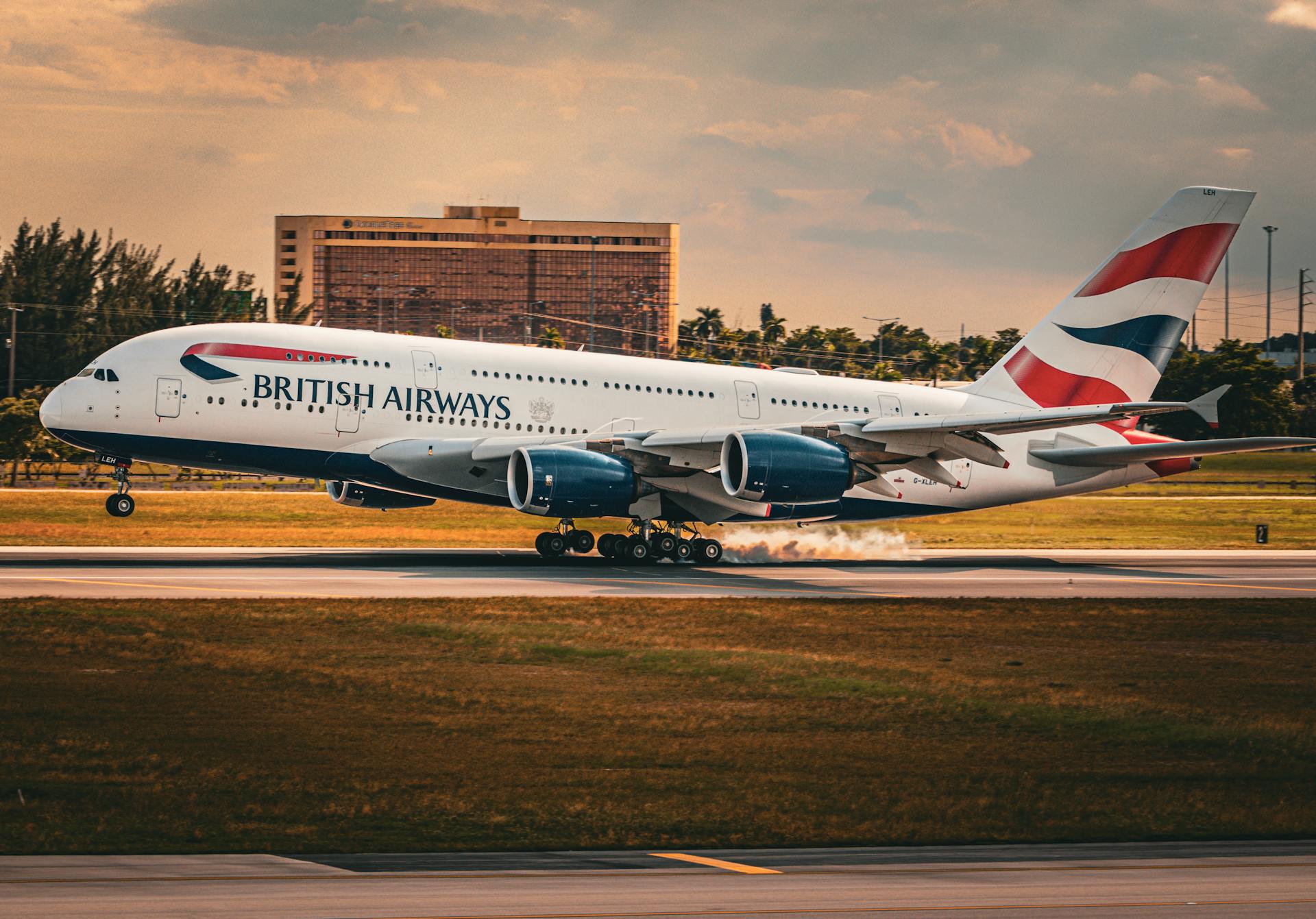
Tailwind CSS Rails development is a game-changer for web developers. With Tailwind CSS, you can build custom user interfaces without writing any custom CSS.
Tailwind CSS is a utility-first CSS framework that provides a set of pre-defined classes to style your HTML elements. This approach simplifies the development process and reduces the time spent on styling.
By integrating Tailwind CSS with Rails, you can create responsive and maintainable web applications. Tailwind CSS provides a set of pre-defined classes that can be used to style your Rails application's layout, components, and pages.
The Tailwind CSS integration with Rails is made possible through a gem called tailwind-rails. This gem provides a simple way to get started with Tailwind CSS in your Rails application.
Broaden your view: Front-end Web Development
Install
To install Tailwind CSS in your Ruby on Rails project, you can use the following methods.
If you're starting a new project, you can generate a new application preconfigured with Tailwind by using the `--css tailwind` option. This will set up everything you need to get started.
