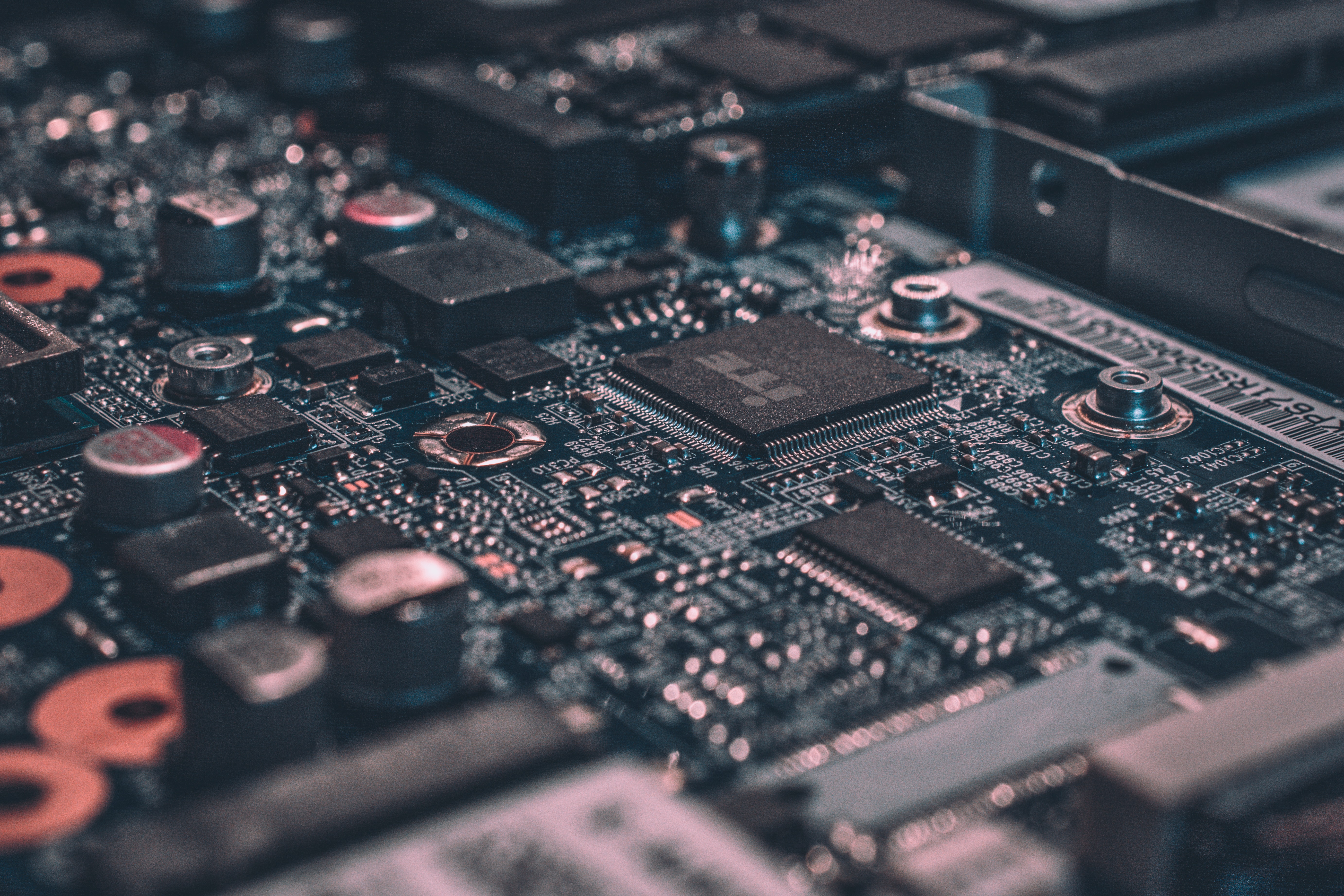
Azure Functions is a serverless compute service that allows you to run small pieces of code, or "functions", in response to events. This can be a game-changer for developers who want to build scalable and efficient applications.
Azure Functions supports containerization, which allows you to package your code and its dependencies into a single container that can be easily deployed and managed. This is a key feature of Azure Function Container.
