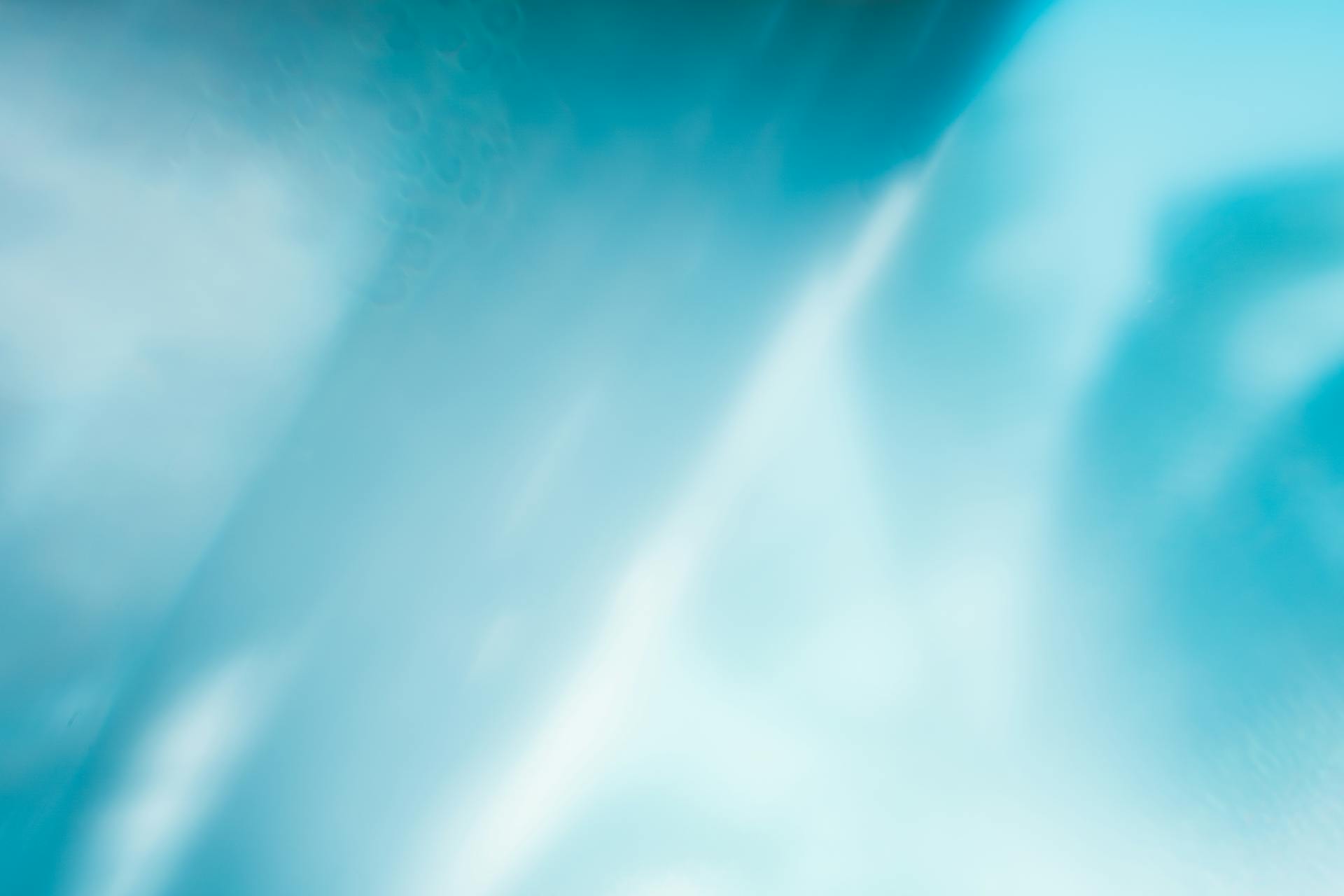
Developing an Azure library can be a complex task, especially for those new to cloud computing. The Azure library is a collection of tools and resources that allow developers to build, deploy, and manage applications on the Azure platform.
