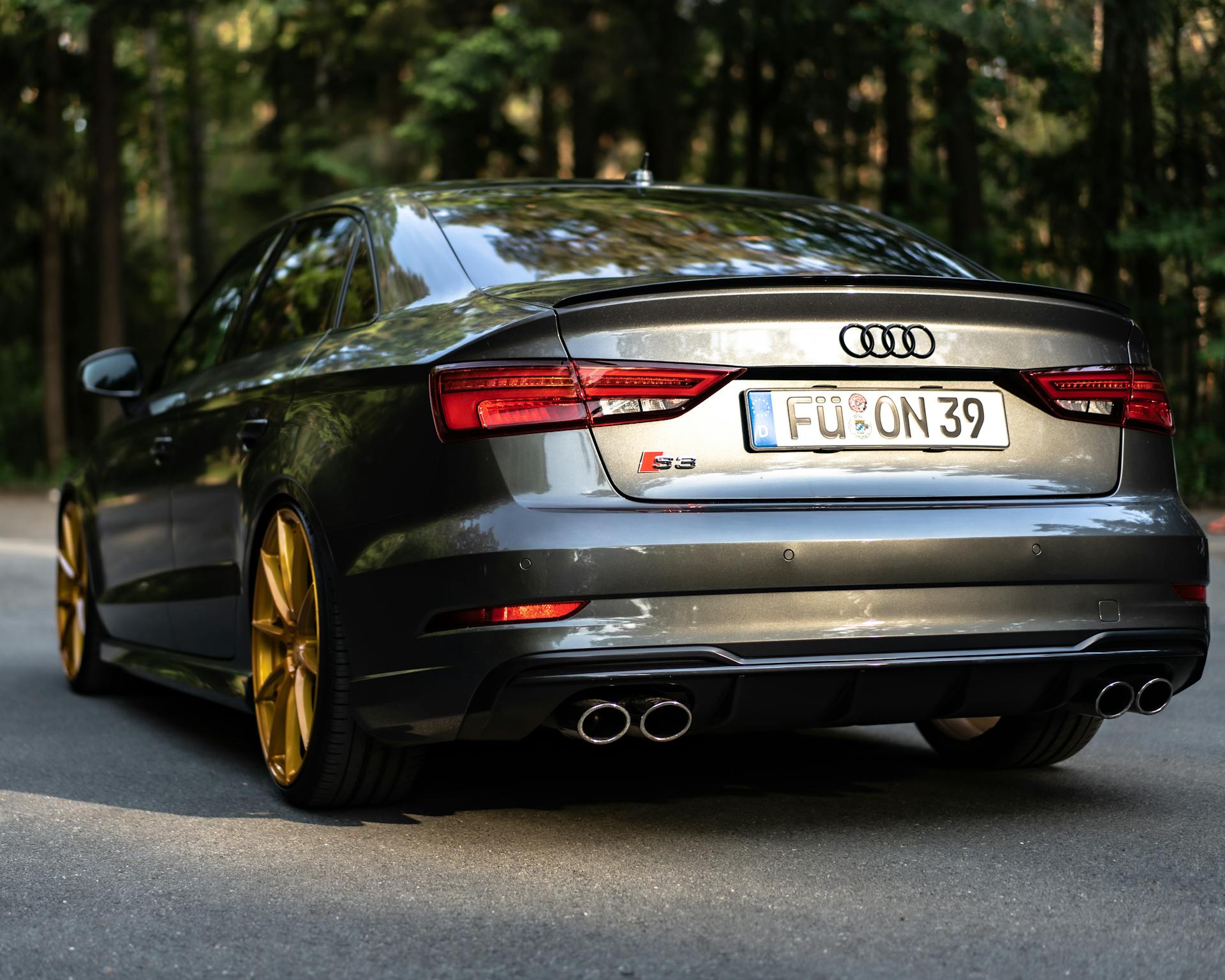
Uploading files to Amazon S3 using the AWS CLI is a straightforward process, but it requires a few key steps. First, you need to install the AWS CLI on your machine and configure it with your AWS credentials.
