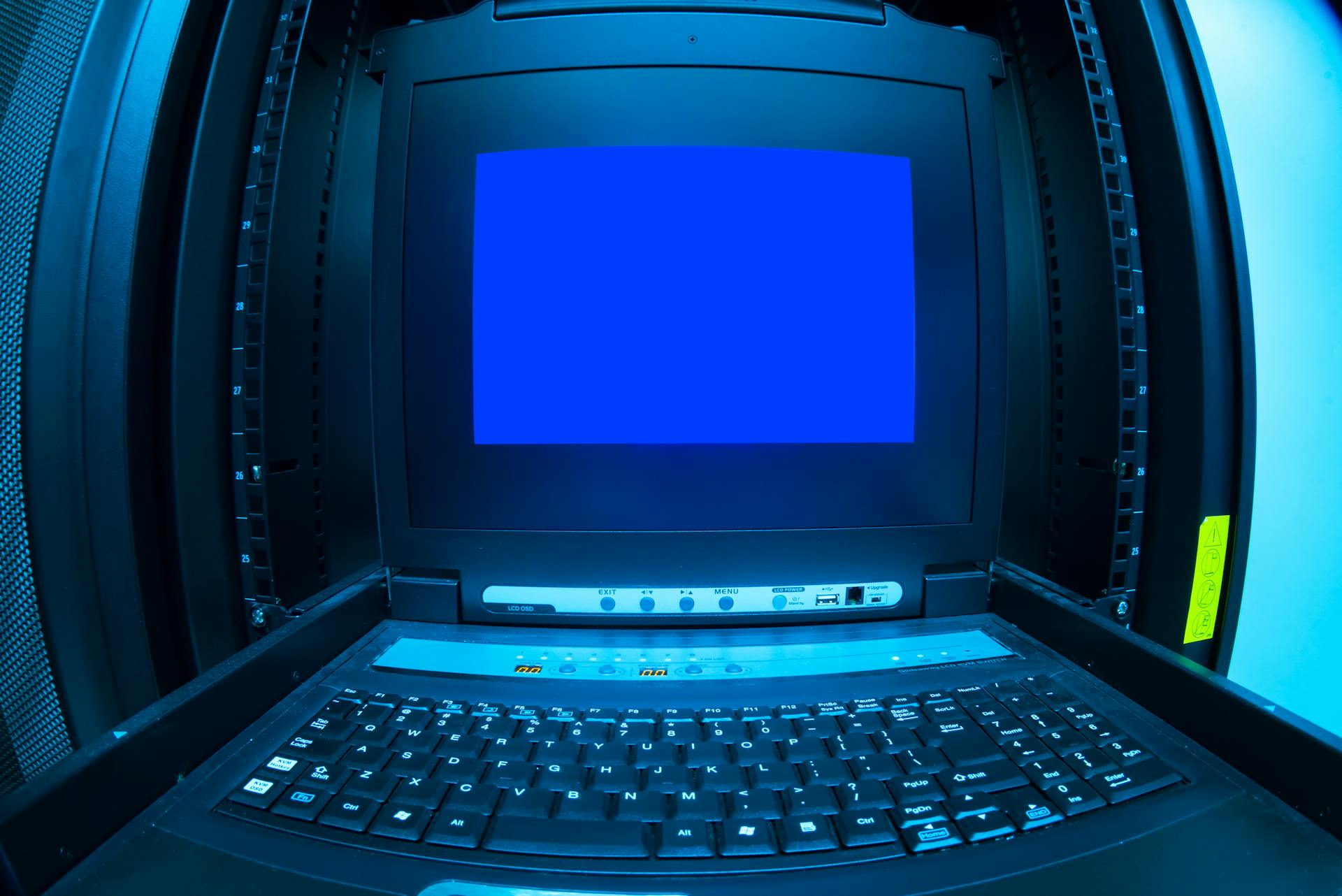
Building Azure and .NET Applications is a match made in heaven.
Azure provides a scalable and secure platform for .NET applications, allowing developers to focus on building features rather than managing infrastructure.
With Azure, developers can take advantage of a wide range of services, including Azure Storage, Azure Cosmos DB, and Azure Functions.
