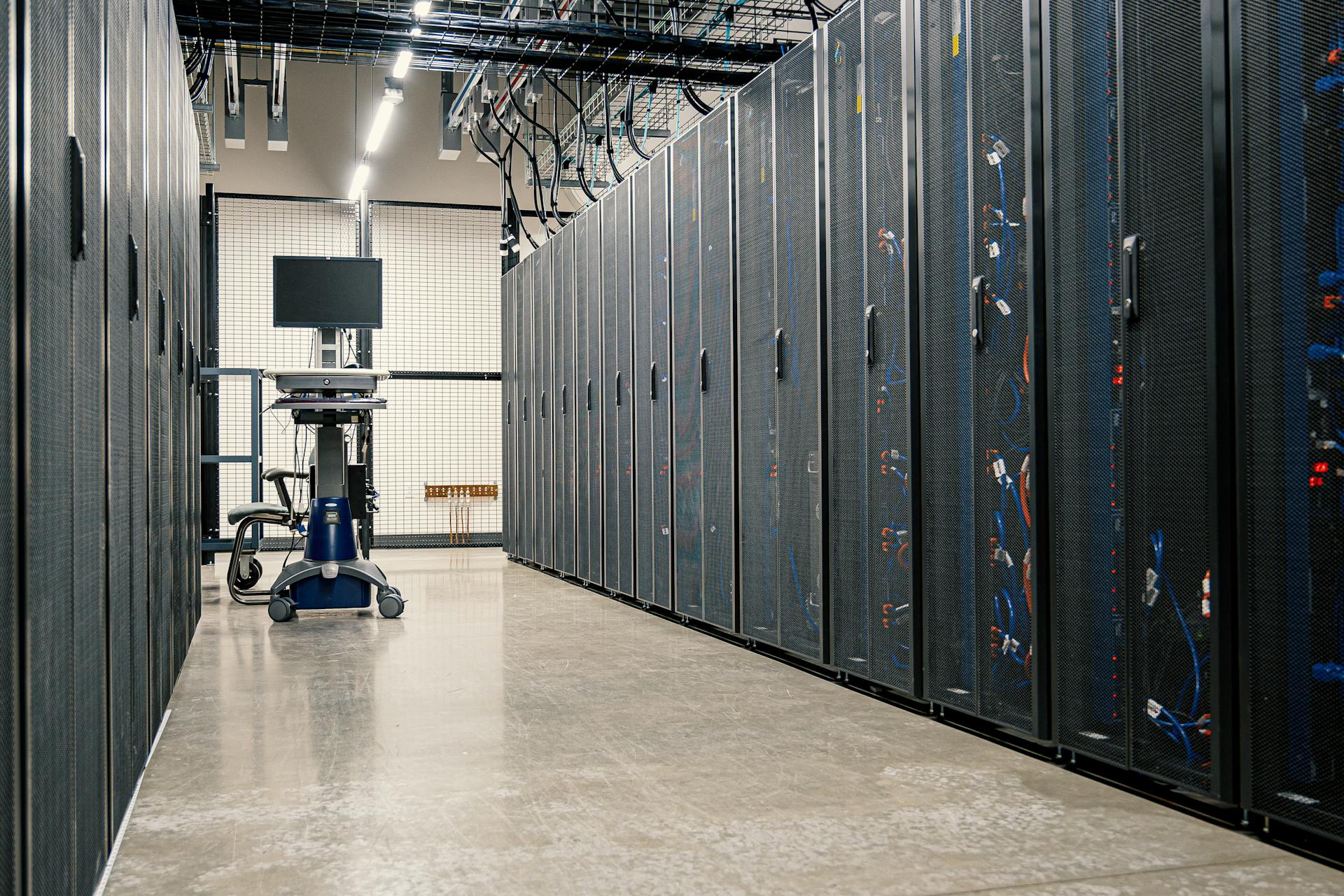
The Azure SDK for .NET is a must-have for any .NET developer looking to tap into the power of Azure services. It provides a comprehensive set of libraries and tools for building cloud-based applications.
One of the key benefits of the Azure SDK is its ability to simplify the process of interacting with Azure services, making it easier to get started with cloud development. This is made possible by the SDK's robust set of APIs and libraries.
With the Azure SDK, you can leverage Azure services such as Azure Storage, Azure Cosmos DB, and Azure Active Directory, among others. This allows you to build scalable, secure, and highly available applications that can take advantage of the cloud's vast resources.
Whether you're building a web application, a mobile app, or a microservices-based system, the Azure SDK has got you covered. Its flexible and modular design makes it easy to incorporate into your existing .NET projects.
Related reading: Azure Sdk for Net
Getting Started
First, set up your local development environment, it's a one-time setup that will make using Azure libraries for Python a breeze.
We strongly recommend doing this setup so you can easily use any of the Azure libraries for Python.
This will save you time and effort in the long run, trust me, I've been there.
By setting up your local dev environment, you'll be ready to start working with Azure libraries in no time.
Additional reading: Python Azure Sdk
Azure SDK Basics
The Azure SDK Basics cover the essential tools you need to get started with Azure development. The management libraries, prefixed with "azure-mgmt-", allow you to create and manage Azure resources from Python scripts.
These libraries are available for all Azure services, and you can use them to write configuration and deployment scripts. You can perform tasks that would normally be done through the Azure portal or CLI using these libraries.
The management libraries can be used to automate tasks such as creating resource groups, listing resource groups in a subscription, and deploying web apps to App Service. You can find more information on working with each library in the README.md or README.rst file located in the library's project folder in the SDK GitHub repository.
