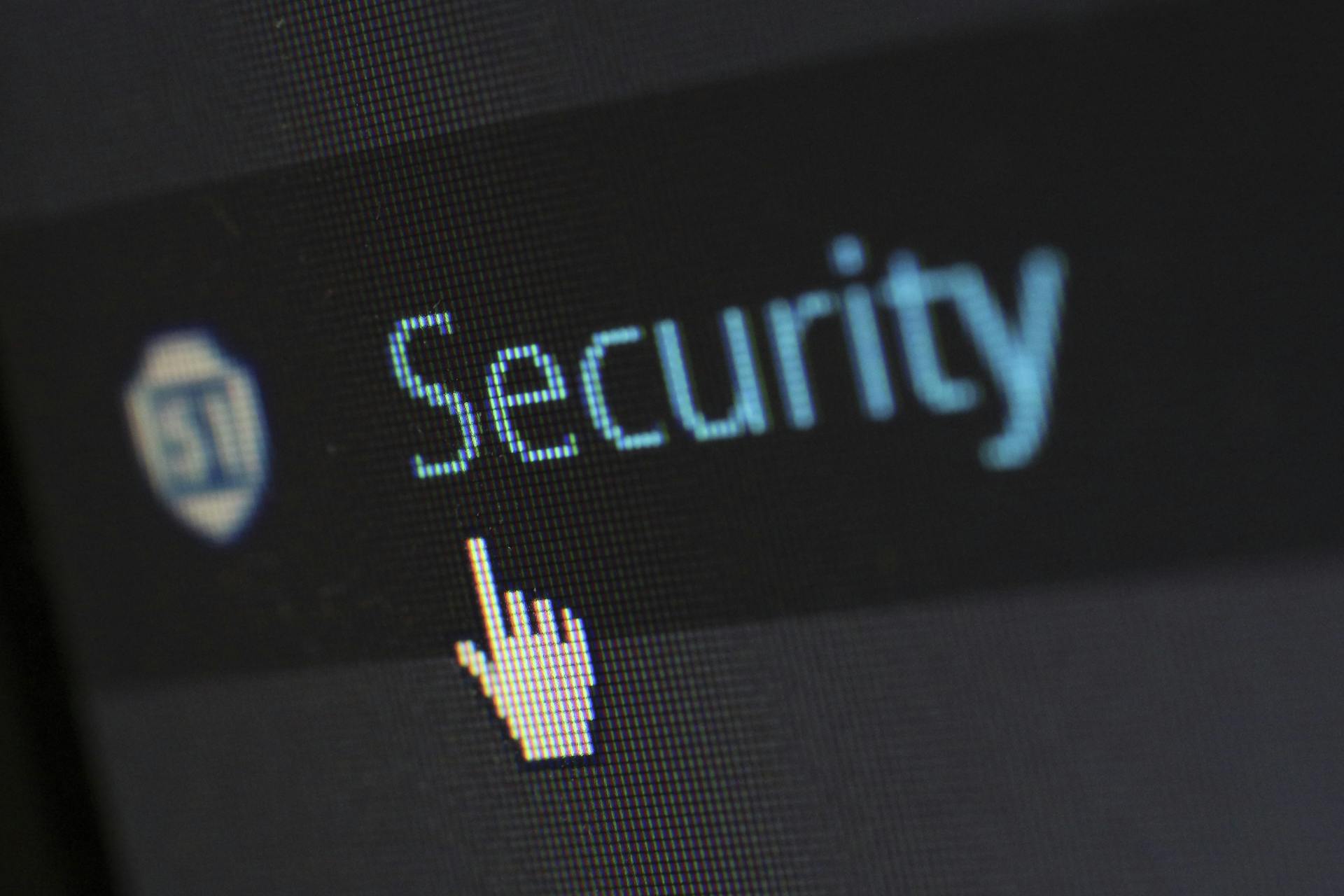
Azure Functions can be authenticated with Identity Providers and Microsoft Graph, allowing for secure access to your functions.
To authenticate with Identity Providers, you can use Azure Active Directory (AAD) B2C, which supports various identity providers such as Google, Facebook, and more.
AAD B2C provides a user flow that can be customized to fit your application's needs.
Azure Functions can also be authenticated with Microsoft Graph, which provides a unified API endpoint for accessing Azure services, including Azure Active Directory.
