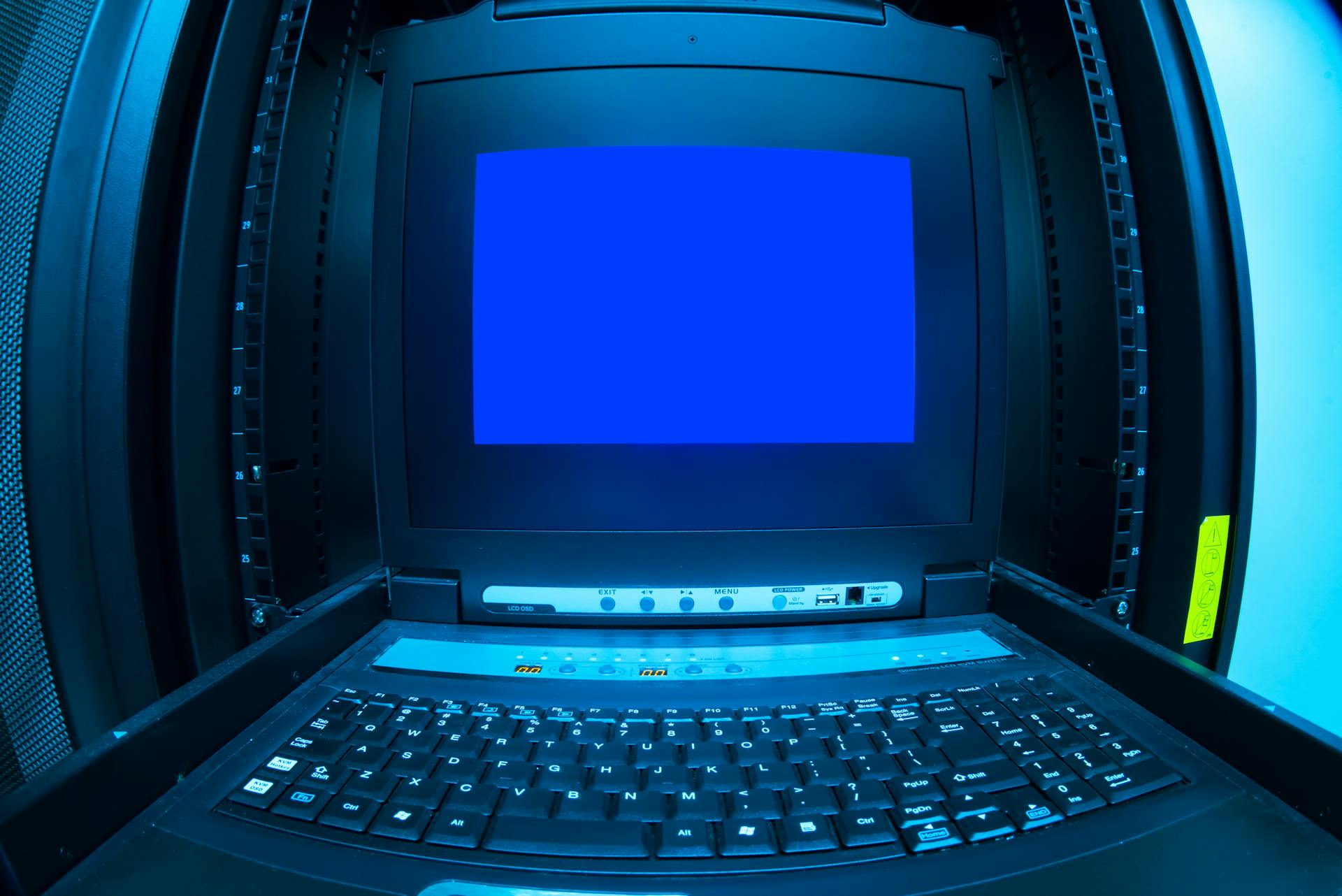
As a developer, you're likely familiar with the importance of storing and managing files in the cloud. AWS S3 is a popular and reliable solution for this purpose, and integrating it into your application can be a game-changer.