To start, it's essential to understand the different types of Azure libraries available, such as the Azure SDK and the Azure Storage library. These libraries provide pre-built functionality for common tasks, making it easier to develop and deploy Azure applications.
One key aspect of Azure library development is choosing the right programming language. According to the article, the Azure SDK supports a wide range of languages, including Python, Java, and C#. This flexibility allows developers to use their preferred language when building Azure applications.
By following best practices and utilizing the right tools, developers can create efficient and scalable Azure applications.
Explore further: Azure Sdk
Install the Package
To install the Azure library, you'll need to install the Azure client libraries from NPM. You can do this by running the command "Install Azure npm packages" in your terminal.
Readers also liked: Pip Install Azure
If you're using .NET, you'll need to install the Azure Blob Storage client library. You can do this by following these steps: right-click the Dependencies node of your project in Visual Studio, select Manage NuGet Packages, search for Azure.Storage.Blobs, select the appropriate result, and select Install.
You can also use the command "dotnet add package Azure.Storage.Blobs" to install the package. However, if this command fails, you'll need to make sure that nuget.org is added as a package source. You can do this by running the command "dotnet nuget list source" to list the package sources, and then adding nuget.org using the command "dotnet nuget add source https://api.nuget.org/v3/index.json -n nuget.org".
Here are the steps to install the Azure Blob Storage client library for .NET:
- Right-click the Dependencies node of your project in Visual Studio.
- Select Manage NuGet Packages.
- Search for Azure.Storage.Blobs.
- Install the package.
You can use either Visual Studio 2022 or the .NET CLI to install the package.
Authentication
Authentication is a crucial aspect of working with the Azure library. The Azure client libraries require credentials to authenticate to the Azure platform, which can be provided using credential classes from the @azure/identity package. These credential classes offer several benefits, including fast onboarding, the most secure method, and the ability to separate the authentication mechanism from the code.
Discover more: Azure Auth Json Website Azure Ad Authentication
DefaultAzureCredential is a class provided by the Azure Identity client library for .NET, which simplifies authentication while developing apps that deploy to Azure by combining credentials used in Azure hosting environments with credentials used in local development.
The Azure Identity library focuses on OAuth authentication with Microsoft Entra ID, and it offers various credential classes capable of acquiring a Microsoft Entra token to authenticate service requests. All of the credential classes in this library are implementations of the TokenCredential abstract class.
You can use DefaultAzureCredential to authenticate to Azure and authorize access to blob data, or you can authorize requests to Azure Blob Storage by using the account access key. However, using the account access key should be used with caution, as it can expose the access key in an unsecure location.
To assign a role at the resource level using the Azure CLI, you first must retrieve the resource id using the az storage account show command.
Here are some examples of credential classes and their usage:
Continuous Access Evaluation (CAE) is possible on a per-request basis, and can be enabled using the GetTokenOptions.enableCae(boolean) API. CAE isn't supported for developer credentials.
You can also authenticate via Visual Studio Code using the Azure Account extension and the @azure/identity-vscode plugin package. However, it's a known issue that VisualStudioCodeCredential doesn't work with Azure Account extension versions newer than 0.9.11.
You might enjoy: Azure Developer Account
Configuration
Configuration is a crucial step in using the Azure library. You need to specify the authority host for your cloud, which is the URL of your cloud's authority.
By default, credentials authenticate to the Microsoft Entra endpoint for Azure Public Cloud. To access resources in other clouds, you need to configure credentials with the authorityHost argument in the constructor.
The AzureAuthorityHosts enum defines authorities for well-known clouds, making it easier to find the right one. This includes the US Government cloud, which you can access by instantiating a credential with the authorityHost argument.
You can also set the AZURE_AUTHORITY_HOST environment variable to the URL of your cloud's authority. This is useful when configuring multiple credentials to authenticate to the same cloud or when the deployed environment needs to define the target cloud.
