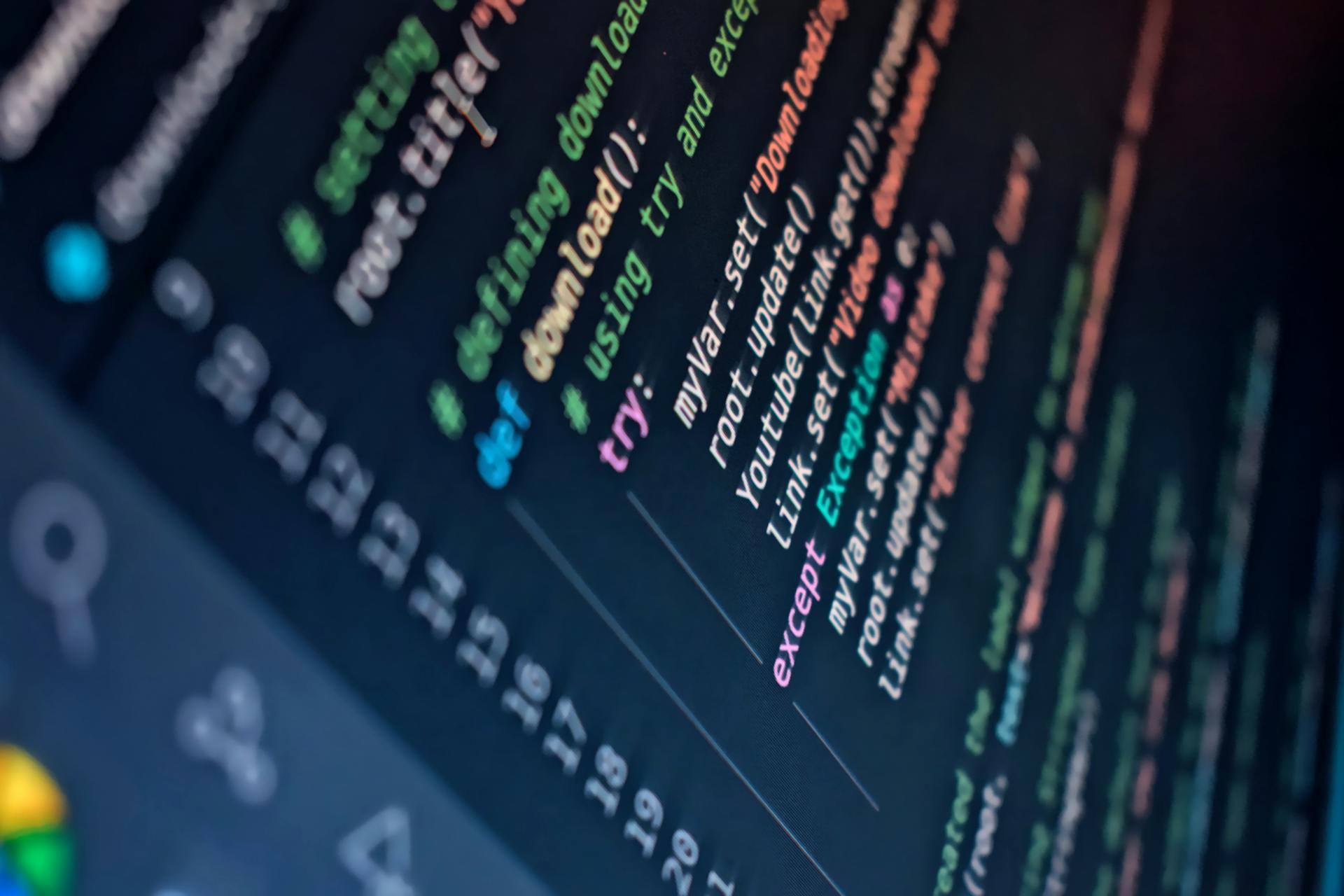
Selenium CSS selectors are a powerful tool for locating elements on a webpage. They allow you to write code that can find specific elements based on their HTML structure.
CSS selectors are used to identify elements on a webpage, and Selenium uses them to interact with those elements. With CSS selectors, you can find elements by their class, id, or even their position in the HTML document.
One of the key benefits of using CSS selectors is that they can be very specific. For example, you can use a CSS selector to find an element with a specific class, such as ".header" or "#logo". This allows you to target very specific elements on a webpage.
Related reading: Create Css Selector from Webpage
What is a CSS Selector?