To get started with uploading files to AWS S3 using TypeScript, you'll need to install the AWS SDK for JavaScript, which includes the necessary libraries for interacting with S3. This can be done using npm or yarn with the command npm install aws-sdk.
The AWS S3 upload process involves creating an S3 client, specifying the bucket and file to upload, and then using the putObject method to upload the file. This process can be handled asynchronously to ensure a smooth user experience.
For example, a simple upload operation might look like this: const s3 = new AWS.S3({ accessKeyId: 'YOUR_ACCESS_KEY_ID', secretAccessKey: 'YOUR_SECRET_ACCESS_KEY' }); const params = { Bucket: 'your-bucket-name', Key: 'your-file-key', Body: fs.createReadStream('path/to/your/file') }; s3.upload(params, (err, data) => { if (err) console.log(err); else console.log(data); });
Curious to learn more? Check out: S3 Console Aws
Prerequisites
To get started with building an AWS S3 upload file app in TypeScript, you'll need to have a solid foundation in some key areas.
First and foremost, you'll need to have basic knowledge of HTML. This will come in handy when working with the front-end of your app.
Additionally, you'll need to have Node.js and the Express.js framework set up on your machine. These tools will help you build the back-end of your app.
It's also essential to have an AWS account with an active S3 bucket. You can find clear steps for setting up your AWS account and creating an S3 bucket in the AWS documentation.
Here are the specific prerequisites you'll need to get started:
- Basic HTML
- The Node.js server
- The Express.js framework
Setting Up S3 Bucket
To set up an S3 bucket, you'll need to log in to your AWS account and navigate to the S3 console. This is the first step in creating a container for storing objects like files in the Amazon S3 cloud.
Click on the “Create bucket” button to start the process. You'll then need to enter a unique name for your bucket, select a region, and choose the default settings for versioning, logging, and permissions.
Here are the key settings to consider when creating your S3 bucket:
- Unique bucket name: This should be a name that is easy to remember and unique across all of AWS.
- Region: Choose a region that is closest to your target audience to minimize latency.
- Versioning: This setting determines whether or not S3 will keep multiple versions of your files.
- Logging: This setting determines whether or not S3 will log changes to your files.
- Permissions: This setting determines who will have access to your files.
Once you've entered these settings, click on the “Create bucket” button to create the bucket.
See what others are reading: Create S3 Bucket Aws Cli
Uploading to S3
Uploading to S3 is a straightforward process that requires a few key steps. You'll need to import the necessary modules, including the Upload module from @aws-sdk/lib-storage and the S3Client from @aws-sdk/client-s3.
To configure the upload, you'll need your AWS credentials, which you can retrieve from the AWS website. You'll also need to confirm your bucket name and region in the S3 console.
The Upload module allows you to upload files in parts, and you'll need to configure it with options such as client, params, queueSize, and partSize. The client is the destination of the file, which in this case is an S3 bucket. You'll need to specify the bucket's region in the client.
Related reading: Aws S3 Cross Region Replication
The params object contains the name of the S3 bucket, the Key (that is, the file name), the access control list (ACL) that defines access to the data, and the Body (that is, the generated transform stream). You can also define the number of parts to be processed simultaneously with the queueSize option, and the size of each part that is processed with the partSize option.
If the upload is successful, the promise resolves with an object containing information about the uploaded file, including the status code, the number of upload attempts, and so on. The form instance emits a data event with the complete name and the returned data, which sends a successful response to the client.
Here are some best practices for uploading files to Amazon S3:
- Use Server-Side Encryption (SSE): Enable SSE to encrypt your files at rest, providing an additional layer of security.
- Set Content-Type: Explicitly set the Content-Type header for your files to ensure proper handling by browsers and applications.
- Use Transfer Acceleration: Leverage Amazon S3 Transfer Acceleration for faster file uploads.
File Upload
To upload a file to S3, you'll need to import the necessary modules, including the Upload module from @aws-sdk/lib-storage and the S3Client from @aws-sdk/client-s3. This will allow you to configure the upload with your AWS credentials, which you can retrieve from the AWS website.