To upload a file to S3, you can use the `aws s3 cp` command, which copies a file from your local machine to an S3 bucket. For example, if you have a file named `example.txt` in your current directory and you want to upload it to an S3 bucket named `my-bucket`, you can use the command `aws s3 cp example.txt s3://my-bucket/`.
The AWS CLI also allows you to upload files to S3 in parallel, which can speed up the upload process for large files. This is especially useful when uploading multiple files to S3 at the same time.
On a similar theme: Aws S3 Cp Multiple Files
Prerequisites
To get started with AWS CLI S3 upload, you'll need to meet some basic prerequisites.
You'll need an AWS account, which you can sign up for with the AWS Free Tier if you don't already have one.
An AWS S3 bucket is also required, and it's recommended to create an empty bucket for this purpose.
You'll need a Windows 10 computer with at least Windows PowerShell 5.1, although PowerShell 7.0.2 will be used in this article.
The AWS CLI version 2 tool must be installed on your computer.
You'll also need local folders and files that you'll be uploading or synchronizing with Amazon S3.
Here are the specific requirements for local directories and files:
- Local directory and files located under c:\sync.
Uploading Files
Uploading Files is a straightforward process using the AWS CLI. You can use the `aws s3 cp` command to upload files to your S3 bucket.
To get started, you'll need to create a data object from scratch or use the data from an existing file. This data object will then be uploaded to the appropriate bucket.
Explore further: Aws S3 Delete Object
You'll need to specify the bucket's name and the path to the file on your local system. You can also change the uploaded S3 object name during the upload operation if needed.
One thing to keep in mind is that you can specify the S3 storage class during upload. The supported parameters for the `--storage-class` argument are:
- STANDARD – default, Amazon S3 Standard
- REDUCED_REDUNDANCY – Amazon S3 Reduced Redundancy Storage
- STANDARD_IA – Amazon S3 Standard-Infrequent Access
- ONEZONE-IA – Amazon S3 One Zone-Infrequent Access
- INTELLIGENT_TIERING – Amazon S3 Intelligent-Tiering
- GLACIER – Amazon S3 Glacier
- DEEP_ARCHIVE – Amazon S3 Glacier Deep Archive
If you need to encrypt the file with default SSE encryption, you'll need to provide the `--sse` argument.
Managing Objects
Managing Objects with AWS CLI S3 Upload is a breeze. You can achieve the same result by using the aws s3 sync command, which supports the same arguments for setting up the S3 storage class and encryption.
To upload files recursively to the S3 bucket, you need to use either the aws s3 cp command with the --recursive argument or the aws s3 sync command. The aws s3 sync command will upload only changed files from your local file system at the next execution.
A unique perspective: Processing Large S3 Files with Aws Lambda
You can use the --delete argument to delete objects from the S3 bucket if they were deleted on your local file system. This is especially useful for maintaining a synchronized copy of your files between your local system and the S3 bucket.
Here are the supported parameters for the --storage-class argument:
- STANDARD – default, Amazon S3 Standard
- REDUCED_REDUNDANCY – Amazon S3 Reduced Redundancy Storage
- STANDARD_IA – Amazon S3 Standard-Infrequent Access
- ONEZONE-IA – Amazon S3 One Zone-Infrequent Access
- INTELLIGENT_TIERING – Amazon S3 Intelligent-Tiering
- GLACIER – Amazon S3 Glacier
- DEEP_ARCHIVE – Amazon S3 Glacier Deep Archive
Creating a Directory
Creating an empty S3 "directory" is a bit different than creating a regular directory on your local machine. To create an empty S3 "directory" using AWS CLI, you need to use the aws s3 put-object command.
The / character in the object name is required to create an empty directory. Otherwise, the command will create a file object with the name directory_name.
For example, if you want to create an empty S3 "directory" called "mydirectory", you would use the following command: aws s3 put-object --bucket mybucket --key mydirectory/.