A CSS Selector is a pattern-based approach for locating and interacting with HTML elements on a web page. It utilizes the syntax and rules of CSS to target elements based on their attributes.
If this caught your attention, see: Css Selector the Last 2 Child Elements
CSS Selectors can be used to locate elements on a web page, and they're beneficial because they're shorter than XPath locators. They offer a more clear and crisp method to locate the element.
There are several types of CSS Selectors, including:
- Using ID
- Using ClassName
- Using Attribute
- Using Substring
- Using nth-child
CSS Selectors are supported in all modern browsers and provide a flexible and efficient way to locate elements.
Locating Elements
Locating elements is a crucial step in Selenium WebDriver, and CSS selectors make it efficient. You can locate elements using CSS selectors by prefixing the ID value with a hash (#) symbol.
To locate elements by ID, use the CSS selector "#username" if an element is defined in HTML as . This method is recommended when you know the exact ID of the element, ensuring quick and precise element selection.
Classes are not unique, so locating elements by class attributes might locate multiple elements. Use the period (.) symbol followed by the class name, as in .container, to access elements with a specific class.
A different take: How to Find Css Selector
Locating Elements
Locating elements is a crucial step in web automation, and it's essential to do it correctly. You can use CSS selectors to locate elements by their ID, class, or attributes.
To locate an element by its ID, you can use the hash (#) symbol followed by the ID value. For example, if an element has an ID of "username", you can use the CSS selector "#username". This method is recommended when you know the exact ID of the element.
When using CSS selectors, it's essential to verify their validity to ensure they are correctly written. Typos or missing characters can cause them to fail. You can use online CSS validator tools or browser extensions to validate the syntax and correctness of your selectors.
Locating elements by class is also possible using CSS selectors. You can use the period (.) symbol followed by the class name to access elements with a specific class attribute. For instance, if an HTML element is marked with the class attribute as "container", it can be accessed in Selenium using the CSS selector ".container".
On a similar theme: Css Selector That Styles Child If Parent Has an Attribute
Using CSS selectors, you can find elements based on any attribute, not just the 'id' or 'class' attributes. You can do this by enclosing the attribute name with the value in square brackets []. For example, to find 'input' elements that have a "name" attribute with the value "email", you would use 'input[name="email"]'.
Combining selectors can significantly enhance your ability to locate elements with precision. For example, to find a button within a specific section that has both an ID and a class, you might use a selector like "#main .button". This selector locates elements with the class "button" inside an element with the ID "main".
You can use online CSS selector testing tools to enter a selector and see which elements it matches. These tools can help verify the accuracy of your selectors before incorporating them into your Selenium automation scripts.
Expand your knowledge: Css Select Data Attribute in Pseudo Selector
Locating by Attributes
Locating by Attributes is a powerful technique in finding elements on a webpage. You can use CSS selectors to find elements based on any attribute, not just 'id' or 'class'.
The syntax involves enclosing the attribute name with the value in square brackets. For example, to find 'input' elements that have a "name" attribute with the value "email", you would use 'input[name="email"]'.
You can use this approach to find elements based on any attribute, such as 'href', 'title', 'type', etc. It's a very flexible method that can be applied to any element.
To locate an element using multiple attributes, you can use the syntax 'tagName[@attributeName='attributeValue'][@attributeName='attributeValue']'. This is similar to using the AND operator.
For example, to find an 'input' element with 'name' and 'type' attributes, you would use 'input[name='keyword'][type='text']'.
You can also use the OR operator by separating the attributes with a comma. For example, to find an 'input' element with either 'name' or 'type' attribute, you would use 'input[name='keyword'], [type='text']'.
Here's a summary of the syntax for locating elements by attributes:
Selector Types
Selector Types are the building blocks of Selenium CSS Selectors. They help identify elements on a webpage based on various criteria.
There are several types of selectors, each with its own purpose. The Element Selector, for example, targets elements based on their tag names, such as "div" which selects all div elements.
The ID Selector is another type, which selects elements based on their unique IDs. This is useful when you need to target a specific element with a unique identifier, like "#myElement".
A Class Selector targets elements based on their class attribute, selecting elements with a specific class, like ".myClass". This is helpful when you need to target multiple elements with a common class.
The Attribute Selector selects elements based on their attributes and attribute values, such as "[name='email']" which selects elements with the attribute name="email". This is useful when you need to target elements with specific attributes.
Here are some commonly used selector types in Selenium CSS Selectors:
Types with Examples
In this section, we'll explore the different types of selectors and how they're used in CSS. We'll start with the element selector, which targets elements based on their tag names.
