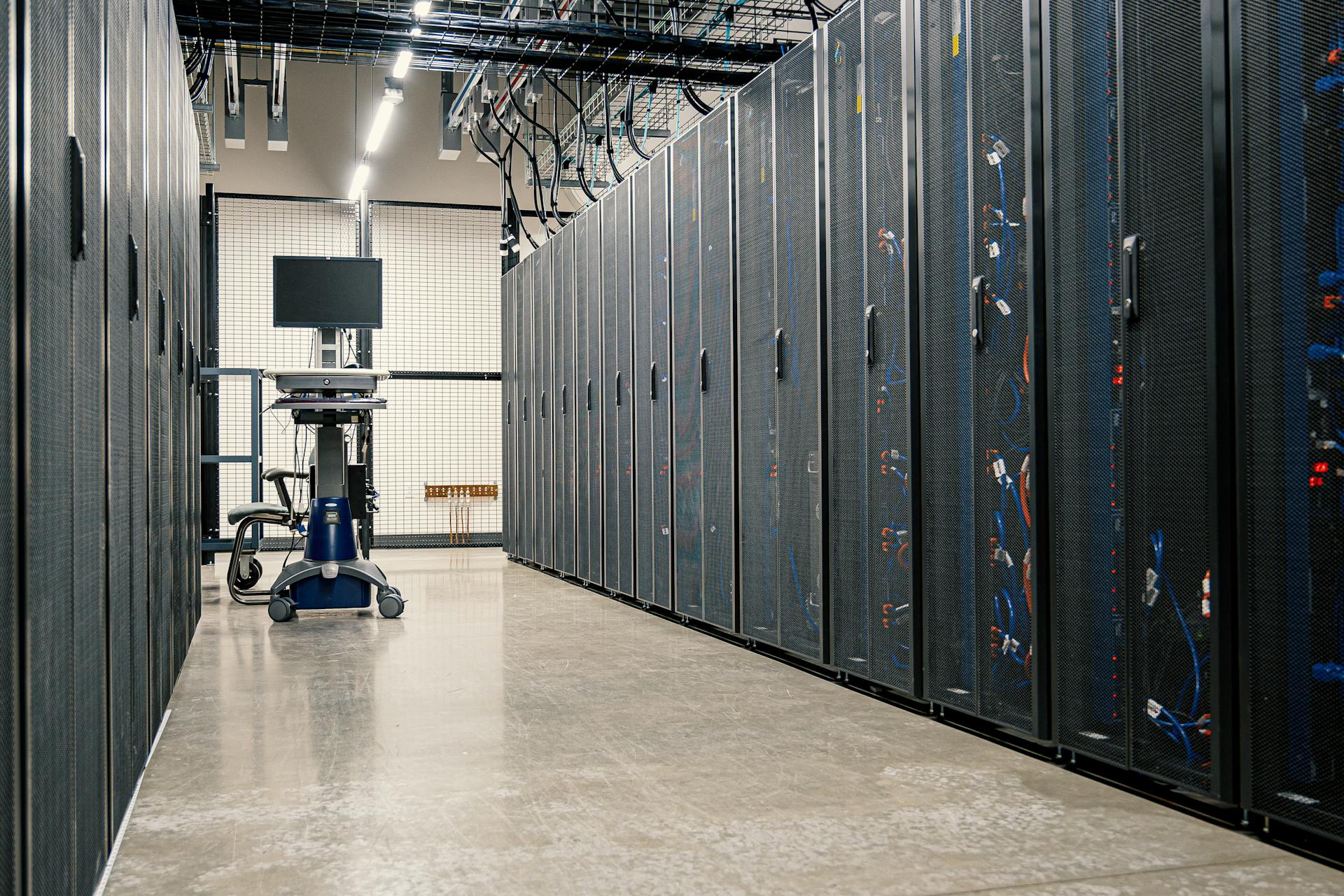
Building serverless apps with AWS DynamoDB, Lambda, S3, and Next.js is a game-changer for developers.
With AWS DynamoDB, you can store and manage large amounts of data in a fully managed NoSQL database service.
This setup allows for seamless scalability and high performance, making it perfect for modern web applications.
