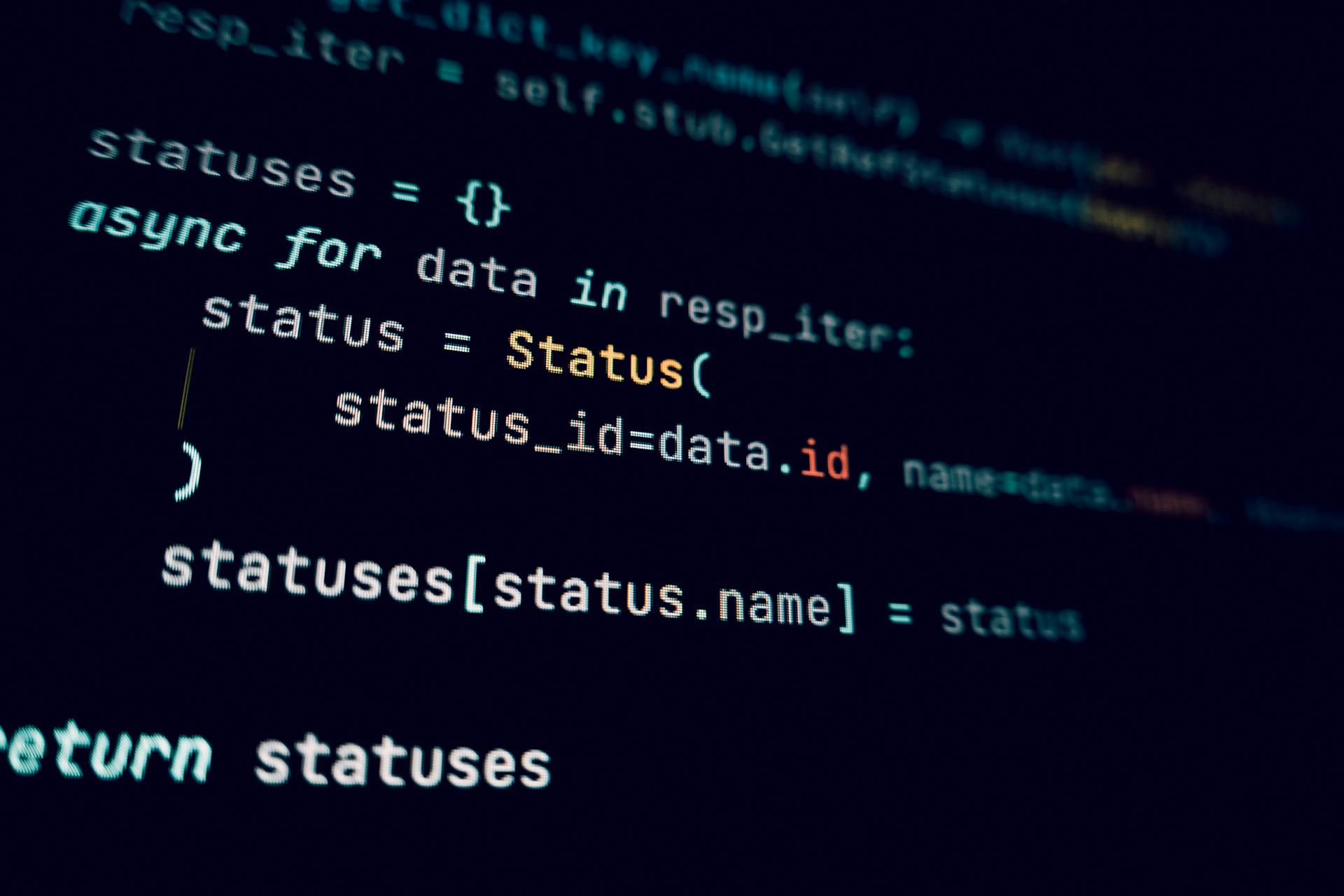
To send an email from Azure Function using SendGrid, you'll need to create a SendGrid account and obtain your API key. This key will be used to authenticate your Azure Function and allow it to send emails through SendGrid.
First, ensure you have the SendGrid NuGet package installed in your Azure Function project. This package provides the necessary functionality to interact with the SendGrid API.
To configure SendGrid in your Azure Function, add the following code to your function's configuration: `SendGridClient sendGridClient = new SendGridClient("YOUR_API_KEY");`. Replace `YOUR_API_KEY` with your actual SendGrid API key.
A different take: Azure Send Email
Prerequisites
Before we dive into sending emails with Azure Functions, make sure you have the following prerequisites in place.
You'll need an Azure Function App (PowerShell) set up and ready to go. This is the foundation of our project.
To create an HTTPTrigger Function, you'll need a PowerShell Azure Function. This type of function will allow us to trigger an email send when a request is made.
Curious to learn more? Check out: Azure Powershell vs Azure Cli
Next, you'll need to create an Azure SendGrid Account. This will be used to send emails from your Azure Function.
