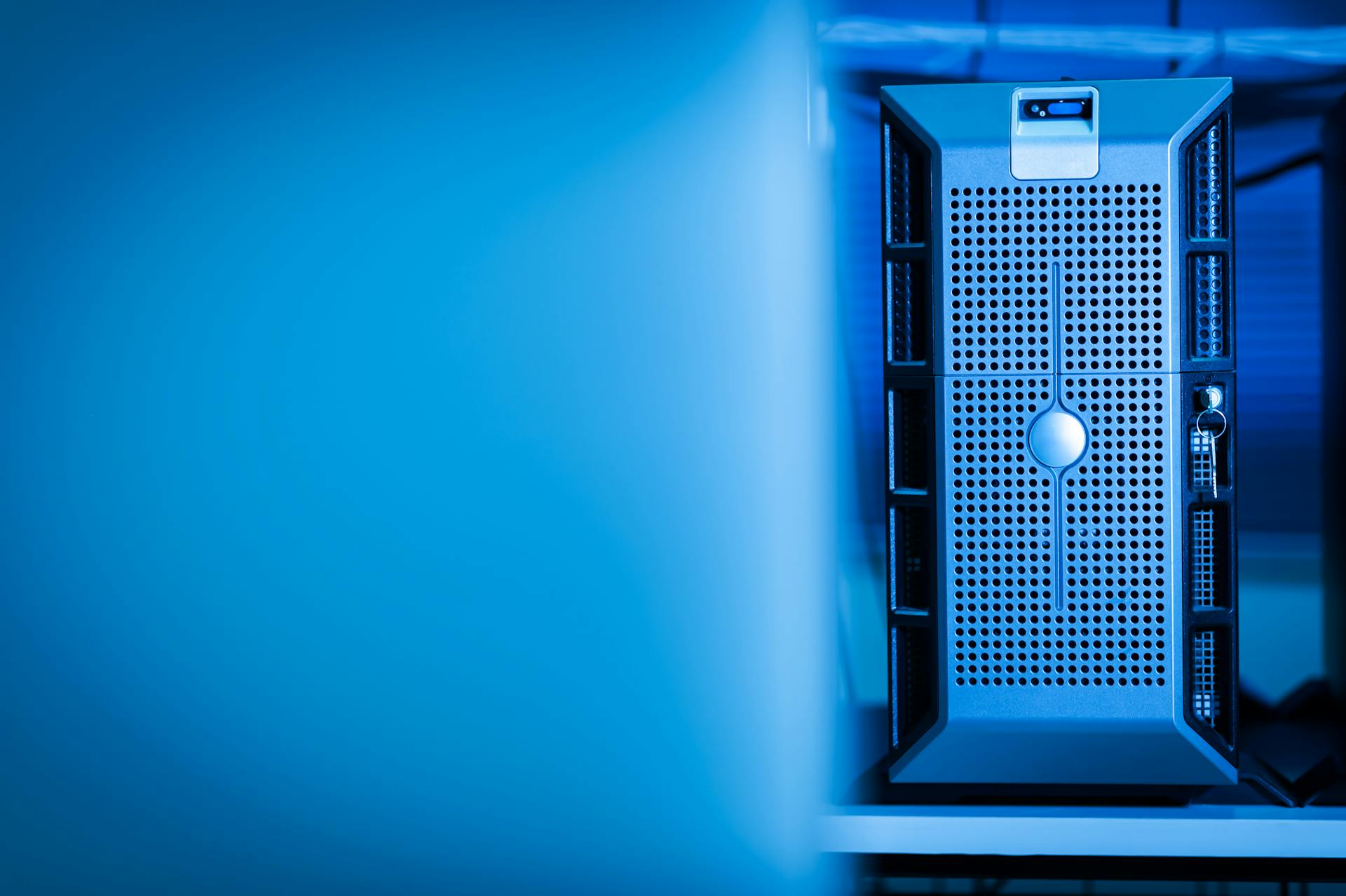
AWS S3 is an object storage service that can be used as a data source for your serverless application. It allows you to store and serve large amounts of data.