To add Tailwind to an existing project, you'll need to run two commands: `./bin/bundle add tailwindcss-rails` and `./bin/rails tailwindcss:install`. This will install the necessary gem and configure Tailwind for your project.
Here are the steps in a more detailed format:
1. Run `./bin/bundle add tailwindcss-rails`
2. Run `./bin/rails tailwindcss:install`
These commands will install the tailwindcss-rails gem and configure Tailwind for your project.
A fresh viewpoint: Add a Class in Css
Implementing Tailwind CSS
You can set up Tailwind in a Ruby on Rails environment using the application generator, which directly supports Tailwind in Rails 7.x.
To use Tailwind's neat feature that generates the CSS file your application needs, you'll need to run a little utility in development mode: bin/rails tailwindcss:watch.
The tailwindcss-rails gem makes several Rails tasks available, including bin/rails tailwindcss:install, which installs the configuration file, output file, and Procfile.dev.
You can generate the output file using bin/rails tailwindcss:build, or use bin/rails assets:precompile for production use.
Here are some common commands you can use with the tailwindcss-rails gem:
- bin/rails tailwindcss:install - installs the configuration file, output file, and Procfile.dev
- bin/rails tailwindcss:build - generate the output file
- bin/rails tailwindcss:watch - start live rebuilds, generating output on file changes
The gem also generates a Procfile.dev file which will run both the rails server and a live rebuild process.
Implementing Our Builder
To implement our Tailwind CSS builder, we need to create a custom builder that inherits from the default FormBuilder provided by ActionView.
We'll define our builder and move our Tailwind class string into the text_field method to style each input field individually.
Our ultimate goal is to give the form_with block access to each of the input field methods.
We'll use class_eval to generate methods for us and include two paths through every method; one for applying styling and one for pointing back to the default behavior.
We'll call for field_helpers, which comes from ActionView::Helpers::FormBuilder, and subtract a few methods from field_helpers that don't behave like a text_field.
We'll add our class_eval loop, which handles our metaprogramming, and rename our text_field method to text_like_field to show we're handling many different types.
We'll adjust text_like_field to call send instead of super and introduce an option called tailwindified to control our flow.
On a similar theme: Tailwind Css Input Range
This approach will allow us to modify each text_field call individually and make our builder more reusable.
Here's a list of the steps we'll take to implement our builder:
- Define our custom builder and inherit from the default FormBuilder.
- Move our Tailwind class string into the text_field method to style each input field individually.
- Use class_eval to generate methods for us.
- Include two paths through every method; one for applying styling and one for pointing back to the default behavior.
- Call for field_helpers and subtract a few methods from field_helpers that don't behave like a text_field.
- Adjust text_like_field to call send instead of super and introduce an option called tailwindified to control our flow.
By following these steps, we'll be able to create a reusable and maintainable builder that styles our input fields with Tailwind CSS.
Landing Page Analysis
A landing page is a crucial part of any application, and in this case, we're using Tailwind CSS to style it.
A title is essential for any landing page, and in our example, it's configured to use Tailwind as its CSS framework.
The title is styled with only two classes for the h1 tag: font-bold and text-4xl.
The font-bold class controls the font weight, and the text-4xl class controls the font size.
If you change the text-4xl class to text-xl, the new style will be automatically applied.
For another approach, see: Font Weight Tailwind Css
Create a Homepage
To create a homepage in your application, start by creating a new pages directory inside the app/views directory. This is where you'll store your homepage template.
Create a new home.html.erb file inside the app/views/pages directory. This is where you'll add the HTML code for your homepage. You can copy and paste a component from Flowbite, such as a tooltip, into this file to get started.
To handle requests to your homepage, create a new controller called pages_controller.rb inside the app/controllers directory. Add the necessary code to this file to render your homepage template.
Finally, set the homepage as the root page inside the routes.rb file inside the config directory. This will allow you to access your homepage at localhost:3000/.
A unique perspective: Tailwind Css Examples Code
Customizing and Styling
To allow styling to be handled from both the view and the builder level, we need to isolate the class passed from the view with our structure styling. Then we need to combine it with the appearance styling declared in our builder.
Partitioning out the :class from our other options gives us access to do just that. We can use a template literal to safely combine the two styles.
Our resulting HTML in the browser has both the view-layer structure styling matched with the appearance styling from the builder. This allows our view to manage the structure only, while the builder handles the appearance.
For more insights, see: Tailwind Css Nav Styling
Combine Appearance and Structure Styling
By isolating the class passed from the view with our structure styling, we can combine it with the appearance styling declared in our builder.
Partitioning out the :class from our other options gives us access to do just that. I've opted to use a template literal, so we have some safety if no class is given from the view.
Our resulting HTML in the browser has both the view-layer structure styling matched with the appearance styling from the builder.