Some common tasks you can perform with the management libraries include:
- Create a resource group
- List resource groups in a subscription
- Create an Azure Storage account and a Blob storage container
- Create and deploy a web app to App Service
- Create and query an Azure MySQL database
- Create a virtual machine
Prerequisites
To get started with Azure SDK, you'll need to meet some prerequisites.
You'll need to have the Azure Developer CLI installed. This is a must-have for working with Azure SDK. If you don't have it installed, you can download it from the Azure website.
You'll also need Docker Desktop installed on your machine. This will allow you to create and manage containers for your Azure SDK projects.
The specific programming language you'll be using will also determine the prerequisites. For example, if you're using .NET, you'll need version 9.0 or later installed. If you're using Go, you'll need version 1.21 or later installed. If you're using Java, you'll need version 21 or later installed.
Here are the specific language prerequisites:
Language | Prerequisite |
---|---|
.NET | .NET 9.0 or later |
Go | Go 1.21 or later |
Java | Java 21 or later |
If you don't have an Azure account, you'll need to create a free account before you can start working with Azure SDK.
You might like: Azure Accounts
Initialize The Project
To initialize an Azure Cosmos DB for Table account and deploy a containerized sample application, you'll need to use the Azure Developer CLI (azd). Open a terminal in an empty directory to start the process.
The first step is to authenticate to the Azure Developer CLI using azd auth login. This will prompt you to follow the steps specified by the tool to authenticate using your preferred Azure credentials.
Once authenticated, use azd init to initialize the project. You can choose from different templates, such as cosmos-db-nosql-dotnet-quickstart, cosmos-db-nosql-go-quickstart, or cosmos-db-nosql-java-quickstart, depending on your programming language of choice.
During initialization, configure a unique environment name to keep your projects organized.
Here are the steps to initialize the project:
- Open a terminal in an empty directory
- Authenticate to the Azure Developer CLI using azd auth login
- Use azd init to initialize the project, choosing a template (e.g. cosmos-db-nosql-dotnet-quickstart)
- Configure a unique environment name
- Deploy the Azure Cosmos DB account using azd up
The provisioning process will take approximately five minutes, during which you'll be prompted to select your subscription, desired location, and target resource group. Once complete, a URL to the running web application will be included in the output.
Install the Library
Installing the Azure SDK library is a straightforward process that can be completed using various programming languages. For example, in Java, you can use the Azure Developer CLI (azd) to initialize a project and deploy a containerized sample application, which includes the client library.
To install the client library in Java, you need to authenticate to the Azure Developer CLI using azd auth login. Once authenticated, you can use azd init to initialize the project and configure a unique environment name.
In Python, the client library is available through NuGet as the Microsoft.Azure.Cosmos package. To install it, open a terminal and navigate to the /src/web folder, then use dotnet add package to install the package.
The client library is also available in Go, as the azcosmos package, which can be installed using go install. Similarly, the azidentity package can be installed using go install. The same applies to Maven, where the azure-spring-data-cosmos package can be added to the pom.xml file.
Here's a summary of the steps to install the client library in different programming languages:
Language | Steps |
---|---|
Java | azd auth login, azd init, azd up |
Python | dotnet add package Microsoft.Azure.Cosmos |
Go | go install azcosmos, go install azidentity |
Maven | add azure-spring-data-cosmos and azure-identity dependencies to pom.xml |
By following these steps, you can successfully install the Azure SDK library and start working with Azure resources in your preferred programming language.
Object Model
The object model of the Azure SDK is a crucial aspect of understanding how to work with Azure services from Python code. It's essentially a set of classes and methods that allow you to interact with Azure resources.
The primary client class in the object model is the CosmosClient, which is used to manage account-wide metadata or databases. This class is the foundation for many other classes and methods in the object model.
A database within the account is represented by the Database class, which is a crucial part of the object model. This class allows you to perform various operations on the database, such as creating, updating, and deleting it.
The Container class is primarily used to perform read, update, and delete operations on either the container or the items stored within the container. This class is an essential part of the object model, as it allows you to interact with the data stored in the container.
The PartitionKey class represents a logical partition key, which is required for many common operations and queries. This class is an important part of the object model, as it allows you to specify how data is partitioned within the container.
Here's a summary of the main classes in the object model:
Name | Description |
---|---|
CosmosClient | Primary client class to manage account-wide metadata or databases |
Database | Represents a database within the account |
Container | Used to perform read, update, and delete operations on the container or items |
PartitionKey | Represents a logical partition key required for many operations and queries |
By understanding the object model of the Azure SDK, you'll be able to write more effective and efficient code that interacts with Azure resources.
Frequently Asked Questions
What is the Azure SDK?
The Azure SDK is a collection of libraries that helps you interact with Azure services from your preferred programming language. It simplifies Azure usage with consistent, approachable, and dependable libraries.
How do I install Azure SDK?
To install the Azure SDK, download the SDK and run the downloaded .exe file on your PC. Follow the installation prompts to complete the setup.
Sources
- https://learn.microsoft.com/en-us/azure/cosmos-db/nosql/quickstart-dotnet
- https://learn.microsoft.com/en-us/azure/cosmos-db/nosql/quickstart-go
- https://learn.microsoft.com/en-us/azure/cosmos-db/nosql/quickstart-java
- https://learn.microsoft.com/en-us/azure/developer/python/sdk/azure-sdk-overview
- https://learn.microsoft.com/en-us/azure/iot-hub/iot-hub-devguide-sdks
Featured Images: pexels.com