One of the main benefits of using Azure Function Container is that it provides a consistent and reliable way to deploy your application, regardless of the underlying infrastructure. This is achieved through the use of Docker containers, which isolate your application from the host environment.
To get started with Azure Function Container, you'll need to create a new Azure Function project in your preferred programming language. This will give you a basic project structure and configuration that you can build upon.
Getting Started
To get started with Azure Function Container, you'll want to create your first containerized functions. You can start by creating a function app in a local Linux container. This will give you a foundation to build upon as you explore the world of Azure Function Container.
To create a function app in a local Linux container, simply follow the steps outlined in "Create a function app in a local Linux container". This will walk you through the process of setting up your environment and creating your first containerized function.
If you're looking to deploy functions to Azure Container Apps, you'll want to check out "Create your first containerized functions on Azure Container Apps". This will show you how to create and deploy functions to Azure Container Apps.
Alternatively, you can also create and deploy containerized functions to Azure Functions using "Create your first containerized Azure Functions". This will give you a step-by-step guide on how to create and deploy containerized functions to Azure Functions.
If you're interested in deploying functions to Azure Arc-enabled Kubernetes, you can follow the steps outlined in "Create your first containerized Azure Functions on Azure Arc (preview)". This will show you how to create and deploy containerized functions to Azure Arc-enabled Kubernetes.
Here's a quick summary of the options:
Creating and Deploying
Creating and deploying Azure Function containers can be a straightforward process. You can create a containerized function app from the command line and publish the image to a container registry.
To deploy your containerized function app on Azure Container Apps, you have two options for setting up continuous deployment from a source code repository: Azure Pipelines or GitHub Actions. However, you can't continuously deploy containers based on image changes in a container registry, so you must use these source-code based continuous deployment pipelines.
You can enable continuous deployment from a source code repository using the Azure Functions extension for Visual Studio Code, which creates your Dockerfile when it creates your code project. Alternatively, you can use the Azure Functions GitHub Action to automate your workflow to deploy Azure Functions in a customized image.
Running Locally
You can run your Azure Function locally in a container using Docker. To do this, you'll need a Functions-generated Dockerfile in your code project, which allows you to create a containerized function app on your local computer.
The Docker build command creates an image of your containerized functions from the project in the local directory. You can test the container image locally by mounting a volume and setting environment variables to enable console logging and use the container's special folder for secrets.
To test the function, you can use cURL and omit the function key to get a 401 Unauthorized response. Adding the function key as a request-header will resolve the issue. This approach is especially useful for troubleshooting purposes.
You can run an Azure Function inside a Container locally using the v2 Runtime and Container support, which are still in preview. This approach offers more control over the code and runtime compared to the Azure Portal, making it less likely for your Functions to break.
There are two deployment models for Azure Functions on Linux: Bring Your Own Container (BYOC) and Bring Only Your Code. To use the new tooling, ensure you have the latest versions of the Functions Runtime and Azure CLI tools.
Create Using
You can create a containerized function app using Azure Functions. The Functions team maintains a set of language-specific base images that you can use when creating containerized function apps.
To create a containerized function app, you can use the Azure Functions extension for Visual Studio Code, which creates a Dockerfile when it creates your code project. Alternatively, you can create a containerized function app from the command line using the Docker build command.
To create a containerized function app using Docker, you can use the following command:
```
docker build -t myfunctionapp .
```
This command creates an image of your containerized functions from the project in the local directory.
You can also use Azure Functions on Linux to create and run an Azure Function inside a container locally. This is still in preview, but it allows you to fully control the code and runtime, making it less likely for your Functions to break.
To use Azure Functions on Linux, you need to ensure you have the latest versions of the Functions Runtime and the Azure CLI tools. You can also use the Azure Cloud Shell, which is an equally powerful and always up-to-date browser-based tool that doesn't require installation.
Here are the two deployment models for Azure Functions on Linux:
- Bring your own Container (BYOC) - you create, deploy and run your own custom image
- Bring only your code - you deploy your code in the container already provisioned and managed by the Azure Functions platform
You can choose between these two models when creating the Function App for the first time through the Portal, CLI or PoSH.