In your local.settings.json file, add a variable to contain your SendGrid API key. In the example, the variable was named 'SendGrid'.
Here's a quick rundown of the prerequisites:
- Azure Function App (PowerShell)
- PowerShell Azure Function (HTTPTrigger Function)
- Azure SendGrid Account
Don't forget to add the SendGrid API key to your local.settings.json file.
Configuring SendGrid
Configuring SendGrid is a crucial step in setting up an Azure Function to send emails.
To start, you need to add the SendGrid Output Binding to your Azure Function. This involves right-clicking on your Function and selecting Add Binding, then selecting the Application Setting 'SendGrid' that contains your SendGrid API key.
The SendGrid Output Binding will then be added to your Azure Function, allowing you to configure it further.
You'll need to leave the "To address", "From address", "Message Subject", and "Message Text" fields blank, as we'll generate these in the Azure Function.
To use SendGrid from a PowerShell Azure Function, you'll need to use the SendGrid Output Binding that you've already configured. You can do this by creating variables for the SendGrid API key, "sendGridToAddress", and "sendGridFromAddress" in your Application Settings.
The format of the email message output object to send from the Azure Function to SendGrid is straightforward. You'll need to pass the message's destination, origin, subject, and content.
To generate the message object, you can use the Push-OutputBinding command along with the object name you configured when creating the binding (message) and the variable containing the message from above ($mail) converted to JSON.
When converting the PowerShell Message Object ($mail) to JSON, the values need to be strings for the conversion and the push to SendGrid to be successful.
To integrate SendGrid with your Azure account, create a SendGrid account and go to settings > API Keys. Click create an API Key and give it a name. Restricted access with Mail Send is all we need for permissions. Copy your API key somewhere safe.
The output settings will now be added to the function.json file that Azure created for you if you did a Timer Trigger app. You can also find the schedule using a Cron pattern in there.
On a similar theme: Azure Function Local Settings Json
Here's a step-by-step guide to integrating SendGrid:
1. Create a SendGrid account and go to settings > API Keys.
2. Click create an API Key and give it a name.
3. Restricted access with Mail Send is all we need for permissions.
4. Copy your API key somewhere safe.
5. In the SendGrid API Key drop down menu, select SendGridKey.
6. Fill out the From address & subject as well if you want defaults for the function.
7. Make sure to Save.
Note: Make sure to use Newtonsoft.Json when serializing the SendGrid Personalization class to avoid issues with property names.
Worth a look: Azure Key Vault Tutorial C#
Sending Email with SendGrid
To send emails with SendGrid, you need to add the Output Binding to your Azure Function. This can be done by right-clicking on your Function and selecting Add Binding, then selecting the Application Setting 'SendGrid' that contains your SendGrid API key.
The SendGrid Output Binding will then be added to your Azure Function. You'll also need to configure the From address, To address, Message Subject, and Message Text in the binding settings.
To use the SendGrid Output Binding, you'll need to create a variable for the SendGrid API key and other Application Settings for the from and to addresses. The format of the email message output object to send from the Azure Function to SendGrid includes the from and to addresses, the subject of the email message, and the content.
When converting the message object to JSON, the values need to be strings for the conversion and the push to SendGrid to be successful. You can achieve this by using the Push-OutputBinding along with the object name you configured when creating the binding and the variable containing the message from above.
Here are the steps to add the SendGrid Output Binding:
- Right-click on your Function and select Add Binding
- Select the Application Setting 'SendGrid' that contains your SendGrid API key
- Press enter to leave blank "To address", "From address", "Message Subject" and "Message Text" as we'll generate these in the Azure Function
The SendGrid Output Binding will then be added to your Azure Function.
Testing Integration
Testing Integration is a crucial step in verifying that your Azure Function can send emails successfully.
To test end-to-end functionality, you need to complete your Azure Function and trigger it with dynamic data, specifically the subject and content.
Triggering your Azure Function from within a PowerShell script in VSCode involves using the HTTP Post request on the HTTPTrigger based Function.
The Azure Function should leverage the Function Application Settings for the "to" and "from" address, which are essential for sending emails.
After triggering your Azure Function, wait a few minutes for the email to arrive in the destination mailbox.
A working PowerShell Azure Function with SendGrid and HTTPResponse Output Bindings can be created using the provided run.ps1 script, which also lacks error handling.
Sources
- https://simonholman.dev/sending-email-from-azure-functions-with-sendgrid
- https://blog.darrenjrobinson.com/using-the-azure-functions-sendgrid-output-binding-with-powershell/
- https://mattferderer.com/scrape-a-website-and-send-an-email-with-azure-functions
- https://faun.pub/sending-automated-emails-on-azure-a-step-by-step-guide-c881eea30efe
- https://www.socketlabs.com/blog/how-to-send-email-from-an-azure-web-app/
Featured Images: pexels.com