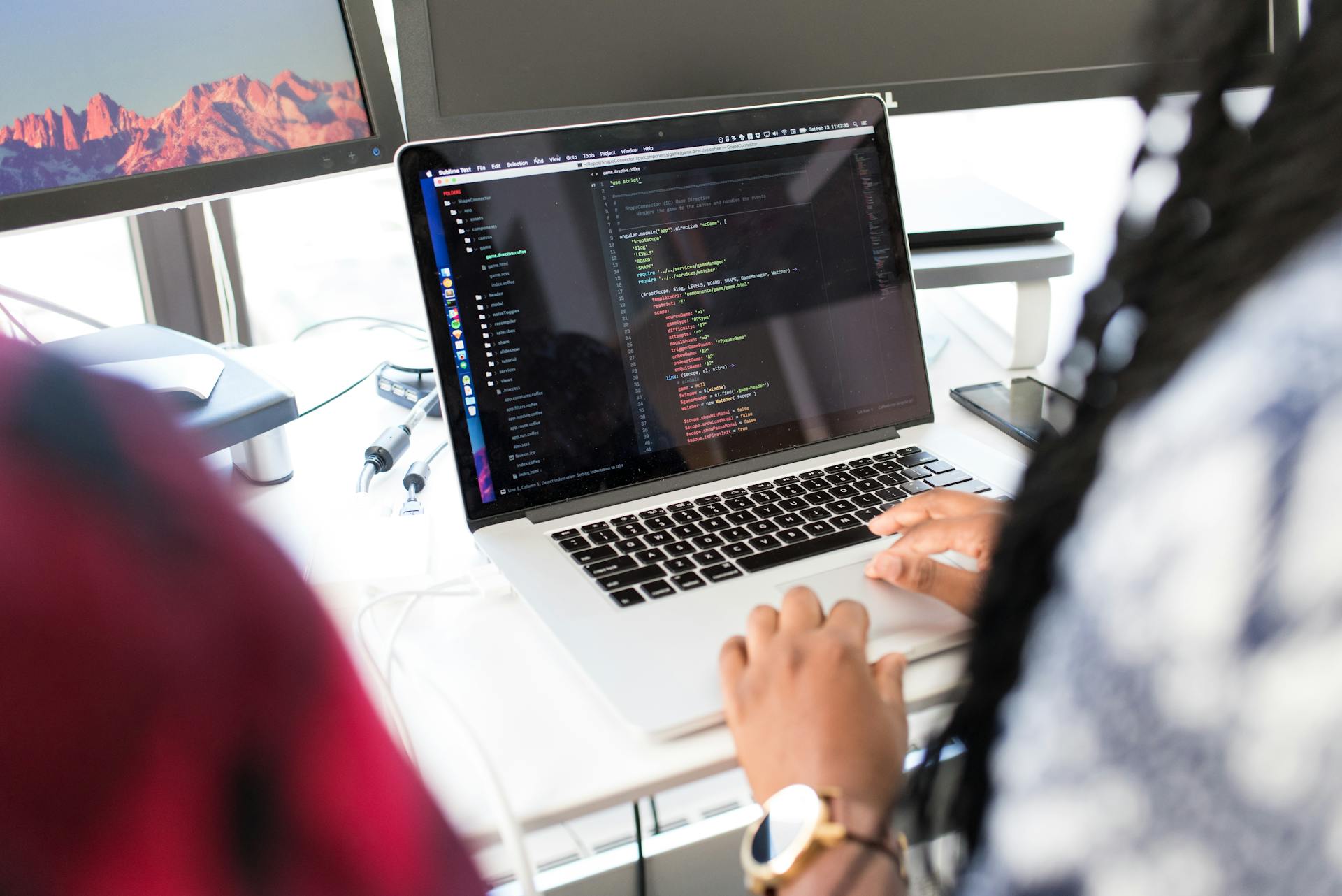
Publishing and managing Azure DevOps pipeline artifacts is a crucial step in the software development process. Azure DevOps provides a robust feature for storing and managing pipeline artifacts, making it easier to share and reuse assets across different teams and projects.
