature. Here's a breakdown of some key features:
Rails has a modular architecture, making it easy to add or remove functionality as needed.
This modularity allows developers to focus on specific aspects of the application without worrying about the underlying structure.
Another important feature is its use of the Model-View-Controller (MVC) pattern, which separates application logic into three interconnected components.
This separation of concerns makes it easier to maintain and update the application over time.
Ideal Use Cases
When choosing the right framework for your web development project, it's essential to consider the ideal use cases for each. Here are some key points to keep in mind:
Ruby on Rails excels in rapid prototyping, MVP development, and building user-centric applications like e-commerce platforms and content management systems.
Django, on the other hand, is well-suited for data-driven web applications, complex back-end logic, and scientific computing projects alongside web development.
Laravel is ideal for enterprise applications, complex web applications, and projects requiring high performance.
Express.js shines in building APIs, microservices, real-time applications, and single-page applications (SPAs) when combined with front-end frameworks like React or Vue.js.
Here's a quick rundown of the ideal use cases for each framework:
Ultimately, the choice between these frameworks will depend on the specific requirements of your project, your development team's familiarity with the underlying language, and the ecosystem that best supports your application's needs.
Security and Scalability
Ruby on Rails has made significant strides in addressing scalability concerns, with over 1.2 million websites running the framework as of 2016. Some of the largest sites, including Airbnb and GitHub, have successfully built scalable web applications using Rails.
A robust architecture is key to scaling Rails applications, allowing developers to handle increasing user traffic and data volume efficiently. This is achieved through horizontal scaling, which enables adding more servers to distribute workload and improve performance as needed.
Despite initial criticisms, Rails has proven itself capable of handling large-scale applications, with many high-profile consumer web firms relying on the framework to build scalable web applications.
What Are the Disadvantages of?
Ruby on Rails, a popular web development framework, has its fair share of drawbacks when it comes to security and scalability.
One of the major disadvantages of Ruby on Rails is its run-time speed, which can be a significant issue for large-scale applications.
Improper documentation is another significant disadvantage, making it difficult for developers to learn and troubleshoot issues.
Here are some of the key disadvantages of Ruby on Rails:
- Run-time Speed
- Lack of Flexibility
- Improper Documentation
- Huge Cost for Mistakes
- Mature Framework
A mature framework like Ruby on Rails can also make it harder to adapt to new technologies and best practices, which can hinder scalability.
Security Features
Ruby on Rails prioritizes application security by offering built-in mechanisms for preventing common web vulnerabilities like SQL injection and Cross-Site Scripting (XSS). These features help developers build secure applications without needing to implement security measures from scratch.
In 2013, a session cookie persistence security flaw was reported in Ruby on Rails, but administrators can configure cookies to be stored on the server using mechanisms like ActiveRecordStore to prevent unauthorized access.
Researchers Daniel Jackson and Joseph Near developed a data debugger called "Space" that can analyze the data access of a Rails program and determine if the program properly adheres to rules regarding access restrictions. Their analysis of 50 popular Web applications using Space uncovered 23 previously unknown security flaws.
Fortunately, Rails' built-in security features make it easier for developers to avoid common web vulnerabilities, allowing them to focus on building secure and efficient applications.
Scalability
Scalability is a crucial aspect of building a robust web application. Despite initial criticisms, Ruby on Rails has proven to be scalable, as evident from the large-scale adoption by high-profile consumer web firms like Airbnb, Cookpad, GitHub, GitLab, Scribd, Shopify, and Basecamp.
In fact, Gartner Research noted in 2011 that many of these firms are using Ruby on Rails to build scalable web applications. This is a testament to the framework's ability to handle increasing user traffic and data volume.
Ruby on Rails applications can be efficiently scaled to handle growing demands, thanks to their robust architecture. This allows developers to focus on building a solid foundation, rather than worrying about scalability issues later on.
Here are some key scalability features of Ruby on Rails:
- Robust architecture: Rails applications can be efficiently scaled to handle increasing user traffic and data volume.
- Horizontal scaling: Allows adding more servers to distribute workload and improve performance as needed.
As of January 2016, it's estimated that over 1.2 million web sites are running Ruby on Rails, demonstrating its widespread adoption and scalability.
Community and Ecosystem
Ruby web development has a thriving community that's hard to ignore. With extensive online resources, tutorials, and forums, you'll have access to support, best practices, and solutions from a large and active developer community.