API Gateway is a fully managed service that can handle up to 10,000 concurrent API calls per second. This makes it a great choice for building scalable serverless applications.
Lambda is a serverless compute service that can be triggered by API Gateway. It allows you to run code without provisioning or managing servers.
Explore further: Aws S3 Service Control Policy
Prerequisites
To get started with our AWS S3 API Gateway Lambda DynamoDB project, you'll need to meet some prerequisites.
First and foremost, you'll need to create an AWS account to access the necessary services.
You should also have a basic understanding of AWS Identity and Access Management (IAM) for managing user permissions and roles within the AWS environment.
Familiarize yourself with AWS Lambda, as it will be used to write the serverless functions for this application.
To write the Lambda functions, you'll need to have a basic knowledge of Python programming language.
You'll also need to have a basic understanding of REST APIs, including the HTTP methods such as GET, POST, and DELETE, which will be used to interact with the API Gateway.
Here are the specific prerequisites you'll need to have:
- AWS Account
- AWS IAM
- AWS Lambda
- Basic knowledge of Python
- Understanding of REST APIs
Additionally, make sure you have the latest copy of the AWS CLI, SAM CLI, and Python 3 installed on your development machine.
Solutions Architecture
Serverless architecture is a cloud computing paradigm that eliminates the need for managing servers and infrastructure, allowing developers to focus solely on writing code.
In this model, applications are built using small, stateless functions that are triggered by events, executed in a managed environment, and automatically scale based on demand.
Serverless architecture offers benefits such as cost efficiency, scalability, reduced operational overhead, and rapid development.
To build our serverless web application, we used the following AWS services:
- Amazon S3 (Simple Storage Service): a highly scalable and durable cloud storage service that allows you to store and retrieve large amounts of data.
- AWS Lambda: a serverless compute service that allows you to run your code without provisioning or managing servers.
- DynamoDB: a fully managed NoSQL database service that delivers seamless and scalable performance for applications that require low-latency, consistent, and highly available data storage.
- AWS API Gateway: a fully managed service that allows you to create, publish, and manage APIs for your applications.
- CloudFront: a content delivery network (CDN) service that enables the efficient distribution of your content to end users across the globe.
- AWS WAF (Web Application Firewall): a cloud-based firewall service that helps protect your web applications from common web exploits and attacks.
These services work together seamlessly to provide a robust and scalable solution for our web application.
Setting Up S3 Bucket
To set up an S3 bucket, simply log in to your AWS console and navigate to the AWS S3 page. From there, you can create a new S3 bucket by clicking on Create bucket.
Choose a unique name for your bucket, as it must be globally unique within the entire AWS S3 namespace to avoid naming conflicts with existing buckets. Select the region where you want to host your web application.
Once the bucket is created, you can upload your web application files by clicking on your bucket and selecting the Upload option. From there, you can add files from your local machine by selecting them and clicking on Upload.
For another approach, see: Create S3 Bucket Aws Cli
Configure CloudFront and WAF
To start, navigate to the AWS Console and click on Management > CloudFront to create a new CloudFront distribution. Click on Create Distribution to begin the process.
In the Create Distribution interface, select the previously created S3 bucket from the Origin Domain field. This will ensure that the CloudFront distribution is connected to your S3 bucket.
You might enjoy: Create S3 Bucket Aws
Under Origin Access, select Origin access control setting to ensure the bucket is only accessible via the CloudFront distribution. This is an important security measure to protect your S3 bucket.
In the Default Root Object section, enter the name of your main HTML file, in our case, index.html. This will set the default page that users will see when they visit your website.
Next, navigate to the S3 service and select the bucket containing your website. Go to Permissions and click on Edit to update the bucket policy.
Delete the existing content in the Bucket Policy section and paste the policy copied from the CloudFront distribution. This policy will grant CloudFront access to your S3 bucket.
Click on Save Changes to apply the updated policy to your S3 bucket. Wait a few minutes for your distribution to deploy, and then copy the generated domain name.