Suggestion: S3 Command Line Aws
Managing Objects
Managing objects in S3 is a crucial part of managing your data in the cloud. You can achieve the same result by using the aws s3 sync command, which supports the same arguments for setting up the S3 storage class and encryption.
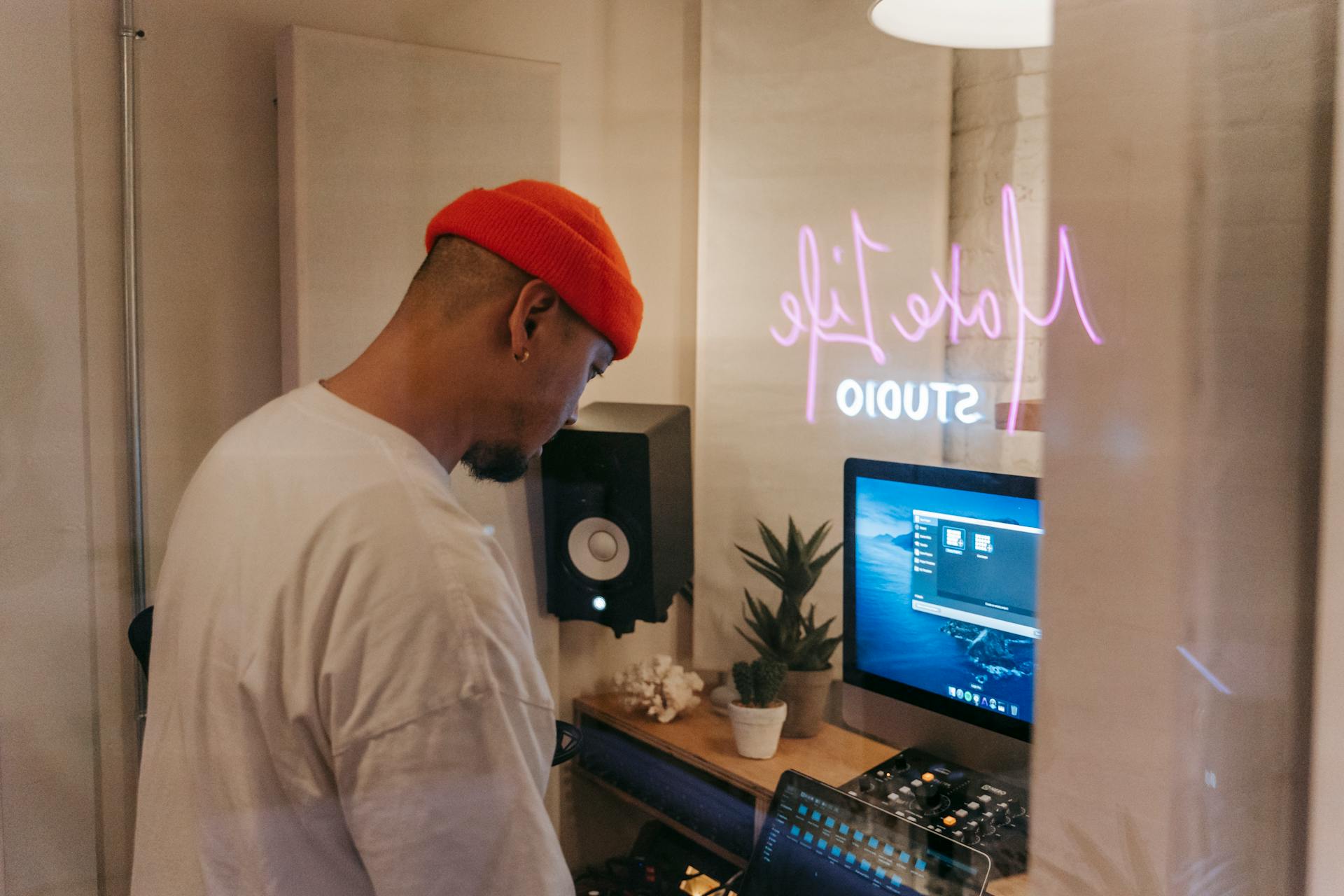
You can upload multiple files and folders to S3 recursively using the aws s3 cp command with the --recursive argument. This command will upload all files and subdirectories within the specified directory.