To publish pipeline artifacts, you can use the "Publish Pipeline Artifact" task in your Azure DevOps pipeline. This task allows you to specify the artifact name, description, and path, making it easy to identify and retrieve the artifact later.
Azure DevOps supports multiple artifact storage options, including Azure Blob Storage and Azure File Storage. This flexibility makes it easy to choose the storage solution that best fits your organization's needs.
By publishing and managing pipeline artifacts effectively, you can improve collaboration, reduce errors, and increase efficiency in your software development process.
Artifact Configuration
Artifact Configuration is where the magic happens. You can specify the name of the artifact to download, and if left empty, all associated artifacts will be downloaded.
Only files for that specific artifact are downloaded, so make sure the artifact exists, or the task will fail. File matching patterns are evaluated relative to the root of the artifact.
For example, if you want to download all *.js files from an artifact named WebApp, you can specify the artifact name and the pattern. The pattern is evaluated relative to the root of the artifact, so *.jar matches all files with a .jar extension at the root of the artifact.
You can also specify one or more file matching patterns that limit which files get downloaded. These patterns are used to filter the files to be downloaded.
Here's a breakdown of the key elements:
To ignore certain files when publishing artifacts, you can use the .artifactignore file. This file uses a similar syntax to .gitignore to specify which files should be ignored. The plus sign character + is not supported in URL paths and some builds metadata for package types such as Maven.
Artifact Management
Artifact management is a crucial aspect of Azure DevOps pipeline publish artifacts. You can download artifacts using YAML, the classic editor, or Azure CLI.
To download artifacts, you can specify the artifact name, and if left empty, all artifacts associated with the pipeline run will be downloaded. If the artifact doesn't exist, the task will fail.
You can use file matching patterns to limit which files get downloaded, and these patterns are evaluated relative to the root of the artifact. For example, the pattern *.jar matches all files with a .jar extension at the root of the artifact.
By default, files are downloaded to $(Pipeline.Workspace), and if an artifact name wasn't specified, a subdirectory will be created for each downloaded artifact. You can use matching patterns to limit which files get downloaded.
Here are the options for downloading artifacts:
- current: download artifacts produced by the current pipeline run.
- specific: download a specific artifact.
To download a pipeline artifact from a different project within your organization, make sure that you have the appropriate permissions configured for both the downstream project and the pipeline generating the artifact.
You can use the Download Pipeline Artifact task to download artifacts, and you can specify the artifact name, file matching patterns, and destination directory. The task will download the specified files and create a subdirectory for each downloaded artifact.
The Download Pipeline Artifact task has the following options:
- artifact: The name of the artifact to download. If left empty, all artifacts associated with the pipeline run will be downloaded.
- patterns: One or more file matching patterns that limit which files get downloaded.
- path: The destination directory. Can be relative or absolute path.
Make sure to check the permissions for the downstream project and the pipeline generating the artifact when downloading artifacts from a different project.
Pipeline Setup
To set up your pipeline, start by using the browser to create your YAML file in Azure Pipelines. This will store it in your repository for future use.
You can then install the Azure Pipeline extension in Visual Studio Code for syntax highlighting and code completion.
Navigate to Pipelines in the Azure DevOps sidebar and create your first pipeline. Choose Azure Repos Git to select where your code is located.
Select your repository and choose a starter pipeline, such as Node.js.
NuGet Package
To build the NuGet package, you'll need to add two tasks called NuGetCommand@2 and PublishBuildArtifacts@.
You can modify the pipeline using the Multi-Stage Pipeline UI experience, which is currently in Preview.
The NuGetCommand@2 task allows you to restore, pack, and publish NuGet packages to a NuGet feed, and it's used to create a package based on the information in the NUSPEC file.