Not all credentials require this configuration, so don't assume you need to do it for every credential. Credentials that authenticate through a development tool, such as AzureCliCredential, use that tool's configuration by default.
For more insights, see: Azure Function Env Variables
Libraries
Azure offers a range of libraries to simplify development and access to Azure services. These libraries abstract away boilerplate code required for cloud-based Azure platform REST requests, such as authentication, retries, and logging.
The Azure client libraries are the preferred method of accessing Azure services, as they provide a more straightforward way to authenticate Azure SDK clients locally, in development environments, and in production. You can use these libraries to create and manage Azure resources, as well as consume and interact with existing resources.
Azure client libraries are available for various programming languages, including .NET, and can be used to interact with Azure Storage, Azure Blob Storage, and other Azure services. The libraries are designed to be used with Azure SDK clients, which handle intelligent caching and token refreshing at the core HTTP layer.
Here are some key Azure client library types:
- Management libraries (arm- in their package names): enable you to create and manage Azure resources.
- Client libraries: enable you to consume and interact with existing Azure resources.
Azure client libraries can be used to authenticate Azure SDK clients locally, in development environments, and in production, and are designed to provide a more straightforward way to authenticate Azure services.
Differences Between Client Libraries and REST APIs
Client libraries are the preferred method of accessing your Azure service because they abstract away the boilerplate code required to manage cloud-based Azure platform REST requests such as authentication, retries, and logging.
Using client libraries can save you a lot of time and effort, as they handle the underlying complexities for you.
If you're building an application that requires a high level of customization, Azure REST APIs might be a better fit.
Here are the key differences between client libraries and REST APIs:
- Client libraries are the preferred method of accessing your Azure service.
- Azure REST APIs are the preferred method if you are using client libraries.
This means that if you're working with Azure, it's usually best to start with the client libraries and see if they meet your needs.
Libraries
Azure offers various libraries to access and manage Azure services. The Azure client libraries are the preferred method of accessing your Azure service, abstracting away the boilerplate code required to manage cloud-based Azure platform REST requests such as authentication, retries, and logging.
There are two types of Azure client libraries: Management and Client. Management libraries enable you to create and manage Azure resources, while Client libraries allow you to consume and interact with existing Azure resources. You can recognize Management libraries by the "arm-" prefix in their package names.
The Azure Storage client library for data access is version 12.x.x, which is the recommended version for new applications. If you cannot update to version 12.x.x, Microsoft recommends using version 11.x.x. The source code for the Azure Storage client libraries for .NET is available on GitHub.
The Azure Storage client library for resource management is version 1.x.x, which is the recommended version for new applications. If you cannot update to version 1.x.x, Microsoft recommends using version 25.x.x.
Here are the key types of Azure libraries:
Bundling
Bundling is a powerful technique that can simplify your code and make it more efficient. Bundling with the Azure SDK is a great way to do this.
By bundling, you can combine multiple files or libraries into a single file, reducing the number of HTTP requests your application needs to make. This can lead to faster load times and a better user experience.
To learn more about bundling with the Azure SDK, you can check out the Azure documentation for more information.
Curious to learn more? Check out: Windows Azure Sdk for Java
Usage
To authenticate with Azure, you can use the Azure Developer CLI, Azure CLI, or InteractiveBrowserCredential. The Azure Developer CLI can be authenticated with the command `azd auth login`, while the Azure CLI uses `az login` or `az login --use-device-code` for systems without a default web browser.
You can also create an SDK client and call methods using the DefaultAzureCredential, which can be used to list subscriptions that the credential has access to read. To create a container, you can use the `CreateBlobContainerAsync` method on the `blobServiceClient` object.
To use Azure npm package sample code, refer to the specific NPM packages you use to learn how to use them. To sign in and connect your app code to Azure using DefaultAzureCredential, make sure you're authenticated with the same Microsoft Entra account you assigned the role to, and update your `Program.cs` code to use the `DefaultAzureCredential`.
