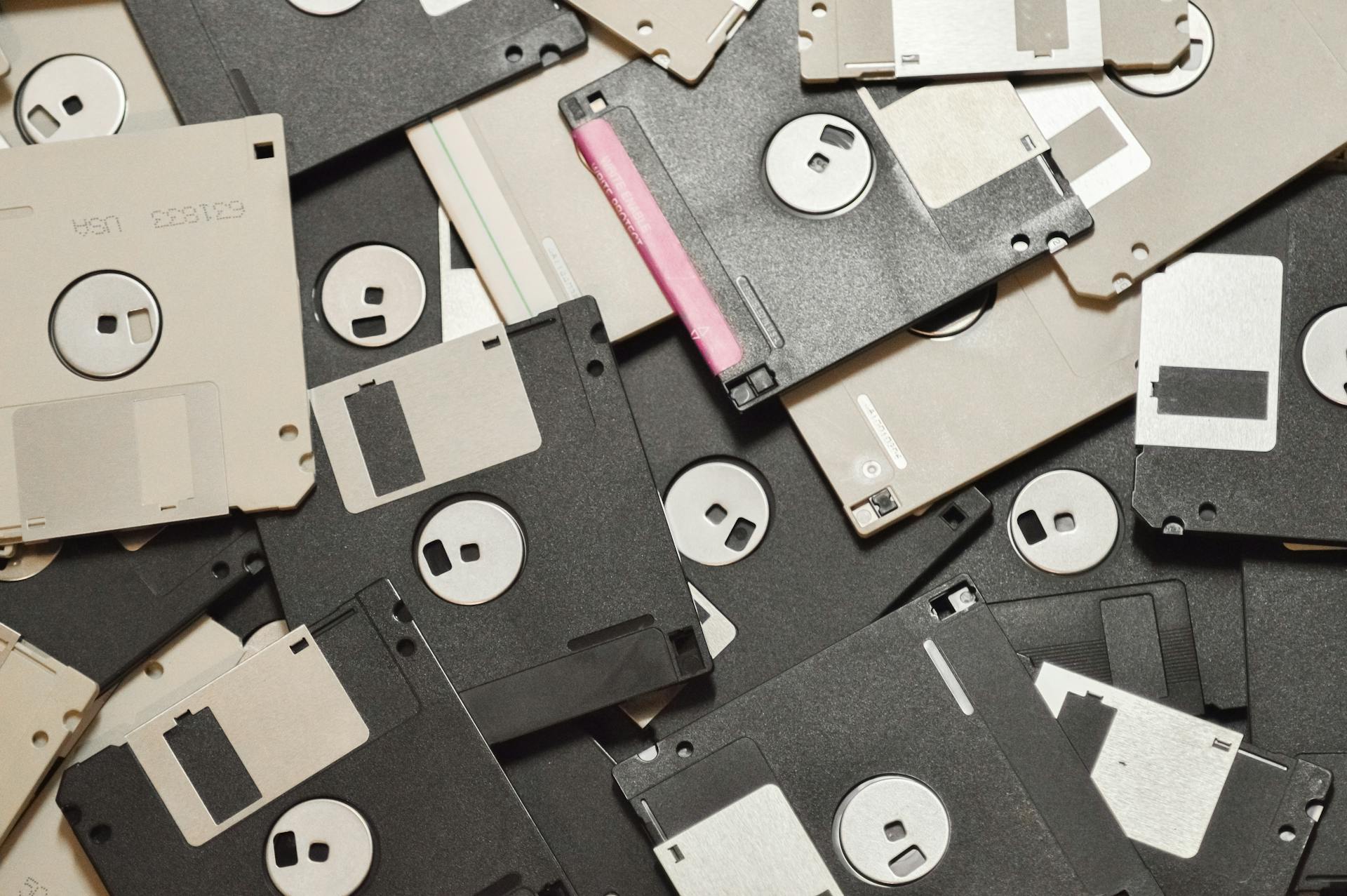
Enabling S3 bucket versioning with version control is a straightforward process using Terraform.