The command attribute in this task is set to pack, which tells NuGet to create a package, and the packagesToPack attribute specifies the NUSPEC file to use.
The versioning scheme is defined using the versioningScheme and versionEnvVar attributes, and in this example, it's being handled via semantic versioning.
You must use byEnvVar as the versioning scheme and specify the variable that holds the current build version iteration for versionEnvVar.
The buildProperties attribute is used to replace the placeholder in the NUSPEC file with the build version.
To make the NuGet package available to the Deploy stage, you'll need to use the PublishBuildArtifacts@1 task.
This task takes an artifact build from the current stage and makes it available to other stages in the pipeline.
The pipeline is looking for a NuGet package in the artifact staging directory and saving it to the pipeline itself using the ContainerPublishLocation.
Once the package is available, all stages in the pipeline will have access to it.
You can publish the NuGet package from an Azure DevOps Release Pipeline using the NuGetCommand@2 task with the push command.
This task defines where to look for the package, the internal Artifacts feed, and the name of the internal Artifacts feed.
The name of the NuGet feed provided to the publishVstsFeed attribute will always be the name of your Azure DevOps organization.
Release and Deployment
To make your NuGet package available to the deploy stage, you can use the PublishBuildArtifacts@1 task. This task takes an artifact build from the current stages and makes them available to other stages in the pipeline.
Artifacts in the deploy stage are only downloaded automatically, and by default, they are saved to the $(Pipeline.Workspace) directory.
To stop artifacts from being downloaded automatically, you can add a download step and set its value to none.
You can also explicitly use the download step keyword or the Download Pipeline Artifact task to download artifacts in a regular build job.
Project Overview
In this project, we'll be publishing a build artifact to a NuGet feed using Azure DevOps pipeline. We'll be creating a NuGet package and delivering it to a client with PowerShell.
The project will be broken down into six main sections. Here's a brief overview of each:
- Ensuring the project is set up for Azure Artifacts