To upload multiple files and folders to S3 selectively, you can use the --include and --exclude options with the aws s3 cp command. For example, you can include only files with specific extensions, such as *.ps1.
Here are some common use cases for uploading files to S3:
- Uploading multiple files to S3: `aws s3 cp --recursive /local/file/path s3://bucket-name`
- Uploading multiple files with specific extensions: `aws s3 cp --include *.ps1 --exclude *.txt /local/file/path s3://bucket-name`
- Uploading a single file to S3: `aws s3 cp /local/file/path s3://bucket-name`
You can also use the aws s3 sync command to upload only changed files from your local file system to S3. This command will upload only the files that have been modified since the last sync operation.
The aws s3 sync command also supports the --delete option, which will delete objects from the S3 bucket if they were deleted on your local file system. This is useful for keeping the contents of an S3 bucket updated and synchronized with a local directory on a server.
Curious to learn more? Check out: Aws S3 Delete Bucket Cli
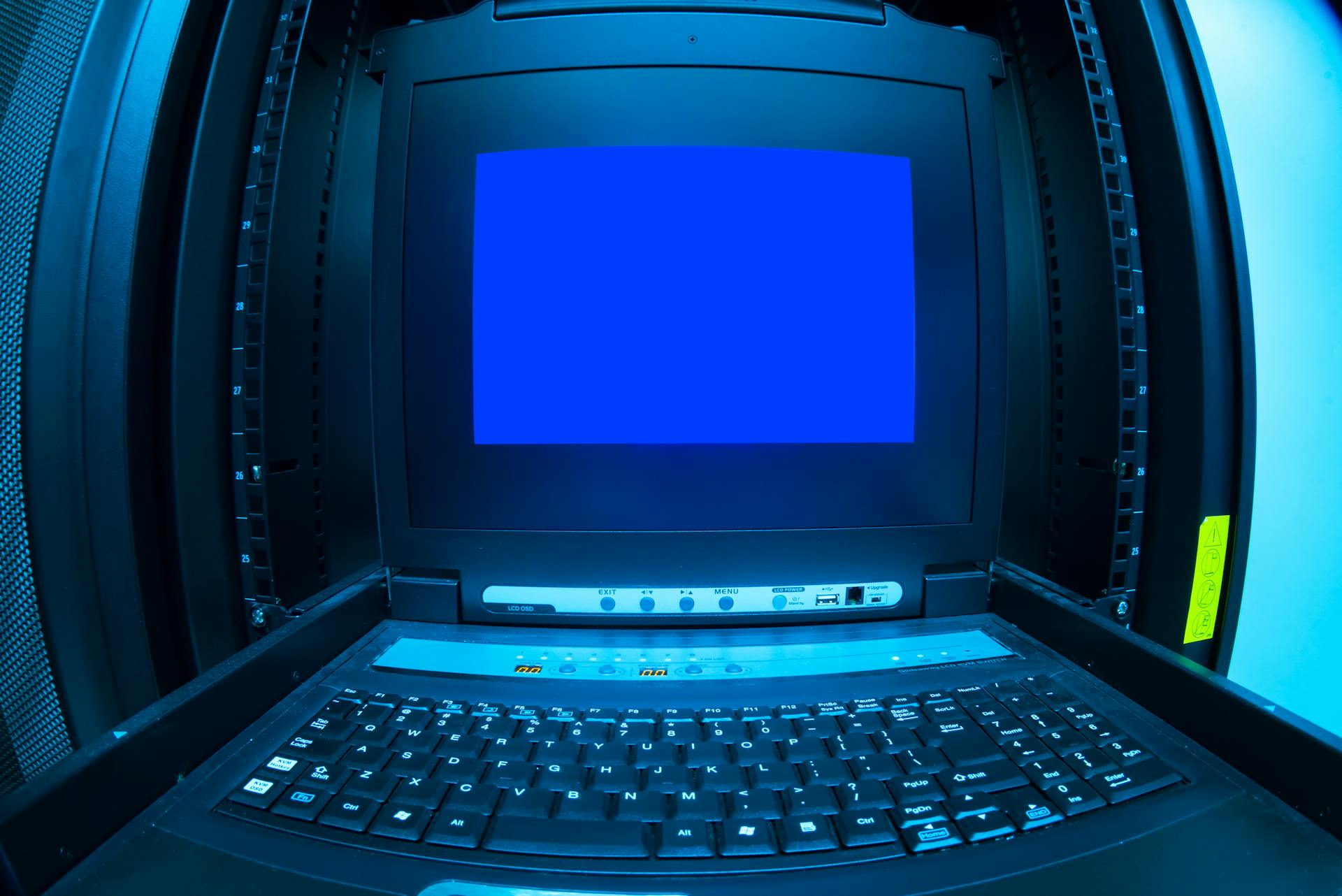
Here are some examples of using the aws s3 sync command:
- Uploading changed files to S3: `aws s3 sync /local/file/path s3://bucket-name`
- Deleting objects from S3: `aws s3 sync --delete /local/file/path s3://bucket-name`
In summary, managing objects in S3 is a powerful tool for managing your data in the cloud. By using the aws s3 cp and aws s3 sync commands, you can upload and synchronize files between your local file system and S3.