A fresh viewpoint: Aws S3 Sdk
You'll also need to create a new instance of the Upload module and configure it with options such as the destination S3 bucket, file key, and access control list. The queueSize and partSize options can be set to control the number of parts to be processed simultaneously and the size of each part, respectively.
Here are some best practices to keep in mind when uploading files to S3:
- Use Server-Side Encryption (SSE) to encrypt your files at rest.
- Set the Content-Type header for your files to ensure proper handling by browsers and applications.
- Use Transfer Acceleration for faster file uploads.
Upload a File
To upload a file, you'll need to import the necessary modules, such as the Upload module from @aws-sdk/lib-storage and the S3Client from @aws-sdk/client-s3.
You'll also need your AWS credentials to configure the upload, which can be retrieved from the AWS website and confirmed in the S3 console.
To create a new instance of the Upload module, you'll configure it with options such as the client, params, queueSize, and partSize.
The client is the destination of the file, which in this case is an S3 bucket. You'll create a new instance of the S3Client and add your AWS credentials to configure it.
Readers also liked: Aws Glue Create Table from S3 Table Properties
The params object contains the name of the S3 bucket, the file name, the access control list, and the body of the file.
The queueSize and partSize options define the number of parts to be processed simultaneously and the size of each part, respectively.
If the upload is successful, the promise resolves with an object containing information about the uploaded file, including the status code, the number of upload attempts, and the file name.
If the upload is unsuccessful, it rejects the promise with an error message.
Here are the key options you'll need to configure for the Upload module:
- client: The S3Client instance with your AWS credentials
- params: The object containing the S3 bucket name, file name, access control list, and body
- queueSize: The number of parts to be processed simultaneously (default is 4)
- partSize: The size of each part (smallest size possible is 5MB)
Handling Form Events
Handling form events is crucial when working with file uploads. Events are things that happen in the system you are programming, which the system tells you about so your code can react to them.
You can specify how you want to handle these events using the form.on() method. The on() method accepts an event name and a listener function, which is triggered whenever the form emits the event.
Suggestion: Html Form File Upload
The form instance emits different events while processing a file, including an error event for errors in the parsing process, a file event when it receives a file/field pair, and a progress event after parsing each chunk of data.
You can control what happens when the program emits a particular event in the listener function. If the event is not handled, the request will sometimes timeout, like in an error event.
To handle these events, you can use the following event names:
- Error event for errors in the parsing process.
- File event when it receives a file/field pair.
- Progress event after parsing each chunk of data.
Another event you will listen for is the fileBegin event, which is emitted whenever a new file is detected in the upload stream.
See what others are reading: Aws Lambda S3 Put Event Example Typescript
File Upload Best Practices
When uploading files to a server, especially a cloud storage service like Amazon S3, security and efficiency are top priorities.
To ensure your files are properly encrypted, use Server-Side Encryption (SSE). This adds an extra layer of security to protect your files at rest.
A unique perspective: Aws S3 Security
Setting the Content-Type header for your files is crucial for proper handling by browsers and applications. This ensures that your files are displayed correctly and can be used as intended.
Leveraging Amazon S3 Transfer Acceleration can significantly speed up file uploads. This feature can greatly reduce the time it takes to upload large files.
Here are some key best practices to keep in mind:
- Use Server-Side Encryption (SSE)
- Set Content-Type
- Use Transfer Acceleration
Frequently Asked Questions
How to upload a file using Typescript?
To upload a file using Typescript, install the required packages with `npm install multer @types/multer multer-s3 @types/multer-s3 aws-sdk --save`. This will enable you to handle file uploads in your Typescript application.
Sources
- https://chatwithcloud.ai/aws-practical-examples/upload-file-to-s3-using-aws-s3client-typescript
- https://www.freecodecamp.org/news/how-to-upload-files-to-aws-s3-with-node/
- https://jaluwibowo.id/blog/en/upload-next-shadcn-to-aws-s3/
- https://saturncloud.io/blog/how-to-upload-a-file-to-amazon-s3-with-nodejs/
- https://dev.to/joaosczip/build-a-file-upload-service-with-nodejs-typescript-clean-architecture-and-aws-s3-3h9b
Featured Images: pexels.com