The community's generosity is evident in the wide range of readily available plugins and tools developed by its members, further enhancing functionality and extending Ruby on Rails' capabilities.
A rich ecosystem with a wide array of gems (libraries) for various functionalities is one of the key features of Rails. This means you can easily find and integrate libraries that suit your project's needs.
Django, another popular framework, has a strong ecosystem, especially for data-driven applications. The Python community is large and active, providing numerous packages and tools that integrate well with Django.
Laravel features a robust ecosystem with many packages for extending its capabilities. The Laravel community is known for its enthusiasm and the availability of learning resources.
Express.js benefits from the vast npm ecosystem, offering a multitude of packages for nearly any functionality. The Node.js and Express community is large and active, contributing to a wealth of resources and support.
Here are some key differences in the ecosystems of these popular frameworks:
Getting Started
To get started with Ruby on Rails development, you'll want to follow a roadmap that guides you on your journey towards becoming a Rails developer.
First, learn Ruby on Rails programming from reliable sources like Rails Guides and Thinkful. You can start by installing Rails and creating an application using the Ruby on Rails language.
To install Rails, download and install the latest stable version of Ruby from https://www.ruby-lang.org/en/, and then use the gem command to install Rails. You can also set up a Text Editor or IDE like Visual Studio Code or RubyMine to configure for Ruby and Rails development.
It's essential to understand the core principles of Rails, including DRY (Don't Repeat Yourself), Convention over Configuration, CRUD (Create, Read, Update, Delete), and REST (Representational State Transfer). These principles will help you write efficient and maintainable code.
Here's a quick rundown of the key areas to focus on:
- DRY: Every piece of knowledge must have a single, unambiguous, authoritative representation within a system.
- Convention over Configuration: This paradigm increases productivity by adhering to coding conventions and the best way of doing things.
- CRUD: A function that guides actions on databases.
- REST: Guides actions performed on URLs.
After setting up your development environment, create a simple Rails application to confirm everything is set up correctly. This will generate the basic structure of a Rails application.
Consider reading: Person Application Share Web Dev
Learning and Resources
Learning Ruby programming is a crucial step before diving into Rails development. To grasp the fundamentals, focus on understanding variables, data types, operators, control flow statements, methods, and objects.
Several online tutorials and resources can help you learn Ruby effectively, such as Codecademy's interactive Ruby learning pathways. Don't just read - engage with the material through exercises and small coding challenges.
Practice and experimentation are key to learning Ruby. Online platforms like Codecademy offer interactive learning pathways that can help you learn Ruby effectively.
To explore Rails tutorials and resources, start with the official Ruby on Rails Guides, which cover a wide range of topics from basic concepts to advanced functionalities. They're an excellent starting point for learning Rails.
Interactive coding environments, such as Try Ruby and Rails Tutorials, offer hands-on learning experiences where you can learn by doing. Online courses and video tutorials can provide structured learning paths and visual explanations of Rails development concepts.
Curious to learn more? Check out: Codeigniter Web Development
The Rails community is active and helpful, so engage in forums like Stack Overflow and the official Rails forum to ask questions and learn from others.
Here are some recommended resources for learning Ruby and Rails:
- Official Ruby on Rails Guides
- Try Ruby
- Rails Tutorials
- Codecademy's Ruby learning pathways
- Stack Overflow
- Official Rails forum
Remember, learning Rails takes dedication and practice. As you progress, consider building small projects to solidify your understanding and explore different functionalities in a practical setting.
Frequently Asked Questions
Is Ruby good for web development?
Yes, Ruby is a popular choice for web development due to its simplicity and helpful features. It's a great language to consider for building web applications, especially with its many useful frameworks.
Is Ruby still relevant in 2024?
Yes, Ruby on Rails remains a relevant and in-demand web development framework in 2024. Its strong community and full-stack capabilities make it a popular choice for developers.
Is Ruby backend or frontend?
Ruby on Rails is a backend framework, used for building server-side logic and database integration in web applications
What is the difference between HTML and Ruby?
HTML is a markup language that defines web page structure, whereas Ruby is a programming language that adds functionality with concise syntax and methods. Understanding the difference between these two is key to building dynamic and interactive web experiences.
Sources
Featured Images: pexels.com