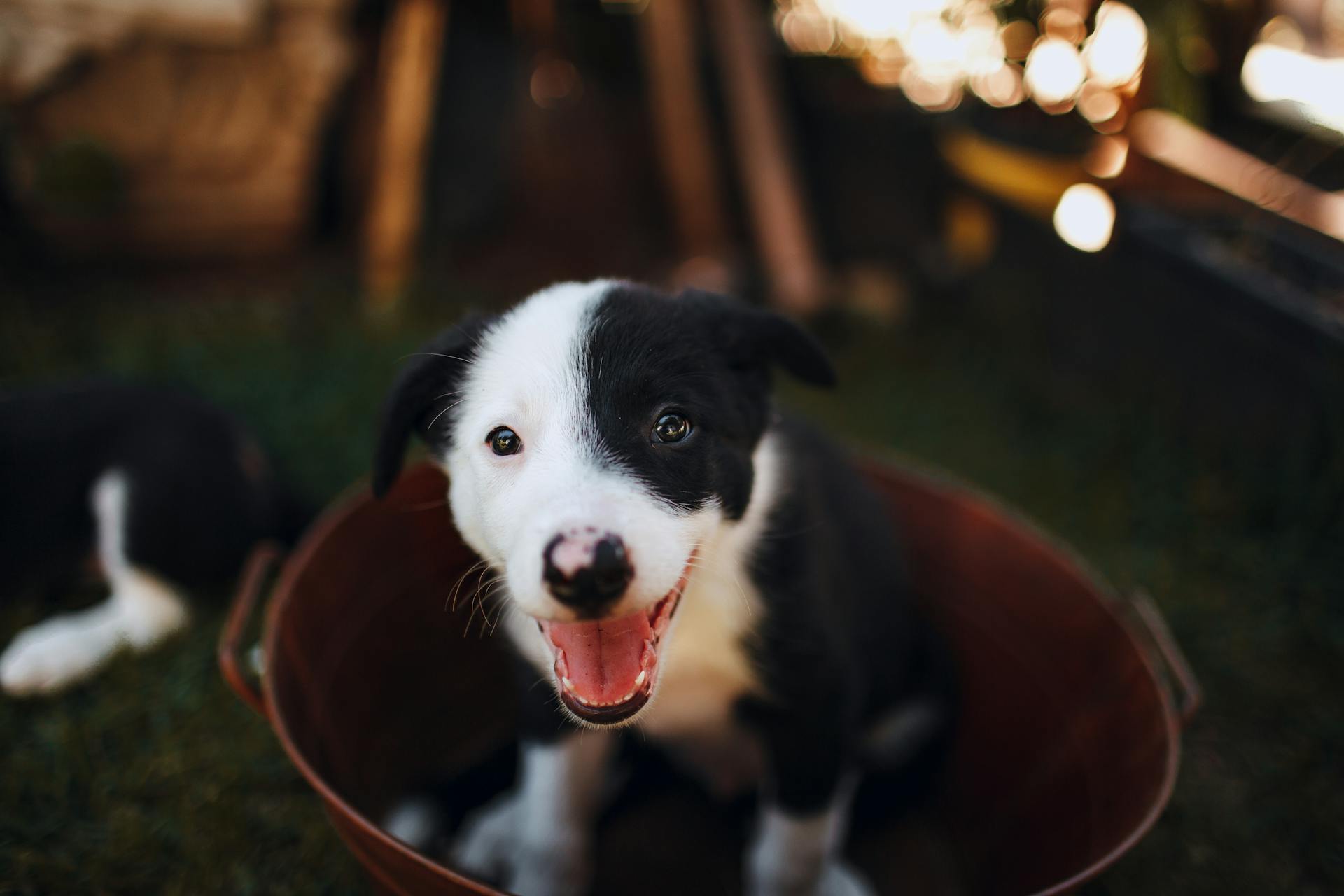
AWS S3 is a highly durable and available object store that can be used to store and serve large amounts of data.
It's designed to handle high traffic and can be used as a data lake or a static website host.
Data is stored in buckets, which can be organized into folders and subfolders for better management.
Each object in S3 is identified by a unique key, and versions of an object can be stored to track changes.
Here's an interesting read: Aws S3 Object
Getting Started
Getting Started with AWS S3 from R can be a bit overwhelming, but don't worry, we'll break it down step by step.
To start, you'll need to connect to S3 using the r2lambda::aws_connect function, which establishes an S3 service.
This is the first step in interacting with S3 from R, and it's essential to understand this process before moving on to more complex tasks.
You'll need to set some environmental variables to run the code, so be sure to check the Setup section in the {r2lambda} package readme for more details.
The example code used a local file, but you might wonder how to stream data directly to S3 without saving to file. The answer lies in serializing your data object before putting it in the bucket.
This means calling serialize with connection=NULL to generate a raw vector without writing to a file, which can then be put into your S3 bucket.
With these basics under your belt, you'll be well on your way to writing your Lambda function and interacting with S3 from R.
Readers also liked: Aws Upload File to S3 Api Gateway
S3 Configuration
To configure an Amazon S3 bucket, you need to select an AWS Region. This is a crucial step in setting up your bucket.
You'll also need to enter a unique bucket name to create a new bucket. This name will identify your bucket in the AWS Management Console.
Upon successful creation, you can see the list of buckets in the AWS Management Console.
Check this out: S3 Console Aws
Configure the
To configure your Amazon S3 bucket, start by selecting an AWS Region. This is a crucial step in creating a new bucket.
You'll also need to enter a unique bucket name to create a new bucket. This name will be used to identify your bucket in the AWS Management Console.
Upon successful creation, you can see the list of buckets in the AWS Management Console. This is where you can manage your bucket and its contents.