See what others are reading: Aws S3 Select
IAM Roles and Permissions
To set up IAM roles and permissions for your Lambda functions, click on Roles in the left sidebar and then Create role. You should select the AmazonDynamoDBFullAccess policy for DynamoDB permissions.
For production usage, it's essential to apply the Least Privilege Principle, which means not giving unnecessary permissions like DynamoDB full access, but only the precise actions your Lambda functions need. This will enforce a better security of your infrastructure.
You can create a new IAM role by clicking on Create role and then selecting the policy, in this case, AmazonDynamoDBFullAccess. After selecting the policy, click on Next to proceed.
To verify the chosen policy, you'll need to enter a role name and review the policy details. Make sure to review the policy carefully before clicking on Create role.
Worth a look: Aws Glue Create Table from S3 Example
Functions
In a serverless architecture, your application can be split into multiple smaller functions, each with its own specific task, which can be executed independently.
To create a Lambda function, you can navigate to the AWS Management Console > AWS Lambda, click on create a function, and select Python 3.9 as the runtime. You can also create a function with a name like insertEmployee and select a previously created role.
A unique perspective: Invoke Aws Lambda Function Sam with S3 Trigger
A Lambda function can be created with a name, an IAM Role with permissions to access your DynamoDB table, and an environment variable for the table name. You can also define a policy for the function by creating an IAM Policy statement and attaching it to the function's role.
Here's a breakdown of the properties you can pass to Lambda Construct:
- code: the folder's path to find the code to run when the Lambda is triggered.
- handler: the entry file and function to run inside this file.
- runtime: the platform needed for this Lambda function, like Node.js or Python.
- initialPolicy: the permissions that this Lambda can access.
These properties can be used to create a Lambda function that can talk to DynamoDB, like in our example where we'll just have a single Lambda function that talks to DynamoDB.
For your interest: Aws Dynamodb Lambda S3
Design Your Application
Designing your application is the first step in building a serverless architecture, and it's essential to have a clear understanding of how your application's different components will fit together.
Your application can be split into multiple smaller functions, each with its own specific task, which can be executed independently. This process is called microservice design.
Identify the things your app needs to do, group them into functions, and understand how they talk to each other. This will help you create a clear and organized design for your application.
In our example, we'll just have a single Lambda function that talks to DynamoDB. This will allow us to focus on the AWS side of things and keep things simple.
Create Functions
Creating functions is a crucial step in building a serverless application. You can create a Lambda function in the AWS Management Console by clicking on "Create a function" in the AWS Lambda dashboard.
To create a function, you'll need to give it a name, select a runtime, and choose an IAM role with the necessary permissions. For example, to create a Lambda function that interacts with DynamoDB, you'll need to select a role that has permissions to access your DynamoDB table.
You can also create a function using the AWS CLI or SDKs. However, for this example, we'll focus on creating a function in the AWS Management Console.
Here are the key steps to create a Lambda function:
- Name: Give your function a unique name.
- Runtimes: Choose from a variety of runtimes, such as Python 3.9 or Node.js 14.
- IAM Role: Select a role that has the necessary permissions for your function.
- Code: Enter your code in the Code tab and click on Deploy.
In some cases, you may need to attach an inline policy to your function's role to define its permissions. This can be done by creating a policy statement and attaching it to the function's role.
For example, to attach a policy to access S3, you can create a policy statement and attach it to the function's role. This will add the necessary permissions to your function to access S3 resources.
By following these steps, you can create a Lambda function that interacts with other AWS services, such as DynamoDB.
API Gateway
API Gateway is a crucial component of our application, enabling us to create a RESTful API that interacts with our Lambda functions and DynamoDB table.
To implement API Gateway, navigate to the API Gateway service from the AWS Management Console. Create a method to retrieve employees saved in our DynamoDB table by selecting the GET method, choosing Lambda Function as the Integration type, and entering the name of the previously created Lambda function. Click Save to complete this step.