Docker and Images
You can use Docker to create and manage images for your Azure Functions container. To get data about the image used for deployment, use the command az functionapp config container show.
The az functionapp config container set command allows you to change registry settings or update the image used for deployment. This command is useful for making updates to your image settings, such as the Image tag.
In the Azure portal, you can review information about the currently deployed image or change the deployment to a different image under Image settings. To make updates, modify any of the image settings and select Save.
You can also review information about the currently deployed image by selecting Container registry for Source under Settings in the Deployment center.
To create a customized Azure Functions image, use the Azure Functions GitHub Action. This action automates your workflow to deploy Azure Functions in a customized image.
You can generate a Dockerfile using the Functions tooling, which provides a Docker option that generates a Dockerfile with your functions code project. To create a Dockerfile, use the func init command with the --docker option when creating a new project.
Alternatively, you can add a Dockerfile to an existing project by using the --docker-only option when running the func init command in an existing project folder.
Here are the steps to create a Dockerfile:
1. Run the command func init --docker in the Terminal windows of an existing project folder.
2. Open the Dockerfile in the root folder of your project to review its contents.
By following these steps, you can create a Dockerfile that helps you manage your images for Azure Functions container.
GitHub and CI/CD
You can use GitHub Actions to automate the deployment of your Azure Functions container, making it easier to manage and update your application.
To deploy a customized Azure Functions image using GitHub Actions, you'll need to create a new workflow file in your repository, such as linux-container-functionapp-on-azure.yml, and commit it to your GitHub repository.
GitHub Actions supports continuous deployment from a source code repository, allowing you to automatically update your deployment whenever you update the image in the registry.
To enable continuous deployment, you'll need to use the az functionapp deployment container config command to get the webhook URL, and then update the DOCKER_ENABLE_CI application setting to true.
GitHub Actions for Customized Image Deployment
You can automate your workflow to deploy Azure Functions in a customized image with the Azure Functions GitHub Action.
This action is available for the Azure public cloud as well as Azure government clouds ('AzureUSGovernment' or 'AzureChinaCloud').
The definition of this GitHub Action is in action.yml.
To use this action, you'll need to paste the json response from Azure CLI to your GitHub Repository > Settings > Secrets > Add a new secret > AZURE_CREDENTIALS.
To set up continuous deployment from a source code repository, you can use either Azure Pipelines or GitHub Actions.
Here are the steps to enable continuous deployment using GitHub Actions:
1. Follow the tutorial Create a function on Linux using a custom image.
2. Customize your Dockerfile to ensure the function app dependencies can be resolved properly on runtime (e.g. npm install).
3. Use the linux-container-functionapp-on-azure.yml template as a reference, create a new workflow.yml file under your project ./github/workflows/.
4. Commit and push your project to GitHub repository, you should see a new GitHub Action initiated in Actions tab.
Note that you must use source-code based continuous deployment pipelines, as you can't continuously deploy containers based on image changes in a container registry.
Enable SSH Connections
Enabling SSH connections is a crucial step in securing communication between your container and a client. This allows you to connect to your container using App Service Advanced Tools (Kudu) for easy management.
Azure Functions provides a base image with SSH already enabled, making it easy to connect to your container. You just need to edit your Dockerfile, rebuild, and redeploy the image.
You can then connect to the container through the Advanced Tools (Kudu). This is particularly useful for Azure Container Apps hosting of Azure Functions, as well as Kubernetes-based serverless hosting.
Frequently Asked Questions
Does Azure Functions use containers?
Yes, Azure Functions uses containers to run your code project on Linux, which are automatically created and managed by Functions. This makes it easy to deploy and run your code in a containerized environment.
What is Azure container?
Azure Container is a scalable and efficient way to run batch jobs, allowing you to process data without managing environments and dependencies. It's a key component of Azure's dynamic compute options, enabling seamless data ingestion and storage.
Sources
- https://martinbjorkstrom.com/posts/2020-02-12-testing-protected-azure-functions
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-how-to-custom-container
- https://learn.microsoft.com/en-us/azure/azure-functions/container-concepts
- https://github.com/Azure/functions-container-action
- https://cmatskas.com/running-azure-functions-anywhere-with-the-power-of-containers/
Featured Images: pexels.com