Using AWS Lambda, you can run serverless code in response to events, eliminating the need for a traditional server.
AWS S3 is a great storage solution for serving static assets, and when combined with Next.js, you can create fast and secure web applications.
This combination of services provides a powerful and flexible way to build scalable web applications.
If this caught your attention, see: Aws S3 Api Gateway Lambda Dynamodb
Setting Up AWS
To set up AWS, you'll need to create an account if you don't already have one. You can do this by going to the AWS Console and following the sign-up process.
First, log in to the AWS Console. If you don't have an AWS account, create one here.
A different take: Aws S3 Create Bucket
Once logged in, navigate to the S3 service in the AWS Console. This is where you'll be hosting your static assets like images and JavaScript files.
To create a new bucket, click the "Create bucket" button and follow the steps to create a new bucket.
Before you start building your serverless solution, make sure you have the following prerequisites:
- AWS Account: This is essential for accessing and utilizing the AWS services mentioned in this article.
- AWS IAM: Understand the basics of AWS Identity and Access Management (IAM) for managing user permissions and roles within the AWS environment.
- Knowledge of AWS Lambda: Familiarize yourself with AWS Lambda, as it will be used to write the serverless functions for this application.
- Basic knowledge of Python: Read and write simple statements with Python programming language, as the Lambda functions in this tutorial will be written in Python.
- Understanding of REST APIs: Have a basic understanding of REST (Representational State Transfer) APIs and their fundamental principles, including the HTTP methods such as GET, POST, and DELETE.
Working with S3
Working with S3 is a crucial part of the AWS DynamoDB Lambda S3 workflow. To set up an S3 bucket, log in to the AWS Console and navigate to the S3 service. From there, create a new bucket by clicking the "Create bucket" button and follow the steps to configure your bucket settings, including region and access permissions.
You can also create a new S3 bucket by navigating to the AWS Console Management > AWS S3, selecting the region where you want to host your web application, and clicking on Create bucket. Once the bucket is created, you can upload your web application files by clicking on your bucket and selecting the Upload option.
To upload your static assets, you can use the AWS CLI, SDKs, or even the AWS Console web interface. Make sure to replace the path to your static assets and the name of your S3 bucket with the actual values.
You might enjoy: Aws Glue Create Table from S3
Setting Up a Bucket
To set up a bucket, you need to log in to the AWS Console. If you don't have an AWS account, you can create one here.
Once logged in, navigate to the S3 service in the AWS Console. From there, you can create a new S3 bucket by clicking on the "Create bucket" button.
Provide a unique name for your bucket, which should be globally unique within the entire AWS S3 namespace. This ensures that there are no naming conflicts with existing buckets.
Select the region where you want to host your web application. Then click on Create bucket. Your bucket is now created and ready for use.
You can upload your web application files by clicking on your bucket and selecting the Upload option, then Add files.
A different take: Aws Cli Create S3 Bucket
Storing Static Assets
Storing Static Assets is a straightforward process with S3. You can use the AWS CLI to upload your static assets.
Make sure to replace the path to your assets with the actual file location on your computer, just like replacing "my-assets/*" with your own path.
You can also use the AWS Console web interface to upload your static assets.
Create and Upload CSV
To create a CSV file, you'll need to create a .csv file with the desired data.
You can create a .csv file with the following data.
Create a new .csv file with the data you want to upload to S3.
Once you have your .csv file ready, you can upload it to an S3 bucket.
To do this, navigate to the AWS Console Management > AWS S3, and create a new S3 bucket.
You can also upload the file programmatically using a boto3 session and an S3 client.
Here's a step-by-step guide to uploading a CSV file to an S3 bucket:
1. Create a new S3 bucket and navigate to it.
2. Click on the "Upload" option and select "Add files" to upload your CSV file.
3. Make sure to block public access to the bucket for security purposes.
4. Use the S3 client to initiate an upload of your CSV file.
By following these steps, you can successfully upload your CSV file to an S3 bucket.
A different take: Apache Airflow Aws Data Pipeline S3 Athena
Serverless Functions
Serverless Functions are a great way to run code without provisioning or managing servers, making it an excellent choice for implementing serverless functions in your NEXT.js application.