A GET method is used to retrieve data, and in our case, it's used to fetch employees from DynamoDB.
The deleteEmployee function requires a method of type DELETE.
For proper communication between the API Gateway and CloudFront, we need to configure CORS (Cross-Origin Resource Sharing). This is necessary because these two services reside in different domains. To enable CORS, go back to the Resources tab, click on Action, and then Enable CORS.
Enabling CORS allows the API Gateway to handle requests from different origins, preventing CORS origin errors.
Here are the steps to deploy our API:
1. Click on Action, then Deploy API.
2. Select New stage and enter the stage name.
3. Deploy the API.
After deploying our API, we need to redeploy it to apply the changes made to the CORS configuration.
The API Gateway Event Source for Lambda allows us to trigger a Lambda function when an HTTP(S) request is made to the API Gateway. This is achieved through the API Gateway trigger for the Lambda.
In our application, the API Gateway trigger for the Lambda is used to invoke a Lambda function when an end-user makes an HTTP(S) request via the RESTful API exposed by the API Gateway.
Expand your knowledge: Aws S3 Cors Configuration
The event triggered by the API Gateway contains details about the HTTP request that is received.
Here are the HTTP methods that can be used to interact with our API:
- GET (DynamoDB get)
- POST (put)
- PUT (update)
- DELETE (delete)
- SCAN (scan)
Each of these methods corresponds to a different Lambda function handler, written in Node.js.
For example, the getMessage handler function accepts two inputs: a path parameter, the date, which is the primary partition key for the DynamoDB table, and a query parameter, the time, which is the primary sort key for the DynamoDB table.
The Lambda code for the getMessage handler function can be seen below.
```html
exports.getMessage = async (event, context) => {
if (tableName == null) {
tableName = process.env.TABLE_NAME;
}
params = {
TableName: tableName,
Key: {
"date": event.pathParameters.date,
"time": event.queryStringParameters.time
}
};
console.debug(params.Key);
return await new Promise((resolve, reject) => {
docClient.get(params, (error, data) => {
if (error) {
console.error(`getMessage ERROR=${error.stack}`);
resolve({
statusCode: 400,
error: `Could not get messages: ${error.stack}`
});
} else {
console.info(`getMessage data=${JSON.stringify(data)}`);
resolve({
statusCode: 200,
body: JSON.stringify(data)
});
}
});
});
};
```
Here's an interesting read: Apache Airflow Aws Data Pipeline S3 Athena
Serverless Application
A serverless application is a great way to build scalable and cost-effective systems. In a serverless architecture, your application can be split into multiple smaller functions, each with its own specific task, which can be executed independently.
Recommended read: Serverless Aws S3 Api Gateway Upload Portal
This approach eliminates the need for server management, reducing operations work and making it easier to scale. Serverless applications are also more expensive per request, but the final bill can come out cheaper due to zero unused capacity.
Here are some key points about serverless vs serverful architectures:
- Operations: In serverless you don't manage the servers, so there's way less ops work.
- Scalability: It's pretty easy to make serverful scale with an Auto Scaling Group, but there's always a delay in scaling.
- Cost: Serverless is more expensive per request, but can be cheaper overall due to zero unused capacity.
- Development speed: Serverless development is faster since AWS takes care of server management.
- Developer experience: Serverless developers typically like the infrastructure work being pushed to them.
- Optimization: There's usually a lot to optimize in serverless applications.
Demonstration
In a serverless application, events trigger functions to execute specific tasks. We'll build an application that extracts messages from CSV files in S3 and processes them through Lambda functions.
To demonstrate this, we'll deploy an application that uses API Gateway to expose a RESTful API for CRUD-like operations on messages in DynamoDB. This API will allow us to perform operations on the messages stored in DynamoDB.
The application will be built using Lambda functions to extract messages from CSV files, transform them, and queue them to SQS. SQS will then write the messages to DynamoDB.
We'll also review the attributes of an individual item within the table in DynamoDB, which should match the rows in the CSV file.