You might enjoy: Azure Powershell vs Cli
When to Use @Identity
When to use @azure/identity is a crucial question for any Azure developer. @azure/identity is focused on providing the most straightforward way to authenticate Azure SDK clients locally, in development environments, and in production.
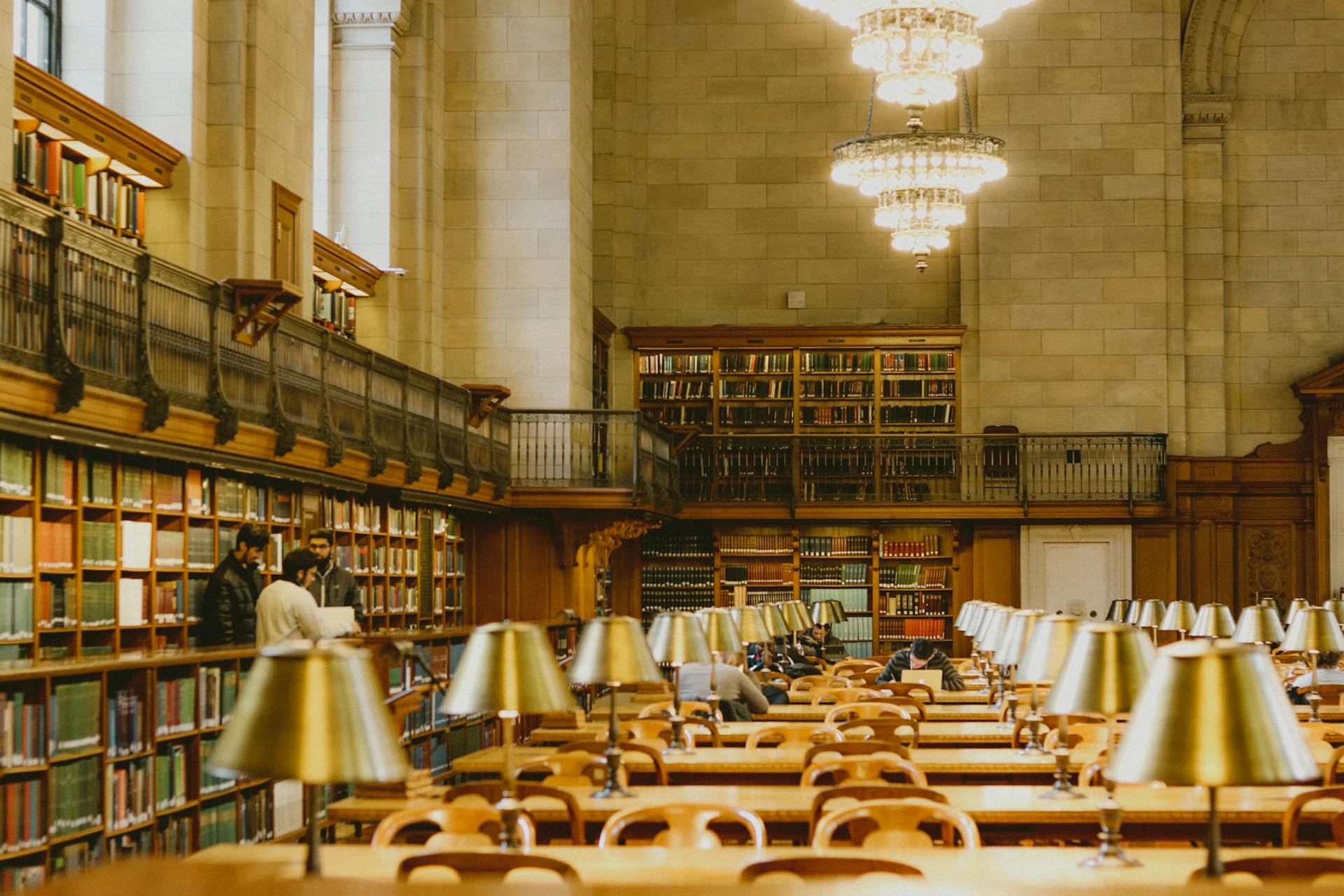
It's supported in Node.js, but for browsers, InteractiveBrowserCredential is the credential type to use for basic authentication scenarios.