The element selector is straightforward, and it's often used to select all elements of a specific type. For example, selecting all div elements on a page can be done with the selector "div".
ID selectors are another type of selector that targets elements based on their unique IDs. This is useful when you need to select a specific element on the page. For instance, the selector "#myElement" would select the element with the ID "myElement".
The class selector targets elements based on their class attribute. This is useful when you need to select multiple elements that share the same class. For example, the selector ".myClass" would select all elements with the class "myClass".
Attribute selectors target elements based on their attributes and attribute values. This can be useful when you need to select elements based on specific attributes. For example, the selector "[name='email']" would select all elements with the attribute name="email".
The descendant selector targets elements that are descendants of another element. This can be useful when you need to select elements that are nested inside other elements. For example, the selector "div p" would select all paragraphs within div elements.
You might enjoy: Tailwind Css Select
Here are the different types of selectors we've discussed, summarized in a table:
The child selector is similar to the descendant selector, but it targets only direct children of another element. For example, the selector "div > p" would select only paragraphs that are direct children of div elements.
Intriguing read: Div Class Css
Nth-child
Nth-child is a powerful selector that helps you target a particular element based on its position. It's like saying "get me the 6th child within this list" and nth-child does just that.
You can use nth-child to select the nth child of an element, like ul> li:nth-child(6), which would select the country code of Algeria. It's a great way to iterate through a list and select the required element.
The nth-child selector takes an input like a number, odd, or even, and it can also use the An+B syntax, like 3n+2. For example, #profile > img:nth-child(2) will select the second img tag within the #profile element.
You might enjoy: Css Selector List
If you want to get an element counting from the bottom, you can use the :nth-last-child pseudo-class. For instance, #profile > *:nth-last-child(1) will give you the last child, which is a p tag with the text "Conclusion…".
The :nth-last-child pseudo-class is similar to :last-child, but it's more flexible and can be used with different values.
If this caught your attention, see: Css Last Child Selector
Adjacent Sibling Selector
The adjacent sibling selector is a powerful tool in CSS. It allows you to select an element that follows another element, using a plus sign (+) between the two element types.
To use the adjacent sibling selector, you need to specify the element that comes before it. For example, if you want to select all h1 tags that are placed right after an img tag, you can use the following: img + h1.
The element that comes after the plus sign is the one that gets selected. For instance, using h1 + p, the p element is selected, not the h1 element.
You can reuse existing HTML snippets to locate elements using the adjacent sibling selector.
Take a look at this: Css Selector Select Child of Parent
Multiple Attributes
When you need to locate a web element based on multiple attributes, you can use the CSS syntax: tagName[@attributeName='attributeValue'][@attributeName='attributeValue']. This is similar to the AND operator.
You can use this syntax to locate elements with specific tag and attribute names and values. For example, input[name='keyword'][type='text'].
Alternatively, you can use the OR operator by separating the attribute-value pairs with a comma. This is useful for dynamic elements whose attributes keep changing but to some specific values only. For instance, input[name='keyword'], [type='text'].
If an element is not located by a single attribute, you can use multiple attributes to narrow down the search. This is especially useful for handling dynamic web elements.
Here are some examples of using multiple attributes:
By using multiple attributes, you can write more specific and effective CSS selectors that help you locate the web elements you need.
Selector Syntax
Selector syntax is a crucial part of Selenium CSS selectors. It's used to locate elements on a webpage.
The syntax consists of a tag name, optionally followed by a class name, an ID, or an attribute. The dot notation is used to select elements by their class name.
For example, a selector like `.header` selects all elements with the class name "header".
Attributes
Attributes are a powerful way to select elements in CSS, and they're used to identify web elements based on their attributes and values.
You can use attributes to select elements based on their presence or value, and this is done by enclosing the attribute name in square brackets [].
For example, if you want to select all input elements where the type attribute equals "text", you would write "input[type='text']".
You can also use attribute selectors to select elements based on the presence of a given attribute, regardless of its value. This is done by using the syntax "tagName[attributeName]".
For instance, if you want to select all elements that have a "name" attribute, you would write "[name]".
Attribute selectors can be used with any attribute, not just "id" or "class". You can also use them with any tag, not just "input".
Here's a summary of how to use attribute selectors:
- To select elements with a specified tag and attribute name and its value, use "tagName[attributeName='attributeValue']".
- To select elements with any tag but has specified attribute name and its value, use "[attributeName='attributeValue']".