Consider reading: Aws Architecture Athena Query Csv Table Stored S3
Steps to Create a Serverless Application
To create a serverless application, you'll need to follow these steps.
First, design your application by identifying the tasks it needs to perform and grouping them into functions. This process is called microservice design.
Next, choose your AWS services, such as Lambda, SQS, DynamoDB, and API Gateway, to build your application.
Create a Lambda function by giving it a name, an IAM role with permissions to access your DynamoDB table, and putting your code in.
For your Lambda function, use an existing role and choose the previously created role.
Create your Lambda function by giving it a name, an IAM Role with permissions to access your DynamoDB table, put the table name in an environment variable, and put your code in.
Use the SAM CLI to validate, build, package, and deploy your project. You can use the following commands to do this:
Once you've deployed your project, use the CloudFormation management console to review the AWS resources created by SAM.
Finally, host your static website on S3 and use CloudFront for HTTPS and as a CDN.
Security
Security is a top priority when building an AWS S3 API Gateway Lambda DynamoDB application. Set up authentication using API Gateway to keep your API endpoints private, and consider using Amazon Cognito or a custom authorizer.
To add an extra layer of protection, use AWS Web Application Firewall (WAF) in your API Gateway APIs. This will help filter out malicious traffic and prevent common web attacks.
Data encryption is also crucial. Both S3 and DynamoDB encrypt data by default, but you can change the configuration to use a key you manage. This gives you more control over your encryption keys.
For data in transit, DynamoDB already encrypts data, but for your static website in S3, set up CloudFront with an SSL certificate. This will ensure that data is encrypted when it's transmitted between the client and server.
To implement least privilege access, give your Lambda functions an IAM Role that only grants them the permissions they need. For example, you can give the role read permissions on table1, instead of granting it permissions on all DynamoDB resources.
Worth a look: Aws S3 Encryption
Here are some key security best practices to keep in mind:
- Encrypt data at rest using a key you manage.
- Encrypt data in transit using CloudFront with an SSL certificate.
- Implement least privilege access using IAM Roles.
- Use AWS Web Application Firewall (WAF) in your API Gateway APIs.
- Set up authentication using API Gateway or Amazon Cognito.
Operational Excellence
Operational Excellence is key to a smooth-running AWS S3 API Gateway Lambda DynamoDB system. Always use Infrastructure as Code, which can be achieved through tools like AWS SAM, CloudFormation, or the Serverless Framework.
This approach ensures consistency and reproducibility in your environment. For instance, you can use Lambda functions triggered by S3 events to process uploaded files in the background, or use SQS to queue up messages for later processing by Lambda functions.
Monitoring your app is also crucial. Use CloudWatch to track your app's metrics, logs, and alarms, and set up X-Ray to identify bottlenecks and errors in your application. By doing so, you'll be able to pinpoint issues and make data-driven decisions to improve your system's performance.
Readers also liked: Aws S3 Upload File App Typescript
Operational Excellence
Operational Excellence is all about streamlining your workflow to get the most out of your resources. Always use Infrastructure as Code to manage your serverless solutions, and consider using AWS SAM or the Serverless Framework to get started.
Using asynchronous processing is a game-changer for scalability and cost-effectiveness. You can use Lambda functions triggered by S3 events to process uploaded files in the background, or use SQS to queue up messages for later processing by Lambda functions.
Monitoring your app is crucial to identify bottlenecks and errors. Use CloudWatch to track your app's metrics, logs, and alarms, and set up X-Ray to trace requests through your application.
Automation is key to efficient deployment and testing. Use a CI/CD pipeline for each function, and stick to the same practices as for microservices, even if your Lambdas aren't microservices.
Here are some key tools to consider for Operational Excellence:
- AWS SAM for Infrastructure as Code
- Serverless Framework for declarative infrastructure management
- CloudWatch for monitoring app metrics and logs
- X-Ray for tracing application requests
- CI/CD pipelines for automated deployment and testing
Serverless Applications Best Practices
Always use Infrastructure as Code to manage your serverless applications, and consider using AWS SAM or the Serverless Framework for declarative options.