To access your bucket, go to the AWS Management Console and look for the list of buckets.
Worth a look: Aws S3 Bucket List
Creating a New
Creating a New S3 Bucket is Easy!
To create a new S3 bucket, you can use the put_bucket() function, which is surprisingly easy to use. You can create a bucket called "tiny-herbs" with just a few lines of code.
Selecting an AWS Region is a crucial step when creating a new bucket. You can choose from a list of available regions to create your bucket.
You can verify if your new bucket exists by using the bucket_exists() function. This function will check if the bucket is present in the specified region.
Additional reading: Aws S3 Cross Region Replication
If you've created a new bucket, you can use the get_bucket_df() function to inspect its contents. This function will return a data frame showing the contents of the bucket.
Here's a step-by-step guide to creating a new S3 bucket:
Objects
Objects in S3 buckets can be accessed and managed using various functions.
The `bucketlist()` function provides data frames of buckets to which the user has access.
To check if an object exists in a bucket, you can use the `object_exists()` function.
The `get_bucket()` and `get_bucket_df()` functions provide a list and data frame, respectively, of objects in a given bucket.
You can use the `s3read_using()` function to read from S3 objects using a user-defined function.
The `s3write_using()` function provides a generic interface for writing to S3 objects using a user-defined function.
To save an S3 object to a local file, you can use the `save_object()` function.
The `get_object()` function returns a raw vector representation of an S3 object.
Discover more: Invoke Aws Lambda Function Sam with S3 Trigger
To upload a local file to an S3 bucket, you can use the `put_object()` function.
Here are some common use cases for the `put_object()` function:
You can use the `s3connection()` function to stream an S3 object into R.
The `s3save()` function saves one or more in-memory R objects to an .Rdata file in S3.
You can use the `s3load()` function to load one or more objects into memory from an .Rdata file stored in S3.
The `s3source()` function sources an R script directly from S3.
To get a list of objects in a bucket, you can use the `get_bucket()` or `get_bucket_df()` functions.
You can use the `object_exists()` function to check if an object exists in a bucket.
The `s3read_using()` function can be used to read from S3 objects using a user-defined function.
To upload a local file to an S3 bucket, you can use the `put_object()` function with the `multipart = TRUE` argument.
On a similar theme: Aws S3 Object Locking
The `put_object()` function can be used to upload large files in pieces by setting the `multipart = TRUE` argument.
You can use the `save_object()` function to download an S3 object to a local file.
The `get_object()` function can be used to read a byte range of an S3 object.
You can use the `s3connection()` function to stream an S3 object into R.
Take a look at this: Aws S3 Delete Object
Managing Access Control
Managing Access Control is crucial when working with AWS S3 from R. By default, S3 buckets are set to private, allowing only the owner to read and write to them.
To soften this setting, you can use the put_acl() function to change the Access Control List (ACL) settings. For example, you can allow anyone to read from a bucket by using put_acl() with the argument "public-read".
It's worth noting that you can also set the ACL settings when creating a bucket using the put_bucket() function. However, if you've already created a bucket, you'll need to use put_acl() to make changes.
The key takeaway is to make sure you have the necessary IAM policies linked to your AWS login credentials to perform S3 operations.
Intriguing read: Aws S3 Acl
S3 CLI
You can interact with AWS S3 object storage using the AWS Command Line Interface (CLI), which requires appropriately configured AWS credentials.
With the AWS CLI, you can execute commands to manage your S3 bucket and objects. To get the bucket location, simply replace the bucket name in the command line interface.
You can also verify the creation of a subfolder on the AWS S3 console or using the AWS CLI by executing a specific command, which is a great way to ensure your subfolder has been successfully created.
For another approach, see: Aws Cli S3 Commands
CLI
You can access S3 object storage in the command line with appropriately configured AWS credentials. This allows you to interact with your S3 bucket and perform various tasks.
One way to verify if a subfolder has been generated on your existing folder on the AWS S3 console is to check the S3 console directly. Alternatively, you can use the AWS CLI by executing a specific command.
To create a subfolder on AWS S3 using the AWS CLI, you can use a command that involves specifying the object name with a key that ends in a slash (/). This is similar to how you would create a folder in a file system.
The AWS CLI or SDK can be used to create a "folder" (really, an S3 prefix) from an EC2 instance. This is done by using the aws s3api put-object command and constructing the folder-like structure in your S3 bucket.
You can get the bucket location by using the AWS CLI with a specific command. Simply replace the bucket name with your actual bucket name, and the command will display the bucket location using the command line interface.
Here are some common AWS CLI commands for S3:
- `aws s3 ls`: List the objects in a bucket
- `aws s3 cp`: Copy an object from one bucket to another
- `aws s3 rm`: Delete an object from a bucket
- `aws s3 mv`: Move an object from one bucket to another
Note that each S3 bucket is a flat datastore, meaning it doesn't contain any subfolders. The "/" is treated as part of the object name, nothing more.
Client Package
There are several client packages available for interacting with AWS S3, including the aws.s3 package. This package is a simple client for the S3 REST API, allowing users to connect to S3 from R.
To use the aws.s3 package, you'll need an AWS account and to enter your credentials into R. Your keypair can be generated on the IAM Management Console under the heading Access Keys.
Note that you only have access to your secret key once, so be sure to save it in a secure location. New keypairs can be generated at any time if yours has been lost, stolen, or forgotten.
Here are some key points to keep in mind when using the aws.s3 package:
- To use the package with S3-compatible storage provided by other cloud platforms, set the AWS_S3_ENDPOINT environment variable to the appropriate host name.
- To use the package from an EC2 instance, you would need to install aws.ec2metadata. This way, credential will be obtained from the machine's role.
Featured Images: pexels.com