You can also use multiple attributes to select elements, which is similar to using the AND operator. This is done by using the syntax "tagName[@attributeName='attributeValue'][@attributeName='attributeValue']".
For example, if you want to select an input element with a name attribute value of "keyword" and a type attribute value of "text", you would write "input[name='keyword'][type='text']".
Finally, you can use the "~=" operator to match a word within an attribute value. This is useful when you want to select elements based on a partial match of an attribute value.
For instance, if you want to select all elements with a class name containing the word "iti__country", you would write "li[class~='iti__country']".
See what others are reading: Input Type Selector Css
Substring
Substring matching is a powerful feature in CSS selectors that allows you to locate elements based on partial strings.
To match a substring, you can use the *= operator, as shown in the example `input[name*=’ser’]`. This operator matches the string using a substring.
Matching a substring can be useful when you need to locate an element based on a partial attribute value, such as a name or a class.
Discover more: Css Name Selector
Here are some examples of how to match a substring:
The key thing to remember is that the *= operator is used to match a substring, whereas the ^= and $= operators are used to match a prefix and a suffix respectively. The ~= operator is used to match a word.
In practice, this means you can use the *= operator to locate an element based on a partial attribute value, such as `input[name*=’ser’]`. This could be useful if you need to locate an element based on a partial name or class.
Hash for IDs
Hash for IDs is a quick and reliable way to locate elements on a webpage.
Using a hash (#) symbol is the syntax for referencing an ID in CSS selectors.
You can locate an element by its ID using the CSS selector "#logout", which is as simple as prefixing a hash # to the ID.
This method is efficient because IDs are unique in HTML, making it a fair assumption that there's usually one.
A unique perspective: Css Id Selector
You can pair the ID with a tag name to make the selector even more specific, such as "a#logout", which finds an anchor tag with an ID valued as "logout".
The ID selector is one of the easiest and most effective methods of choosing elements using CSS.
Locating elements by ID is highly efficient in Selenium WebDriver due to the uniqueness of the ID attribute in HTML.
You can use CSS selectors for this purpose by prefixing the ID value with a hash (#) symbol, as in "#username".
The CSS selector "#logout" will help you locate an element that has an ID with a value "logout", regardless of its tag name.
This selector is even more specific when paired with a tag, such as "a#logout", which finds an anchor tag with an ID valued as "logout".
Using the ID value alone, like "#logout", is a direct way to locate a web element which has that ID as an attribute.
You can use the CSS syntax "tagName#IDValue" to find a web element with a specified tag and ID, such as "input#inputValEnter".
A unique perspective: Css Selector That Targets a Specific Style Declaration
Dot for Classes
You can use a dot . to locate elements by their class attributes. This is done by prefixing the dot . to the class name.
To access an element with a class attribute like, you use the CSS selector ".container".
Classes are not unique, so this selector might locate multiple elements. In such cases, further specificity might be needed either by chaining more conditions or by using other element attributes.
The dot . selector will identify an element with a class btn_red. If more than one element is found, the first one is returned.
You can combine a tag name and a class name by putting the tag name in front of the class. For example, find an anchor tag with an ID valued as_logout_, which can be written as "a #logout".
A fresh viewpoint: Css Selector Multiple Attributes
Frequently Asked Questions
How to find XPath using CSS selector?
To find the XPath of an element, switch to Selection Mode in DevTools, select the element, and get its CSS selector, then use the Elements tab to generate the XPath. This process allows you to easily obtain the XPath for any element on the page.
How to find elements by selector in CSS?
To find elements by CSS selector, use the find_element or find_elements method with the By.CSS_SELECTOR argument in Selenium. This will return the first or all matching elements on the page, respectively.
How to get element by class in CSS selector?
To select an element by class in CSS, use the dot notation (.class_name) after the element type. This syntax targets elements with the specified class attribute value.
How do I search for an element in CSS selector in Chrome?
To find a CSS selector in Chrome, navigate to the "Elements" page of the Dev tools, select the element, and click on the "CSS Selector" tab. From there, click the button to copy the complete and properly formatted selector.
Sources
- https://www.pcloudy.com/blogs/understanding-css-selectors-in-selenium/
- https://contextqa.com/css-selector-in-selenium/
- https://hackernoon.com/why-css-selectors-are-the-most-useful-selenium-webdriver-locators-28082be1cd3b
- https://intellipaat.com/blog/css-selector-in-selenium/
- http://makeseleniumeasy.com/2017/04/26/css-selector-in-selenium-webdriver-points-you-must-know/
Featured Images: pexels.com