Commands and Tools
You can use AWS CLI S3 commands to manage S3 in scripts or CICD automation pipelines. This allows for automation of S3 operations.
For example, you can configure a Jenkins pipeline to execute an AWS CLI command for any AWS account in your environment. This makes it easier to manage multiple accounts.
AWS CLI S3 commands are useful for automating S3 operations, and can be used in scripts or pipelines to streamline your workflow.
For your interest: Aws S3 Batch Operations
Commands
You can use AWS CLI S3 commands to automate S3 operations using scripts or in your CICD automation pipeline.
For instance, you can configure the Jenkins pipeline to execute the AWS CLI command for any AWS account in your environment. This makes it easier to manage your S3 bucket and objects programmatically.
The AWS CLI S3 commands are usually used for managing S3 when automation is needed.
Readers also liked: Aws Cloudshell S3 Commands
Tools Required
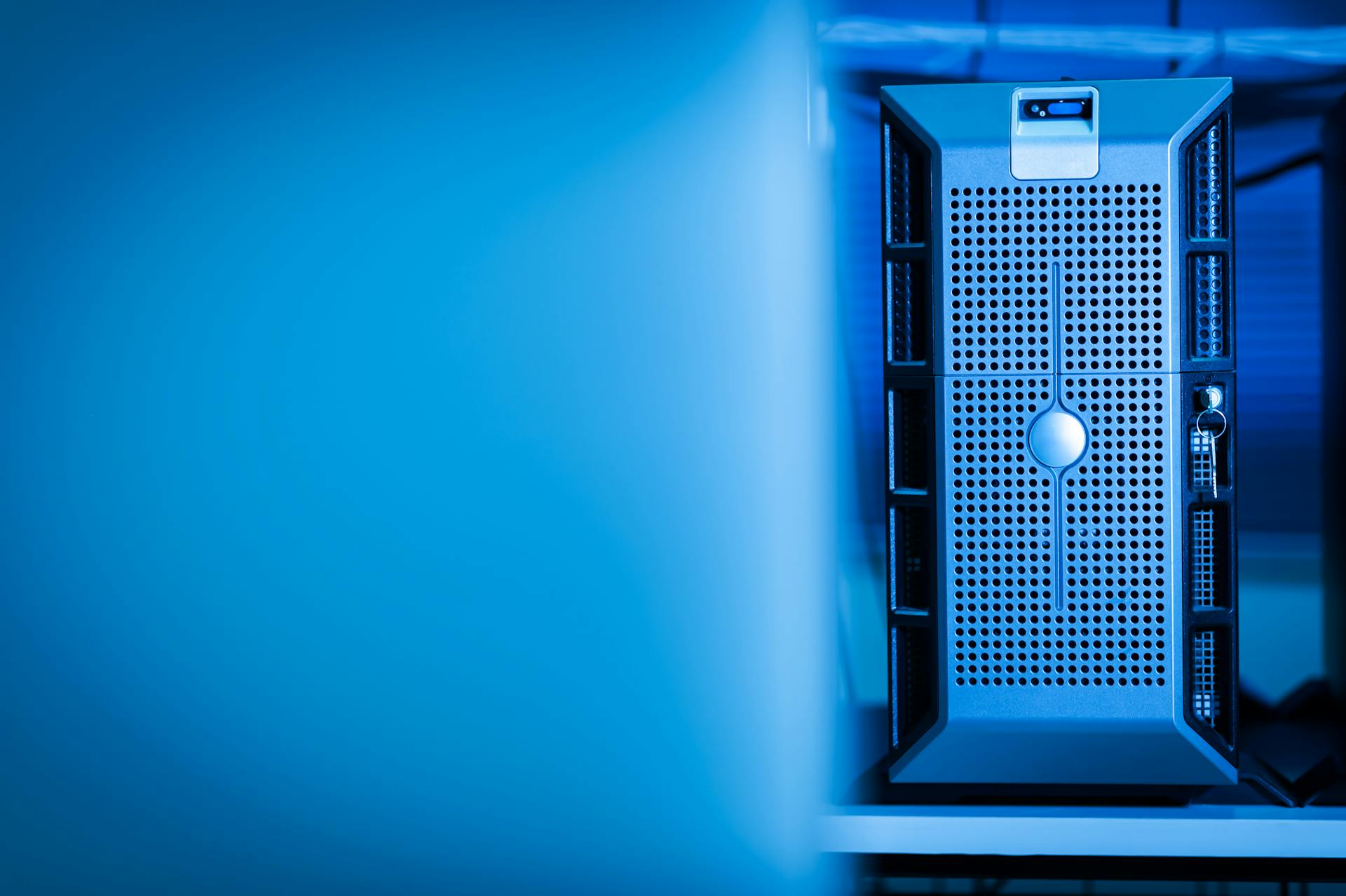
To get started with using AWS CLI, you'll need a few essential tools.
You'll need an AWS account, which you can create on the AWS website.
First, make sure you have Python installed on your system, as the AWS CLI depends on it.
To use the AWS CLI, you'll also need to have it configured properly. This can be done using the AWS CLI itself.
Here are the specific tools you'll need to get started:
- AWS CLI configured
- AWS account
- Python Installed (AWS CLI depends on it)
To download and install the AWS CLI, you can visit the AWS website and select your operating system.