These services can be easily integrated into .NET applications, making it easier to build and deploy scalable and high-performance systems.
Azure also provides a robust set of tools and frameworks for building cloud-native .NET applications, including the Azure SDK for .NET and the Azure App Service.
Using these tools, developers can build applications that are optimized for cloud deployment and can take advantage of Azure's global reach and scalability.
Intriguing read: Azure Lab Services
Azure and .NET Basics
To start working with Azure and .NET, you'll need a basic understanding of building applications with the ASP.NET Core framework. This knowledge will serve as a foundation for more complex topics.
Check this out: .net Azure Sdk
A .Net Core runtime must be installed on your computer, and you'll also need Docker Desktop installed on your local machine. This will allow you to work with Docker containers and other Azure services.
To get started with Azure, you'll need a Microsoft Azure account, a CircleCI account, and a GitHub account. These accounts will provide you with the necessary tools and infrastructure to deploy and manage your .NET applications on Azure.
For your interest: Docker Compose Azure Container Apps
Prerequisites
To get started with Azure and .NET Basics, you'll need to have some prerequisites in place. You'll need a basic knowledge of building applications with the ASP.NET Core framework.
Make sure you have the .Net Core runtime installed on your computer, as this will allow you to run .NET applications. If you're on Windows or macOS, you can follow Docker's tutorial to install Docker Desktop on your local machine.
To work with Azure, you'll need a Microsoft Azure account. You'll also need a CircleCI account and a GitHub account to manage your code and automate your builds.
Here's an interesting read: Azure Developer Account
Here's a quick rundown of the specific tools you'll need to install:
- .Net Core runtime
- Docker Desktop (for Windows or macOS)
- Microsoft Azure account
- CircleCI account
- GitHub account
If you're planning to use Visual Studio 2015, you'll need to download the Visual Studio 2015 Update 3 and the Azure SDK for VS 2015 from the official Microsoft websites. Additionally, you'll need to download Microsoft Web Deploy to deploy your web applications to Azure.
Take a look at this: Azure Data Studio Connect to Azure Sql
Cloning Demo Project
To clone the demo project, you'll need to run a command in the terminal.
Run the following command to clone the project from its repository on GitHub: "Once the process is complete, you will have the project downloaded into a deploy-dotnet-azure-instance folder."
This folder will contain the project that we'll be working with throughout this tutorial.
The command to run is not specified in this section, but it can be found in other parts of the tutorial.
For your interest: Azure Terraform Examples
Identity Client Library
The Azure Identity client library for .NET is a must-have for any Azure developer. It provides Microsoft Entra ID (formerly Azure Active Directory) token authentication support across the Azure SDK.
You can install the library with NuGet, and it offers numerous credentials capable of acquiring a Microsoft Entra token to authenticate service requests.
The library focuses on OAuth authentication with Microsoft Entra ID, and it provides a set of TokenCredential implementations that can be used to construct Azure SDK clients that support Microsoft Entra token authentication.
Developers can use the library to authenticate with their own account for debugging and executing code locally. There are several developer tools that can be used to perform this authentication in their development environment.
The library supports multiple authentication methods, including Visual Studio, Visual Studio Code, Azure CLI, and Azure Developer CLI. Each of these methods has its own specific steps for authentication.
Here are the supported authentication methods:
The library also supports managed identity authentication, which allows you to authenticate with a user-assigned managed identity. This can be done by specifying the client ID or resource ID of the managed identity.
DefaultAzureCredential simplifies authentication while developing apps that deploy to Azure by combining credentials used in Azure hosting environments with credentials used in local development.
Additional reading: Azure Auth Json Website Azure Ad Authentication
Proxy Server Scenarios
When hosting apps out-of-process, the IIS Integration Middleware configures Forwarded Headers Middleware to forward the scheme (HTTP/HTTPS) and the remote IP address where the request originated.
Additional configuration might be required for apps hosted behind additional proxy servers and load balancers, specifically to handle scenarios beyond a single proxy server and load balancer.
The ASP.NET Core Module is also configured to forward the scheme (HTTP/HTTPS) and the remote IP address where the request originated, but further configuration may be necessary to ensure proper functioning.