The credential types offered by @azure/identity use the Microsoft Authentication Library for JavaScript (MSAL.js), specifically the v2 MSAL.js libraries, which use OAuth 2.0 Authorization Code Flow with PKCE and are OpenID-compliant.
If you're new to @azure/identity or Microsoft Entra ID, it's a good idea to read the Using @azure/identity with Microsoft Entra ID document first.
Developers using Visual Studio Code can use the Azure Account extension to authenticate via the editor, but it's essential to note that VisualStudioCodeCredential doesn't work with Azure Account extension versions newer than 0.9.11.
Here are some scenarios where you should use @azure/identity:
- For local development, use the Azure CLI or Azure PowerShell to authenticate with the same Microsoft Entra account you assigned the role to.
- When using DefaultAzureCredential, add the Azure.Identity package to your application.
- If you're deploying to Azure, use managed identity on your app and configure your storage account to allow that managed identity to connect.
Remember to update your storage account name in the URI of your BlobServiceClient, and be careful not to expose storage account access keys in an unsecure location.
If this caught your attention, see: Azure Blob Storage Move Files between Containers C#
Create SDK Client and Call Methods
To create an SDK client and call methods, you'll need to programmatically create a credential and pass it to your Azure client. The client may require additional information such as a subscription ID or service endpoint, which are available in the Azure portal for your resource.
A unique perspective: Create Sample Azure Function for .net Frame Work
You can use the DefaultAzureCredential, which abstracts away the boilerplate code required to manage cloud-based Azure platform REST requests such as authentication, retries, and logging. This is the preferred method of accessing your Azure service.
The DefaultAzureCredential can be used with the arm subscription client library to list subscriptions which this credential has access to read. For example, the code example in Example 15 uses the DefaultAzureCredential and the arm subscription client library to list subscriptions.
To list subscriptions with the @azure/arm-subscriptions SDK, you can use the following code:
```csharp
using Azure.Identity;
using Azure.ResourceManager.Subscriptions;
var credential = new DefaultAzureCredential();
var subscriptionClient = new SubscriptionClient(credential);
var subscriptions = await subscriptionClient.ListAsync();
```
This code creates a credential, passes it to the subscription client, and then lists the subscriptions.
You can also use the Azure CLI to authenticate and then use the DefaultAzureCredential in your code. For example, you can run the command `az login` to authenticate with the Azure CLI, and then use the DefaultAzureCredential in your code.
By following these steps, you can create an SDK client and call methods using the Azure SDK for .NET.
A unique perspective: Brew Install Azure Cli
Asynchronous Paging
Asynchronous Paging is a powerful feature that allows you to handle large result sets without overwhelming your application.
You can return an asynchronous iterator, PagedAsyncIterableIterator, to enable asynchronous results. This iterator breaks up result sets using paging and continuation tokens.
Paging size can be set to control the number of results returned at a time. For example, setting an artificially short paging size of 2 can quickly demonstrate the process.
Learn more about paging and iterators on Azure by checking out the resources listed below:
- Async Iterators in the Azure SDK for JavaScript/TypeScript
Long Running Operations
Long Running Operations are a reality in cloud computing, and Azure is no exception. An SDK method can return a long running operation (LRO) raw response that includes information about the status of your request.
This response can indicate whether your request has completed or is still in process. It's essential to wait for the LRO to complete before continuing with your code.
The Azure documentation recommends using the .pollUntilDone() method to wait for an LRO to complete. This method allows you to poll the LRO until it's done, ensuring that your code doesn't proceed until the operation is complete.
If you're interested in learning more about LROs, the @azure/core-lro package is a great resource to explore.