This approach allows us to handle styling from both the view and the builder level, making our code more concise and easier to manage.
Programmatic Labels
Programmatic labels allow for more control over the appearance and structure of form labels. This is achieved by modifying the `text_like_field` method to return both a label and the input element.
By using a helper method like `tailwind_label`, you can pass label options to it, which will then choose default values if none are provided at the view level. This enables you to add error labels to form inputs using the same API.
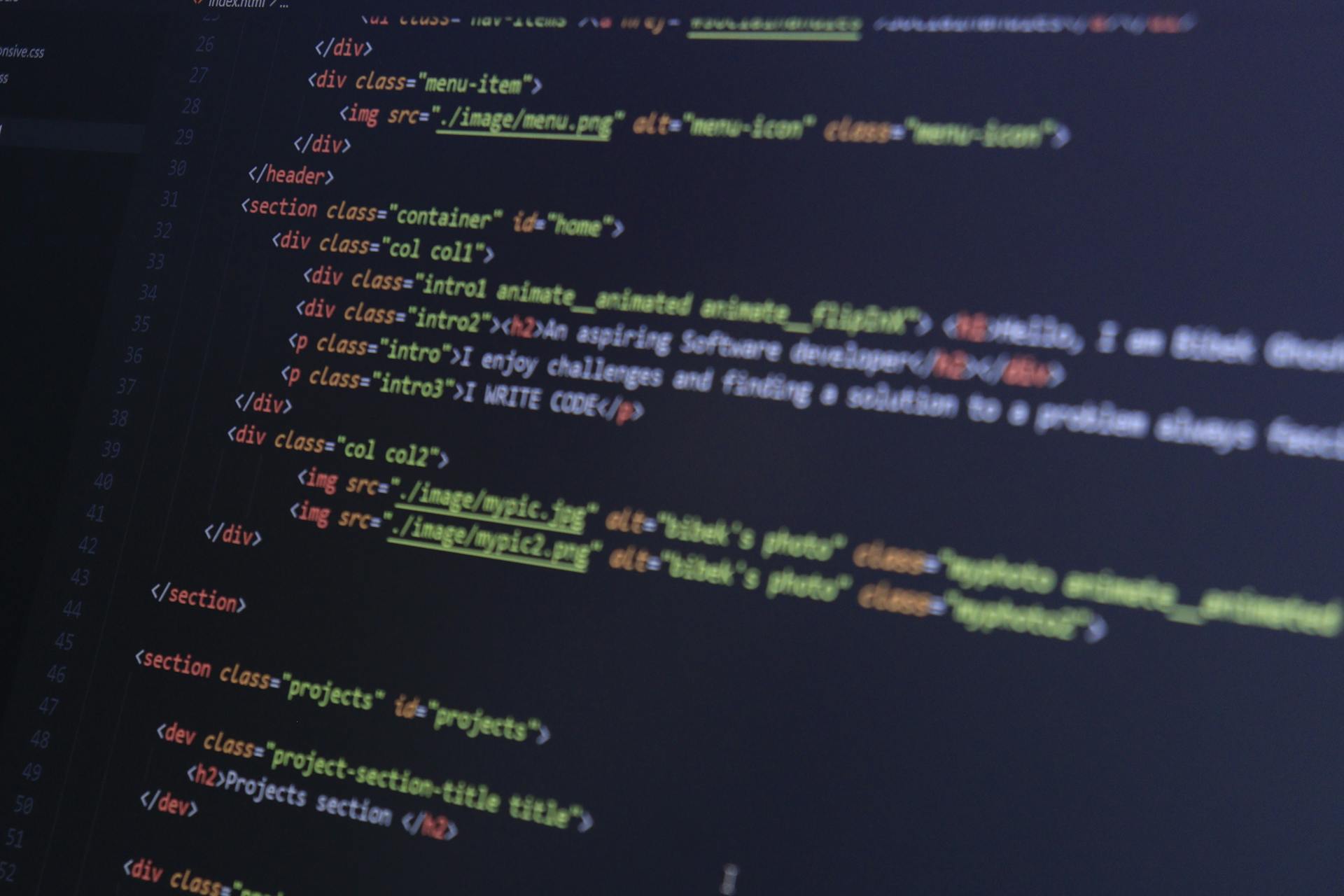
Here's a summary of how programmatic labels work:
- `text_like_field` returns both an input and a label for the input
- Label options are passed to the `tailwind_label` helper method
- `tailwind_label` chooses defaults if no label is provided at the view level
- The appearance style is applied to the label and the default label method is called
Programmatic labels may feel like a small step in functionality compared to the `f.label` approach, but they offer the flexibility to add error labels to form inputs. The decision to use programmatic labels ultimately depends on your project's context and requirements.
Responsive Navigation Bar
A Responsive Navigation Bar is a game-changer for any website. It can change its layout based on the window size, making it easier to navigate on smaller screens.