If this caught your attention, see: Azure Application Configuration
Specify .NET Core SDK Version
To specify the .NET Core SDK version, you can use Azure Pipelines. You can set up a continuous integration build with Azure DevOps and configure the build to use a specific SDK version.
Azure Pipelines allows you to specify the .NET Core SDK version during the build process. You can do this by adding a new step to the Agent job list, searching for ".NET Core SDK" in the search bar, and moving the step to the first position in the build.
A fresh viewpoint: Azure Version Control
To specify the SDK version, you can search for ".NET Core SDK" in the search bar and select the version you want to use. In this example, the SDK is set to 3.0.100.
If you're using the App Service deployment center to create an Azure DevOps build, you can add a new step to the build pipeline to specify the .NET Core SDK version. You can then move this step to the first position in the build to ensure that the steps following it use the specified version of the .NET Core SDK.
Here are some common .NET Core SDK versions and their corresponding RIDs:
Remember to specify the correct RID for your .NET Core SDK version to ensure that your app runs correctly on Azure App Service.
Platform
The platform architecture of an App Services app is set in the app's settings in the Azure Portal for apps that are hosted on an A-series compute (Basic) or higher hosting tier.
Confirm that the app's publish settings match the setting in the app's service configuration in the Azure Portal, especially when using Visual Studio publish profiles.
ASP.NET Core apps can be published framework-dependent because the runtimes for 64-bit and 32-bit apps are present on Azure App Service.
The .NET Core SDK available on App Service is 32-bit, but you can deploy 64-bit apps built locally using the Kudu console or the publish process in Visual Studio.
Runtimes for 32-bit apps are present on Azure App Service, making it a suitable choice for apps with native dependencies.
The .NET Core SDK available on App Service is 32-bit, so keep this in mind when deploying apps with native dependencies.
A different take: How to Connect to Azure Cosmos Db Using Connection String
Authentication and Identity
The Azure Identity library provides Microsoft Entra ID (formerly Azure Active Directory) token authentication support across the Azure SDK. It offers a set of TokenCredential implementations that can be used to construct Azure SDK clients that support Microsoft Entra token authentication.
You might like: Microsoft Azure Dev
DefaultAzureCredential is a credential that provides a simplified authentication experience to quickly start developing apps run in Azure. It attempts to authenticate using a client secret, certificate, or managed identity.
To use a managed identity, you can specify a client ID or a resource ID. A client ID is used to identify a user-assigned managed identity, while a resource ID takes the form /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ManagedIdentity/userAssignedIdentities/{identityName}.
Here are the supported Azure services that use managed identity authentication:
- Azure App Service and Azure Functions
- Azure Arc
- Azure Cloud Shell
- Azure Kubernetes Service
- Azure Service Fabric
- Azure Virtual Machines
- Azure Virtual Machines Scale Sets
You can authenticate with a user-assigned managed identity by specifying one of the following IDs for the managed identity: Client ID.
The Azure Identity library focuses on OAuth authentication with Microsoft Entra ID. It offers numerous credentials capable of acquiring a Microsoft Entra token to authenticate service requests. Each credential in this library is an implementation of the TokenCredential abstract class in Azure.Core.
Here are some credential classes available in the Azure Identity library:
- DefaultAzureCredential
- ChainedTokenCredential
- ManagedIdentityCredential
- ClientCertificateCredential
- ClientSecretCredential
- UsernamePasswordCredential
- VisualStudioCredential
- VisualStudioCodeCredential
- InteractiveBrowserCredential
- DeviceCodeCredential
- AuthorityHostCredential
- EnvironmentCredential
- VisualStudioCodeCredential
- VisualStudioCredential
- UsernamePasswordCredential
- ClientCertificateCredential
- ClientSecretCredential
- ManagedIdentityCredential
- DeviceCodeCredential
- InteractiveBrowserCredential
- AuthorityHostCredential
- EnvironmentCredential
Note that this is not an exhaustive list, and you can refer to the Credential classes section for a complete listing of available credential types.
Authentication Flows
You can define a custom authentication flow with ChainedTokenCredential, which enables users to combine multiple credential instances to create a customized chain of credentials. This is especially useful when DefaultAzureCredential isn't the quickest way to authenticate apps for Azure.