Common Use Cases
Backing up your important data is a top priority, and using the AWS CLI to store it securely in Amazon S3 is a great way to ensure data backup and recovery.
You can use the CLI to upload files to S3 and generate temporary access links to share documents and media with external users. This makes it easy to collaborate with others without compromising your data's security.
For another approach, see: Aws Data Pipeline S3 Athena
Serving static content for websites is another common use case for uploading files to S3 using the CLI. Hosting static assets like HTML, CSS, and media files on S3 can provide efficient content delivery for websites.
Here are some common use cases for uploading files to S3 using the CLI:
- Backing up Data
- Sharing Files with Others
- Serving Static Content for Websites
- Hosting Images and Videos
Frequently Asked Questions
How to connect S3 using AWS CLI?
To connect S3 using AWS CLI, you need to install and configure the AWS CLI with a profile that has the necessary permissions. See Installing, updating, and uninstalling the AWS CLI and Authentication and access credentials for the AWS CLI for more information.
Sources
- https://www.educative.io/answers/how-to-create-an-s3-bucket-and-upload-objects-using-aws-cli
- https://adamtheautomator.com/upload-file-to-s3/
- https://www.geeksforgeeks.org/how-to-upload-files-to-aws-s3-using-cli/
- https://hands-on.cloud/aws-cli-s3-examples/
- https://intellipaat.com/blog/aws-s3-cli-commands/
Featured Images: pexels.com