You might enjoy: Azure Identity
Azure Function Authentication Basics
Protecting your Azure Functions is crucial to prevent unauthorized access. You can start by following Step 2: Protect Azure Functions with Authorization.
This step is essential to ensure that only authorized users can access your functions. The example of a secured Azure Function in C# shows how this can be achieved.
To implement authorization, you need to use authentication mechanisms such as Azure Active Directory (AAD) or Azure Active Directory B2C (Azure AD B2C). These services can help you manage user identities and access control.
By following the example of a secured Azure Function in C#, you can see how to use these authentication mechanisms to secure your functions. This is a great starting point for implementing authentication in your Azure Functions.
A fresh viewpoint: Azure Active Directory Tutorial
Authentication Setup
To set up authentication for your Azure Functions, you'll need to configure the authentication settings in the Azure portal. This involves selecting the identity provider, such as Microsoft, and configuring the authentication flow.
You can either create a new registration or use an existing one. To create a new registration, select "Pick an existing app registration" and choose an app registration from the dropdown. Alternatively, you can select "Provide the details of an existing app registration" and enter the details of an existing app registration.
To create a new user flow, enter a name for the user flow, select the sign-in method for your external users, and select "Create" to create the user flow.
Once you've created the user flow, you can configure the function app for authentication. This involves adding a userId property to the SignalRConnectionInfo binding with the value {headers.x-ms-client-principal-name}. This sets the username of the SignalR client to the name of the authenticated user.
For another approach, see: Create Sample Azure Function for .net Frame Work
You can also enable App Service authentication in the Azure portal by going to the resource page of your function app, selecting "Settings" > "Authentication", and selecting "Add identity provider". Select "Microsoft" as the identity provider and follow the prompts to create the app registration.
Here are the steps to configure authentication for your Azure Function App:
1. Go to the Azure portal and navigate to your Azure Function App.
2. Click on "Authentication" in the left-hand menu.
3. Select "Microsoft" as the identity provider.
4. Configure the authentication flow settings as desired.
5. Click on "Save" to enable authentication for your Azure Function App.
Note: You can customize the authentication flow settings later by choosing "Edit" next to "Authentication settings".
On a similar theme: Azure Active Directory Identity Protection
Identity Providers
To set up identity providers in Azure Functions authentication, you can select either workforce or external configuration.
If you choose workforce configuration, you can add Microsoft Graph permissions needed by the application after selecting Next: Permissions.
These permissions will be added to the app registration, but you can also change them later.
Check this out: Azure Web App Permissions
Add Identity Provider
Adding an identity provider is a straightforward process. You can select either workforce configuration or external configuration to begin.
If you choose workforce configuration, you can proceed to add Microsoft Graph permissions needed by the application. These permissions will be added to the app registration.
You can change them later if needed. If you selected external configuration, you can add Microsoft Graph permissions later.
The provider will be listed on the Authentication screen, from where you can edit or delete the provider configuration.
Migrating to Microsoft Graph
Migrating to Microsoft Graph can be a straightforward process, especially with the guidance provided by Microsoft Entra. You may need to make changes to your configuration of App Service authentication.
If your app code has called Azure AD Graph to check group membership as part of an authorization filter in a middleware pipeline, it's time to move to Microsoft Graph. This will ensure your app remains compatible with the latest Azure AD Graph deprecation process.
A different take: Azure Graph
To start, you'll need to add Microsoft Graph permissions to your app registration. This will replace the deprecated Azure AD Graph permissions.
Once you've made this change, App Service Authentication will no longer request permissions to the Azure AD Graph, and instead will get a token for the Microsoft Graph. Any use of that token from your application code will also need to be updated.
Here are the resources you'll need to complete this process:
- App Service Authentication / Authorization overview.
- Tutorial: Authenticate and authorize users end-to-end in Azure App Service
Authorization and Policy
Authorization and Policy is a crucial aspect of Azure Functions authentication. Built-in authorization policies can be enabled using the App Service authentication V2 API, allowing for restrictions on access to be applied.
The Microsoft Entra identity provider configuration has a validation section that includes a defaultAuthorizationPolicy object, which can be used to configure access requirements. This object has several properties, including allowedApplications, allowedPrincipals, and identities.