The ChainedTokenCredential is a powerful tool that allows you to create a customized chain of credentials.
There are several options for authentication flows, including Brokered authentication. This involves using an authentication broker, which is an app that runs on a user's machine and manages the authentication handshakes and token maintenance for connected accounts.
Brokered authentication is currently only supported on Windows via Web Account Manager (WAM). To enable support, you'll need to use the Azure.Identity.Broker package.
Here are the supported products and versions for the Azure.Identity.Broker package:
Service Principals and Users
Service principals and users have different authentication methods. You can authenticate service principals using AzurePipelinesCredential, ClientAssertionCredential, ClientCertificateCredential, or ClientSecretCredential.
For users, the options are more varied. You can use AuthorizationCodeCredential, DeviceCodeCredential, InteractiveBrowserCredential, OnBehalfOfCredential, or UsernamePasswordCredential to authenticate them.
Here are some specific authentication methods for service principals and users:
Service Principals
Service principals are a crucial part of Azure Pipelines, allowing you to authenticate and authorize services to access your resources.
There are several types of credentials you can use to authenticate service principals, including AzurePipelinesCredential, ClientAssertionCredential, ClientCertificateCredential, and ClientSecretCredential.
AzurePipelinesCredential supports Microsoft Entra Workload ID on Azure Pipelines.
ClientCertificateCredential authenticates a service principal using a certificate.
ClientSecretCredential authenticates a service principal using a secret.
You can use a service principal with a certificate by setting the following variables: AZURE_CLIENT_ID, AZURE_TENANT_ID, AZURE_CLIENT_CERTIFICATE_PATH, AZURE_CLIENT_CERTIFICATE_PASSWORD, AZURE_CLIENT_SEND_CERTIFICATE_CHAIN.
Users
Users play a crucial role in the authentication process, and there are several ways to authenticate them.
Some common credentials used to authenticate users include AuthorizationCodeCredential, which authenticates a user with a previously obtained authorization code, and UsernamePasswordCredential, which authenticates a user with a username and password.
DeviceCodeCredential is another option, which is used to interactively authenticate a user on devices with limited UI.
InteractiveBrowserCredential is similar, but it uses the default system browser to authenticate the user.
OnBehalfOfCredential is used to propagate the delegated user identity and permissions through the request chain.
Here's a brief summary of the different user credentials:
Each of these credentials has its own strengths and weaknesses, and the choice of which to use will depend on the specific use case and requirements.
Curious to learn more? Check out: Azure Key Vault Tutorial C#
Token Management
Token Management is a crucial aspect of working with Azure and .NET. Managed identity support is available for various Azure services, including Azure App Service and Azure Functions, Azure Arc, Azure Cloud Shell, Azure Kubernetes Service, Azure Service Fabric, Azure Virtual Machines, and Azure Virtual Machines Scale Sets.
These services can leverage the Azure Identity library's token caching feature to improve resilience and performance. Token caching allows apps to cache tokens in memory or on disk, reducing the number of requests made to Microsoft Entra ID.
The Azure Identity library offers both in-memory and persistent disk caching. This can be particularly useful for applications that require frequent authentication.
To authenticate with a user-assigned managed identity, you must specify one of the following IDs for the managed identity: Client ID.
Curious to learn more? Check out: Azure Library
Frequently Asked Questions
Does Azure use C#?
Azure Functions supports C# as a programming language for creating serverless functions. You can use Visual Studio to create and publish C# function projects to run in Azure.
What is the salary of .NET with Azure?
The average salary for a .NET developer with Azure expertise in India is ₹ 1,600,000 per year. This translates to ₹ 641 per hour, making it a lucrative career choice for professionals in the field.
Does Azure support .NET core?
Yes, Azure App Service supports .NET Core, allowing you to host web apps built with this framework. Learn more about hosting your .NET Core apps on Azure.
What is .net in Azure?
.NET in Azure refers to a set of tools and libraries for building web applications and services. It's a popular choice for hosting web applications due to its ease of use and seamless integration with Azure
Is Azure good for .NET developers?
Azure is a top choice for .NET developers, especially those working with Microsoft technologies and cloud computing. It's ideal for those already in the .NET ecosystem or looking to transition into cloud computing careers.
Featured Images: pexels.com