To start, you need to create an S3 bucket, which can be done using the `aws_s3_bucket` resource in Terraform. This resource creates a new S3 bucket with the specified name and configuration.
The `versioning` block is used to enable versioning on the S3 bucket. This block has a single attribute, `enabled`, which is set to `true` to enable versioning.
Versioning must be enabled before uploading any objects to the S3 bucket.
Broaden your view: Aws S3 Bucket Public Access Block
Write Terraform Configuration
To write Terraform configuration for an S3 bucket, you'll need to create a file called s3.tf. In this file, you'll define the AWS provider and the S3 bucket resource. The bucket attribute will give the bucket a globally unique name.
The first step is to initialize Terraform, which will install the AWS provider. This is necessary to create AWS resources.
To define the AWS provider and S3 bucket resource, you'll use the following code in the s3.tf file:
```
provider "aws" {
region = "your-region"
}
resource "aws_s3_bucket" "example" {
bucket = "example-bucket"
}
```
Replace "your-region" with the region where you want to create the bucket, and "example-bucket" with the desired name of your bucket.
Once you've defined the provider and the bucket resource, you'll need to create the S3 bucket by running `terraform apply` and typing yes at the prompt. This will output the aws_s3_bucket resource details, showing the new S3 bucket that was created.
S3 Bucket Setup
To set up an S3 bucket with Terraform, you'll need to create an AWS S3 bucket resource in your Terraform configuration file. This can be done by writing the required configurations to create S3 buckets in the AWS infrastructure using the Terraform code.
To enable versioning on the S3 bucket, you'll need to update the s3.tf file with an aws_s3_bucket_versioning resource. This resource takes a versioning configuration block, and by setting status = "Enabled", you'll enable versioning on the linked S3 bucket.
A fresh viewpoint: Enable Sspr Azure
Here are the steps to create an S3 bucket using Terraform:
1. Create an AWS S3 bucket resource in your Terraform configuration file.
2. Initialize the Terraform configuration by running `terraform init` in your terminal or command prompt.
3. Apply the Terraform configuration by running `terraform apply` and confirming the changes.
4. Verify that the S3 bucket was created correctly and versioning is enabled by checking the S3 console.
To enable versioning for a Terraform-created bucket, you can define an S3 bucket resource with Versioning enabled in your Terraform configuration file. For a bucket created through the AWS Management Console, you'll need to import the existing bucket into your Terraform configuration and then enable Versioning.
A fresh viewpoint: S3 Console Aws
Create S3 Bucket
To create an S3 bucket, you'll need to write the required configurations in your Terraform code. This will allow you to create the bucket in the AWS infrastructure.
First, define an S3 bucket resource with the desired name, replacing "my-terraform-bucket-27" with your chosen name. Open your terminal or command prompt and navigate to the directory containing the Terraform configuration file.
Worth a look: S3 Bucket Naming
You can then initialize the Terraform configuration by running terraform init. After that, apply the Terraform configuration by running terraform apply, confirming the changes and typing "yes" to apply them.
By following these steps, you'll be able to create an S3 bucket using Terraform and set the stage for further configuration and management.
S3 Bucket Overview
An S3 bucket is a cloud storage resource provided by AWS that allows you to store and retrieve objects. It's essentially a container for your data.
S3 buckets are incredibly versatile, and you can store any type of data in them, from images and videos to text files and even executable files.
An S3 bucket has a unique identifier called a bucket name, which is used to access and manage the bucket and its contents.
Here are some key facts about S3 buckets:
- Each S3 bucket has a unique DNS name and can be accessed via the internet
- S3 buckets can be used to store data of any size, from small files to large datasets
Versioning is a feature of S3 buckets that allows you to preserve, retrieve, and restore every version of an object stored inside it.