You can use Tailwind's utility classes to add conditional breakpoints based on a browser's minimum width. For example, the color of a title can change depending on the window size.
By default, the color is a dark shade of gray. If a browser window's width is between 640px and 1024px, it's a shade of teal. If a window's width is above 1024px, it's a shade of purple.
Here's a breakdown of the breakpoints and their widths:
Tailwind also makes it easy to handle columns, allowing you to define a grid that changes its width based on the window size. For example, a label can span two or three columns depending on the window size.
Recommended read: Tailwind Css Font Size
Building for Production
Building for production is a breeze with Tailwind CSS in Rails. The tailwindcss:build command is automatically attached to assets:precompile.
This means that before the asset pipeline digests the files, the Tailwind output will be generated. No extra steps required.
In production use, you can rely on bin/rails assets:precompile to directly call bin/rails tailwindcss:build. This ensures your stylesheets are properly compiled and ready for deployment.
With this setup, you can focus on writing great code without worrying about the technical details of Tailwind CSS and the asset pipeline.
Troubleshooting and Optimization
Upgrading tailwindcss-rails to v2.4.1 or later versions can address some common problems experienced by users.
If you're experiencing issues with tailwindcss-rails, check your version to see if it's up to date.
Error Styling
Error Styling is a crucial aspect of providing a smooth user experience. We want to show users which field was incorrect with styling and possibly labels when they fail a form validation.
ActionView makes it easy to interact with our model and its built-in validations. This is a major advantage of using ActionView.
We store our appearance styling in a variable in text_like_field. This variable is used to apply styling to the form.
The styling is composed by another method that blends structure styling from the view into the appearance styling provided by our builder. This ensures a consistent look and feel.
A new border_color_classes method adds a red border to the input if errors are present on the model attribute. This helps draw attention to the incorrect field.
We also have a helper method to check for errors on the object. This method is used to determine if the form should be styled with errors.
Here are the key points about error styling:
- Store appearance styling in a variable in text_like_field
- Use a method to compose the whole style string
- Add a red border to the input with border_color_classes method
- Use a helper method to check for errors on the object
Troubleshooting
If you're experiencing issues with TailwindCSS, upgrading to v2.4.1 or later versions can resolve common problems.
You may encounter a warning "Unrecognized at-rule or error parsing at-rule ‘@tailwind’" in the browser console due to a misconfiguration with pre-existing asset pipeline stylesheets. This occurs when the asset pipeline incorrectly processes application.tailwind.css.
To fix this, you can either remove the application.css file, relying solely on TailwindCSS for styles, or remove the require_tree . line from the application.css file if you want to keep using the asset pipeline.
For another approach, see: Tailwindcss Not Working
Conflict with Sass
If you're using Tailwind with Rails, you might encounter issues with the sassc-rails extension.
In Rails 6, sassc-rails is included by default in the Gemfile, but it can conflict with Tailwind's modern CSS features.
Removing sassc-rails from your Gemfile is a simple solution to avoid errors like SassC::SyntaxError.
Consider reading: Jquery Ui Rails
Best Practices and Comparison
Tailwind CSS is a game-changer for Rails developers, and understanding its best practices is crucial for getting the most out of it.
One of the key benefits of Tailwind is its utility-based approach, which allows us to compose the style of each element using specific CSS classes that focus on different parts of the style. This makes it easy to get exactly what we need without having to write a lot of custom CSS.
Tailwind's extensibility is another major advantage, as we can easily extend or customize its defaults through a simple configuration file.
This means we can tailor Tailwind to our specific needs without having to start from scratch.
Less custom CSS is a major benefit of using Tailwind, as we only assemble classes to style elements, which reduces our reliance on custom CSS and makes it easier to share styles.
Here are some of the key ways Tailwind compares to other frameworks:
- Utility-based: We compose the style of each element using specific CSS classes, each focusing on different parts of the style.
- Extensible: We can extend or customize TailwindCSS' defaults through a simple configuration file.
- Less custom CSS: Since we only assemble classes to style elements, we rely less on custom CSS and can share styles (including complete themes) using HTML files and snippets.
With Tailwind, we can also easily shade colors and simplify spacing, making it a breeze to create professional-looking designs.
The combination of these features makes Tailwind an ideal choice for Rails developers who want to create fast, efficient, and beautiful websites.
Sources
- https://testdouble.com/insights/optimizing-rails-forms-with-tailwind
- https://github.com/rails/tailwindcss-rails
- https://blog.appsignal.com/2024/06/05/how-to-use-tailwind-css-for-your-ruby-on-rails-project.html
- https://flowbite.com/docs/getting-started/rails/
- https://medium.com/@ahmednadar/setup-tailwindcss-postcss-and-esbuild-on-rails-7-d01cebaa493e
Featured Images: pexels.com