You can configure the allowedApplications property with a list of client IDs representing the client resource that is calling into the app. The allowedPrincipals property can be used to specify a grouping of checks that determine if the principal represented by the incoming request may access the app. The identities property can be used to specify an allowlist of string object IDs representing users or applications that have access.
Here are the possible configuration options for the defaultAuthorizationPolicy object:
Built-in Authorization Policy
You can use a built-in authorization policy to restrict access to your Azure Functions app. This policy is configured within the API object, specifically in the Microsoft Entra identity provider configuration's validation section.
The defaultAuthorizationPolicy object is a grouping of requirements that must be met in order to access the app. Access is granted based on a logical AND over each of its configured properties.
The allowedApplications property is an allowlist of string application client IDs representing the client resource that is calling into the app. Only tokens obtained by an application specified in the list will be accepted.
The allowedPrincipals property is a grouping of checks that determine if the principal represented by the incoming request may access the app. Satisfaction of allowedPrincipals is based on a logical OR over its configured properties.
Here's a breakdown of the allowedPrincipals properties:
Additionally, some checks can be configured through an application setting, regardless of the API version being used. The WEBSITE_AUTH_AAD_ALLOWED_TENANTS application setting can be configured with a comma-separated list of up to 10 tenant IDs to require that the incoming token is from one of the specified tenants.
The WEBSITE_AUTH_AAD_REQUIRE_CLIENT_SERVICE_PRINCIPAL application setting can be configured to "true" or "1" to require the incoming token to include an oid claim. This setting is ignored and treated as true if allowedPrincipals.identities has been configured.
Requests that fail these built-in checks are given an HTTP 403 Forbidden response.
You might enjoy: Azure Storage Sas Token
Validate from Application Code
You can work directly with the underlying access token from the injected x-ms-token-aad-access-token header. This allows you to perform validations from your application code.
To do this, you'll need to access the access token that's been injected into your application. The x-ms-token-aad-access-token header is a key part of this process.
You can use the access token to validate user identities and permissions in your application. This is especially useful when working with Azure Active Directory.
Here's a simple example of how you can use the access token to validate a user's identity:
- Select the access token from the x-ms-token-aad-access-token header
- Use the access token to validate the user's identity in your application code
By working directly with the access token, you can simplify your application's authentication and authorization process.
Service-to-Service Calls
To make service-to-service calls in Azure Functions, you can create a daemon client application. This allows your client application to acquire a token to call an App Service or Function app on behalf of the client app itself.
To do this, you'll need to register your app in the Microsoft Entra portal. From the portal menu, select Microsoft Entra, then App registrations > New registration. Enter a Name for your app registration and leave the Redirect URI field empty, as daemon applications don't need it.
Check this out: How to Enable Mfa in Azure Portal
You'll also need to create a client secret by going to Certificates & secrets > Client secrets > New client secret. Enter a description and expiration, then select Add. Copy the client secret value, as it won't be shown again.
To request an access token, set the resource parameter to the Application ID URI of the target app. This will give you an access token that you can present to the target app using the standard OAuth 2.0 Authorization header.
If you also want to enforce authorization to allow only certain client applications, you'll need to define an App Role in the manifest of the app registration representing the App Service or Function app you want to protect. You can do this by going to API permissions > Add a permission > My APIs and selecting the app registration you created earlier.
If this caught your attention, see: Azure Keyvault Secrets
Best Practices and Security
To keep your Azure Function App secure, it's essential to follow best practices for authentication. Configure each App Service app with its own app registration in Microsoft Entra to ensure unique permissions.
This practice helps prevent issues from affecting the production app when you're testing new code. Separate app registrations for separate deployment slots can save you a lot of headache.
Giving each App Service app its own permissions and consent is also crucial. This allows you to define detailed access policies and restrict access to specific users or groups.
To implement fine-grained access control, use the Microsoft Entra (Azure AD) tenant to configure authentication settings. This will help you centralize identity management and simplify access control to functions and other Azure resources.
Here are some key best practices to keep in mind:
- Configure each App Service app with its own app registration in Microsoft Entra.
- Give each App Service app its own permissions and consent.
- Avoid permission sharing between environments by using separate app registrations for separate deployment slots.
Middleware and Authorization
Middleware in Azure Functions can be used to implement cross-cutting concerns such as authentication and authorization.
In Azure Functions, middleware is defined as a class that can wrap code around all of your functions, current and future. This allows you to run code before/after a function executes, or even decide not to call the function at all.