If this caught your attention, see: Azure Code
Troubleshooting
If you're experiencing issues with your Azure Library, start by checking the connection to your Azure account. Make sure your credentials are correct and your account is active.
Azure Library requires a stable internet connection, so ensure your network is stable and your internet service provider is not experiencing outages.
If you're still encountering issues, try clearing your browser cache and restarting your browser to refresh the session.
Broaden your view: How to Connect to Azure Cosmos Db Using Connection String
Known Issues
In troubleshooting, it's essential to be aware of known issues that can affect your progress. The Azure Storage client libraries for .NET have several known issues that you should be aware of.
One known issue is that the libraries may not properly handle certain types of errors, which can lead to unexpected behavior. This can be frustrating, especially if you're working under a tight deadline.
Another issue is that the libraries may not work correctly with certain versions of the .NET framework. This means you may need to upgrade or downgrade your framework to get things working.
In some cases, the libraries may not be able to handle large amounts of data, which can cause problems if you're working with big datasets. This is something to keep in mind if you're planning to use the libraries for a project that involves large amounts of data.
Client-Side Encryption Bug with Unauthenticated Symmetric Algorithm
The Client-Side Encryption Bug with Unauthenticated Symmetric Algorithm is a known issue that might affect customers using Azure.Storage.Blobs. A bug was found in BlobClient.UpdateClientSideEncryptionKey that might silently rotate an unauthenticated symmetric encryption key incorrectly.
This bug only affects a small subset of key wrapping algorithms that don't throw an exception during key rotation. Most key wrapping algorithms safely throw an exception and don't actually rotate the key.
Customers who use an authenticated symmetric key wrapping algorithm or asymmetric keys are not affected by this bug. Asymmetric keys are used by Azure Key Vault and are not impacted by this issue.
The async API BlobClient.UpdateClientSideEncryptionKeyAsync is also not affected by this bug, providing an alternative solution for customers who need to rotate their keys.
Additional reading: Azure Api Key
Invalid Header Value Error in Beta SDK
If you're experiencing an Invalid Header Value error in the beta SDK, it's likely due to a rare scenario where the latest upgrade causes issues with the Storage libraries.
This error can occur even after upgrading to the latest beta or generally available version of the SDK.
The error message will look similar to the following sample: "InvalidHeaderValue".
To resolve this issue, try downgrading to the previous generally available version of the SDK to see if the problem resolves.
If downgrading is not feasible or the issue persists, open a support ticket to explore further options.
A fresh viewpoint: Azure Version Control
Canceling Async Operations
Canceling async operations can be a real pain, but thankfully, the @azure/abort-controller package has got you covered. It provides AbortController and AbortSignal classes that make it easy to cancel pending work.
To create an AbortSignal, you'll use the AbortController class. This signal can then be passed to Azure SDK operations to cancel them.
Azure SDK operations can be aborted based on your own logic, which is super handy for custom scenarios. Or, you can use a timeout limit to automatically cancel operations after a set amount of time.
Here are some ways you can use abort signals to cancel operations in the Azure SDK:
- Aborted based on your own logic
- Aborted based on a timeout limit
- Aborted based on a parent task's signal
- Aborted based on a parent task's signal or a timeout limit
Frequently Asked Questions
What is the Azure Core library?
The Azure Core library is a collection of shared tools and helpers for building modern .NET Azure SDK client libraries. It provides a foundation for creating efficient and scalable Azure applications.
How to use Azure library variables?
To update Azure library variables, navigate to Pipelines > Library in your Azure DevOps project and select the variable group you want to modify. From there, make your changes and select Save to apply the updates.
Sources
- https://www.npmjs.com/package/@azure/identity
- https://learn.microsoft.com/en-us/azure/developer/javascript/core/use-azure-sdk
- https://cloudacademy.com/library/azure/
- https://learn.microsoft.com/en-us/azure/storage/blobs/storage-quickstart-blobs-dotnet
- https://learn.microsoft.com/en-us/dotnet/api/overview/azure/storage
Featured Images: pexels.com