- Setting feed permissions to ensure the pipeline can publish to the NuGet feed
- Creating the NUSPEC definition file
- Creating the package and publishing it to the pipeline
- Adding the deployment task to the pipeline
- Running the pipeline to create the NuGet package and published to the Azure DevOps project’s Azure Artifacts feed
Azure Artifacts supports both org-based and project-based NuGet feeds. In this article, we'll be using the pre-created NuGet feed that comes with every organization.
Example and Selection
In Azure DevOps pipelines, you can copy and publish a script folder from your repo to the $(Build.ArtifactStagingDirectory) in one stage, and then download and run your script in the next stage.
You can download multiple artifacts at once by leaving the artifact name field empty and using file matching patterns to limit which files will be downloaded. ** is the default file matching pattern, which means all files in all artifacts will be downloaded.
Example
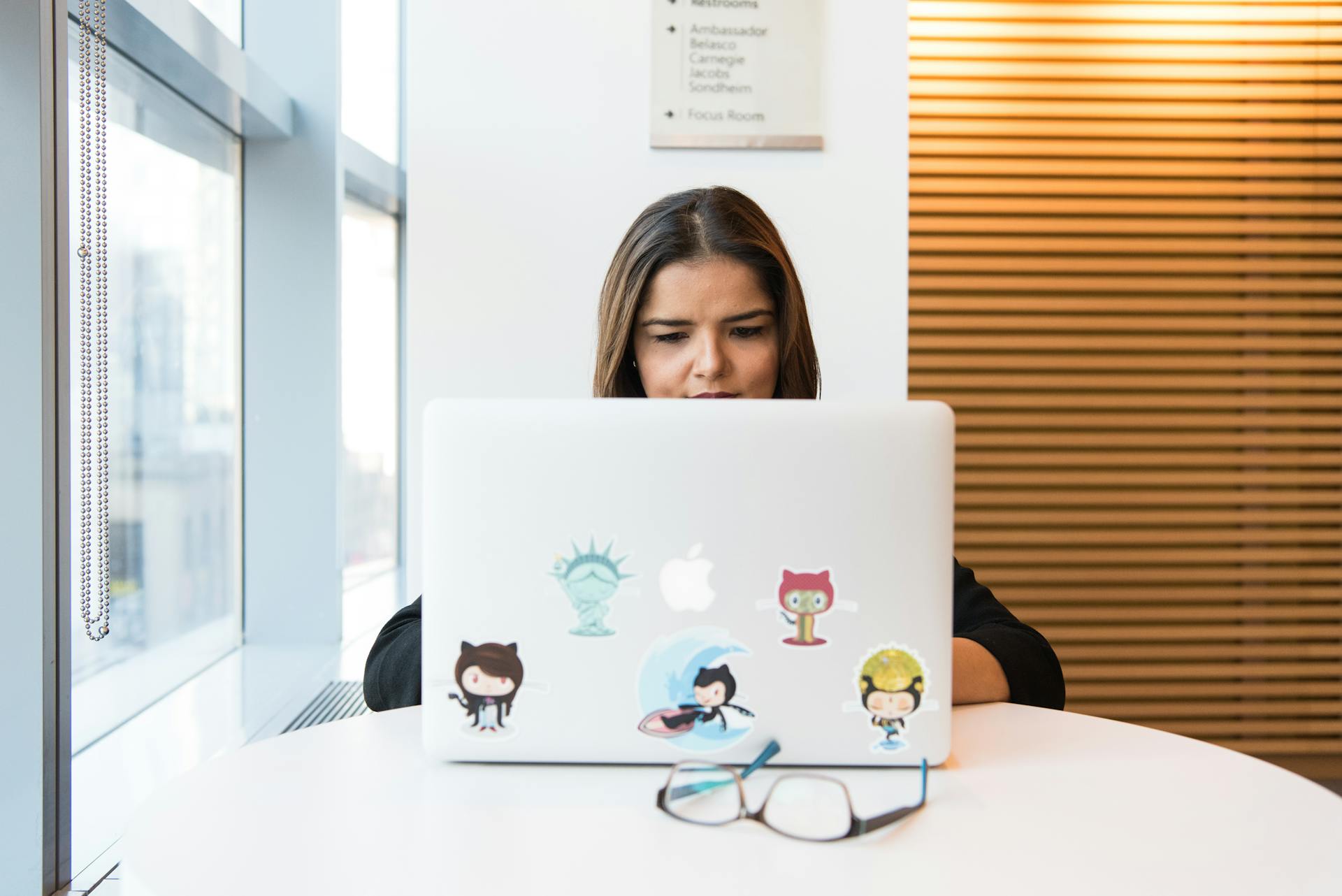
In the world of scripting, there are times when we need to copy and publish a script folder from our repository to a specific directory. This is exactly what we do in the first stage of a two-stage process.
We use the $(Build.ArtifactStagingDirectory) to store the script folder, which is a common practice in continuous integration and continuous deployment pipelines.
The script folder is copied from our repository, making it easily accessible for the next stage.
In the second stage, we download and run our script, which is a crucial step in automating tasks and workflows.
Selection
Selection is a crucial part of the download process. You can download multiple artifacts at once by leaving the artifact name field empty.
To limit which files will be downloaded, use file matching patterns. ** is the default file matching pattern, which means all files in all artifacts will be downloaded.
Sources
- https://learn.microsoft.com/en-us/azure/devops/pipelines/artifacts/pipeline-artifacts
- https://adamtheautomator.com/azure-devops-add-nuget-feed/
- https://technology.amis.nl/azure/azure-pipelines-publish-to-azure-artifacts/
- https://learn.microsoft.com/en-us/azure/devops/pipelines/tasks/reference/publish-build-artifacts-v1
- https://learn.microsoft.com/sl-si/azure/devops/pipelines/release/publish-pipeline-artifact
Featured Images: pexels.com