Enable S3 Bucket Versioning
S3 bucket versioning allows you to preserve, retrieve and restore every version of an object stored inside it. With versioning enabled, when an object is updated or deleted, the old version is preserved as a new object version.
Multiple variants of an object are saved, allowing easy rollback. This provides protection from accidental or unintended updates and deletes.
To enable versioning, you can use Terraform. Update the s3.tf file to create an aws_s3_bucket_versioning resource that takes a versioning configuration block. Set status = "Enabled" to enable versioning on the linked S3 bucket.
Versioning state can be Enabled, Suspended or Disabled. Integrating with S3 lifecycle rules allows for automated cleanup. You can also delete individual object versions.
Here are some key benefits of S3 bucket versioning:
You can also verify versioning configuration by checking the S3 console. Go to the S3 service, click on the created bucket, go to the Properties tab, and check the Versioning card. This will confirm that versioning is truly enabled.
A unique perspective: Aws Versioning S3
Versioning Configuration
To enable versioning on an S3 bucket, you'll need to create an aws_s3_bucket_versioning resource in your Terraform configuration file. This resource takes a versioning configuration block, which you can set to "Enabled" to turn on versioning.
By setting status = "Enabled", you'll configure an S3 bucket with versioning turned on as you defined.
To verify that versioning is enabled, head to the S3 service on your AWS console, click on the created bucket, go to the Properties tab, and check the Versioning card. As you can see, versioning shows as Enabled, confirming your Terraform configuration worked.
Here are the steps to follow:
- Go to the S3 service on your AWS console
- Click on the created bucket
- Go to the Properties tab
- Check the Versioning card
Now that versioning is enabled, whenever an object in the bucket gets modified, the previous version will be automatically preserved as an older variant. This is a crucial data protection capability that you can leverage to recover from a disaster or file corruption by reverting to a previous version.
AWS S3 bucket versioning allows you to keep a historical record of all the modifications done to any of the files in the bucket. This is especially useful for state files, where it's better to recover from a previous version than to rebuild the state files manually.
To demonstrate enabling versioning through Terraform, you can follow these steps:
- Define an S3 bucket resource with Versioning enabled in your Terraform configuration file
- Initialize the Terraform configuration by running `terraform init`
- Apply the Terraform configuration by running `terraform apply`
By following these steps, you'll have successfully enabled versioning for both Terraform-created and Console-created buckets using Terraform.
Terraform S3 Backend Best Practices
When using AWS S3 buckets as the remote backend for Terraform operations, it's essential to follow some best practices to ensure the security and integrity of your data.
Encryption is a must-have, as it protects your data from unauthorized access.
Access Control should also be implemented to restrict access to your S3 bucket, only allowing authorized users to make changes.
Versioning is another crucial aspect, as it allows you to keep a record of all changes made to your Terraform state file.
Locking is also important, as it prevents concurrent modifications to your Terraform state file.
Using the Backend First approach ensures that your Terraform state file is stored in the remote backend, rather than locally.
Here are the Terraform S3 backend best practices summarized in a list:
- Encryption
- Access Control
- Versioning
- Locking
- Backend First
Frequently Asked Questions
How do I know if my S3 bucket has object versioning enabled?
To check if your S3 bucket has object versioning enabled, navigate to the Properties tab and look for the Versioning status in the Versioning section. If it's enabled, you'll see a status indicating that versioning is turned on.
Sources
- https://linuxhaxor.net/code/enable-versioning-s3-bucket-terraform.html
- https://developer.hashicorp.com/terraform/language/backend/s3
- https://spacelift.io/blog/terraform-s3-backend
- https://www.easydeploy.io/blog/s3-bucket-aws-terraform-cli-command/
- https://medium.com/@mohasina.clt/step-by-step-guide-to-enabling-versioning-in-amazon-s3-buckets-3c10f8156742
Featured Images: pexels.com