Using asynchronous processing can improve scalability and reduce costs by handling tasks that don't require real-time execution, such as processing uploaded files in the background with Lambda functions triggered by S3 events.
Consider reading: Processing Large S3 Files with Aws Lambda
CloudWatch is essential for monitoring your app's metrics, logs, and alarms, while X-Ray helps identify bottlenecks and errors by tracing requests through your application.
A CI/CD pipeline is crucial for automating deployment and testing, even for serverless applications, to ensure smooth and efficient delivery of your functions.
Here are some key best practices to keep in mind:
- Use asynchronous processing to handle tasks that don't require real-time execution.
- Monitor your app's metrics, logs, and alarms with CloudWatch.
- Use X-Ray to identify bottlenecks and errors in your application.
- Automate deployment and testing with a CI/CD pipeline.
Reliability and Performance
Reliability is key when building scalable applications on AWS. You can implement retries and circuit breakers to recover from network errors or service throttling. Use randomized exponential backoff for retries and circuit breakers to prevent failures from cascading.
To deploy and test new versions without disrupting your production environment, use Lambda versions. You can create multiple versions of your Lambda functions and point API Gateway routes to a specific version. This allows you to roll back if needed.
Here are some key reliability and performance strategies to keep in mind:
- Implement retries and circuit breakers
- Use Lambda versions
- Use canary releases
- Back up DynamoDB
Reliability
Reliability is a top priority when building scalable and fault-tolerant applications. Implementing retries and circuit breakers can help you recover from network errors or service throttling. Randomized exponential backoff is a good approach for retries.
You can create versions of your Lambda functions and point API Gateway routes to a specific version. This allows you to deploy and test a new version without disrupting your production environment.
Versions also enable canary releases, where API Gateway sends a small part of the traffic to the new version. This way, you can test it with real data without impacting all of your users.
DynamoDB backups are essential to protect against data loss. Use point-in-time recovery to restore the data if it's lost.
Intriguing read: Aws Data Pipeline S3 Athena
Performance Efficiency
Performance efficiency is a crucial aspect of ensuring your application runs smoothly and efficiently.
To reduce latency and improve performance, consider adding caches, such as using CloudFront to cache your static website and API Gateway responses.
Optimizing database access is also key, and using indexes whenever needed can make a big difference. Always use query instead of scan, and don't fetch all attributes if you don't need them.
Understanding how DynamoDB scales is essential to making the most of its capabilities. You can read more about it in the article "Understanding how DynamoDB scales."
Right-sizing your Lambdas is also important - if they're too small, they'll run slow, and if they're too big, they'll get super expensive. You can use a profiling tool to determine how much memory your Lambda needs, but often it's simpler to just try different values until you find the sweet spot.
Minimizing response payload can also improve response times - sending less data in the response can make a big difference. If you have multiple use cases for the data, consider creating a new endpoint for the summary.
Optimizing cold starts is another important consideration - Lambda functions have a few instances running, and launch more when needed. The time for a new Lambda instance to launch is called cold start, and you can optimize it by putting initialization code outside the handler function.
Here are some key strategies for improving performance efficiency:
- Use caching to reduce latency and improve performance
- Optimize database access by using indexes and minimizing attribute fetching
- Right-size your Lambdas to avoid slow performance and high costs
- Minimize response payload to improve response times
- Optimize cold starts by putting initialization code outside the handler function
Sources
- https://numericaideas.com/blog/aws-serverless-web-application/
- https://newsletter.simpleaws.dev/p/serverless-application-aws-lambda-dynamodb
- https://programmaticponderings.com/2019/10/04/event-driven-serverless-architectures-with-aws-lambda-sqs-dynamodb-and-api-gateway/
- https://www.pluralsight.com/resources/blog/guides/building-a-serverless-web-app-on-aws-services
- https://tkssharma.com/deploy-lambda-with-s3-dynamo-and-api-gateway-cdk-part-4/
Featured Images: pexels.com