You can create a Lambda function to perform tasks like reading data from S3 and ingesting it into DynamoDB. This requires attaching an IAM role to the Lambda function to access S3 and DynamoDB programmatically.
The default python runtime environment of Lambda does not come with Pandas, so you'll need to import it separately. This is a good opportunity to use your S3 client to get the object and read the CSV file contents into a dataframe.
If you're loading a lot of data at a time, you can use DynamoDB.Table.batch_writer() to speed up the process and reduce the number of write requests made to the service. This can significantly improve the speed of data ingestion, depending on the provisioned read/write capacity units.
A unique perspective: Serverless Aws S3 Api Gateway Upload Portal
Working with DynamoDB
To interact with DynamoDB, you can use the AWS SDK as well. Here's an example of how to put an item into a DynamoDB table.
DynamoDB is a managed NoSQL database service provided by AWS, highly scalable and can handle large amounts of data with low latency.
To set up a DynamoDB table for your application, navigate to DynamoDB in the AWS Console and you'll have a table ready to store data in no time.
To create a DynamoDB table, navigate to DynamoDB: In the AWS Console, go to the DynamoDB service.
Here's a quick rundown of the steps to create a DynamoDB table: Navigate to DynamoDB: In the AWS Console, go to the DynamoDB service.
Take a look at this: Aws Data Pipeline S3 Athena
NoSQL Database
DynamoDB is a managed NoSQL database service provided by AWS, highly scalable and capable of handling large amounts of data with low latency.
To get started with DynamoDB, navigate to the DynamoDB service in the AWS Console. This will get you set up with a DynamoDB table ready to store data for your application.
You can create a DynamoDB table using the create_table() function, which takes specific arguments. There are two examples of this: one with a primary key only and another with a primary key and sort key.
A fresh viewpoint: Aws S3 Service Control Policy
Read CSV to Table
To read CSV files into a DynamoDB table, you'll need to create a Lambda function. This function will be responsible for fetching the CSV file from an S3 bucket and pushing its contents into the DynamoDB table.
First, you'll need to create a Lambda function in the AWS Management Console. Select "Author from Scratch" and choose Python as the runtime environment. Attach the IAM role you created earlier to the function, allowing it to access both S3 and DynamoDB programmatically.
To read the CSV file from S3, you'll use the S3 client's `get_object()` function, passing in the bucket name and CSV filename as parameters. This will fetch the CSV file contents into the `Body` field of the response.
You can then use the DynamoDB client's `put_item()` function to push each row of the CSV file into the DynamoDB table. This is done by traversing through the list of CSV file contents and picking elements one by one to insert into the table.
On a similar theme: Invoke Aws Lambda Function Sam with S3 Trigger
Here's a step-by-step guide to creating the Lambda function:
- Create a Lambda function in the AWS Management Console.
- Select "Author from Scratch" and choose Python as the runtime environment.
- Attach the IAM role you created earlier to the function, allowing it to access both S3 and DynamoDB programmatically.
- Use the S3 client's `get_object()` function to fetch the CSV file contents from S3.
- Use the DynamoDB client's `put_item()` function to push each row of the CSV file into the DynamoDB table.
Frequently Asked Questions
Does DynamoDB use S3?
DynamoDB integrates with S3, allowing you to export data to S3 for analysis with AWS services. You can also import data from S3 into DynamoDB.
Can Lambda connect to S3?
Yes, Lambda can connect to S3, but you need to create an IAM role that grants access to both the Lambda function and the S3 bucket. This allows secure interaction between your Lambda function and S3 resources.
Sources
- https://clouddevs.com/next/s3-lambda-and-dynamodb-integratio/
- https://medium.com/@xinweiiiii/export-aws-dynamodb-data-to-s3-on-a-recurring-basis-using-lambda-198d290c8e64
- https://www.dheeraj3choudhary.com/aws-lambda-csv-s3-dynamodb-automation/
- https://medium.com/analytics-vidhya/bulk-data-ingestion-from-s3-into-dynamodb-via-aws-lambda-b5bdc30bd5cd
- https://numericaideas.com/blog/aws-serverless-web-application/
Featured Images: pexels.com