Middleware are registered in the Program class, where you can start with an empty middleware class.
To implement authentication middleware, you can check the request for an access token and validate it. If the token is valid, you can assign the user to the request and continue the request. If the token is not found or is invalid, you can return a 401 Unauthorized status code.
Here are the steps to implement authentication middleware:
- Check the request for an access token and validate it.
- If the token is valid, assign the user to the request and continue the request.
- If the token is not found or is invalid, return a 401 Unauthorized status code.
To get the access token, you can deserialize the headers as a JSON string from the "binding data" dictionary. From there, you can get the access token from the Authorization header.
Here are the classes and methods you can use to implement authentication middleware:
- `SetHttpResponseStatusCode`: an extension method to set the status code.
- `TokenValidator`: a class to validate the token.
- `ConfigurationManager`: a class to load the configuration metadata from the "well known" endpoint.
Example code for implementing authentication middleware can be found in the sample here.
Deployment and Testing
You'll need to deploy your function app and chat app to Azure to enable authentication and private messaging. This involves uploading your code to Azure's cloud platform.
To deploy, follow these steps:
- Deploy your Azure Function App with the changes made in the previous steps.
- Access the secured function URL in your web browser or use tools like cURL or Postman.
- Sign in to Microsoft Entra if you're not already authenticated.
- Once authenticated, you should be able to access the function.
Deploy to
Deploying your function app and chat app to Azure is a crucial step in making them accessible to a wider audience. You've probably been running them locally, but now it's time to take the next step.
To deploy to Azure, you'll need to enable authentication. This allows users to sign in and access your app securely. Authentication is a must-have for private messaging, which is a key feature of your chat app.
Deploying to Azure also requires you to set up your function app and chat app to work together seamlessly. This means configuring your Azure resources to support your app's functionality.
Testing the Secured
Testing the Secured Function is a crucial step in ensuring it's working as expected.
To deploy your Azure Function App, you'll need to follow the steps outlined in the documentation. Deploy your Azure Function App with the changes made in Step 2.
Access the secured function URL in your web browser or use tools like cURL or Postman. If not already authenticated, Microsoft Entra will prompt you to sign in.
Once authenticated, you should be able to access the function.
Here's a step-by-step guide to testing the secured function:
- Deploy your Azure Function App with the changes made in Step 2.
- Access the secured function URL in your web browser or use tools like cURL or Postman.
- If not already authenticated, Microsoft Entra will prompt you to sign in.
- Once authenticated, you should be able to access the function.
Monitoring and Logging
Monitoring and Logging is crucial for secured Azure Functions. Azure provides robust logging and diagnostic capabilities to monitor security-related events.
In the Azure portal, navigate to Application Insights for your Function App. This is where you'll find valuable insights into your app's performance and security.
To get started, configure logging for authentication requests and other metrics. This will help you track important events and identify potential security issues.
Use Azure Monitor and Log Analytics to track security incidents, such as failed login attempts and suspicious activity. This tool is powerful and can help you stay on top of your app's security.
Set up alerts for specific thresholds, like multiple failed login attempts from the same IP. This will ensure you're notified when something unusual happens, so you can take action quickly.
Here's a quick rundown of the steps to follow:
- Access Application Insights in the Azure portal.
- Configure logging for authentication requests and other metrics.
- Use Azure Monitor and Log Analytics to track security incidents.
- Set up alerts for specific thresholds.
Frequently Asked Questions
What are the different types of authentication in Azure?
Azure offers three main types of authentication: username and password, multi-factor authentication, and federated authentication, each providing a different level of security. Multi-factor authentication adds an extra layer of protection with additional identity verification.
What is the authorization level in Azure Functions?
In Azure Functions, the authorization level determines which API keys are required to invoke a function. This setting controls access to your function and helps secure it from unauthorized calls.
Sources
- https://learn.microsoft.com/en-us/azure/app-service/configure-authentication-provider-aad
- https://rakhesh.com/azure/authenticating-against-azure-functions-using-azure-ad/
- https://joonasw.net/view/azure-ad-jwt-authentication-in-net-isolated-process-azure-functions
- https://learn.microsoft.com/en-us/azure/azure-signalr/signalr-tutorial-authenticate-azure-functions
- https://blog.nashtechglobal.com/securing-azure-functions-with-azure-active-directory/
Featured Images: pexels.com